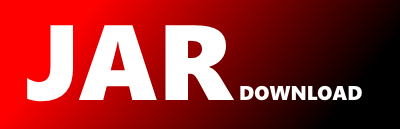
info.kwarc.sally4.servlet.impl.ServletController Maven / Gradle / Ivy
The newest version!
package info.kwarc.sally4.servlet.impl;
import info.kwarc.sally4.components.TemplatingComponent;
import info.kwarc.sally4.processors.FileServeProcessor;
import org.apache.camel.CamelContext;
import org.apache.camel.Component;
import org.apache.camel.builder.RouteBuilder;
import org.apache.camel.component.freemarker.FreemarkerComponent;
public class ServletController {
CamelContext context;
ClassLoader loader;
boolean debugTemplating;
String debugTemplatingPath;
public ServletController(CamelContext context, ClassLoader loader) {
this.context = context;
this.loader = loader;
debugTemplating = false;
debugTemplatingPath="";
}
public ServletController setDebugTemplating(boolean debugTemplating) {
this.debugTemplating = debugTemplating;
return this;
}
public String tpl(String fileName) {
return "templatingComponent:"+debugTemplatingPath+fileName;
}
static String makeSureEndsInSlash(String s) {
if (s.charAt(s.length()-1) == '/') {
return s;
} else {
return s+"/";
}
}
static String makeSureStartsInSlash(String s) {
if (s.charAt(0) == '/') {
return s;
} else {
return "/"+s;
}
}
void replaceTemplatingComponent(Component comp) {
if (context.getComponent("templatingComponent") != null) {
context.removeComponent("templatingComponent");
}
context.addComponent("templatingComponent", comp);
}
public ServletController initDebugTemplating(String fullPath) {
if (debugTemplating) {
fullPath = makeSureEndsInSlash(fullPath);
debugTemplatingPath = "file://"+fullPath;
replaceTemplatingComponent(new FreemarkerComponent());
}
return this;
}
public ServletController initTemplating(String jarPath) {
jarPath = makeSureEndsInSlash(jarPath);
if (!debugTemplating || context.getComponent("templatingComponent")==null) {
replaceTemplatingComponent(new TemplatingComponent(jarPath, loader));
}
return this;
}
public ServletController serveStaticFiles(String servletPath, final String jarPath) {
final String _servletPath = makeSureStartsInSlash(servletPath);
try {
context.addRoutes(new RouteBuilder() {
@Override
public void configure() throws Exception {
from("sallyservlet://"+_servletPath+"?matchOnUriPrefix=true")
.process(new FileServeProcessor(jarPath, loader));
}
});
} catch (Exception e) {
e.printStackTrace();
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy