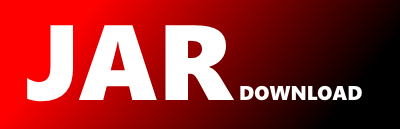
io.activej.async.function.AsyncBiPredicates Maven / Gradle / Ivy
Show all versions of activej-promise Show documentation
/*
* Copyright (C) 2020 ActiveJ LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.activej.async.function;
import io.activej.async.AsyncAccumulator;
import io.activej.async.process.AsyncExecutor;
import io.activej.async.process.AsyncExecutors;
import io.activej.common.ref.RefBoolean;
import org.jetbrains.annotations.Contract;
import java.util.Collection;
import java.util.List;
import java.util.function.Predicate;
public final class AsyncBiPredicates {
@Contract(pure = true)
public static AsyncBiPredicate buffer(AsyncBiPredicate actual) {
return buffer(1, Integer.MAX_VALUE, actual);
}
@Contract(pure = true)
public static AsyncBiPredicate buffer(int maxParallelCalls, int maxBufferedCalls, AsyncBiPredicate predicate) {
return ofExecutor(AsyncExecutors.buffered(maxParallelCalls, maxBufferedCalls), predicate);
}
@Contract(pure = true)
public static AsyncBiPredicate ofExecutor(AsyncExecutor executor, AsyncBiPredicate predicate) {
return (t, u) -> executor.execute(() -> predicate.test(t, u));
}
/**
* Returns a composed {@link AsyncBiPredicate} that represents a logical AND of all predicates
* in a given collection
*
* Unlike Java's {@link Predicate#and(Predicate)} this method does not provide short-circuit.
* If either predicate returns an exceptionally completed promise,
* the combined asynchronous predicate also returns an exceptionally completed promise
*
* @param predicates a collection of {@link AsyncBiPredicate}s that will be logically ANDed
* @return a composed {@link AsyncBiPredicate} that represents a logical AND of asynchronous predicates
* in a given collection
*/
public static AsyncBiPredicate and(Collection> predicates) {
return (t, u) -> {
AsyncAccumulator accumulator = AsyncAccumulator.create(new RefBoolean(true));
for (AsyncBiPredicate super T, ? super U> predicate : predicates) {
accumulator.addPromise(predicate.test(t, u), (ref, result) -> ref.set(ref.get() && result));
}
return accumulator.run().map(RefBoolean::get);
};
}
/**
* Returns an {@link AsyncBiPredicate} that always returns promise of {@code true}
*
* @see #and(Collection)
*/
public static AsyncBiPredicate and() {
return AsyncBiPredicate.alwaysTrue();
}
/**
* Returns a given asynchronous predicate
*
* A shortcut for {@link #and(AsyncBiPredicate[])}
*
* @see #and(Collection)
* @see #and(AsyncBiPredicate[])
*/
public static AsyncBiPredicate and(AsyncBiPredicate super T, ? super U> predicate1) {
//noinspection unchecked
return (AsyncBiPredicate) predicate1;
}
/**
* An optimization for {@link #and(AsyncBiPredicate[])}
*
* @see #and(Collection)
* @see #and(AsyncBiPredicate[])
*/
public static AsyncBiPredicate and(AsyncBiPredicate super T, ? super U> predicate1, AsyncBiPredicate super T, ? super U> predicate2) {
//noinspection unchecked
return ((AsyncBiPredicate) predicate1).and(predicate2);
}
/**
* Returns a composed {@link AsyncBiPredicate} that represents a logical AND of all predicates
* in a given array
*
* @see #and(Collection)
*/
@SafeVarargs
public static AsyncBiPredicate and(AsyncBiPredicate super T, ? super U>... predicates) {
//noinspection RedundantCast
return and(((List>) List.of(predicates)));
}
/**
* Returns a composed {@link AsyncBiPredicate} that represents a logical OR of all predicates
* in a given collection
*
* Unlike Java's {@link Predicate#or(Predicate)} this method does not provide short-circuit.
* If either predicate returns an exceptionally completed promise,
* the combined asynchronous predicate also returns an exceptionally completed promise
*
* @param predicates a collection of {@link AsyncBiPredicate}s that will be logically ORed
* @return a composed {@link AsyncBiPredicate} that represents a logical OR of asynchronous predicates
* in a given collection
*/
public static AsyncBiPredicate or(Collection> predicates) {
return (t, u) -> {
AsyncAccumulator accumulator = AsyncAccumulator.create(new RefBoolean(false));
for (AsyncBiPredicate super T, ? super U> predicate : predicates) {
accumulator.addPromise(predicate.test(t, u), (ref, result) -> ref.set(ref.get() || result));
}
return accumulator.run().map(RefBoolean::get);
};
}
/**
* Returns an {@link AsyncBiPredicate} that always returns promise of {@code false}
*
* @see #or(Collection)
*/
public static AsyncBiPredicate or() {
return AsyncBiPredicate.alwaysFalse();
}
/**
* Returns a given asynchronous predicate
*
* A shortcut for {@link #or(AsyncBiPredicate[])}
*
* @see #or(Collection)
* @see #or(AsyncBiPredicate[])
*/
public static AsyncBiPredicate or(AsyncBiPredicate super T, ? super U> predicate1) {
//noinspection unchecked
return (AsyncBiPredicate) predicate1;
}
/**
* An optimization for {@link #or(AsyncBiPredicate[])}
*
* @see #or(Collection)
* @see #or(AsyncBiPredicate[])
*/
public static AsyncBiPredicate or(AsyncBiPredicate super T, ? super U> predicate1, AsyncBiPredicate super T, ? super U> predicate2) {
//noinspection unchecked
return ((AsyncBiPredicate) predicate1).or(predicate2);
}
/**
* Returns a composed {@link AsyncBiPredicate} that represents a logical OR of all predicates
* in a given array
*
* @see #or(Collection)
*/
@SafeVarargs
public static AsyncBiPredicate or(AsyncBiPredicate super T, ? super U>... predicates) {
//noinspection RedundantCast
return or(((List>) List.of(predicates)));
}
}