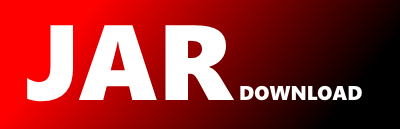
org.marc4j.marc.MarcFactory Maven / Gradle / Ivy
/**
* Copyright (C) 2004 Bas Peters
*
* This file is part of MARC4J
*
* MARC4J is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* MARC4J is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with MARC4J; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.marc4j.marc;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.Properties;
/**
* Factory for creating MARC record objects.
*
* You can use MarcFactory
to create records from scratch:
*
*
*
*
* MarcFactory factory = MarcFactory.newInstance();
* Record record = factory.newRecord();
* ControlField cf = factory.newControlField("001");
* record.addVariableField(cf);
* etc...
*
*
*
* @author Bas Peters
*/
public abstract class MarcFactory {
protected MarcFactory() {
}
/**
* Creates a new factory instance. The implementation class to load is the
* first found in the following locations:
*
* - the
org.marc4j.marc.MarcFactory
system property
* - the above named property value in the
*
$JAVA_HOME/lib/marc4j.properties
file
* - the class name specified in the
*
META-INF/services/org.marc4j.marc.MarcFactory
system
* resource
* - the default factory class
*
*/
public static MarcFactory newInstance() {
ClassLoader loader = Thread.currentThread().getContextClassLoader();
if (loader == null) {
loader = MarcFactory.class.getClassLoader();
}
String className = null;
int count = 0;
do {
className = getFactoryClassName(loader, count++);
if (className != null) {
try {
final Class> t =
(loader != null) ? loader.loadClass(className)
: Class.forName(className);
return (MarcFactory) t.newInstance();
} catch (final ClassNotFoundException e) {
className = null;
} catch (final Exception e) {
}
}
} while (className == null && count < 3);
return new info.freelibrary.marc4j.impl.MarcFactoryImpl();
}
private static String getFactoryClassName(final ClassLoader loader, final int attempt) {
final String propertyName = "org.marc4j.marc.MarcFactory";
switch (attempt) {
case 0:
return System.getProperty(propertyName);
case 1:
try {
File file = new File(System.getProperty("java.home"));
file = new File(file, "lib");
file = new File(file, "marc4j.properties");
final InputStream in = new FileInputStream(file);
final Properties props = new Properties();
props.load(in);
in.close();
return props.getProperty(propertyName);
} catch (final IOException e) {
return null;
}
case 2:
try {
final String serviceKey = "/META-INF/services/" + propertyName;
final InputStream in =
(loader != null) ? loader
.getResourceAsStream(serviceKey)
: MarcFactory.class
.getResourceAsStream(serviceKey);
if (in != null) {
final BufferedReader r =
new BufferedReader(new InputStreamReader(in));
final String ret = r.readLine();
r.close();
return ret;
}
} catch (final IOException e) {
}
return null;
default:
return null;
}
}
/**
* Returns a new control field instance.
*
* @return ControlField
*/
public abstract ControlField newControlField();
/**
* Creates a new control field with the given tag and returns the instance.
*
* @return ControlField
*/
public abstract ControlField newControlField(String tag);
/**
* Creates a new control field with the given tag and data and returns the
* instance.
*
* @return ControlField
*/
public abstract ControlField newControlField(String tag, String data);
/**
* Returns a new data field instance.
*
* @return DataField
*/
public abstract DataField newDataField();
/**
* Creates a new data field with the given tag and indicators and returns
* the instance.
*
* @return DataField
*/
public abstract DataField newDataField(String tag, char ind1, char ind2);
/**
* Creates a new data field with the given tag and indicators and subfields
* and returns the instance.
*
* @return DataField
*/
public abstract DataField newDataField(String tag, char ind1, char ind2,
String... subfieldCodesAndData);
/**
* Returns a new leader instance.
*
* @return Leader
*/
public abstract Leader newLeader();
/**
* Creates a new leader with the given String
object.
*
* @return Leader
*/
public abstract Leader newLeader(String ldr);
/**
* Returns a new record instance.
*
* @return Record
*/
public abstract Record newRecord();
/**
* Returns a new record instance.
*
* @return Record
*/
public abstract Record newRecord(Leader leader);
/**
* Returns a new record instance.
*
* @return Record
*/
public abstract Record newRecord(String leader);
/**
* Returns a new subfield instance.
*
* @return Leader
*/
public abstract Subfield newSubfield();
/**
* Creates a new subfield with the given identifier.
*
* @return Subfield
*/
public abstract Subfield newSubfield(char code);
/**
* Creates a new subfield with the given identifier and data.
*
* @return Subfield
*/
public abstract Subfield newSubfield(char code, String data);
/**
* Returns true
if the {@link Record} is valid; else,
* false
.
*
* @param record
* @return
*/
public boolean validateRecord(final Record record) {
if (record.getLeader() == null) {
return false;
}
for (final ControlField controlField : record.getControlFields()) {
if (!validateControlField(controlField)) {
return false;
}
}
for (final DataField dataField : record.getDataFields()) {
if (!validateDataField(dataField)) {
return false;
}
}
return true;
}
/**
* Returns true
if supplied {@link VariableField} is valid;
* else, false
.
*
* @param field
* @return
*/
public boolean validateVariableField(final VariableField field) {
return field.getTag() != null;
}
/**
* Returns true
if supplied {@link ControlField} is valid;
* else, false
.
*
* @param field
* @return
*/
public boolean validateControlField(final ControlField field) {
return validateVariableField(field) && field.getData() != null;
}
/**
* Returns true
if supplied {@link DataField} is valid; else,
* false
.
*
* @param field
* @return
*/
public boolean validateDataField(final DataField field) {
if (!validateVariableField(field)) {
return false;
}
if (field.getIndicator1() == 0 || field.getIndicator2() == 0) {
return false;
}
for (final Subfield subfield : field.getSubfields()) {
if (!validateSubField(subfield)) {
return false;
}
}
return true;
}
/**
* Returns true
if the supplied {@link Subfield} is value;
* else, false
.
*
* @param subfield
* @return
*/
public boolean validateSubField(final Subfield subfield) {
return subfield.getCode() != 0 && subfield.getData() != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy