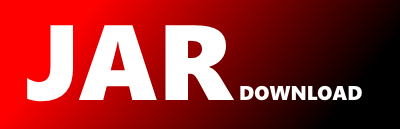
org.marc4j.util.CustomDecimalFormat Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of freelib-marc4j Show documentation
Show all versions of freelib-marc4j Show documentation
An easy to use Application Programming Interface (API) for working with MARC and MARCXML in
Java.
/** A formatter that is like a << new DecimalFormat("00000") with
* setMaximumIntegerDigits(5), but if
* the number to be formatted is greater than 99999, it will truncate
* it to 99999 instead of just writing out 5 least significant digits.
* If you'd like 00000 instead of 99999, you can set static variable
* overflowRepresentation to 0.
*/
package org.marc4j.util;
import java.text.DecimalFormat;
import java.text.FieldPosition;
public class CustomDecimalFormat extends DecimalFormat {
/**
* The serialVersionUID
for the class.
*/
private static final long serialVersionUID = 2377613559633630577L;
static String formatString = "00000000000000000000";
static String maxString = "99999999999999999999";
public final static int REP_ALL_ZEROS = 0;
public final static int REP_ALL_NINES = 1;
public final static int REP_TRUNCATE = 2;
int overflowRepresentation = REP_ALL_NINES;
long maximumValue;
/**
* Creates a custom decimal format with the supplied number of digits.
*
* @param numberDigits
*/
public CustomDecimalFormat(int numberDigits) {
super(formatString.substring(0, numberDigits));
maximumValue = Long.parseLong(maxString.substring(0, numberDigits));
overflowRepresentation = REP_ALL_NINES;
this.setMaximumIntegerDigits(numberDigits);
}
/**
* Creates a custom decimal format with the supplied number of digits.
*
* @param numberDigits
* @param overflowType
*/
public CustomDecimalFormat(int numberDigits, int overflowType) {
super(formatString.substring(0, numberDigits));
maximumValue = Long.parseLong(maxString.substring(0, numberDigits));
overflowRepresentation = overflowType;
this.setMaximumIntegerDigits(5);
}
@Override
public StringBuffer format(double number, StringBuffer toAppendTo,
FieldPosition pos) {
if (number > maximumValue) {
number = getOverflowRepresentation((long) number);
}
return super.format(number, toAppendTo, pos);
}
@Override
public StringBuffer format(long number, StringBuffer toAppendTo,
FieldPosition pos) {
if (number > maximumValue) {
number = getOverflowRepresentation(number);
}
return super.format(number, toAppendTo, pos);
}
private long getOverflowRepresentation(long number) {
switch (overflowRepresentation) {
case REP_ALL_ZEROS:
return (0);
default:
case REP_ALL_NINES:
return (maximumValue);
case REP_TRUNCATE:
return (number % (maximumValue + 1));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy