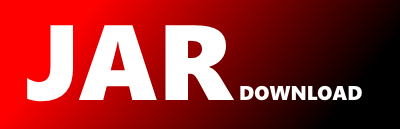
info.freelibrary.iiif.presentation.v3.AnnotationPage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jiiify-presentation-v3 Show documentation
Show all versions of jiiify-presentation-v3 Show documentation
A Java Library for version 3 of the IIIF Presentation API
package info.freelibrary.iiif.presentation.v3;
import java.net.URI;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonGetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonSetter;
import info.freelibrary.util.Logger;
import info.freelibrary.util.LoggerFactory;
import info.freelibrary.util.warnings.PMD;
import info.freelibrary.iiif.presentation.v3.ids.Minter;
import info.freelibrary.iiif.presentation.v3.properties.Behavior;
import info.freelibrary.iiif.presentation.v3.properties.Homepage;
import info.freelibrary.iiif.presentation.v3.properties.Label;
import info.freelibrary.iiif.presentation.v3.properties.Metadata;
import info.freelibrary.iiif.presentation.v3.properties.PartOf;
import info.freelibrary.iiif.presentation.v3.properties.Provider;
import info.freelibrary.iiif.presentation.v3.properties.Rendering;
import info.freelibrary.iiif.presentation.v3.properties.RequiredStatement;
import info.freelibrary.iiif.presentation.v3.properties.SeeAlso;
import info.freelibrary.iiif.presentation.v3.properties.Summary;
import info.freelibrary.iiif.presentation.v3.properties.behaviors.ResourceBehavior;
import info.freelibrary.iiif.presentation.v3.utils.JsonKeys;
import info.freelibrary.iiif.presentation.v3.utils.MessageCodes;
/**
* A collection of {@link Annotation}(s) included in the items property of the Canvas (and whose target is that Canvas).
*/
@SuppressWarnings(PMD.GOD_CLASS)
public class AnnotationPage> extends AbstractResource> // NOPMD
implements Resource> {
/**
* The logger used by the AnnotationPage.
*/
private static final Logger LOGGER = LoggerFactory.getLogger(AnnotationPage.class, MessageCodes.BUNDLE);
/**
* The AnnotationPage's annotations.
*/
private List myAnnotations;
/**
* Creates a new annotation page.
*
* @param aID An annotation page ID in string form
*/
public AnnotationPage(final String aID) {
super(ResourceTypes.ANNOTATION_PAGE, aID);
}
/**
* Creates a new annotation page.
*
* @param aID An annotation page ID
*/
public AnnotationPage(final URI aID) {
super(ResourceTypes.ANNOTATION_PAGE, aID);
}
/**
* Creates a new annotation page for a supplied {@link CanvasResource}.
*
* @param The type of CanvasResource
* @param aMinter An ID minter
* @param aCanvas A canvas resource
*/
public > AnnotationPage(final Minter aMinter, final CanvasResource aCanvas) {
super(ResourceTypes.ANNOTATION_PAGE, aMinter.getAnnotationPageID(aCanvas));
}
/**
* Allows Jackson to deserialize JSON.
*/
private AnnotationPage() {
super(ResourceTypes.ANNOTATION_PAGE);
}
@Override
@JsonSetter(JsonKeys.PROVIDER)
public AnnotationPage setProviders(final Provider... aProviderArray) {
return setProviders(Arrays.asList(aProviderArray));
}
@Override
@JsonIgnore
public AnnotationPage setProviders(final List aProviderList) {
return (AnnotationPage) super.setProviders(aProviderList);
}
/**
* Sets the annotation page's annotations.
*
* @param aAnnotationArray An annotation array
* @return The annotation page
*/
@JsonSetter(JsonKeys.ITEMS)
@SafeVarargs
public final AnnotationPage setAnnotations(final T... aAnnotationArray) {
if (myAnnotations != null) {
myAnnotations.clear();
}
return addAnnotations(aAnnotationArray);
}
/**
* Sets the annotation page's annotations.
*
* @param aAnnotationList A list of annotations
* @return The annotation page
*/
@JsonIgnore
public final AnnotationPage setAnnotations(final List aAnnotationList) {
if (myAnnotations != null) {
myAnnotations.clear();
}
return addAnnotations(aAnnotationList);
}
/**
* Adds annotations to the annotation page.
*
* @param aAnnotationArray Annotations to be added to the annotation page
* @return The annotation page
*/
@SafeVarargs
public final AnnotationPage addAnnotations(final T... aAnnotationArray) {
if (!Collections.addAll(getAnnotations(), Objects.requireNonNull(aAnnotationArray))) {
final String details = getListIDs(Arrays.asList(aAnnotationArray));
throw new UnsupportedOperationException(LOGGER.getMessage(MessageCodes.JPA_050, details));
}
return this;
}
/**
* Adds annotations to the annotation page.
*
* @param aAnnotationList Annotations to be added to the annotation page
* @return The annotation page
*/
public final AnnotationPage addAnnotations(final List aAnnotationList) {
if (!getAnnotations().addAll(Objects.requireNonNull(aAnnotationList))) {
final String details = getListIDs(aAnnotationList);
throw new UnsupportedOperationException(LOGGER.getMessage(MessageCodes.JPA_050, details));
}
return this;
}
/**
* Gets the annotation page's annotations.
*
* @return The annotation page's annotations
*/
@JsonGetter(JsonKeys.ITEMS)
public List getAnnotations() {
if (myAnnotations == null) {
myAnnotations = new ArrayList<>();
}
return myAnnotations;
}
@Override
public AnnotationPage clearBehaviors() {
return (AnnotationPage) super.clearBehaviors();
}
@Override
@JsonSetter(JsonKeys.BEHAVIOR)
public AnnotationPage setBehaviors(final Behavior... aBehaviorArray) {
return (AnnotationPage) super.setBehaviors(checkBehaviors(ResourceBehavior.class, true, aBehaviorArray));
}
@Override
public AnnotationPage setBehaviors(final List aBehaviorList) {
return (AnnotationPage) super.setBehaviors(checkBehaviors(ResourceBehavior.class, true, aBehaviorList));
}
@Override
public AnnotationPage addBehaviors(final Behavior... aBehaviorArray) {
return (AnnotationPage) super.addBehaviors(checkBehaviors(ResourceBehavior.class, false, aBehaviorArray));
}
@Override
public AnnotationPage addBehaviors(final List aBehaviorList) {
return (AnnotationPage) super.addBehaviors(checkBehaviors(ResourceBehavior.class, false, aBehaviorList));
}
@Override
public AnnotationPage setSeeAlsoRefs(final SeeAlso... aSeeAlsoArray) {
return (AnnotationPage) super.setSeeAlsoRefs(aSeeAlsoArray);
}
@Override
public AnnotationPage setSeeAlsoRefs(final List aSeeAlsoList) {
return (AnnotationPage) super.setSeeAlsoRefs(aSeeAlsoList);
}
@Override
public AnnotationPage setServices(final Service>... aServiceArray) {
return (AnnotationPage) super.setServices(aServiceArray);
}
@Override
public AnnotationPage setServices(final List> aServiceList) {
return (AnnotationPage) super.setServices(aServiceList);
}
@Override
public AnnotationPage setPartOfs(final PartOf... aPartOfArray) {
return (AnnotationPage) super.setPartOfs(aPartOfArray);
}
@Override
public AnnotationPage setPartOfs(final List aPartOfList) {
return (AnnotationPage) super.setPartOfs(aPartOfList);
}
@Override
public AnnotationPage setRenderings(final Rendering... aRenderingArray) {
return (AnnotationPage) super.setRenderings(aRenderingArray);
}
@Override
public AnnotationPage setRenderings(final List aRenderingList) {
return (AnnotationPage) super.setRenderings(aRenderingList);
}
@Override
public AnnotationPage setHomepages(final Homepage... aHomepageArray) {
return (AnnotationPage) super.setHomepages(aHomepageArray);
}
@Override
public AnnotationPage setHomepages(final List aHomepageList) {
return (AnnotationPage) super.setHomepages(aHomepageList);
}
@Override
public AnnotationPage setThumbnails(final ContentResource>... aThumbnailArray) {
return (AnnotationPage) super.setThumbnails(aThumbnailArray);
}
@Override
public AnnotationPage setThumbnails(final List> aThumbnailList) {
return (AnnotationPage) super.setThumbnails(aThumbnailList);
}
@Override
public AnnotationPage setID(final String aID) {
return (AnnotationPage) super.setID(aID);
}
@Override
public AnnotationPage setID(final URI aID) {
return (AnnotationPage) super.setID(aID);
}
@Override
public AnnotationPage setRights(final String aRights) {
return (AnnotationPage) super.setRights(aRights);
}
@Override
public AnnotationPage setRights(final URI aRights) {
return (AnnotationPage) super.setRights(aRights);
}
@Override
public AnnotationPage setRequiredStatement(final RequiredStatement aStatement) {
return (AnnotationPage) super.setRequiredStatement(aStatement);
}
@Override
public AnnotationPage setSummary(final String aSummary) {
return (AnnotationPage) super.setSummary(aSummary);
}
@Override
public AnnotationPage setSummary(final Summary aSummary) {
return (AnnotationPage) super.setSummary(aSummary);
}
@Override
public AnnotationPage setMetadata(final Metadata... aMetadataArray) {
return (AnnotationPage) super.setMetadata(aMetadataArray);
}
@Override
public AnnotationPage setMetadata(final List aMetadataList) {
return (AnnotationPage) super.setMetadata(aMetadataList);
}
@Override
public AnnotationPage setLabel(final String aLabel) {
return (AnnotationPage) super.setLabel(aLabel);
}
@Override
public AnnotationPage setLabel(final Label aLabel) {
return (AnnotationPage) super.setLabel(aLabel);
}
/**
* Gets a string representation of the annotation page.
*
* @return A string representation of the annotation page
*/
@Override
public String toString() {
return String.join(":", getClass().getSimpleName(), getID().toString());
}
/**
* Get the IDs of the annotations in the supplied list and return them as a single string.
*
* @param aAnnotationList A list of annotations
* @return A string containing the IDs
*/
private String getListIDs(final List aAnnotationList) {
final StringBuilder builder = new StringBuilder();
for (final T annotation : aAnnotationList) {
builder.append(annotation.getID()).append('|');
}
if (builder.length() > 0) {
builder.deleteCharAt(builder.length() - 1);
}
return builder.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy