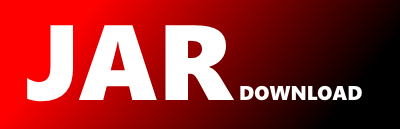
io.activej.fs.tcp.RemoteFsCommands Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of activej-fs Show documentation
Show all versions of activej-fs Show documentation
Provides tools for building efficient, scalable local, remote or clustered file servers.
It utilizes ActiveJ CSP for fast and reliable file transfer.
/*
* Copyright (C) 2020 ActiveJ LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.activej.fs.tcp;
import com.dslplatform.json.CompiledJson;
import com.dslplatform.json.JsonAttribute;
import org.jetbrains.annotations.Nullable;
import java.util.Map;
import java.util.Set;
@SuppressWarnings("WeakerAccess")
public final class RemoteFsCommands {
public abstract static class FsCommand {
}
@CompiledJson
public static final class Upload extends FsCommand {
private final String name;
private final @Nullable Long size;
public Upload(String name, @Nullable Long size) {
this.name = name;
this.size = size;
}
public String getName() {
return name;
}
public @Nullable Long getSize() {
return size;
}
@Override
public String toString() {
return "Upload{name='" + name + "', size=" + size + '}';
}
}
@CompiledJson
public static final class Append extends FsCommand {
private final String name;
private final long offset;
public Append(String name, long offset) {
this.name = name;
this.offset = offset;
}
public String getName() {
return name;
}
public long getOffset() {
return offset;
}
@Override
public String toString() {
return "Append{name='" + name + "', offset=" + offset + '}';
}
}
@CompiledJson
public static final class Download extends FsCommand {
private final String name;
private final long offset;
private final long limit;
public Download(String name, long offset, long limit) {
this.name = name;
this.offset = offset;
this.limit = limit;
}
public String getName() {
return name;
}
public long getOffset() {
return offset;
}
public long getLimit() {
return limit;
}
@Override
public String toString() {
return "Download{name='" + name + "', offset=" + offset + ", limit=" + limit + '}';
}
}
@CompiledJson
public static final class Copy extends FsCommand {
private final String name;
private final String target;
public Copy(String name, String target) {
this.name = name;
this.target = target;
}
public String getName() {
return name;
}
public String getTarget() {
return target;
}
@Override
public String toString() {
return "Copy{name=" + name + ", target=" + target + '}';
}
}
@CompiledJson
public static final class CopyAll extends FsCommand {
private final Map sourceToTarget;
public CopyAll(Map sourceToTarget) {
this.sourceToTarget = sourceToTarget;
}
public Map getSourceToTarget() {
return sourceToTarget;
}
@Override
public String toString() {
return "CopyAll{sourceToTarget=" + sourceToTarget + '}';
}
}
@CompiledJson
public static final class Move extends FsCommand {
private final String name;
private final String target;
public Move(String name, String target) {
this.name = name;
this.target = target;
}
public String getName() {
return name;
}
public String getTarget() {
return target;
}
@Override
public String toString() {
return "Move{name=" + name + ", target=" + target + '}';
}
}
@CompiledJson
public static final class MoveAll extends FsCommand {
private final Map sourceToTarget;
public MoveAll(Map sourceToTarget) {
this.sourceToTarget = sourceToTarget;
}
public Map getSourceToTarget() {
return sourceToTarget;
}
@Override
public String toString() {
return "MoveAll{sourceToTarget=" + sourceToTarget + '}';
}
}
@CompiledJson
public static final class Delete extends FsCommand {
private final String name;
public Delete(String name) {
this.name = name;
}
public String getName() {
return name;
}
@Override
public String toString() {
return "Delete{name='" + name + '}';
}
}
@CompiledJson
public static final class DeleteAll extends FsCommand {
private final Set toDelete;
public DeleteAll(Set filesToDelete) {
this.toDelete = filesToDelete;
}
@JsonAttribute(name = "toDelete")
public Set getFilesToDelete() {
return toDelete;
}
@Override
public String toString() {
return "DeleteAll{toDelete=" + toDelete + '}';
}
}
@CompiledJson
public static final class List extends FsCommand {
private final String glob;
public List(String glob) {
this.glob = glob;
}
public String getGlob() {
return glob;
}
@Override
public String toString() {
return "List{glob='" + glob + "'}";
}
}
@CompiledJson
public static final class Info extends FsCommand {
private final String name;
public Info(String name) {
this.name = name;
}
public String getName() {
return name;
}
@Override
public String toString() {
return "Info{name='" + name + "'}";
}
}
@CompiledJson
public static final class InfoAll extends FsCommand {
private final Set names;
public InfoAll(Set names) {
this.names = names;
}
public Set getNames() {
return names;
}
@Override
public String toString() {
return "InfoAll{names='" + names + "'}";
}
}
public static final class Ping extends FsCommand {
@Override
public String toString() {
return "Ping{}";
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy