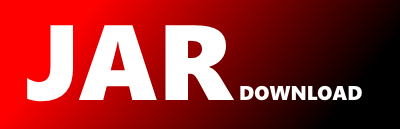
info.freelibrary.maven.LLP2JLPMojo Maven / Gradle / Ivy
The newest version!
package info.freelibrary.maven;
import org.apache.maven.plugins.annotations.Component;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugin.logging.Log;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.model.Profile;
import org.apache.maven.project.MavenProject;
import info.freelibrary.util.FileUtils;
import info.freelibrary.util.StringUtils;
import java.io.File;
import java.io.IOException;
import java.util.List;
import java.util.Properties;
/**
* Goal which copies native files from the LD_LIBRARY_PATH
to the
* java.library.path
.
*/
@Mojo(name = "copy", defaultPhase = LifecyclePhase.GENERATE_RESOURCES)
public class LLP2JLPMojo extends AbstractMojo {
/**
* A string possible used in the LD_LIBRARY_PATH variable declaration.
*/
private static String BASEDIR_PROPERTY = "${project.basedir}";
/**
* The Maven project from which this plugin is being run.
*/
@Component
private MavenProject myProject;
/**
* Executes the LLP2JLP-Maven-Plugin, copying the files from the file system
* represented by the ${LD_LIBRARY_PATH} variable to the file system
* represented by the ${java.library.path} variable. These should be defined
* in a Maven profile, in the case of the LD_LIBRARY_PATH, and the
* ${MAVEN_OPTS} variable, in the case of the java.library.path.
*/
public void execute() throws MojoExecutionException {
String jlp = System.getProperty("java.library.path");
Log log = getLog();
List> profiles = myProject.getActiveProfiles();
for (int index = 0; index < profiles.size(); index++) {
Profile profile = (Profile) profiles.get(index);
Properties properties = profile.getProperties();
String llp = properties.getProperty("LD_LIBRARY_PATH");
// If that's not found, perhaps this is a Mac build?
if (llp == null) {
llp = properties.getProperty("DYLD_LIBRARY_PATH");
}
if (llp == null) {
continue; // this isn't a project specific profile
}
if (jlp != null && !jlp.contains(":")) {
File jlpDir = new File(jlp);
File llpDir;
if (llp.startsWith(BASEDIR_PROPERTY)) {
llp = llp.substring(BASEDIR_PROPERTY.length());
llpDir = new File(myProject.getBasedir(), llp);
} else {
if (log.isDebugEnabled()) {
log.debug(llp + " wasn't a basedir path");
}
llpDir = new File(llp);
}
if (log.isInfoEnabled()) {
log.info(StringUtils.formatMessage(
"Copying files from {} to {}", new String[] {
llpDir.getAbsolutePath(),
jlpDir.getAbsolutePath()}));
}
try {
FileUtils.copy(llpDir, jlpDir);
} catch (IOException details) {
throw new MojoExecutionException("Couldn't copy files",
details);
}
} else {
throw new MojoExecutionException(
"java.library.path should be set in MAVEN_OPTS");
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy