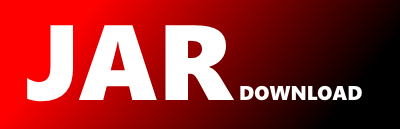
org.ccsds.moims.mo.com.event.consumer.EventStub Maven / Gradle / Ivy
The newest version!
package org.ccsds.moims.mo.com.event.consumer;
/**
* Consumer stub for Event service.
*/
public class EventStub implements org.ccsds.moims.mo.com.event.consumer.Event {
private final org.ccsds.moims.mo.mal.consumer.MALConsumer consumer;
/**
* Wraps a MALconsumer connection with service specific methods that map from
* the high level service API to the generic MAL API.
*
* @param consumer consumer The MALConsumer to use in this stub.
*/
public EventStub(org.ccsds.moims.mo.mal.consumer.MALConsumer consumer) {
this.consumer = consumer;
}
/**
* Returns the internal MAL consumer object used for sending of messages from
* this interface.
*
* @return The MAL consumer object.
*/
public org.ccsds.moims.mo.mal.consumer.MALConsumer getConsumer() {
return consumer;
}
/**
* Register method for the monitorEvent PubSub interaction.
*
* @param subscription subscription the subscription to register for.
* @param adapter adapter Listener in charge of receiving the messages from
* the service provider.
* @throws org.ccsds.moims.mo.mal.MALInteractionException if there is a problem
* during the interaction as defined by the MAL specification.
* @throws org.ccsds.moims.mo.mal.MALException if there is an implementation
* exception.
*/
public void monitorEventRegister(org.ccsds.moims.mo.mal.structures.Subscription subscription,
org.ccsds.moims.mo.com.event.consumer.EventAdapter adapter) throws org.ccsds.moims.mo.mal.MALInteractionException, org.ccsds.moims.mo.mal.MALException {
consumer.register(org.ccsds.moims.mo.com.event.EventServiceInfo.MONITOREVENT_OP, subscription, adapter);
}
/**
* Asynchronous version of method monitorEventRegister.
*
* @param subscription subscription the subscription to register for.
* @param adapter adapter Listener in charge of receiving the messages from
* the service provider.
* @return the MAL message sent to initiate the interaction.
* @throws org.ccsds.moims.mo.mal.MALInteractionException if there is a problem
* during the interaction as defined by the MAL specification.
* @throws org.ccsds.moims.mo.mal.MALException if there is an implementation
* exception.
*/
public org.ccsds.moims.mo.mal.transport.MALMessage asyncMonitorEventRegister(org.ccsds.moims.mo.mal.structures.Subscription subscription,
org.ccsds.moims.mo.com.event.consumer.EventAdapter adapter) throws org.ccsds.moims.mo.mal.MALInteractionException, org.ccsds.moims.mo.mal.MALException {
return consumer.asyncRegister(org.ccsds.moims.mo.com.event.EventServiceInfo.MONITOREVENT_OP, subscription, adapter);
}
/**
* Deregister method for the monitorEvent PubSub interaction.
*
* @param identifierList identifierList the subscription identifiers to deregister.
* @throws org.ccsds.moims.mo.mal.MALInteractionException if there is a problem
* during the interaction as defined by the MAL specification.
* @throws org.ccsds.moims.mo.mal.MALException if there is an implementation
* exception.
*/
public void monitorEventDeregister(org.ccsds.moims.mo.mal.structures.IdentifierList identifierList) throws org.ccsds.moims.mo.mal.MALInteractionException, org.ccsds.moims.mo.mal.MALException {
consumer.deregister(org.ccsds.moims.mo.com.event.EventServiceInfo.MONITOREVENT_OP, identifierList);
}
/**
* Asynchronous version of method monitorEventDeregister.
*
* @param identifierList identifierList the subscription identifiers to deregister.
* @param adapter adapter Listener in charge of receiving the messages from
* the service provider.
* @return the MAL message sent to initiate the interaction.
* @throws org.ccsds.moims.mo.mal.MALInteractionException if there is a problem
* during the interaction as defined by the MAL specification.
* @throws org.ccsds.moims.mo.mal.MALException if there is an implementation
* exception.
*/
public org.ccsds.moims.mo.mal.transport.MALMessage asyncMonitorEventDeregister(org.ccsds.moims.mo.mal.structures.IdentifierList identifierList,
org.ccsds.moims.mo.com.event.consumer.EventAdapter adapter) throws org.ccsds.moims.mo.mal.MALInteractionException, org.ccsds.moims.mo.mal.MALException {
return consumer.asyncDeregister(org.ccsds.moims.mo.com.event.EventServiceInfo.MONITOREVENT_OP, identifierList, adapter);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy