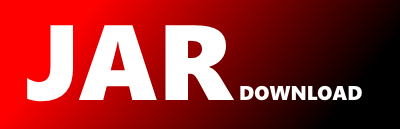
info.kwarc.sally4.mhw.base.impl.MathHubRepository Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mhw-base Show documentation
Show all versions of mhw-base Show documentation
Implements the interaction of assigning a MathHub document to a Worker.
The newest version!
package info.kwarc.sally4.mhw.base.impl;
import info.kwarc.sally4.lmh.factories.LMH;
import info.kwarc.sally4.mhw.base.BuildWorkflowConfig;
import info.kwarc.sally4.mhw.base.IBuildWorkflow;
import info.kwarc.sally4.mhw.base.IBuildWorkflowFactory;
import info.kwarc.sally4.mhw.base.IMathHubRepository;
import info.kwarc.sally4.mhw.base.IMathHubUser;
import info.kwarc.sally4.mhw.base.IMathHubWorker;
import info.kwarc.sally4.os.events.EventBusContext;
import java.util.HashMap;
import java.util.Map.Entry;
import org.apache.felix.ipojo.annotations.Bind;
import org.apache.felix.ipojo.annotations.Component;
import org.apache.felix.ipojo.annotations.Invalidate;
import org.apache.felix.ipojo.annotations.Property;
import org.apache.felix.ipojo.annotations.Provides;
import org.apache.felix.ipojo.annotations.Requires;
import org.apache.felix.ipojo.annotations.ServiceProperty;
import org.apache.felix.ipojo.annotations.Unbind;
import org.apache.felix.ipojo.annotations.Validate;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@Component(name=MathHubRepository.factory_id)
@Provides(specifications=IMathHubRepository.class)
public class MathHubRepository implements IMathHubRepository {
final static public String factory_id="info.kwarc.sally4.mhw.base.impl.MathHubRepository";
Logger log;
@ServiceProperty
String repositoryid;
@ServiceProperty
public String mhwid;
@Requires(id = mathHubUser, filter = "(filterThatWillNeverSucceed=1)")
IMathHubUser user;
@Requires(id = "mathHubWorker", filter = "(filterThatWillNeverSucceed=1)")
IMathHubWorker worker;
String repoAbsolutePath;
@Requires(id = lmhInstance, filter = "(filterThatWillNeverSucceed=1)")
LMH lmh;
@Property
String repoName;
protected MathHubRepository() {
init();
}
public MathHubRepository(LMH lmh, String mhwid, String repoName) {
this.lmh = lmh;
this.repoName = repoName;
this.mhwid = mhwid;
init();
}
HashMap configuredWorkflows;
HashMap buildFactories = new HashMap();
HashMap activeWorkflowsInstances = new HashMap();
HashMap FSEventHandlers = new HashMap();
@Override
public HashMap getBuildFactories() {
return buildFactories;
}
@Override
public HashMap getConfiguredWorkflows() {
return configuredWorkflows;
}
/**
* A new started workflow is created by a factory
* @param newWorkflow
*/
@Bind(aggregate=true, optional=true)
void addBuildWorkflow(final IBuildWorkflow newWorkflow) {
if (!newWorkflow.getRepositoryId().equals(repositoryid))
return;
activeWorkflowsInstances.put(newWorkflow.getExtension(), newWorkflow);
EventBusContext context = lmh.watchFiles(repoName, newWorkflow.getExtension());
FSEventHandlers.put(newWorkflow.getExtension(), new FSEventSubscriber(context, newWorkflow));
}
@Unbind(aggregate=true, optional=true)
void removeBuildWorkflow(IBuildWorkflow newWorkflow) {
if (!newWorkflow.getRepositoryId().equals(repositoryid))
return;
log.info("remove workflow "+newWorkflow.getExtension()+" registered");
activeWorkflowsInstances.remove(newWorkflow.getExtension());
FSEventHandlers.get(newWorkflow.getExtension()).unregister();
}
@Bind(aggregate=true, optional=true)
@Override
public void addBuildFactory(IBuildWorkflowFactory newFactory) {
buildFactories.put(newFactory.getId(), newFactory);
for (Entry pairs : configuredWorkflows.entrySet()) {
String ext = pairs.getKey();
BuildWorkflowConfig conf = pairs.getValue();
if (!conf.getBuildWorkflowId().equals(newFactory.getId())) {
continue;
}
enableBuilders(ext);
}
}
@Override
public IBuildWorkflowFactory getBuildFactory(String workflow_id) {
return buildFactories.get(workflow_id);
}
@Unbind(aggregate=true, optional=true)
@Override
public void removeBuildFactory(IBuildWorkflowFactory newFactory) {
buildFactories.remove(newFactory.getId());
for (Entry pairs : configuredWorkflows.entrySet()) {
String ext = pairs.getKey();
BuildWorkflowConfig conf = pairs.getValue();
if (!conf.getBuildWorkflowId().equals(newFactory.getId())) {
continue;
}
disableBuilders(ext);
}
}
@Override
public void addWorkflowConfig(String extension, BuildWorkflowConfig config) {
configuredWorkflows.put(extension, config);
if (!buildFactories.containsKey(config.getBuildWorkflowId())) {
return;
}
enableBuilders(extension);
}
@Override
public void removeWorkflowConfig(String extension) {
configuredWorkflows.remove(extension);
disableBuilders(extension);
}
void init() {
log = LoggerFactory.getLogger(getClass());
configuredWorkflows = new HashMap();
}
@Validate
void start() throws Exception {
mhwid = worker.getMHWID();
repositoryid = mhwid+"/"+repoName;
repoAbsolutePath = lmh.getAbsolutePath(repoName);
}
@Invalidate
void stop() throws Exception {
}
void disableBuilders(String ext) {
if (!activeWorkflowsInstances.containsKey(ext))
return;
IBuildWorkflow worflow = activeWorkflowsInstances.get(ext);
activeWorkflowsInstances.remove(ext);
worflow.invalidate();
}
void enableBuilders(String ext) {
if (activeWorkflowsInstances.containsKey(ext))
return;
BuildWorkflowConfig config = configuredWorkflows.get(ext);
String wid = config.getBuildWorkflowId();
if (!buildFactories.containsKey(wid)) {
log.warn("Workflow "+wid+" undefined for extension "+ext);
return;
}
try {
buildFactories.get(wid).createWorkflow(ext, config, this);
} catch (Throwable e) {
log.error("Failed enabling BuildWorkflow ",e);
}
}
@Override
public String getRepositoryName() {
return repoName;
}
@Override
public String getAbsolutePath() {
return repoAbsolutePath;
}
@Override
public String getRepositoryid() {
return repositoryid;
}
@Override
public IBuildWorkflow getWorkflowInstance(String extension) {
return activeWorkflowsInstances.get(extension);
}
@Override
public IMathHubUser getUser() {
return user;
}
@Override
public IMathHubWorker getWorker() {
return worker;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy