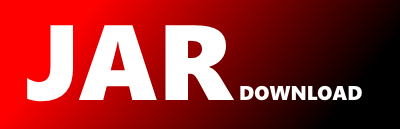
info.freelibrary.vertx.s3.HttpHeaders Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-s3-service Show documentation
Show all versions of vertx-s3-service Show documentation
An S3 client library for the Vert.x toolkit
package info.freelibrary.vertx.s3;
import static info.freelibrary.util.Constants.COMMA;
import static info.freelibrary.util.Constants.VERTICAL_BAR;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import io.vertx.codegen.annotations.DataObject;
import io.vertx.core.MultiMap;
import io.vertx.core.json.JsonObject;
/**
* HTTP headers and methods to support working with them.
*/
@DataObject
public class HttpHeaders implements io.vertx.core.http.HttpHeaders {
/**
* Header patterns, used to match headers.
*/
private static final String HEADER_PATTERN = String.join("|", ACCEPT, ACCEPT_CHARSET, ACCEPT_ENCODING,
ACCEPT_LANGUAGE, CONNECTION, ACCESS_CONTROL_ALLOW_HEADERS, ACCESS_CONTROL_ALLOW_METHODS,
ACCESS_CONTROL_ALLOW_ORIGIN, ACCESS_CONTROL_EXPOSE_HEADERS, ACCESS_CONTROL_REQUEST_HEADERS, CONTENT_LANGUAGE,
ACCESS_CONTROL_REQUEST_METHOD);
/**
* A header multi-map.
*/
private final MultiMap myMultiMap;
/**
* Creates a new HttpHeaders object.
*/
public HttpHeaders() {
myMultiMap = io.vertx.core.http.HttpHeaders.headers();
}
/**
* Creates a new HttpHeaders object from the supplied JsonObject.
*
* @param aHeadersSerialization A JSON representation of the HttpHeaders object
*/
public HttpHeaders(final JsonObject aHeadersSerialization) {
myMultiMap = io.vertx.core.http.HttpHeaders.headers();
aHeadersSerialization.forEach(entry -> {
final String serializedValue = entry.getValue().toString();
final String key = entry.getKey();
// If we have a value with a vertical bar, it has multiple values
if (serializedValue.contains(VERTICAL_BAR)) {
for (final String value : serializedValue.split(VERTICAL_BAR)) {
myMultiMap.add(key, value);
}
} else {
myMultiMap.add(key, serializedValue);
}
});
}
/**
* Creates a new HttpHeaders object.
*
* @param aMap A map of header names and values
*/
public HttpHeaders(final Map aMap) {
myMultiMap = io.vertx.core.http.HttpHeaders.headers();
myMultiMap.addAll(aMap);
}
/**
* Creates a new HttpHeaders object from a Vert.x MultiMap.
*
* @param aMultiMap A MultiMap generated by Vert.x
*/
HttpHeaders(final MultiMap aMultiMap) {
myMultiMap = aMultiMap;
}
/**
* Gets the value of the supplied header.
*
* @param aName A header name
* @return The value of a requested header
*/
public String get(final CharSequence aName) {
return myMultiMap.get(aName);
}
/**
* Gets all the values of the supplied header name. If a header is comma delimited, those values are returned
* separate strings.
*
* @param aName A header name
* @return The values of the requested header
*/
public List getAll(final CharSequence aName) {
final String name = aName.toString().toLowerCase(Locale.US);
final List list = new ArrayList<>();
if (name.matches(HEADER_PATTERN)) {
for (final String values : myMultiMap.getAll(aName)) {
Collections.addAll(list, values.split(COMMA));
}
} else {
list.addAll(myMultiMap.getAll(aName));
}
return list;
}
/**
* Tests whether the headers contain a header for the supplied name.
*
* @param aName A header name
* @return True if the headers contain the supplied header name; else, false
*/
public boolean contains(final CharSequence aName) {
return myMultiMap.contains(aName);
}
/**
* Tests whether there are any headers.
*
* @return True if there are no headers; else, false
*/
public boolean isEmpty() {
return myMultiMap.isEmpty();
}
/**
* Gets the names of all the headers.
*
* @return The names of all the headers
*/
public Set names() {
return myMultiMap.names();
}
/**
* Adds the supplied header.
*
* @param aName A header name
* @param aValue A header value
* @return The headers
*/
public HttpHeaders add(final CharSequence aName, final CharSequence aValue) {
myMultiMap.add(aName, aValue);
return this;
}
/**
* Adds all the headers in the supplied HttpHeaders.
*
* @param aHeaders A headers object
* @return The headers
*/
public HttpHeaders addAll(final HttpHeaders aHeaders) {
myMultiMap.addAll(aHeaders.myMultiMap);
return this;
}
/**
* Adds all the headers in the supplied map.
*
* @param aHeadersMap A map of headers
* @return The headers
*/
public HttpHeaders addAll(final Map aHeadersMap) {
myMultiMap.addAll(aHeadersMap);
return this;
}
/**
* Sets a header.
*
* @param aName A header name
* @param aValue A header value
* @return The headers
*/
public HttpHeaders set(final CharSequence aName, final CharSequence aValue) {
myMultiMap.set(aName, aValue);
return this;
}
/**
* Sets all the supplied headers.
*
* @param aHeaders A headers object
* @return The headers
*/
public HttpHeaders setAll(final HttpHeaders aHeaders) {
myMultiMap.setAll(aHeaders.myMultiMap);
return this;
}
/**
* Sets all the headers in the supplied map.
*
* @param aHeaders A map of headers
* @return The headers
*/
public HttpHeaders setAll(final Map aHeaders) {
myMultiMap.setAll(aHeaders);
return this;
}
/**
* Remove the supplied header.
*
* @param aName A name of a header
* @return The headers
*/
public HttpHeaders remove(final CharSequence aName) {
myMultiMap.remove(aName);
return this;
}
/**
* Clears all the headers.
*
* @return The empty headers
*/
public HttpHeaders clear() {
myMultiMap.clear();
return this;
}
/**
* The number of headers.
*
* @return The number of headers
*/
public int size() {
return myMultiMap.size();
}
@Override
public String toString() {
return myMultiMap.toString();
}
/**
* Returns a JSON representation of the HttpHeaders.
*
* @return A JSON representation
*/
public JsonObject toJson() {
final Iterator> iterator = myMultiMap.iterator();
final JsonObject jsonObject = new JsonObject();
while (iterator.hasNext()) {
final Entry entry = iterator.next();
final String value = entry.getValue();
final String key = entry.getKey();
// If JsonObject already contains key, append to its value; else, put the value as is
if (jsonObject.containsKey(key)) {
jsonObject.put(key, jsonObject.getString(key) + VERTICAL_BAR + value);
} else {
jsonObject.put(key, value);
}
}
return jsonObject;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy