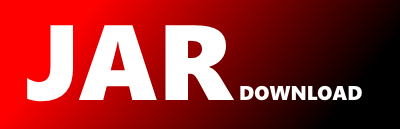
cucumber.runtime.RuntimeOptions Maven / Gradle / Ivy
package cucumber.runtime;
import cucumber.formatter.ColorAware;
import cucumber.formatter.FormatterConverter;
import cucumber.formatter.ProgressFormatter;
import cucumber.io.ResourceLoader;
import cucumber.runtime.model.CucumberFeature;
import gherkin.formatter.Formatter;
import gherkin.formatter.Reporter;
import gherkin.util.FixJava;
import java.io.File;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
import java.util.ArrayList;
import java.util.List;
import java.util.ResourceBundle;
import static cucumber.runtime.model.CucumberFeature.load;
import static java.util.Arrays.asList;
public class RuntimeOptions {
public static final String VERSION = ResourceBundle.getBundle("cucumber.version").getString("cucumber-jvm.version");
public static final String USAGE = FixJava.readResource("/cucumber/runtime/USAGE.txt");
public List glue = new ArrayList();
public File dotCucumber;
public boolean dryRun;
public List tags = new ArrayList();
public List formatters = new ArrayList();
public List featurePaths = new ArrayList();
private boolean monochrome = false;
public RuntimeOptions(String... argv) {
parse(new ArrayList(asList(argv)));
if (formatters.isEmpty()) {
formatters.add(new ProgressFormatter(System.out));
}
for (Formatter formatter : formatters) {
if(formatter instanceof ColorAware) {
ColorAware colorAware = (ColorAware) formatter;
colorAware.setMonochrome(monochrome);
}
}
}
private void parse(ArrayList args) {
FormatterConverter formatterConverter = new FormatterConverter();
while (!args.isEmpty()) {
String arg = args.remove(0);
if (arg.equals("--help") || arg.equals("-h")) {
System.out.println(USAGE);
System.exit(0);
} else if (arg.equals("--version") || arg.equals("-v")) {
System.out.println(VERSION);
System.exit(0);
} else if (arg.equals("--glue") || arg.equals("-g")) {
String gluePath = args.remove(0);
glue.add(gluePath);
} else if (arg.equals("--tags") || arg.equals("-t")) {
tags.add(args.remove(0));
} else if (arg.equals("--format") || arg.equals("-f")) {
formatters.add(formatterConverter.convert(args.remove(0)));
} else if (arg.equals("--dotcucumber")) {
dotCucumber = new File(args.remove(0));
} else if (arg.equals("--dry-run") || arg.equals("-d")) {
dryRun = true;
} else if (arg.equals("--monochrome") || arg.equals("-m")) {
monochrome = true;
} else {
// TODO: Use PathWithLines and add line filter if any
featurePaths.add(arg);
}
}
}
public List cucumberFeatures(ResourceLoader resourceLoader) {
return load(resourceLoader, featurePaths, filters());
}
public Formatter formatter(ClassLoader classLoader) {
return (Formatter) Proxy.newProxyInstance(classLoader, new Class>[]{Formatter.class}, new InvocationHandler() {
@Override
public Object invoke(Object target, Method method, Object[] args) throws Throwable {
for (Formatter formatter : formatters) {
method.invoke(formatter, args);
}
return null;
}
});
}
public Reporter reporter(ClassLoader classLoader) {
return (Reporter) Proxy.newProxyInstance(classLoader, new Class>[]{Reporter.class}, new InvocationHandler() {
@Override
public Object invoke(Object target, Method method, Object[] args) throws Throwable {
for (Formatter formatter : formatters) {
if (formatter instanceof Reporter) {
method.invoke(formatter, args);
}
}
return null;
}
});
}
private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy