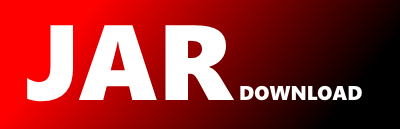
info.freelibrary.iiif.presentation.v3.Resource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jiiify-presentation-v3 Show documentation
Show all versions of jiiify-presentation-v3 Show documentation
A Java Library for version 3 of the IIIF Presentation API
package info.freelibrary.iiif.presentation.v3; // NOPMD
import java.net.URI;
import java.util.List;
import info.freelibrary.util.warnings.PMD;
import info.freelibrary.iiif.presentation.v3.properties.Behavior;
import info.freelibrary.iiif.presentation.v3.properties.Homepage;
import info.freelibrary.iiif.presentation.v3.properties.Label;
import info.freelibrary.iiif.presentation.v3.properties.Metadata;
import info.freelibrary.iiif.presentation.v3.properties.PartOf;
import info.freelibrary.iiif.presentation.v3.properties.Provider;
import info.freelibrary.iiif.presentation.v3.properties.Rendering;
import info.freelibrary.iiif.presentation.v3.properties.RequiredStatement;
import info.freelibrary.iiif.presentation.v3.properties.SeeAlso;
import info.freelibrary.iiif.presentation.v3.properties.Summary;
/**
* An interface that defines methods relevant to all resources.
*
* @param The class that implements {@code Resource}
*/
@SuppressWarnings(PMD.EXCESSIVE_PUBLIC_COUNT)
public interface Resource> {
/**
* Gets the resource label.
*
* @return The resource's label
*/
Label getLabel();
/**
* Sets the resource label from the supplied string.
*
* @param aLabel The string form of the label to set
* @return The resource
*/
T setLabel(String aLabel);
/**
* Sets the resource label.
*
* @param aLabel The resource's label
* @return The resource
*/
T setLabel(Label aLabel);
/**
* Gets the resource metadata.
*
* @return The resource's metadata
*/
List getMetadata();
/**
* Sets the resource metadata.
*
* @param aMetadataArray An array of metadata properties
* @return The resource
*/
T setMetadata(Metadata... aMetadataArray);
/**
* Sets the resource metadata.
*
* @param aMetadataList A list of metadata properties
* @return The resource
*/
T setMetadata(List aMetadataList);
/**
* Gets the resource summary.
*
* @return The resource's summary
*/
Summary getSummary();
/**
* Sets the resource summary.
*
* @param aSummary A summary in string form
* @return The resource
*/
T setSummary(String aSummary);
/**
* Sets the resource summary.
*
* @param aSummary A resource's summary
* @return The resource
*/
T setSummary(Summary aSummary);
/**
* Gets a list of resource thumbnails. A thumbnail can be any type of content resource, not just
* {@link ImageContent}.
*
* @return The resource's thumbnails
*/
List> getThumbnails();
/**
* Sets the thumbnails for this resource. A thumbnail can be any type of content resource, not just
* {@link ImageContent}.
*
* @param aThumbnailArray The thumbnails to set for this resource
* @return The resource
*/
T setThumbnails(ContentResource>... aThumbnailArray);
/**
* Sets the thumbnails for this resource. A thumbnail can be any type of content resource, not just
* {@link ImageContent}.
*
* @param aThumbnailList The thumbnails to set for this resource
* @return The resource
*/
T setThumbnails(List> aThumbnailList);
/**
* Gets the resource's required statement.
*
* @return The required statement of the resource
*/
RequiredStatement getRequiredStatement();
/**
* Sets the resource's required statement.
*
* @param aStatement A required statement
* @return The resource
*/
T setRequiredStatement(RequiredStatement aStatement);
/**
* Gets the resource's rights URI.
*
* @return The rights URI
*/
URI getRights();
/**
* Sets the resource's rights URI.
*
* @param aRights A rights URI
* @return The resource
*/
T setRights(URI aRights);
/**
* Sets the resource's rights URI from the supplied string.
*
* @param aRights A resource's rights URI in string form
* @return The resource
*/
T setRights(String aRights);
/**
* Gets a list of resource homepages.
*
* @return The resource's homepages
*/
List getHomepages();
/**
* Sets the homepages for this resource.
*
* @param aHomepageArray The homepages to set for this resource
* @return The resource
*/
T setHomepages(Homepage... aHomepageArray);
/**
* Sets the homepages for this resource.
*
* @param aHomepageList The homepages to set for this resource
* @return The resource
*/
T setHomepages(List aHomepageList);
/**
* Gets a list of resource providers.
*
* @return The resource's providers
*/
List getProviders();
/**
* Sets the providers for this resource.
*
* @param aProviderArray The providers to set for this resource
* @return The resource
*/
T setProviders(Provider... aProviderArray);
/**
* Sets the providers for this resource.
*
* @param aProviderList The providers to set for this resource
* @return The resource
*/
T setProviders(List aProviderList);
/**
* Gets a list of resource renderings.
*
* @return The resource's renderings
*/
List getRenderings();
/**
* Sets the renderings for this resource.
*
* @param aRenderingArray The renderings to set for this resource
* @return The resource
*/
T setRenderings(Rendering... aRenderingArray);
/**
* Sets the renderings for this resource.
*
* @param aRenderingList The renderings to set for this resource
* @return The resource
*/
T setRenderings(List aRenderingList);
/**
* Gets the resource ID.
*
* @return The resource's ID
*/
URI getID();
/**
* Sets the resource ID from the supplied string.
*
* @param aID A resource ID in string form
* @return The resource
*/
T setID(String aID);
/**
* Sets the resource ID.
*
* @param aID A resource ID
* @return The resource
*/
T setID(URI aID);
/**
* Gets a list of resource partOfs.
*
* @return The resource's partOfs
*/
List getPartOfs();
/**
* Sets the partOfs for this resource.
*
* @param aPartOfArray The partOfs to set for this resource
* @return The resource
*/
T setPartOfs(PartOf... aPartOfArray);
/**
* Sets the partOfs for this resource.
*
* @param aPartOfList The partOfs to set for this resource
* @return The resource
*/
T setPartOfs(List aPartOfList);
/**
* Gets the resource type.
*
* @return The resource's type
*/
String getType();
/**
* Gets the resource's behaviors in an unmodifiable list.
*
* @return The resource's behaviors
*/
List getBehaviors();
/**
* Sets the behaviors for this resource. Different types of resources allow different types of behaviors. For
* instance, on a Manifest
resource the setBehaviors(Behavior aBehavior)
method only
* allows a ManifestBehavior to be passed. If a CollectionBehavior, for instance, is passed, an
* IllegalArgumentException
will be thrown. Manifests, collections, canvases, and ranges have their own
* behaviors. Other resources use the ResourceBehavior
class.
*
* @param aBehaviorArray The behaviors to set for this resource
* @return The resource
* @throws IllegalArgumentException If a passed behavior is not appropriate for the type of resource in hand
*/
T setBehaviors(Behavior... aBehaviorArray);
/**
* Sets the behaviors for this resource. Different types of resources allow different types of behaviors. For
* instance, on a Manifest
resource the setBehaviors(List<Behavior> aBehaviorList)
* method only allows a ManifestBehavior to be passed. If a CollectionBehavior, for instance, is passed, an
* IllegalArgumentException
will be thrown. Manifests, collections, canvases, and ranges have their own
* behaviors. Other resources use the ResourceBehavior
class.
*
* @param aBehaviorList The behaviors to set for this resource
* @return The resource
* @throws IllegalArgumentException If a passed behavior is not appropriate for the type of resource in hand
*/
T setBehaviors(List aBehaviorList);
/**
* Adds behaviors to the resource.
*
* @param aBehaviorArray An array of behaviors to add to the resource
* @return The resource
*/
T addBehaviors(Behavior... aBehaviorArray);
/**
* Adds behaviors to the resource.
*
* @param aBehaviorList A list of behaviors to add to the resource
* @return The resource
*/
T addBehaviors(List aBehaviorList);
/**
* Removes the behaviors associated with this resource.
*
* @return The resource
*/
T clearBehaviors();
/**
* Gets see also reference(s).
*
* @return The see also reference(s)
*/
List getSeeAlsoRefs();
/**
* Sets see also reference(s).
*
* @param aSeeAlsoArray See also reference(s)
* @return The resource
*/
T setSeeAlsoRefs(SeeAlso... aSeeAlsoArray);
/**
* Sets see also reference(s).
*
* @param aSeeAlsoList See also reference(s)
* @return The resource
*/
T setSeeAlsoRefs(List aSeeAlsoList);
/**
* Gets a list of resource services.
*
* @return The resource's services
*/
List> getServices();
/**
* Sets the services for this resource.
*
* @param aServiceArray The services to set for this resource
* @return The resource
*/
T setServices(Service>... aServiceArray);
/**
* Sets the services for this resource.
*
* @param aServiceList The services to set for this resource
* @return The resource
*/
T setServices(List> aServiceList);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy