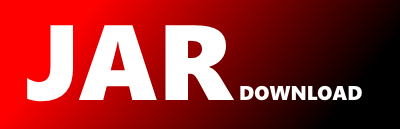
cucumber.io.FlatteningIterator Maven / Gradle / Ivy
package cucumber.io;
import java.lang.reflect.Array;
import java.util.Arrays;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.util.Stack;
/**
* An iterator that 'flattens out' collections, iterators, arrays, etc.
*
* That is it will iterate out their contents in order, descending into any
* iterators, iterables or arrays provided to it.
*
* An example (not valid Java for brevity - some type declarations are ommitted):
*
* new FlattingIterator({1, 2, 3}, {{1, 2}, {3}}, new ArrayList({1, 2, 3}))
*
* Will iterate through the sequence 1, 2, 3, 1, 2, 3, 1, 2, 3.
*
* Note that this implements a non-generic version of the Iterator interface so
* may be cast appropriately - it's very hard to give this class an appropriate
* generic type.
*
* @author david
*/
public class FlatteningIterator implements Iterator {
// Marker object. This is never exposed outside this class, so can be guaranteed
// to be != anything else. We use it to indicate an absense of any other object.
private final Object blank = new Object();
/* This stack stores all the iterators found so far. The head of the stack is
* the iterator which we are currently progressing through */
private final Stack> iterators = new Stack>();
// Storage field for the next element to be returned. blank when the next element
// is currently unknown.
private Object next = blank;
public FlatteningIterator(Object... objects) {
Iterator
© 2015 - 2024 Weber Informatics LLC | Privacy Policy