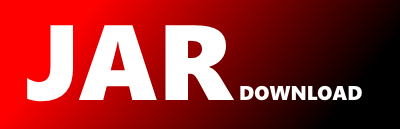
org.ccsds.moims.mo.com.event.provider.EventInheritanceSkeleton Maven / Gradle / Ivy
The newest version!
package org.ccsds.moims.mo.com.event.provider;
/**
* Provider Inheritance skeleton for EventInheritanceSkeleton service.
*/
public abstract class EventInheritanceSkeleton implements org.ccsds.moims.mo.mal.provider.MALInteractionHandler, org.ccsds.moims.mo.com.event.provider.EventSkeleton, org.ccsds.moims.mo.com.event.provider.EventHandler {
private org.ccsds.moims.mo.mal.provider.MALProviderSet providerSet = new org.ccsds.moims.mo.mal.provider.MALProviderSet(org.ccsds.moims.mo.com.event.EventHelper.EVENT_SERVICE);
/**
* Returns the connection object for this provider.
*
* @return the connection object for this provider.
* @throws java.io.IOException if the method was not implemented yet.
*/
public org.ccsds.moims.mo.mal.helpertools.connections.ConnectionProvider getConnection() throws java.io.IOException {
throw new java.io.IOException("This method needs to be overridden!");
}
/**
* Implements the setSkeleton method of the handler interface but does nothing
* as this is the skeleton.
*
* @param skeleton Not used in the inheritance pattern (the skeleton is "this".
*/
public void setSkeleton(org.ccsds.moims.mo.com.event.provider.EventSkeleton skeleton) {
// Not used in the inheritance pattern (the skeleton is 'this');
}
/**
* Adds the supplied MAL provider to the internal list of providers used for
* PubSub.
*
* @param provider The provider to be added.
* @throws org.ccsds.moims.mo.mal.MALException If an error is detected.
*/
public void malInitialize(org.ccsds.moims.mo.mal.provider.MALProvider provider) throws org.ccsds.moims.mo.mal.MALException {
providerSet.addProvider(provider);
}
/**
* Removes the supplied MAL provider from the internal list of providers used
* for PubSub.
*
* @param provider The provider to be added.
* @throws org.ccsds.moims.mo.mal.MALException If an error is detected.
*/
public void malFinalize(org.ccsds.moims.mo.mal.provider.MALProvider provider) throws org.ccsds.moims.mo.mal.MALException {
providerSet.removeProvider(provider);
}
/**
* Creates a publisher object using the current registered provider set for
* the PubSub operation monitorEvent.
*
* @param domain The domain used for publishing.
* @param networkZone The network zone used for publishing.
* @param sessionType The session used for publishing.
* @param sessionName The session name used for publishing.
* @param qos The QoS used for publishing.
* @param qosProps The QoS properties used for publishing.
* @param priority The priority used for publishing.
* @return The new publisher object.
* @throws org.ccsds.moims.mo.mal.MALException if a problem is detected during
* creation of the publisher.
*/
public org.ccsds.moims.mo.com.event.provider.MonitorEventPublisher createMonitorEventPublisher(org.ccsds.moims.mo.mal.structures.IdentifierList domain,
org.ccsds.moims.mo.mal.structures.Identifier networkZone,
org.ccsds.moims.mo.mal.structures.SessionType sessionType,
org.ccsds.moims.mo.mal.structures.Identifier sessionName,
org.ccsds.moims.mo.mal.structures.QoSLevel qos,
java.util.Map qosProps,
org.ccsds.moims.mo.mal.structures.UInteger priority) throws org.ccsds.moims.mo.mal.MALException {
return new org.ccsds.moims.mo.com.event.provider.MonitorEventPublisher(providerSet.createPublisherSet(org.ccsds.moims.mo.com.event.EventServiceInfo.MONITOREVENT_OP, domain, sessionType, sessionName, qos, qosProps, null));
}
/**
* Called by the provider MAL layer on reception of a message to handle the
* interaction.
*
* @param interaction The interaction object.
* @param body The message body.
* @throws org.ccsds.moims.mo.mal.MALException if there is a internal error.
* @throws org.ccsds.moims.mo.mal.MALInteractionException if there is a operation
* interaction error.
*/
public void handleSend(org.ccsds.moims.mo.mal.provider.MALInteraction interaction,
org.ccsds.moims.mo.mal.transport.MALMessageBody body) throws org.ccsds.moims.mo.mal.MALInteractionException, org.ccsds.moims.mo.mal.MALException {
int opNumber = interaction.getOperation().getNumber().getValue();
switch (opNumber) {
default:
throw new org.ccsds.moims.mo.mal.MALInteractionException(new org.ccsds.moims.mo.mal.UnsupportedOperationException(
org.ccsds.moims.mo.mal.provider.MALInteractionHandler.ERROR_MSG_UNSUPPORTED + opNumber));
}
}
/**
* Called by the provider MAL layer on reception of a message to handle the
* interaction.
*
* @param interaction The interaction object.
* @param body The message body.
* @throws org.ccsds.moims.mo.mal.MALException if there is a internal error.
* @throws org.ccsds.moims.mo.mal.MALInteractionException if there is a operation
* interaction error.
*/
public void handleSubmit(org.ccsds.moims.mo.mal.provider.MALSubmit interaction,
org.ccsds.moims.mo.mal.transport.MALMessageBody body) throws org.ccsds.moims.mo.mal.MALInteractionException, org.ccsds.moims.mo.mal.MALException {
int opNumber = interaction.getOperation().getNumber().getValue();
switch (opNumber) {
default:
interaction.sendError(new org.ccsds.moims.mo.mal.UnsupportedOperationException(
org.ccsds.moims.mo.mal.provider.MALInteractionHandler.ERROR_MSG_UNSUPPORTED + opNumber));
throw new org.ccsds.moims.mo.mal.MALInteractionException(new org.ccsds.moims.mo.mal.UnsupportedOperationException(
org.ccsds.moims.mo.mal.provider.MALInteractionHandler.ERROR_MSG_UNSUPPORTED + opNumber));
}
}
/**
* Called by the provider MAL layer on reception of a message to handle the
* interaction.
*
* @param interaction The interaction object.
* @param body The message body.
* @throws org.ccsds.moims.mo.mal.MALException if there is a internal error.
* @throws org.ccsds.moims.mo.mal.MALInteractionException if there is a operation
* interaction error.
*/
public void handleRequest(org.ccsds.moims.mo.mal.provider.MALRequest interaction,
org.ccsds.moims.mo.mal.transport.MALMessageBody body) throws org.ccsds.moims.mo.mal.MALInteractionException, org.ccsds.moims.mo.mal.MALException {
int opNumber = interaction.getOperation().getNumber().getValue();
switch (opNumber) {
default:
interaction.sendError(new org.ccsds.moims.mo.mal.UnsupportedOperationException(
org.ccsds.moims.mo.mal.provider.MALInteractionHandler.ERROR_MSG_UNSUPPORTED + opNumber));
throw new org.ccsds.moims.mo.mal.MALInteractionException(new org.ccsds.moims.mo.mal.UnsupportedOperationException(
org.ccsds.moims.mo.mal.provider.MALInteractionHandler.ERROR_MSG_UNSUPPORTED + opNumber));
}
}
/**
* Called by the provider MAL layer on reception of a message to handle the
* interaction.
*
* @param interaction The interaction object.
* @param body The message body.
* @throws org.ccsds.moims.mo.mal.MALException if there is a internal error.
* @throws org.ccsds.moims.mo.mal.MALInteractionException if there is a operation
* interaction error.
*/
public void handleInvoke(org.ccsds.moims.mo.mal.provider.MALInvoke interaction,
org.ccsds.moims.mo.mal.transport.MALMessageBody body) throws org.ccsds.moims.mo.mal.MALInteractionException, org.ccsds.moims.mo.mal.MALException {
int opNumber = interaction.getOperation().getNumber().getValue();
switch (opNumber) {
default:
interaction.sendError(new org.ccsds.moims.mo.mal.UnsupportedOperationException(
org.ccsds.moims.mo.mal.provider.MALInteractionHandler.ERROR_MSG_UNSUPPORTED + opNumber));
throw new org.ccsds.moims.mo.mal.MALInteractionException(new org.ccsds.moims.mo.mal.UnsupportedOperationException(
org.ccsds.moims.mo.mal.provider.MALInteractionHandler.ERROR_MSG_UNSUPPORTED + opNumber));
}
}
/**
* Called by the provider MAL layer on reception of a message to handle the
* interaction.
*
* @param interaction The interaction object.
* @param body The message body.
* @throws org.ccsds.moims.mo.mal.MALException if there is a internal error.
* @throws org.ccsds.moims.mo.mal.MALInteractionException if there is a operation
* interaction error.
*/
public void handleProgress(org.ccsds.moims.mo.mal.provider.MALProgress interaction,
org.ccsds.moims.mo.mal.transport.MALMessageBody body) throws org.ccsds.moims.mo.mal.MALInteractionException, org.ccsds.moims.mo.mal.MALException {
int opNumber = interaction.getOperation().getNumber().getValue();
switch (opNumber) {
default:
interaction.sendError(new org.ccsds.moims.mo.mal.UnsupportedOperationException(
org.ccsds.moims.mo.mal.provider.MALInteractionHandler.ERROR_MSG_UNSUPPORTED + opNumber));
throw new org.ccsds.moims.mo.mal.MALInteractionException(new org.ccsds.moims.mo.mal.UnsupportedOperationException(
org.ccsds.moims.mo.mal.provider.MALInteractionHandler.ERROR_MSG_UNSUPPORTED + opNumber));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy