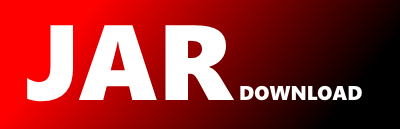
io.activej.ot.reducers.GraphReducer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of activej-ot Show documentation
Show all versions of activej-ot Show documentation
Implementation of operational transformation technology. Allows building collaborative software systems.
/*
* Copyright (C) 2020 ActiveJ LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.activej.ot.reducers;
import io.activej.ot.OTCommit;
import io.activej.promise.Promise;
import org.jetbrains.annotations.NotNull;
import java.util.Collection;
public interface GraphReducer {
default void onStart(@NotNull Collection> queue) {
}
@SuppressWarnings("WeakerAccess")
final class Result {
private final boolean resume;
private final boolean skip;
private final R value;
public Result(boolean resume, boolean skip, R value) {
this.resume = resume;
this.skip = skip;
this.value = value;
}
public static Result resume() {
return new Result<>(true, false, null);
}
public static Result skip() {
return new Result<>(false, true, null);
}
public static Result complete(T value) {
return new Result<>(false, false, value);
}
public static Promise> resumePromise() {
return Promise.of(resume());
}
public static Promise> skipPromise() {
return Promise.of(skip());
}
public static Promise> completePromise(T value) {
return Promise.of(complete(value));
}
public boolean isResume() {
return resume;
}
public boolean isSkip() {
return skip;
}
public boolean isComplete() {
return !resume && !skip;
}
public R get() {
if (isComplete()) {
return value;
}
throw new IllegalStateException();
}
}
@NotNull Promise> onCommit(@NotNull OTCommit commit);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy