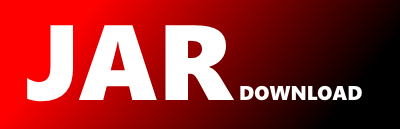
info.kwarc.sally4.theo.impl.TheoInstance Maven / Gradle / Ivy
The newest version!
package info.kwarc.sally4.theo.impl;
import java.util.concurrent.ExecutionException;
import info.kwarc.sally.comm.theo.CloseTheoWindow;
import info.kwarc.sally.comm.theo.OpenTheoWindowRequest;
import info.kwarc.sally.comm.theo.OpenTheoWindowResponse;
import info.kwarc.sally4.components.TemplatingComponent;
import info.kwarc.sally4.docmanager.CallbackManager;
import info.kwarc.sally4.docmanager.SallyMenuItem;
import info.kwarc.sally4.docmanager.impl.SallyMenuItemList;
import info.kwarc.sally4.registration.SallyClient;
import info.kwarc.sally4.servlet.SallyServlet;
import info.kwarc.sally4.theo.Theo;
import org.apache.camel.CamelContext;
import org.apache.camel.Exchange;
import org.apache.camel.Processor;
import org.apache.camel.builder.RouteBuilder;
import org.apache.camel.impl.DefaultCamelContext;
import org.apache.felix.ipojo.annotations.Component;
import org.apache.felix.ipojo.annotations.Invalidate;
import org.apache.felix.ipojo.annotations.Provides;
import org.apache.felix.ipojo.annotations.Requires;
import org.apache.felix.ipojo.annotations.ServiceProperty;
import org.apache.felix.ipojo.annotations.Validate;
import com.google.common.util.concurrent.ListenableFuture;
@Component
@Provides(specifications=Theo.class)
public class TheoInstance extends RouteBuilder implements Theo {
@Requires
SallyServlet sallyServlet;
String frameURL;
@Requires
CallbackManager callbackManager;
@ServiceProperty
String environmentid;
@Requires(id="docQueue", filter="(filterThatWillNeverSucceed=1)")
SallyClient theoClient;
CamelContext context;
@Validate
void doStart() throws Exception {
environmentid = theoClient.getEnvironmentID();
context = new DefaultCamelContext();
context.addComponent("sallyservlet", sallyServlet.getCamelComponent());
context.addRoutes(this);
context.start();
}
@Invalidate
void doStop() throws Exception {
context.stop();
}
Processor prepareItems = new Processor() {
@Override
public void process(Exchange exchange) throws Exception {
String id = exchange.getIn().getHeader("id", String.class);
String item = exchange.getIn().getHeader("item", String.class);
SallyMenuItemList items = callbackManager.get(id, SallyMenuItemList.class);
if (item != null) {
log.info("Closing id "+items.getTheoID());
SallyMenuItem menuItem = items.getItems().get(Integer.parseInt(item));
menuItem.run();
closeWindow(items.getTheoID());
}
exchange.getIn().setHeader("id", id);
exchange.getIn().setBody(items.getItems());
}
};
public TheoInstance() {
}
@Override
public void configure() throws Exception {
getContext().addComponent("freemarker", new TemplatingComponent("templates/", getClass().getClassLoader()));
frameURL = "frameselector"+Long.toHexString(Double.doubleToLongBits(Math.random()));
from("sallyservlet:///"+frameURL)
.process(prepareItems)
.to("freemarker:frameselector.html");
}
@Override
public String openNewWindow(String URL, String title, Integer x, Integer y, Integer width,
Integer height) {
OpenTheoWindowRequest wnd = new OpenTheoWindowRequest();
wnd.setTitle(title);
wnd.setUrl(URL);
if (x != null)
wnd.setPosx(x);
if (y != null)
wnd.setPosy(y);
if (width != null)
wnd.setWidth(width);
if (height != null)
wnd.setHeight(height);
ListenableFuture futureResponse = theoClient.sendRequest(wnd, OpenTheoWindowResponse.class);
OpenTheoWindowResponse response;
try {
response = futureResponse.get();
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
return null;
}
if (response == null)
return null;
return response.getId();
}
@Override
public void closeWindow(String id) {
CloseTheoWindow wnd = new CloseTheoWindow ();
wnd.setId(id);
theoClient.sendEvent(wnd);
}
@Override
public String getEnvironmentId() {
return environmentid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy