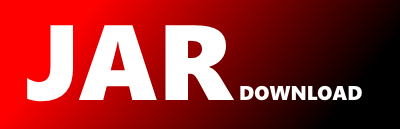
info.freelibrary.iiif.presentation.v3.services.image.ImageService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jiiify-presentation-v3 Show documentation
Show all versions of jiiify-presentation-v3 Show documentation
A Java Library for version 3 of the IIIF Presentation API
package info.freelibrary.iiif.presentation.v3.services.image;
import java.net.URI;
import java.util.List;
import info.freelibrary.iiif.presentation.v3.Service;
/**
* Interface for image services.
*/
public interface ImageService> extends Service {
/**
* Sets the image service's extra formats.
*
* @param aFormatList A list of extra formats
* @return This image service
*/
T setExtraFormats(List aFormatList);
/**
* Sets the image service's extra formats.
*
* @param aFormatArray A list of extra formats
* @return This image service
*/
T setExtraFormats(ImageAPI.ImageFormat... aFormatArray);
/**
* Gets the image service's extra formats.
*
* @return The list of extra formats
*/
List getExtraFormats();
/**
* Sets the image service's extra qualities.
*
* @param aQualityList A list of extra qualities
* @return This image service
*/
T setExtraQualities(List aQualityList);
/**
* Sets the image service's extra qualities.
*
* @param aQualityArray An array of extra qualities
* @return This image service
*/
T setExtraQualities(ImageAPI.ImageQuality... aQualityArray);
/**
* Gets the image service's extra qualities.
*
* @return The list of extra qualities
*/
List getExtraQualities();
/**
* Gets the protocol for an Image API service.
*
* @return The image service protocol
*/
URI getProtocol();
/**
* Sets whether the protocol should be included in the output JSON.
*
* @param aProtocolFlag A protocol flag
* @return The image service
*/
T setProtocol(boolean aProtocolFlag);
/**
* Sets the service's tiles from a list of tiles.
*
* @param aTileList A list of tiles for the service
* @return This image service
*/
T setTiles(List aTileList);
/**
* Sets the service's tiles from an array of tiles.
*
* @param aTileArray An array of tiles for the service
* @return This image service
*/
T setTiles(Tile... aTileArray);
/**
* Gets the service's tiles.
*
* @return A list of tiles supported by the service
*/
List getTiles();
/**
* Sets the service's sizes from a list of sizes.
*
* @param aSizeList A list of sizes for the service
* @return This image service
*/
T setSizes(List aSizeList);
/**
* Sets the service's sizes from an array of sizes.
*
* @param aSizeArray An array of sizes for the service
* @return This image service
*/
T setSizes(Size... aSizeArray);
/**
* Gets the service's sizes.
*
* @return A list of sizes supported by the service
*/
List getSizes();
/**
* Interface for {@link ImageService} profiles.
*/
interface Profile extends Service.Profile {
@Override
String string();
@Override
URI uri();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy