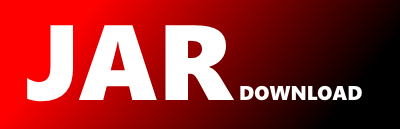
info.archinnov.achilles.generated.meta.udt.UDTWithNestedUDT_AchillesMeta Maven / Gradle / Ivy
package info.archinnov.achilles.generated.meta.udt;
import com.datastax.driver.core.DataType;
import com.datastax.driver.core.UDTValue;
import com.google.common.reflect.TypeToken;
import info.archinnov.achilles.internals.codec.FallThroughCodec;
import info.archinnov.achilles.internals.entities.UDTWithNestedUDT;
import info.archinnov.achilles.internals.entities.UDTWithNoKeyspace;
import info.archinnov.achilles.internals.metamodel.AbstractProperty;
import info.archinnov.achilles.internals.metamodel.AbstractUDTClassProperty;
import info.archinnov.achilles.internals.metamodel.ListProperty;
import info.archinnov.achilles.internals.metamodel.SimpleProperty;
import info.archinnov.achilles.internals.metamodel.Tuple2Property;
import info.archinnov.achilles.internals.metamodel.UDTProperty;
import info.archinnov.achilles.internals.metamodel.columns.ColumnInfo;
import info.archinnov.achilles.internals.metamodel.columns.ColumnType;
import info.archinnov.achilles.internals.metamodel.columns.FieldInfo;
import info.archinnov.achilles.internals.metamodel.index.IndexInfo;
import info.archinnov.achilles.internals.strategy.naming.InternalNamingStrategy;
import info.archinnov.achilles.type.tuples.Tuple2;
import java.lang.Class;
import java.lang.Integer;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
public final class UDTWithNestedUDT_AchillesMeta extends AbstractUDTClassProperty {
/**
* Meta class for 'value' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given UDTWithNestedUDT instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final SimpleProperty value = new SimpleProperty(new FieldInfo<>((UDTWithNestedUDT entity$) -> entity$.getValue(), (UDTWithNestedUDT entity$, String value$) -> entity$.setValue(value$), "value", "value", ColumnType.NORMAL, new ColumnInfo(false), IndexInfo.noIndex()), DataType.text(), gettableData$ -> gettableData$.get("value", java.lang.String.class), (settableData$, value$) -> settableData$.set("value", value$, java.lang.String.class), new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(String.class));
/**
* Meta class for 'udtList' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given UDTWithNestedUDT instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings("unchecked")
public static final ListProperty udtList = new ListProperty<>(new FieldInfo<>((UDTWithNestedUDT entity$) -> entity$.getUdtList(), (UDTWithNestedUDT entity$, List value$) -> entity$.setUdtList(value$), "udtList", "udtlist", ColumnType.NORMAL, new ColumnInfo(false), IndexInfo.noIndex()), false, false, UDTValue.class, new UDTProperty(FieldInfo. of("udtList", "udtlist"), UDTWithNoKeyspace.class, info.archinnov.achilles.generated.meta.udt.UDTWithNoKeyspace_AchillesMeta.INSTANCE));
/**
* Meta class for 'nestedUDT' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given UDTWithNestedUDT instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
public static final UDTProperty nestedUDT = new UDTProperty(new FieldInfo<>((UDTWithNestedUDT entity$) -> entity$.getNestedUDT(), (UDTWithNestedUDT entity$, UDTWithNoKeyspace value$) -> entity$.setNestedUDT(value$), "nestedUDT", "nestedudt", ColumnType.NORMAL, new ColumnInfo(true), IndexInfo.noIndex()), UDTWithNoKeyspace.class, info.archinnov.achilles.generated.meta.udt.UDTWithNoKeyspace_AchillesMeta.INSTANCE);
/**
* Meta class for 'tupleWithUDT' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given UDTWithNestedUDT instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final Tuple2Property tupleWithUDT = new Tuple2Property<>(new FieldInfo<>((UDTWithNestedUDT entity$) -> entity$.getTupleWithUDT(), (UDTWithNestedUDT entity$, Tuple2 value$) -> entity$.setTupleWithUDT(value$), "tupleWithUDT", "tuplewithudt", ColumnType.NORMAL, new ColumnInfo(false), IndexInfo.noIndex()), new SimpleProperty(FieldInfo. of("tupleWithUDT", "tuplewithudt"), DataType.cint(), gettable$ -> null, (udt$, value$) -> {}, new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(Integer.class)), new UDTProperty(FieldInfo. of("tupleWithUDT", "tuplewithudt"), UDTWithNoKeyspace.class, info.archinnov.achilles.generated.meta.udt.UDTWithNoKeyspace_AchillesMeta.INSTANCE));
public static final UDTWithNestedUDT_AchillesMeta INSTANCE = new UDTWithNestedUDT_AchillesMeta();
@Override
protected Optional getStaticKeyspace() {
return Optional.empty();
}
@Override
protected Optional getStaticUdtName() {
return Optional.of("having_nested_type");
}
@Override
protected Optional getStaticNamingStrategy() {
return Optional.empty();
}
@Override
protected String getUdtName() {
return "having_nested_type";
}
@Override
protected Class getUdtClass() {
return UDTWithNestedUDT.class;
}
@Override
protected List> getComponentsProperty() {
return Arrays.asList(value, udtList, nestedUDT, tupleWithUDT);
}
@Override
protected UDTValue createUDTFromBean(UDTWithNestedUDT instance) {
final UDTValue udtValue = userType.newValue();
value.encodeFieldToUdt(instance, udtValue);
udtList.encodeFieldToUdt(instance, udtValue);
nestedUDT.encodeFieldToUdt(instance, udtValue);
tupleWithUDT.encodeFieldToUdt(instance, udtValue);
return udtValue;
}
@Override
protected UDTWithNestedUDT createBeanFromUDT(UDTValue udtValue) {
final UDTWithNestedUDT instance = udtFactory.newInstance(udtClass);
value.decodeField(udtValue, instance);
udtList.decodeField(udtValue, instance);
nestedUDT.decodeField(udtValue, instance);
tupleWithUDT.decodeField(udtValue, instance);
return instance;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy