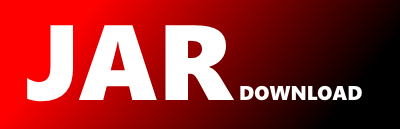
info.freelibrary.iiif.presentation.v3.services.OtherService2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jiiify-presentation-v3 Show documentation
Show all versions of jiiify-presentation-v3 Show documentation
A Java Library for version 3 of the IIIF Presentation API
package info.freelibrary.iiif.presentation.v3.services;
import java.net.URI;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.Optional;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonValue;
import info.freelibrary.iiif.presentation.v3.MediaType;
import info.freelibrary.iiif.presentation.v3.Service;
import info.freelibrary.iiif.presentation.v3.utils.JsonKeys;
/**
* A generic service class for older service implementations that use @id and @type.
*/
public class OtherService2 extends AbstractOtherService implements OtherService {
/**
* Creates a service for the supplied URI.
*
* @param aServiceID A service ID
* @param aType A service type
*/
public OtherService2(final URI aServiceID, final String aType) {
super();
myID = aServiceID;
myType = aType;
}
/**
* Creates a service for the supplied ID.
*
* @param aServiceID A service ID in string form
* @param aType A service type
*/
public OtherService2(final String aServiceID, final String aType) {
super();
myID = URI.create(aServiceID);
myType = aType;
}
/**
* Sets the profile URI for this service.
*
* @param aProfile A profile URI for this service
* @return This service
*/
@JsonIgnore
public OtherService2 setProfile(final OtherService2.Profile aProfile) {
super.setProfile(aProfile);
return this;
}
/**
* Sets the profile URI for this service.
*
* @param aProfile A profile URI for this service
* @return This service
*/
@Override
@JsonIgnore
public OtherService2 setProfile(final String aProfile) {
super.setProfile(Profile.fromString(aProfile));
return this;
}
@Override
@JsonIgnore
public Optional getProfile() {
return Optional.ofNullable(myProfile);
}
@Override
@JsonIgnore
public OtherService2 setID(final URI aID) {
return super.setID(aID);
}
@Override
@JsonIgnore
public OtherService2 setID(final String aID) {
return super.setID(aID);
}
@Override
@JsonIgnore
public OtherService2 setType(final String aType) {
myType = aType;
return this;
}
/**
* Sets the format from a file extension or media type.
*
* @param aMediaType A string representation of media type or file extension
* @return The Service
*/
@Override
@JsonIgnore
public OtherService2 setFormat(final String aMediaType) {
myFormat = MediaType.fromString(aMediaType).orElse(null);
return this;
}
/**
* Sets the format of the image.
*
* @param aMediaType A media type
* @return The service
*/
@Override
@JsonIgnore
public OtherService2 setFormat(final MediaType aMediaType) {
myFormat = aMediaType;
return this;
}
/**
* Gets the media type format of the image.
*
* @return The media type format of the image
*/
@JsonIgnore
public Optional getFormat() {
return Optional.ofNullable(myFormat);
}
/**
* Converts the other service (v2) to an object that JSON can use in serialization.
*
* @return An object for Jackson to use in its serialization process.
*/
@JsonValue
protected Object toJsonValue() {
if (myID != null) {
final LinkedHashMap map = new LinkedHashMap<>();
if (myID != null) {
map.put(JsonKeys.V2_ID, myID);
}
if (myType != null) {
map.put(JsonKeys.V2_TYPE, myType);
}
if (myProfile != null) {
map.put(JsonKeys.PROFILE, myProfile);
}
if (myFormat != null) {
map.put(JsonKeys.FORMAT, getFormat());
}
if (myServices != null && myServices.size() > 0) {
map.put(JsonKeys.SERVICE, myServices);
}
return Collections.unmodifiableMap(map);
} else {
return null;
}
}
/**
* An other service (v2) profile.
*/
public static class Profile implements OtherService.Profile {
/**
* The string representation of the OtherService profile.
*/
private final String myProfile;
/**
* Creates a profile from the supplied string.
*
* @param aProfile
*/
public Profile(final String aProfile) {
myProfile = aProfile;
}
@Override
public String string() {
return myProfile;
}
@Override
public URI uri() {
return URI.create(myProfile);
}
/**
* Creates a profile from the supplied string.
*
* @param aProfile A string form of a service profile
* @return The OtherService profile for the supplied string value
*/
public static Profile fromString(final String aProfile) {
return new Profile(aProfile);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy