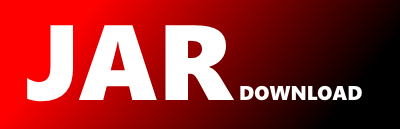
esa.mo.tools.stubgen.docx.DocxWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of generator-docs Show documentation
Show all versions of generator-docs Show documentation
Generates documentation for CCSDS MO service specifications
The newest version!
/* ----------------------------------------------------------------------------
* Copyright (C) 2023 European Space Agency
* European Space Operations Centre
* Darmstadt
* Germany
* ----------------------------------------------------------------------------
* System : CCSDS MO Service Stub Generator
* ----------------------------------------------------------------------------
* Licensed under the European Space Agency Public License, Version 2.0
* You may not use this file except in compliance with the License.
*
* Except as expressly set forth in this License, the Software is provided to
* You on an "as is" basis and without warranties of any kind, including without
* limitation merchantability, fitness for a particular purpose, absence of
* defects or errors, accuracy or non-infringement of intellectual property rights.
*
* See the License for the specific language governing permissions and
* limitations under the License.
* ----------------------------------------------------------------------------
*/
package esa.mo.tools.stubgen.docx;
import esa.mo.tools.stubgen.StubUtils;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.io.Writer;
import org.apache.batik.anim.dom.SVGDOMImplementation;
import org.apache.batik.transcoder.TranscoderException;
import org.apache.batik.transcoder.TranscoderInput;
import org.apache.batik.transcoder.TranscoderOutput;
import org.apache.batik.transcoder.image.PNGTranscoder;
import org.w3c.dom.DOMImplementation;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
/**
*
* @author Cesar.Coelho
*/
public class DocxWriter extends DocxBaseWriter {
public int IMAGE_INDEX = 1;
private final String destinationFolder;
private final Writer file;
private final StringBuffer docxRelBuf;
public DocxWriter(String folder, String className, String ext,
DocxNumberingWriter numberWriter) throws IOException {
super(numberWriter);
destinationFolder = folder;
file = StubUtils.createLowLevelWriter(folder, className, ext);
file.append(makeLine(0, ""));
file.append(makeLine(0, ""));
file.append(makeLine(1, ""));
docxRelBuf = new StringBuffer("");
}
/**
* Adds a diagram.
*
* @param svg The svg to be added.
* @throws IOException if the diagram could not be rasterized.
* @throws TranscoderException if there is a transcoding issue.
*/
public void addDiagram(Object svg) throws IOException, TranscoderException {
if (svg instanceof Element) {
int i = IMAGE_INDEX++;
Element svgElement = (Element) svg;
int[] dims = rasterizeDiagram(svgElement, destinationFolder + "/media", "image" + i);
docxRelBuf.append(" ");
int mx = 5722620 / dims[0];
int my = dims[1] * mx;
StringBuffer b = new StringBuffer("");
b.append(" ");
b.append("");
b.append(" ");
b.append(" ");
b.append("");
b.append(" ");
appendBuffer(b);
}
}
@Override
public void flush() throws IOException {
file.append(getBuffer());
file.append(makeLine(1, " "));
file.append(makeLine(0, " "));
file.flush();
docxRelBuf.append("");
StubUtils.createResource(destinationFolder + "/_rels", "document.xml", "rels", docxRelBuf.toString());
}
/**
* Rasterizes an SVG DOM tree.
*
* @param svg SVG DOM node.
* @param folder Folder to create PNG file in.
* @param name filename without PNG extension.
* @return width and height of rasterized image or null if failed.
* @exception IOException if error.
* @throws org.apache.batik.transcoder.TranscoderException if the
* transcoding could not be performed.
*/
protected int[] rasterizeDiagram(Element svg, String folder, String name) throws IOException, TranscoderException {
int[] dimensions = new int[2];
// Create a Transcoder
PNGTranscoder t = new PNGTranscoder();
// Create a new document.
DOMImplementation impl = SVGDOMImplementation.getDOMImplementation();
String svgNS = SVGDOMImplementation.SVG_NAMESPACE_URI;
Document document = impl.createDocument(svgNS, "svg", null);
Element root = document.getDocumentElement();
dimensions[0] = Integer.valueOf(svg.getAttribute("width"));
dimensions[1] = Integer.valueOf(svg.getAttribute("height"));
root.setAttributeNS(null, "width", svg.getAttribute("width"));
root.setAttributeNS(null, "height", svg.getAttribute("height"));
// Create a duplicate node and transfer ownership of the
// new node into the destination document
Node newNode = document.importNode(svg, true);
// Make the new node an actual item in the target document
root.appendChild(newNode);
// Set the transcoder input and output.
TranscoderInput input = new TranscoderInput(document);
OutputStream ostream = new FileOutputStream(StubUtils.createLowLevelFile(folder, name, "png"));
TranscoderOutput output = new TranscoderOutput(ostream);
// Perform the transcoding.
t.transcode(input, output);
ostream.flush();
ostream.close();
return dimensions;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy