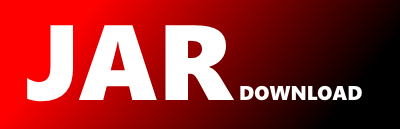
info.freelibrary.marc4j.converter.impl.CodeTableHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of freelib-marc4j Show documentation
Show all versions of freelib-marc4j Show documentation
An easy to use Application Programming Interface (API) for working with MARC and MARCXML in
Java.
/**
* Copyright (C) 2002 Bas Peters
*
* This file is part of MARC4J
*
* MARC4J is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* MARC4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with MARC4J; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package info.freelibrary.marc4j.converter.impl;
import java.io.File;
import java.io.FileInputStream;
import java.util.HashMap;
import java.util.Vector;
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import org.xml.sax.Attributes;
import org.xml.sax.InputSource;
import org.xml.sax.Locator;
import org.xml.sax.SAXParseException;
import org.xml.sax.XMLReader;
import org.xml.sax.helpers.DefaultHandler;
/**
* CodeTableHandler
is a SAX2 ContentHandler
that
* builds a data structure to facilitate AnselToUnicode character conversion.
*
* @author Corey Keith
* @see DefaultHandler
*/
public class CodeTableHandler extends DefaultHandler {
private HashMap> sets;
private HashMap charset;
private HashMap> combiningchars;
/** Data element identifier */
private Integer isocode;
private Integer marc;
private Character ucs;
private boolean useAlt = false;
private boolean iscombining;
private Vector combining;
/** Tag name */
// private String tag;
/** StringBuffer to store data */
private StringBuffer data;
/** Locator object */
protected Locator locator;
/**
* Gets the character sets hashtable.
*
* @return The character sets
*/
public HashMap> getCharSets() {
return sets;
}
/**
* Gets the combining characters.
*
* @return The combining characters
*/
public HashMap> getCombiningChars() {
return combiningchars;
}
/**
*
* Registers the SAX2 Locator
object.
*
*
* @param locator the {@link Locator}object
*/
public void setDocumentLocator(Locator locator) {
this.locator = locator;
}
/**
* An event fired at the start of an element.
*
* @param uri
* @param name
* @param qname
* @param atts
* @throws SAXParseException
*/
public void startElement(String uri, String name, String qName,
Attributes atts) throws SAXParseException {
if (name.equals("characterSet")) {
charset = new HashMap();
isocode = Integer.valueOf(atts.getValue("ISOcode"), 16);
combining = new Vector();
} else if (name.equals("marc")) {
data = new StringBuffer();
} else if (name.equals("codeTables")) {
sets = new HashMap>();
combiningchars = new HashMap>();
} else if (name.equals("ucs")) {
data = new StringBuffer();
} else if (name.equals("alt")) {
data = new StringBuffer();
} else if (name.equals("isCombining")) {
data = new StringBuffer();
} else if (name.equals("code")) {
iscombining = false;
}
}
/**
* An event fired as characters are consumed.
*
* @param ch
* @param start
* @param length
*/
public void characters(char[] ch, int start, int length) {
if (data != null) {
data.append(ch, start, length);
}
}
/**
* An event fired at the end of parsing an element.
*
* @param uri
* @param name
* @param qname
* @throws SAXParseException
*/
public void endElement(String uri, String name, String qName)
throws SAXParseException {
if (name.equals("characterSet")) {
sets.put(isocode, charset);
combiningchars.put(isocode, combining);
combining = null;
charset = null;
} else if (name.equals("marc")) {
marc = Integer.valueOf(data.toString(), 16);
} else if (name.equals("ucs")) {
if (data.length() > 0) {
ucs =
new Character((char) Integer.parseInt(data.toString(),
16));
} else {
ucs = null;
}
} else if (name.equals("alt")) {
if (useAlt && data.length() > 0) {
ucs =
new Character((char) Integer.parseInt(data.toString(),
16));
useAlt = false;
}
} else if (name.equals("code")) {
if (iscombining) {
combining.add(marc);
}
charset.put(marc, ucs);
} else if (name.equals("isCombining")) {
if (data.toString().equals("true")) {
iscombining = true;
}
}
data = null;
}
/**
* The main class on the CodeTableHandler. FIXME needed?
*
* @param args
*/
public static void main(String[] args) {
@SuppressWarnings("unused")
HashMap> charsets = null;
try {
SAXParserFactory factory = SAXParserFactory.newInstance();
factory.setNamespaceAware(true);
factory.setValidating(false);
SAXParser saxParser = factory.newSAXParser();
XMLReader rdr = saxParser.getXMLReader();
// FIXME: Needed?
File file =
new File(
"C:\\Documents and Settings\\ckeith\\Desktop\\Projects\\Code Tables\\codetables.xml");
InputSource src = new InputSource(new FileInputStream(file));
CodeTableHandler saxUms = new CodeTableHandler();
rdr.setContentHandler(saxUms);
rdr.parse(src);
charsets = saxUms.getCharSets();
// System.out.println( charsets.toString() );
System.out.println(saxUms.getCombiningChars());
} catch (Exception exc) {
exc.printStackTrace(System.out);
// System.err.println( "Exception: " + exc );
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy