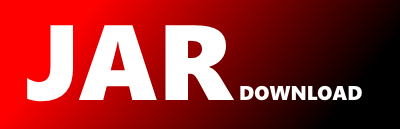
cucumber.table.DataTable Maven / Gradle / Ivy
package cucumber.table;
import gherkin.formatter.PrettyFormatter;
import gherkin.formatter.model.DataTableRow;
import gherkin.formatter.model.Row;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.List;
public class DataTable {
private final List> raw;
private final List gherkinRows;
private final TableConverter tableConverter;
public DataTable(List gherkinRows, TableConverter tableConverter) {
this.gherkinRows = gherkinRows;
this.tableConverter = tableConverter;
this.raw = new ArrayList>();
for (Row row : gherkinRows) {
List list = new ArrayList();
list.addAll(row.getCells());
this.raw.add(list);
}
}
public List> raw() {
return this.raw;
}
public List asList(Type listType) {
return tableConverter.toList(listType, this);
}
List topCells() {
return gherkinRows.get(0).getCells();
}
List> cells(int firstRow) {
List> attributeValues = new ArrayList>();
List valueRows = gherkinRows.subList(firstRow, gherkinRows.size());
for (Row valueRow : valueRows) {
attributeValues.add(toStrings(valueRow));
}
return attributeValues;
}
private List toStrings(Row row) {
List strings = new ArrayList();
for (String string : row.getCells()) {
strings.add(string);
}
return strings;
}
public void diff(List> other) {
diff(tableConverter.toTable(other));
}
private void diff(DataTable other) {
new TableDiffer(this, other).calculateDiffs();
}
public List getGherkinRows() {
return gherkinRows;
}
@Override
public String toString() {
StringBuilder result = new StringBuilder();
PrettyFormatter pf = new PrettyFormatter(result, true, false);
pf.table(getGherkinRows());
pf.eof();
return result.toString();
}
List diffableRows() {
List result = new ArrayList();
List> convertedRows = raw();
for (int i = 0; i < convertedRows.size(); i++) {
result.add(new DiffableRow(getGherkinRows().get(i), convertedRows.get(i)));
}
return result;
}
TableConverter getTableConverter() {
return tableConverter;
}
class DiffableRow {
public final Row row;
public final List convertedRow;
public DiffableRow(Row row, List convertedRow) {
this.row = row;
this.convertedRow = convertedRow;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DiffableRow that = (DiffableRow) o;
return convertedRow.equals(that.convertedRow);
}
@Override
public int hashCode() {
return convertedRow.hashCode();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy