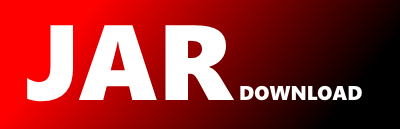
io.activej.ot.uplink._OTUplink$FetchData_DslJsonConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of activej-ot Show documentation
Show all versions of activej-ot Show documentation
Implementation of operational transformation technology. Allows building collaborative software systems.
package io.activej.ot.uplink;
public class _OTUplink$FetchData_DslJsonConverter implements com.dslplatform.json.Configuration {
private static final java.nio.charset.Charset utf8 = java.nio.charset.Charset.forName("UTF-8");
@Override
public void configure(com.dslplatform.json.DslJson __dsljson) {
ConverterFactory factory = new ConverterFactory();
__dsljson.registerReaderFactory(factory);
__dsljson.registerWriterFactory(factory);
}
private final static class ConverterFactory implements com.dslplatform.json.DslJson.ConverterFactory {
@Override
public ObjectFormatConverter tryCreate(java.lang.reflect.Type manifest, com.dslplatform.json.DslJson __dsljson) {
if (manifest instanceof java.lang.reflect.ParameterizedType) {
java.lang.reflect.ParameterizedType pt = (java.lang.reflect.ParameterizedType) manifest;
java.lang.Class> rawClass = (java.lang.Class>) pt.getRawType();
if (rawClass.isAssignableFrom(io.activej.ot.uplink.OTUplink.FetchData.class)) {
return new ObjectFormatConverter(__dsljson, pt.getActualTypeArguments());
}
} else if (io.activej.ot.uplink.OTUplink.FetchData.class.equals(manifest)) {
java.lang.reflect.Type[] unknownArgs = new java.lang.reflect.Type[2];
java.util.Arrays.fill(unknownArgs, Object.class);
if (__dsljson.tryFindReader(Object.class) != null && __dsljson.tryFindWriter(Object.class) != null) {
return new ObjectFormatConverter(__dsljson, unknownArgs);
}
}
return null;
}
}
public final static class ObjectFormatConverter implements com.dslplatform.json.runtime.FormatConverter> {
private final boolean alwaysSerialize;
private final com.dslplatform.json.DslJson __dsljson;
private final java.lang.reflect.Type[] actualTypes;
private final com.dslplatform.json.JsonReader.ReadObject reader_commitId;
private final com.dslplatform.json.JsonWriter.WriteObject writer_commitId;
private final com.dslplatform.json.JsonReader.ReadObject> reader_diffs;
private final com.dslplatform.json.JsonWriter.WriteObject> writer_diffs;
public ObjectFormatConverter(com.dslplatform.json.DslJson __dsljson, java.lang.reflect.Type[] actualTypes) {
this.alwaysSerialize = !__dsljson.omitDefaults;
this.__dsljson = __dsljson;
this.actualTypes = actualTypes;
java.lang.reflect.Type manifest_commitId = actualTypes[0];
this.reader_commitId = __dsljson.tryFindReader(manifest_commitId);
if (reader_commitId == null) {
throw new com.dslplatform.json.ConfigurationException("Unable to find reader for " + manifest_commitId + ". Enable runtime conversion by initializing DslJson with new DslJson<>(Settings.withRuntime().includeServiceLoader())");
}
this.writer_commitId = __dsljson.tryFindWriter(manifest_commitId);
if (writer_commitId == null) {
throw new com.dslplatform.json.ConfigurationException("Unable to find writer for " + manifest_commitId + ". Enable runtime conversion by initializing DslJson with new DslJson<>(Settings.withRuntime().includeServiceLoader())");
}
java.lang.reflect.Type manifest_diffs = com.dslplatform.json.runtime.Generics.makeParameterizedType(java.util.List.class, actualTypes[1]);
this.reader_diffs = __dsljson.tryFindReader(manifest_diffs);
if (reader_diffs == null) {
throw new com.dslplatform.json.ConfigurationException("Unable to find reader for " + manifest_diffs + ". Enable runtime conversion by initializing DslJson with new DslJson<>(Settings.withRuntime().includeServiceLoader())");
}
this.writer_diffs = __dsljson.tryFindWriter(manifest_diffs);
if (writer_diffs == null) {
throw new com.dslplatform.json.ConfigurationException("Unable to find writer for " + manifest_diffs + ". Enable runtime conversion by initializing DslJson with new DslJson<>(Settings.withRuntime().includeServiceLoader())");
}
}
private static final byte[] quoted_commitId = "\"id\":".getBytes(utf8);
private static final byte[] name_commitId = "id".getBytes(utf8);
private static final byte[] quoted_level = ",\"level\":".getBytes(utf8);
private static final byte[] name_level = "level".getBytes(utf8);
private static final byte[] quoted_diffs = ",\"diffs\":".getBytes(utf8);
private static final byte[] name_diffs = "diffs".getBytes(utf8);
public final void write(final com.dslplatform.json.JsonWriter writer, final io.activej.ot.uplink.OTUplink.FetchData instance) {
if (instance == null) writer.writeNull();
else {
writer.writeByte((byte)'{');
if (alwaysSerialize) { writeContentFull(writer, instance); writer.writeByte((byte)'}'); }
else if (writeContentMinimal(writer, instance)) writer.getByteBuffer()[writer.size() - 1] = '}';
else writer.writeByte((byte)'}');
}
}
public void writeContentFull(final com.dslplatform.json.JsonWriter writer, final io.activej.ot.uplink.OTUplink.FetchData instance) {
writer.writeAscii(quoted_commitId);
if (instance.getCommitId() == null) writer.writeNull();
else writer_commitId.write(writer, instance.getCommitId());
writer.writeAscii(quoted_level);
com.dslplatform.json.NumberConverter.serialize(instance.getLevel(), writer);
writer.writeAscii(quoted_diffs);
if (instance.getDiffs() == null) writer.writeNull();
else writer_diffs.write(writer, instance.getDiffs());
}
public boolean writeContentMinimal(final com.dslplatform.json.JsonWriter writer, final io.activej.ot.uplink.OTUplink.FetchData instance) {
boolean hasWritten = false;
if (instance.getCommitId() != null) {
writer.writeByte((byte)'"'); writer.writeAscii(name_commitId); writer.writeByte((byte)'"'); writer.writeByte((byte)':');
writer_commitId.write(writer, instance.getCommitId());
writer.writeByte((byte)','); hasWritten = true;
}
if (instance.getLevel() != 0L) {
writer.writeByte((byte)'"'); writer.writeAscii(name_level); writer.writeByte((byte)'"'); writer.writeByte((byte)':');
com.dslplatform.json.NumberConverter.serialize(instance.getLevel(), writer);
writer.writeByte((byte)','); hasWritten = true;
}
if (instance.getDiffs() != null) {
writer.writeByte((byte)'"'); writer.writeAscii(name_diffs); writer.writeByte((byte)'"'); writer.writeByte((byte)':');
writer_diffs.write(writer, instance.getDiffs());
writer.writeByte((byte)','); hasWritten = true;
}
return hasWritten;
}
public io.activej.ot.uplink.OTUplink.FetchData read(final com.dslplatform.json.JsonReader reader) throws java.io.IOException {
if (reader.wasNull()) return null;
else if (reader.last() != '{') throw reader.newParseError("Expecting '{' for object start");
reader.getNextToken();
return readContent(reader);
}
public io.activej.ot.uplink.OTUplink.FetchData readContent(final com.dslplatform.json.JsonReader reader) throws java.io.IOException {
K _commitId_ = null;
long _level_ = 0L;
java.util.List _diffs_ = null;
if (reader.last() == '}') {
return new io.activej.ot.uplink.OTUplink.FetchData(_commitId_, _level_, _diffs_);
}
switch(reader.fillName()) {
case -1684412451:
reader.getNextToken();
_level_ = com.dslplatform.json.NumberConverter.deserializeLong(reader);
reader.getNextToken();
break;
case 926444256:
reader.getNextToken();
_commitId_ = reader_commitId.read(reader);
reader.getNextToken();
break;
case 658101387:
reader.getNextToken();
_diffs_ = reader_diffs.read(reader);
reader.getNextToken();
break;
default:
reader.getNextToken();
reader.skip();
}
while (reader.last() == ','){
reader.getNextToken();
switch(reader.fillName()) {
case -1684412451:
reader.getNextToken();
_level_ = com.dslplatform.json.NumberConverter.deserializeLong(reader);
reader.getNextToken();
break;
case 926444256:
reader.getNextToken();
_commitId_ = reader_commitId.read(reader);
reader.getNextToken();
break;
case 658101387:
reader.getNextToken();
_diffs_ = reader_diffs.read(reader);
reader.getNextToken();
break;
default:
reader.getNextToken();
reader.skip();
}
}
if (reader.last() != '}') throw reader.newParseError("Expecting '}' for object end");
return new io.activej.ot.uplink.OTUplink.FetchData(_commitId_, _level_, _diffs_);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy