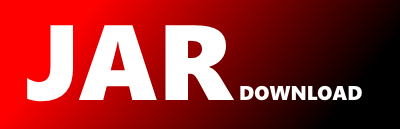
io.activej.ot.TransformResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of activej-ot Show documentation
Show all versions of activej-ot Show documentation
Implementation of operational transformation technology. Allows building collaborative software systems.
/*
* Copyright (C) 2020 ActiveJ LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.activej.ot;
import java.util.List;
import static java.util.Collections.emptyList;
import static java.util.Collections.singletonList;
public class TransformResult {
public enum ConflictResolution {LEFT, RIGHT}
public final ConflictResolution resolution;
public final List left;
public final List right;
private TransformResult(ConflictResolution resolution, List left, List right) {
this.resolution = resolution;
this.left = left;
this.right = right;
}
public static TransformResult conflict(ConflictResolution conflict) {
return new TransformResult<>(conflict, null, null);
}
public static TransformResult empty() {
return new TransformResult<>(null, emptyList(), emptyList());
}
@SuppressWarnings("unchecked")
public static TransformResult of(List extends D> left, List extends D> right) {
return new TransformResult<>(null, (List) left, (List) right);
}
@SuppressWarnings("unchecked")
public static TransformResult of(ConflictResolution conflict, List extends D> left, List extends D> right) {
return new TransformResult<>(conflict, (List) left, (List) right);
}
public static TransformResult of(D left, D right) {
return of(singletonList(left), singletonList(right));
}
public static TransformResult left(D left) {
return of(singletonList(left), emptyList());
}
public static TransformResult left(List extends D> left) {
return of(left, emptyList());
}
public static TransformResult right(D right) {
return of(emptyList(), singletonList(right));
}
public static TransformResult right(List extends D> right) {
return of(emptyList(), right);
}
public boolean hasConflict() {
return resolution != null;
}
@Override
public String toString() {
return "{" +
"left=" + left +
", right=" + right +
'}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy