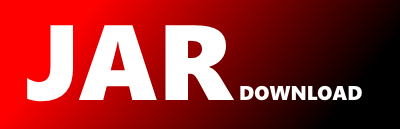
org.hl7.elm.r1.ValueSetDef Maven / Gradle / Ivy
Show all versions of elm Show documentation
//
// This file was generated by the Eclipse Implementation of JAXB, v3.0.2
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.12.20 at 10:51:29 AM MST
//
package org.hl7.elm.r1;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.namespace.QName;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlType;
import org.hl7.cql_annotations.r1.CqlToElmBase;
import org.jvnet.jaxb2_commons.lang.Equals2;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy2;
import org.jvnet.jaxb2_commons.lang.HashCode2;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy2;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString2;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy2;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
* The ValueSetDef type defines a value set identifier that can be referenced by name anywhere within an expression.
*
* The id specifies the globally unique identifier for the value set. This may be an HL7 OID, a FHIR URL, or a CTS2 value set URL.
*
* If version is specified, it will be used to resolve the version of the value set definition to be used. Otherwise, the most current published version of the value set is assumed.
*
* If codeSystems are specified, they will be used to resolve the code systems used within the value set definition to construct the expansion set.
* Note that the recommended approach to statically binding to an expansion set is to use a value set definition that specifies the version of each code system used. The codeSystemVersions attribute is provided only to ensure static binding can be achieved when the value set definition does not specify code system versions as part of the definition header.
*
* Java class for ValueSetDef complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ValueSetDef">
* <complexContent>
* <extension base="{urn:hl7-org:elm:r1}Element">
* <sequence>
* <element name="codeSystem" type="{urn:hl7-org:elm:r1}CodeSystemRef" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="name" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="id" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="version" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="accessLevel" type="{urn:hl7-org:elm:r1}AccessModifier" default="Public" />
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ValueSetDef", namespace = "urn:hl7-org:elm:r1", propOrder = {
"codeSystem"
})
public class ValueSetDef
extends Element
implements Equals2, HashCode2, ToString2
{
@XmlElement(namespace = "urn:hl7-org:elm:r1")
protected List codeSystem;
@XmlAttribute(name = "name")
protected String name;
@XmlAttribute(name = "id", required = true)
protected String id;
@XmlAttribute(name = "version")
protected String version;
@XmlAttribute(name = "accessLevel")
protected AccessModifier accessLevel;
/**
* Gets the value of the codeSystem property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a set
method for the codeSystem property.
*
*
* For example, to add a new item, do as follows:
*
* getCodeSystem().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CodeSystemRef }
*
*
*/
public List getCodeSystem() {
if (codeSystem == null) {
codeSystem = new ArrayList();
}
return this.codeSystem;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
/**
* Gets the value of the version property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVersion() {
return version;
}
/**
* Sets the value of the version property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVersion(String value) {
this.version = value;
}
/**
* Gets the value of the accessLevel property.
*
* @return
* possible object is
* {@link AccessModifier }
*
*/
public AccessModifier getAccessLevel() {
if (accessLevel == null) {
return AccessModifier.PUBLIC;
} else {
return accessLevel;
}
}
/**
* Sets the value of the accessLevel property.
*
* @param value
* allowed object is
* {@link AccessModifier }
*
*/
public void setAccessLevel(AccessModifier value) {
this.accessLevel = value;
}
public ValueSetDef withCodeSystem(CodeSystemRef... values) {
if (values!= null) {
for (CodeSystemRef value: values) {
getCodeSystem().add(value);
}
}
return this;
}
public ValueSetDef withCodeSystem(Collection values) {
if (values!= null) {
getCodeSystem().addAll(values);
}
return this;
}
public ValueSetDef withName(String value) {
setName(value);
return this;
}
public ValueSetDef withId(String value) {
setId(value);
return this;
}
public ValueSetDef withVersion(String value) {
setVersion(value);
return this;
}
public ValueSetDef withAccessLevel(AccessModifier value) {
setAccessLevel(value);
return this;
}
@Override
public ValueSetDef withAnnotation(CqlToElmBase... values) {
if (values!= null) {
for (CqlToElmBase value: values) {
getAnnotation().add(value);
}
}
return this;
}
@Override
public ValueSetDef withAnnotation(Collection values) {
if (values!= null) {
getAnnotation().addAll(values);
}
return this;
}
@Override
public ValueSetDef withResultTypeSpecifier(TypeSpecifier value) {
setResultTypeSpecifier(value);
return this;
}
@Override
public ValueSetDef withLocalId(String value) {
setLocalId(value);
return this;
}
@Override
public ValueSetDef withLocator(String value) {
setLocator(value);
return this;
}
@Override
public ValueSetDef withResultTypeName(QName value) {
setResultTypeName(value);
return this;
}
@Override
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy2 strategy) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
if (!super.equals(thisLocator, thatLocator, object, strategy)) {
return false;
}
final ValueSetDef that = ((ValueSetDef) object);
{
List lhsCodeSystem;
lhsCodeSystem = (((this.codeSystem!= null)&&(!this.codeSystem.isEmpty()))?this.getCodeSystem():null);
List rhsCodeSystem;
rhsCodeSystem = (((that.codeSystem!= null)&&(!that.codeSystem.isEmpty()))?that.getCodeSystem():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "codeSystem", lhsCodeSystem), LocatorUtils.property(thatLocator, "codeSystem", rhsCodeSystem), lhsCodeSystem, rhsCodeSystem, ((this.codeSystem!= null)&&(!this.codeSystem.isEmpty())), ((that.codeSystem!= null)&&(!that.codeSystem.isEmpty())))) {
return false;
}
}
{
String lhsName;
lhsName = this.getName();
String rhsName;
rhsName = that.getName();
if (!strategy.equals(LocatorUtils.property(thisLocator, "name", lhsName), LocatorUtils.property(thatLocator, "name", rhsName), lhsName, rhsName, (this.name!= null), (that.name!= null))) {
return false;
}
}
{
String lhsId;
lhsId = this.getId();
String rhsId;
rhsId = that.getId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "id", lhsId), LocatorUtils.property(thatLocator, "id", rhsId), lhsId, rhsId, (this.id!= null), (that.id!= null))) {
return false;
}
}
{
String lhsVersion;
lhsVersion = this.getVersion();
String rhsVersion;
rhsVersion = that.getVersion();
if (!strategy.equals(LocatorUtils.property(thisLocator, "version", lhsVersion), LocatorUtils.property(thatLocator, "version", rhsVersion), lhsVersion, rhsVersion, (this.version!= null), (that.version!= null))) {
return false;
}
}
{
AccessModifier lhsAccessLevel;
lhsAccessLevel = this.getAccessLevel();
AccessModifier rhsAccessLevel;
rhsAccessLevel = that.getAccessLevel();
if (!strategy.equals(LocatorUtils.property(thisLocator, "accessLevel", lhsAccessLevel), LocatorUtils.property(thatLocator, "accessLevel", rhsAccessLevel), lhsAccessLevel, rhsAccessLevel, (this.accessLevel!= null), (that.accessLevel!= null))) {
return false;
}
}
return true;
}
@Override
public boolean equals(Object object) {
final EqualsStrategy2 strategy = JAXBEqualsStrategy.getInstance();
return equals(null, null, object, strategy);
}
@Override
public int hashCode(ObjectLocator locator, HashCodeStrategy2 strategy) {
int currentHashCode = super.hashCode(locator, strategy);
{
List theCodeSystem;
theCodeSystem = (((this.codeSystem!= null)&&(!this.codeSystem.isEmpty()))?this.getCodeSystem():null);
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "codeSystem", theCodeSystem), currentHashCode, theCodeSystem, ((this.codeSystem!= null)&&(!this.codeSystem.isEmpty())));
}
{
String theName;
theName = this.getName();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "name", theName), currentHashCode, theName, (this.name!= null));
}
{
String theId;
theId = this.getId();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "id", theId), currentHashCode, theId, (this.id!= null));
}
{
String theVersion;
theVersion = this.getVersion();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "version", theVersion), currentHashCode, theVersion, (this.version!= null));
}
{
AccessModifier theAccessLevel;
theAccessLevel = this.getAccessLevel();
currentHashCode = strategy.hashCode(LocatorUtils.property(locator, "accessLevel", theAccessLevel), currentHashCode, theAccessLevel, (this.accessLevel!= null));
}
return currentHashCode;
}
@Override
public int hashCode() {
final HashCodeStrategy2 strategy = JAXBHashCodeStrategy.getInstance();
return this.hashCode(null, strategy);
}
@Override
public String toString() {
final ToStringStrategy2 strategy = JAXBToStringStrategy.getInstance();
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
@Override
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy2 strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
@Override
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy2 strategy) {
super.appendFields(locator, buffer, strategy);
{
List theCodeSystem;
theCodeSystem = (((this.codeSystem!= null)&&(!this.codeSystem.isEmpty()))?this.getCodeSystem():null);
strategy.appendField(locator, this, "codeSystem", buffer, theCodeSystem, ((this.codeSystem!= null)&&(!this.codeSystem.isEmpty())));
}
{
String theName;
theName = this.getName();
strategy.appendField(locator, this, "name", buffer, theName, (this.name!= null));
}
{
String theId;
theId = this.getId();
strategy.appendField(locator, this, "id", buffer, theId, (this.id!= null));
}
{
String theVersion;
theVersion = this.getVersion();
strategy.appendField(locator, this, "version", buffer, theVersion, (this.version!= null));
}
{
AccessModifier theAccessLevel;
theAccessLevel = this.getAccessLevel();
strategy.appendField(locator, this, "accessLevel", buffer, theAccessLevel, (this.accessLevel!= null));
}
return buffer;
}
public void setCodeSystem(List value) {
this.codeSystem = value;
}
}