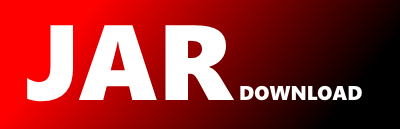
org.ccsds.moims.mo.com.event.consumer.EventAdapter Maven / Gradle / Ivy
The newest version!
package org.ccsds.moims.mo.com.event.consumer;
/**
* Consumer adapter for Event service.
*/
public abstract class EventAdapter extends org.ccsds.moims.mo.mal.consumer.MALInteractionAdapter {
/**
* Called by the MAL when a PubSub register acknowledgement is received from
* a broker for the operation monitorEvent.
*
* @param msgHeader msgHeader The header of the received message.
* @param qosProperties qosProperties The QoS properties associated with the
* message.
*/
public void monitorEventRegisterAckReceived(org.ccsds.moims.mo.mal.transport.MALMessageHeader msgHeader,
java.util.Map qosProperties) {
}
/**
* Called by the MAL when a PubSub register acknowledgement error is received
* from a broker for the operation monitorEvent.
*
* @param msgHeader msgHeader The header of the received message.
* @param error error The received error message.
* @param qosProperties qosProperties The QoS properties associated with the
* message.
*/
public void monitorEventRegisterErrorReceived(org.ccsds.moims.mo.mal.transport.MALMessageHeader msgHeader,
org.ccsds.moims.mo.mal.MOErrorException error,
java.util.Map qosProperties) {
}
/**
* Called by the MAL when a PubSub deregister acknowledgement is received
* from a broker for the operation monitorEvent.
*
* @param msgHeader msgHeader The header of the received message.
* @param qosProperties qosProperties The QoS properties associated with the
* message.
*/
public void monitorEventDeregisterAckReceived(org.ccsds.moims.mo.mal.transport.MALMessageHeader msgHeader,
java.util.Map qosProperties) {
}
/**
* Called by the MAL when a PubSub update is received from a broker for the
* operation monitorEvent.
*
* @param msgHeader msgHeader The header of the received message.
* @param subscriptionId The subscriptionId of the subscription.
* @param updateHeader The Update header.
* @param eventLinks The MAL::EntityKey.firstSubKey shall contain the event
* object number as a base 10 string. For example, for an object number of
* "14" the key value would be "14" with no padding.
* The MAL::EntityKey.secondSubKey shall contain the area, service, and version
* ObjectType fields as a MAL::Long populated as (in hex) 0xAAAASSSSVVXXXXXX
* where AAAA is the area (16 bits), SSSS is the service (16 bits), VV is
* the version (8 bits), and XXXXXX is unused and set to zero (24 bits). For
* example, for an area of "1", and a service of "2",
* and a version of "3" the field would contain (in hex) 0x0001000203000000.
* The MAL::EntityKey.thirdSubKey shall contain the event object instance
* identifier.
* The MAL::EntityKey.fourthSubKey shall contain the area, service, version
* and number fields of the event source ObjectType using same methodology
* as given for the second sub key but replacing the XXXXXX part with the
* number field.
* The related and source links of the event shall populate the ObjectDetails
* part of the publish/notify message.
* The body of the event shall populate the final part of the publish/notify
* message.
* The timestamp of the Event shall be taken from the publish message.
* An event in one domain may be generated by something in another domain
* therefore the domain of the event shall not be required to be the same
* domain as the source of the event.
* @param eventBody eventBody Argument number 3 as defined by the service
* operation.
* @param qosProperties qosProperties The QoS properties associated with the
* message.
*/
public void monitorEventNotifyReceived(org.ccsds.moims.mo.mal.transport.MALMessageHeader msgHeader,
org.ccsds.moims.mo.mal.structures.Identifier subscriptionId,
org.ccsds.moims.mo.mal.structures.UpdateHeader updateHeader,
org.ccsds.moims.mo.com.structures.ObjectDetails eventLinks,
org.ccsds.moims.mo.mal.structures.Element eventBody,
java.util.Map qosProperties) {
}
/**
* Called by the MAL when a PubSub update error is received from a broker
* for the operation monitorEvent.
*
* @param msgHeader msgHeader The header of the received message.
* @param error error The received error message.
* @param qosProperties qosProperties The QoS properties associated with the
* message.
*/
public void monitorEventNotifyErrorReceived(org.ccsds.moims.mo.mal.transport.MALMessageHeader msgHeader,
org.ccsds.moims.mo.mal.MOErrorException error,
java.util.Map qosProperties) {
}
/**
* Called by the MAL when a PubSub register acknowledgement is received from
* a broker.
*
* @param msgHeader msgHeader The header of the received message.
* @param qosProperties qosProperties The QoS properties associated with the
* message.
* @throws org.ccsds.moims.mo.mal.MALException if an error is detected processing
* the message.
*/
public final void registerAckReceived(org.ccsds.moims.mo.mal.transport.MALMessageHeader msgHeader,
java.util.Map qosProperties) throws org.ccsds.moims.mo.mal.MALException {
switch (msgHeader.getOperation().getValue()) {
case org.ccsds.moims.mo.com.event.EventServiceInfo._MONITOREVENT_OP_NUMBER:
monitorEventRegisterAckReceived(msgHeader, qosProperties);
break;
default:
throw new org.ccsds.moims.mo.mal.MALException("Consumer adapter was not expecting operation number " + msgHeader.getOperation().getValue());
}
}
/**
* Called by the MAL when a PubSub register acknowledgement error is received
* from a broker.
*
* @param msgHeader msgHeader The header of the received message.
* @param body body The body of the received message.
* @param qosProperties qosProperties The QoS properties associated with the
* message.
* @throws org.ccsds.moims.mo.mal.MALException if an error is detected processing
* the message.
*/
public final void registerErrorReceived(org.ccsds.moims.mo.mal.transport.MALMessageHeader msgHeader,
org.ccsds.moims.mo.mal.transport.MALErrorBody body,
java.util.Map qosProperties) throws org.ccsds.moims.mo.mal.MALException {
switch (msgHeader.getOperation().getValue()) {
case org.ccsds.moims.mo.com.event.EventServiceInfo._MONITOREVENT_OP_NUMBER:
monitorEventRegisterErrorReceived(msgHeader, body.getError(), qosProperties);
break;
default:
throw new org.ccsds.moims.mo.mal.MALException("Consumer adapter was not expecting operation number " + msgHeader.getOperation().getValue());
}
}
/**
* Called by the MAL when a PubSub update is received from a broker.
*
* @param msgHeader msgHeader The header of the received message.
* @param body body The body of the received message.
* @param qosProperties qosProperties The QoS properties associated with the
* message.
* @throws org.ccsds.moims.mo.mal.MALException if an error is detected processing
* the message.
*/
public final void notifyReceived(org.ccsds.moims.mo.mal.transport.MALMessageHeader msgHeader,
org.ccsds.moims.mo.mal.transport.MALNotifyBody body,
java.util.Map qosProperties) throws org.ccsds.moims.mo.mal.MALException {
if ((org.ccsds.moims.mo.com.COMHelper.COM_AREA_NUMBER.equals(msgHeader.getServiceArea())) && (org.ccsds.moims.mo.com.event.EventServiceInfo.EVENT_SERVICE_NUMBER.equals(msgHeader.getService()))) {
switch (msgHeader.getOperation().getValue()) {
case org.ccsds.moims.mo.com.event.EventServiceInfo._MONITOREVENT_OP_NUMBER:
monitorEventNotifyReceived(msgHeader,
(org.ccsds.moims.mo.mal.structures.Identifier) body.getBodyElement(0, new org.ccsds.moims.mo.mal.structures.Identifier()),
(org.ccsds.moims.mo.mal.structures.UpdateHeader) body.getBodyElement(1, new org.ccsds.moims.mo.mal.structures.UpdateHeader()),
(org.ccsds.moims.mo.com.structures.ObjectDetails) body.getBodyElement(2, new org.ccsds.moims.mo.com.structures.ObjectDetails()),
(org.ccsds.moims.mo.mal.structures.Element) body.getBodyElement(3, null), qosProperties);
break;
default:
throw new org.ccsds.moims.mo.mal.MALException("Consumer adapter was not expecting operation number " + msgHeader.getOperation().getValue());
}
}
else {
notifyReceivedFromOtherService(msgHeader, body, qosProperties);
}
}
/**
* Called by the MAL when a PubSub update error is received from a broker.
*
* @param msgHeader msgHeader The header of the received message.
* @param body body The body of the received message.
* @param qosProperties qosProperties The QoS properties associated with the
* message.
* @throws org.ccsds.moims.mo.mal.MALException if an error is detected processing
* the message.
*/
public final void notifyErrorReceived(org.ccsds.moims.mo.mal.transport.MALMessageHeader msgHeader,
org.ccsds.moims.mo.mal.transport.MALErrorBody body,
java.util.Map qosProperties) throws org.ccsds.moims.mo.mal.MALException {
switch (msgHeader.getOperation().getValue()) {
case org.ccsds.moims.mo.com.event.EventServiceInfo._MONITOREVENT_OP_NUMBER:
monitorEventNotifyErrorReceived(msgHeader, body.getError(), qosProperties);
break;
default:
throw new org.ccsds.moims.mo.mal.MALException("Consumer adapter was not expecting operation number " + msgHeader.getOperation().getValue());
}
}
/**
* Called by the MAL when a PubSub deregister acknowledgement is received
* from a broker.
*
* @param msgHeader msgHeader The header of the received message.
* @param qosProperties qosProperties The QoS properties associated with the
* message.
* @throws org.ccsds.moims.mo.mal.MALException if an error is detected processing
* the message.
*/
public final void deregisterAckReceived(org.ccsds.moims.mo.mal.transport.MALMessageHeader msgHeader,
java.util.Map qosProperties) throws org.ccsds.moims.mo.mal.MALException {
switch (msgHeader.getOperation().getValue()) {
case org.ccsds.moims.mo.com.event.EventServiceInfo._MONITOREVENT_OP_NUMBER:
monitorEventDeregisterAckReceived(msgHeader, qosProperties);
break;
default:
throw new org.ccsds.moims.mo.mal.MALException("Consumer adapter was not expecting operation number " + msgHeader.getOperation().getValue());
}
}
/**
* Called by the MAL when a PubSub update from another service is received
* from a broker.
*
* @param msgHeader msgHeader The header of the received message.
* @param body body The body of the received message.
* @param qosProperties qosProperties The QoS properties associated with the
* message.
* @throws org.ccsds.moims.mo.mal.MALException if an error is detected processing
* the message.
*/
public void notifyReceivedFromOtherService(org.ccsds.moims.mo.mal.transport.MALMessageHeader msgHeader,
org.ccsds.moims.mo.mal.transport.MALNotifyBody body,
java.util.Map qosProperties) throws org.ccsds.moims.mo.mal.MALException {
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy