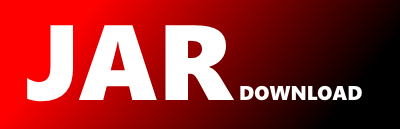
info.archinnov.achilles.generated.dsl.EntityWithComplexIndices_Update Maven / Gradle / Ivy
package info.archinnov.achilles.generated.dsl;
import com.datastax.driver.core.querybuilder.NotEq;
import com.datastax.driver.core.querybuilder.QueryBuilder;
import com.datastax.driver.core.querybuilder.Update;
import com.google.common.collect.Sets;
import info.archinnov.achilles.generated.meta.entity.EntityWithComplexIndices_AchillesMeta;
import info.archinnov.achilles.internals.entities.EntityWithComplexIndices;
import info.archinnov.achilles.internals.metamodel.AbstractEntityProperty;
import info.archinnov.achilles.internals.options.Options;
import info.archinnov.achilles.internals.query.dsl.update.AbstractUpdate;
import info.archinnov.achilles.internals.query.dsl.update.AbstractUpdateColumns;
import info.archinnov.achilles.internals.query.dsl.update.AbstractUpdateEnd;
import info.archinnov.achilles.internals.query.dsl.update.AbstractUpdateFrom;
import info.archinnov.achilles.internals.query.dsl.update.AbstractUpdateWhere;
import info.archinnov.achilles.internals.runtime.RuntimeEngine;
import info.archinnov.achilles.type.SchemaNameProvider;
import info.archinnov.achilles.validation.Validator;
import java.lang.Class;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
import org.apache.commons.lang3.ArrayUtils;
public final class EntityWithComplexIndices_Update extends AbstractUpdate {
protected final EntityWithComplexIndices_AchillesMeta meta;
protected final Class entityClass = EntityWithComplexIndices.class;
public EntityWithComplexIndices_Update(RuntimeEngine rte, EntityWithComplexIndices_AchillesMeta meta) {
super(rte);
this.meta = meta;
}
/**
* Generate an UPDATE FROM ... */
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateFrom fromBaseTable() {
final String currentKeyspace = meta.getKeyspace().orElse("unknown_keyspace_for_" + meta.entityClass.getCanonicalName());
final Update.Where where = QueryBuilder.update(currentKeyspace, meta.getTableOrViewName()).where();
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateFrom(where);
}
/**
* Generate an UPDATE FROM ... using the given SchemaNameProvider */
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateFrom from(final SchemaNameProvider schemaNameProvider) {
final String currentKeyspace = lookupKeyspace(schemaNameProvider, meta.entityClass);
final String currentTable = lookupTable(schemaNameProvider, meta.entityClass);
final Update.Where where = QueryBuilder.update(currentKeyspace, currentTable).where();
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateFrom(where);
}
public class EntityWithComplexIndices_UpdateColumns extends AbstractUpdateColumns {
EntityWithComplexIndices_UpdateColumns(Update.Where where) {
super(where);
}
/**
* Generate an UPDATE FROM ... SET simpleindex = ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns simpleIndex_Set(final String simpleIndex) {
where.with(QueryBuilder.set("simpleindex", QueryBuilder.bindMarker("simpleindex")));
boundValues.add(simpleIndex);
encodedValues.add(meta.simpleIndex.encodeFromJava(simpleIndex));
return this;
}
/**
* Generate an UPDATE FROM ... SET collectionindex = collectionindex + [?] */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_AppendTo(final String collectionIndex_element) {
where.with(QueryBuilder.appendAll("collectionindex", QueryBuilder.bindMarker("collectionindex")));
boundValues.add(Arrays.asList(collectionIndex_element));
encodedValues.add(meta.collectionIndex.encodeFromJava(Arrays.asList(collectionIndex_element)));
return this;
}
/**
* Generate an UPDATE FROM ... SET collectionIndex = collectionIndex + ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_AppendAllTo(final List collectionIndex) {
where.with(QueryBuilder.appendAll("collectionindex", QueryBuilder.bindMarker("collectionindex")));
boundValues.add(collectionIndex);
encodedValues.add(meta.collectionIndex.encodeFromJava(collectionIndex));
return this;
}
/**
* Generate an UPDATE FROM ... SET collectionIndex = [?] + collectionIndex */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_PrependTo(final String collectionIndex_element) {
where.with(QueryBuilder.prependAll("collectionindex", QueryBuilder.bindMarker("collectionindex")));
boundValues.add(Arrays.asList(collectionIndex_element));
encodedValues.add(meta.collectionIndex.encodeFromJava(Arrays.asList(collectionIndex_element)));
return this;
}
/**
* Generate an UPDATE FROM ... SET collectionIndex = ? + collectionIndex */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_PrependAllTo(final List collectionIndex) {
where.with(QueryBuilder.prependAll("collectionindex", QueryBuilder.bindMarker("collectionindex")));
boundValues.add(collectionIndex);
encodedValues.add(meta.collectionIndex.encodeFromJava(collectionIndex));
return this;
}
/**
* Generate an UPDATE FROM ... SET collectionIndex[index] = ? */
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_SetAtIndex(final int index, final String collectionIndex_element) {
where.with(QueryBuilder.setIdx("collectionindex", index, QueryBuilder.bindMarker("collectionindex")));
boundValues.add(collectionIndex_element);
encodedValues.add(meta.collectionIndex.valueProperty.encodeFromJava(collectionIndex_element));
return this;
}
/**
* Generate an UPDATE FROM ... SET collectionIndex[index] = null */
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_RemoveAtIndex(final int index) {
where.with(QueryBuilder.setIdx("collectionindex", index, QueryBuilder.bindMarker("collectionindex")));
boundValues.add(null);
encodedValues.add(null);
return this;
}
/**
* Generate an UPDATE FROM ... SET collectionIndex = collectionIndex - [?] */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_RemoveFrom(final String collectionIndex_element) {
where.with(QueryBuilder.discardAll("collectionindex", QueryBuilder.bindMarker("collectionindex")));
boundValues.add(Arrays.asList(collectionIndex_element));
encodedValues.add(meta.collectionIndex.encodeFromJava(Arrays.asList(collectionIndex_element)));
return this;
}
/**
* Generate an UPDATE FROM ... SET collectionIndex = collectionIndex - ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_RemoveAllFrom(final List collectionIndex) {
where.with(QueryBuilder.discardAll("collectionindex", QueryBuilder.bindMarker("collectionindex")));
boundValues.add(collectionIndex);
encodedValues.add(meta.collectionIndex.encodeFromJava(collectionIndex));
return this;
}
/**
* Generate an UPDATE FROM ... SET collectionIndex = ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_Set(final List collectionIndex) {
where.with(QueryBuilder.set("collectionindex", QueryBuilder.bindMarker("collectionindex")));
boundValues.add(collectionIndex);
encodedValues.add(meta.collectionIndex.encodeFromJava(collectionIndex));
return this;
}
/**
* Generate an UPDATE FROM ... SET fullindexoncollection = fullindexoncollection + {?} */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns fullIndexOnCollection_AddTo(final String fullIndexOnCollection_element) {
where.with(QueryBuilder.addAll("fullindexoncollection", QueryBuilder.bindMarker("fullindexoncollection")));
boundValues.add(Sets.newHashSet(fullIndexOnCollection_element));
encodedValues.add(meta.fullIndexOnCollection.encodeFromJava(Sets.newHashSet(fullIndexOnCollection_element)));
return this;
}
/**
* Generate an UPDATE FROM ... SET fullIndexOnCollection = fullIndexOnCollection + ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns fullIndexOnCollection_AddAllTo(final Set fullIndexOnCollection) {
where.with(QueryBuilder.addAll("fullindexoncollection", QueryBuilder.bindMarker("fullindexoncollection")));
boundValues.add(fullIndexOnCollection);
encodedValues.add(meta.fullIndexOnCollection.encodeFromJava(fullIndexOnCollection));
return this;
}
/**
* Generate an UPDATE FROM ... SET fullIndexOnCollection = fullIndexOnCollection - {?} */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns fullIndexOnCollection_RemoveFrom(final String fullIndexOnCollection_element) {
where.with(QueryBuilder.removeAll("fullindexoncollection", QueryBuilder.bindMarker("fullindexoncollection")));
boundValues.add(Sets.newHashSet(fullIndexOnCollection_element));
encodedValues.add(meta.fullIndexOnCollection.encodeFromJava(Sets.newHashSet(fullIndexOnCollection_element)));
return this;
}
/**
* Generate an UPDATE FROM ... SET fullIndexOnCollection = fullIndexOnCollection - ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns fullIndexOnCollection_RemoveAllFrom(final Set fullIndexOnCollection) {
where.with(QueryBuilder.removeAll("fullindexoncollection", QueryBuilder.bindMarker("fullindexoncollection")));
boundValues.add(fullIndexOnCollection);
encodedValues.add(meta.fullIndexOnCollection.encodeFromJava(fullIndexOnCollection));
return this;
}
/**
* Generate an UPDATE FROM ... SET fullIndexOnCollection = ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns fullIndexOnCollection_Set(final Set fullIndexOnCollection) {
where.with(QueryBuilder.set("fullindexoncollection", QueryBuilder.bindMarker("fullindexoncollection")));
boundValues.add(fullIndexOnCollection);
encodedValues.add(meta.fullIndexOnCollection.encodeFromJava(fullIndexOnCollection));
return this;
}
/**
* Generate an UPDATE FROM ... SET indexonmapkey[?] = ? */
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapKey_PutTo(final String indexOnMapKey_key, final String indexOnMapKey_value) {
where.with(QueryBuilder.put("indexonmapkey", QueryBuilder.bindMarker("indexOnMapKey_key"), QueryBuilder.bindMarker("indexOnMapKey_value")));
boundValues.add(indexOnMapKey_key);
boundValues.add(indexOnMapKey_value);
encodedValues.add(meta.indexOnMapKey.keyProperty.encodeFromJava(indexOnMapKey_key));
encodedValues.add(meta.indexOnMapKey.valueProperty.encodeFromJava(indexOnMapKey_value));
return this;
}
/**
* Generate an UPDATE FROM ... SET indexOnMapKey = indexOnMapKey + ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapKey_AddAllTo(final Map indexOnMapKey) {
where.with(QueryBuilder.addAll("indexonmapkey", QueryBuilder.bindMarker("indexonmapkey")));
boundValues.add(indexOnMapKey);
encodedValues.add(meta.indexOnMapKey.encodeFromJava(indexOnMapKey));
return this;
}
/**
* Generate an UPDATE FROM ... SET indexOnMapKey[?] = null */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapKey_RemoveByKey(final String indexOnMapKey_key) {
where.with(QueryBuilder.put("indexonmapkey", QueryBuilder.bindMarker("indexOnMapKey_key"), QueryBuilder.bindMarker("indexOnMapKey_value")));
boundValues.add(indexOnMapKey_key);
boundValues.add(null);
encodedValues.add(meta.indexOnMapKey.keyProperty.encodeFromJava(indexOnMapKey_key));
encodedValues.add(null);
return this;
}
/**
* Generate an UPDATE FROM ... SET indexOnMapKey = ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapKey_Set(final Map indexOnMapKey) {
where.with(QueryBuilder.set("indexonmapkey", QueryBuilder.bindMarker("indexonmapkey")));
boundValues.add(indexOnMapKey);
encodedValues.add(meta.indexOnMapKey.encodeFromJava(indexOnMapKey));
return this;
}
/**
* Generate an UPDATE FROM ... SET indexonmapentry[?] = ? */
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapEntry_PutTo(final Integer indexOnMapEntry_key, final String indexOnMapEntry_value) {
where.with(QueryBuilder.put("indexonmapentry", QueryBuilder.bindMarker("indexOnMapEntry_key"), QueryBuilder.bindMarker("indexOnMapEntry_value")));
boundValues.add(indexOnMapEntry_key);
boundValues.add(indexOnMapEntry_value);
encodedValues.add(meta.indexOnMapEntry.keyProperty.encodeFromJava(indexOnMapEntry_key));
encodedValues.add(meta.indexOnMapEntry.valueProperty.encodeFromJava(indexOnMapEntry_value));
return this;
}
/**
* Generate an UPDATE FROM ... SET indexOnMapEntry = indexOnMapEntry + ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapEntry_AddAllTo(final Map indexOnMapEntry) {
where.with(QueryBuilder.addAll("indexonmapentry", QueryBuilder.bindMarker("indexonmapentry")));
boundValues.add(indexOnMapEntry);
encodedValues.add(meta.indexOnMapEntry.encodeFromJava(indexOnMapEntry));
return this;
}
/**
* Generate an UPDATE FROM ... SET indexOnMapEntry[?] = null */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapEntry_RemoveByKey(final Integer indexOnMapEntry_key) {
where.with(QueryBuilder.put("indexonmapentry", QueryBuilder.bindMarker("indexOnMapEntry_key"), QueryBuilder.bindMarker("indexOnMapEntry_value")));
boundValues.add(indexOnMapEntry_key);
boundValues.add(null);
encodedValues.add(meta.indexOnMapEntry.keyProperty.encodeFromJava(indexOnMapEntry_key));
encodedValues.add(null);
return this;
}
/**
* Generate an UPDATE FROM ... SET indexOnMapEntry = ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapEntry_Set(final Map indexOnMapEntry) {
where.with(QueryBuilder.set("indexonmapentry", QueryBuilder.bindMarker("indexonmapentry")));
boundValues.add(indexOnMapEntry);
encodedValues.add(meta.indexOnMapEntry.encodeFromJava(indexOnMapEntry));
return this;
}
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateWhere_Id where() {
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateWhere_Id(where);
}
}
public class EntityWithComplexIndices_UpdateFrom extends AbstractUpdateFrom {
EntityWithComplexIndices_UpdateFrom(Update.Where where) {
super(where);
}
/**
* Generate an UPDATE FROM ... SET simpleindex = ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns simpleIndex_Set(final String simpleIndex) {
where.with(QueryBuilder.set("simpleindex", QueryBuilder.bindMarker("simpleindex")));
boundValues.add(simpleIndex);
encodedValues.add(meta.simpleIndex.encodeFromJava(simpleIndex));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET collectionindex = collectionindex + [?] */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_AppendTo(final String collectionIndex_element) {
where.with(QueryBuilder.appendAll("collectionindex", QueryBuilder.bindMarker("collectionindex")));
boundValues.add(Arrays.asList(collectionIndex_element));
encodedValues.add(meta.collectionIndex.encodeFromJava(Arrays.asList(collectionIndex_element)));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET collectionIndex = collectionIndex + ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_AppendAllTo(final List collectionIndex) {
where.with(QueryBuilder.appendAll("collectionindex", QueryBuilder.bindMarker("collectionindex")));
boundValues.add(collectionIndex);
encodedValues.add(meta.collectionIndex.encodeFromJava(collectionIndex));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET collectionIndex = [?] + collectionIndex */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_PrependTo(final String collectionIndex_element) {
where.with(QueryBuilder.prependAll("collectionindex", QueryBuilder.bindMarker("collectionindex")));
boundValues.add(Arrays.asList(collectionIndex_element));
encodedValues.add(meta.collectionIndex.encodeFromJava(Arrays.asList(collectionIndex_element)));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET collectionIndex = ? + collectionIndex */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_PrependAllTo(final List collectionIndex) {
where.with(QueryBuilder.prependAll("collectionindex", QueryBuilder.bindMarker("collectionindex")));
boundValues.add(collectionIndex);
encodedValues.add(meta.collectionIndex.encodeFromJava(collectionIndex));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET collectionIndex[index] = ? */
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_SetAtIndex(final int index, final String collectionIndex_element) {
where.with(QueryBuilder.setIdx("collectionindex", index, QueryBuilder.bindMarker("collectionindex")));
boundValues.add(collectionIndex_element);
encodedValues.add(meta.collectionIndex.valueProperty.encodeFromJava(collectionIndex_element));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET collectionIndex[index] = null */
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_RemoveAtIndex(final int index) {
where.with(QueryBuilder.setIdx("collectionindex", index, QueryBuilder.bindMarker("collectionindex")));
boundValues.add(null);
encodedValues.add(null);
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET collectionIndex = collectionIndex - [?] */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_RemoveFrom(final String collectionIndex_element) {
where.with(QueryBuilder.discardAll("collectionindex", QueryBuilder.bindMarker("collectionindex")));
boundValues.add(Arrays.asList(collectionIndex_element));
encodedValues.add(meta.collectionIndex.encodeFromJava(Arrays.asList(collectionIndex_element)));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET collectionIndex = collectionIndex - ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_RemoveAllFrom(final List collectionIndex) {
where.with(QueryBuilder.discardAll("collectionindex", QueryBuilder.bindMarker("collectionindex")));
boundValues.add(collectionIndex);
encodedValues.add(meta.collectionIndex.encodeFromJava(collectionIndex));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET collectionIndex = ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns collectionIndex_Set(final List collectionIndex) {
where.with(QueryBuilder.set("collectionindex", QueryBuilder.bindMarker("collectionindex")));
boundValues.add(collectionIndex);
encodedValues.add(meta.collectionIndex.encodeFromJava(collectionIndex));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET fullindexoncollection = fullindexoncollection + {?} */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns fullIndexOnCollection_AddTo(final String fullIndexOnCollection_element) {
where.with(QueryBuilder.addAll("fullindexoncollection", QueryBuilder.bindMarker("fullindexoncollection")));
boundValues.add(Sets.newHashSet(fullIndexOnCollection_element));
encodedValues.add(meta.fullIndexOnCollection.encodeFromJava(Sets.newHashSet(fullIndexOnCollection_element)));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET fullIndexOnCollection = fullIndexOnCollection + ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns fullIndexOnCollection_AddAllTo(final Set fullIndexOnCollection) {
where.with(QueryBuilder.addAll("fullindexoncollection", QueryBuilder.bindMarker("fullindexoncollection")));
boundValues.add(fullIndexOnCollection);
encodedValues.add(meta.fullIndexOnCollection.encodeFromJava(fullIndexOnCollection));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET fullIndexOnCollection = fullIndexOnCollection - {?} */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns fullIndexOnCollection_RemoveFrom(final String fullIndexOnCollection_element) {
where.with(QueryBuilder.removeAll("fullindexoncollection", QueryBuilder.bindMarker("fullindexoncollection")));
boundValues.add(Sets.newHashSet(fullIndexOnCollection_element));
encodedValues.add(meta.fullIndexOnCollection.encodeFromJava(Sets.newHashSet(fullIndexOnCollection_element)));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET fullIndexOnCollection = fullIndexOnCollection - ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns fullIndexOnCollection_RemoveAllFrom(final Set fullIndexOnCollection) {
where.with(QueryBuilder.removeAll("fullindexoncollection", QueryBuilder.bindMarker("fullindexoncollection")));
boundValues.add(fullIndexOnCollection);
encodedValues.add(meta.fullIndexOnCollection.encodeFromJava(fullIndexOnCollection));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET fullIndexOnCollection = ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns fullIndexOnCollection_Set(final Set fullIndexOnCollection) {
where.with(QueryBuilder.set("fullindexoncollection", QueryBuilder.bindMarker("fullindexoncollection")));
boundValues.add(fullIndexOnCollection);
encodedValues.add(meta.fullIndexOnCollection.encodeFromJava(fullIndexOnCollection));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET indexonmapkey[?] = ? */
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapKey_PutTo(final String indexOnMapKey_key, final String indexOnMapKey_value) {
where.with(QueryBuilder.put("indexonmapkey", QueryBuilder.bindMarker("indexOnMapKey_key"), QueryBuilder.bindMarker("indexOnMapKey_value")));
boundValues.add(indexOnMapKey_key);
boundValues.add(indexOnMapKey_value);
encodedValues.add(meta.indexOnMapKey.keyProperty.encodeFromJava(indexOnMapKey_key));
encodedValues.add(meta.indexOnMapKey.valueProperty.encodeFromJava(indexOnMapKey_value));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET indexOnMapKey = indexOnMapKey + ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapKey_AddAllTo(final Map indexOnMapKey) {
where.with(QueryBuilder.addAll("indexonmapkey", QueryBuilder.bindMarker("indexonmapkey")));
boundValues.add(indexOnMapKey);
encodedValues.add(meta.indexOnMapKey.encodeFromJava(indexOnMapKey));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET indexOnMapKey[?] = null */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapKey_RemoveByKey(final String indexOnMapKey_key) {
where.with(QueryBuilder.put("indexonmapkey", QueryBuilder.bindMarker("indexOnMapKey_key"), QueryBuilder.bindMarker("indexOnMapKey_value")));
boundValues.add(indexOnMapKey_key);
boundValues.add(null);
encodedValues.add(meta.indexOnMapKey.keyProperty.encodeFromJava(indexOnMapKey_key));
encodedValues.add(null);
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET indexOnMapKey = ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapKey_Set(final Map indexOnMapKey) {
where.with(QueryBuilder.set("indexonmapkey", QueryBuilder.bindMarker("indexonmapkey")));
boundValues.add(indexOnMapKey);
encodedValues.add(meta.indexOnMapKey.encodeFromJava(indexOnMapKey));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET indexonmapentry[?] = ? */
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapEntry_PutTo(final Integer indexOnMapEntry_key, final String indexOnMapEntry_value) {
where.with(QueryBuilder.put("indexonmapentry", QueryBuilder.bindMarker("indexOnMapEntry_key"), QueryBuilder.bindMarker("indexOnMapEntry_value")));
boundValues.add(indexOnMapEntry_key);
boundValues.add(indexOnMapEntry_value);
encodedValues.add(meta.indexOnMapEntry.keyProperty.encodeFromJava(indexOnMapEntry_key));
encodedValues.add(meta.indexOnMapEntry.valueProperty.encodeFromJava(indexOnMapEntry_value));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET indexOnMapEntry = indexOnMapEntry + ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapEntry_AddAllTo(final Map indexOnMapEntry) {
where.with(QueryBuilder.addAll("indexonmapentry", QueryBuilder.bindMarker("indexonmapentry")));
boundValues.add(indexOnMapEntry);
encodedValues.add(meta.indexOnMapEntry.encodeFromJava(indexOnMapEntry));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET indexOnMapEntry[?] = null */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapEntry_RemoveByKey(final Integer indexOnMapEntry_key) {
where.with(QueryBuilder.put("indexonmapentry", QueryBuilder.bindMarker("indexOnMapEntry_key"), QueryBuilder.bindMarker("indexOnMapEntry_value")));
boundValues.add(indexOnMapEntry_key);
boundValues.add(null);
encodedValues.add(meta.indexOnMapEntry.keyProperty.encodeFromJava(indexOnMapEntry_key));
encodedValues.add(null);
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
/**
* Generate an UPDATE FROM ... SET indexOnMapEntry = ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns indexOnMapEntry_Set(final Map indexOnMapEntry) {
where.with(QueryBuilder.set("indexonmapentry", QueryBuilder.bindMarker("indexonmapentry")));
boundValues.add(indexOnMapEntry);
encodedValues.add(meta.indexOnMapEntry.encodeFromJava(indexOnMapEntry));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateColumns(where);
}
}
public final class EntityWithComplexIndices_UpdateWhere_Id extends AbstractUpdateWhere {
public EntityWithComplexIndices_UpdateWhere_Id(Update.Where where) {
super(where);
}
/**
* Generate a SELECT ... FROM ... WHERE ... id = ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateEnd id_Eq(Long id) {
where.and(QueryBuilder.eq("id", QueryBuilder.bindMarker("id_Eq")));
boundValues.add(id);
encodedValues.add(meta.id.encodeFromJava(id));
return new EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateEnd(where);
}
/**
* Generate a SELECT ... FROM ... WHERE ... id IN ? */
@SuppressWarnings("static-access")
public final EntityWithComplexIndices_Update.EntityWithComplexIndices_UpdateEnd id_IN(Long... id) {
Validator.validateTrue(ArrayUtils.isNotEmpty(id), "Varargs for field '%s' should not be null/empty", "id");
where.and(QueryBuilder.in("id",QueryBuilder.bindMarker("id")));
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy