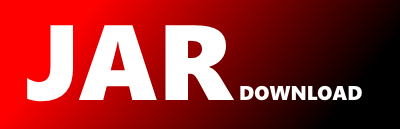
io.activej.promise.CompleteExceptionallyPromise Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of activej-promise Show documentation
Show all versions of activej-promise Show documentation
A convenient way to organize asynchronous code.
Promises are a faster and more efficient version of JavaScript's Promise and Java's CompletionStage's.
/*
* Copyright (C) 2020 ActiveJ LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.activej.promise;
import io.activej.async.callback.Callback;
import io.activej.async.function.*;
import io.activej.common.collection.Try;
import io.activej.common.function.*;
import io.activej.common.recycle.Recyclers;
import org.jetbrains.annotations.Nullable;
import java.util.concurrent.CompletableFuture;
import static io.activej.common.exception.FatalErrorHandler.handleError;
import static io.activej.reactor.Reactor.getCurrentReactor;
/**
* Represents a {@code Promise} which is completed with an exception.
*/
@SuppressWarnings("unchecked")
public final class CompleteExceptionallyPromise implements Promise {
private final Exception exception;
public CompleteExceptionallyPromise(Exception e) {
this.exception = e;
}
@Override
public boolean isComplete() {
return true;
}
@Override
public boolean isResult() {
return false;
}
@Override
public boolean isException() {
return true;
}
@Override
public T getResult() {
return null;
}
@Override
public Exception getException() {
return exception;
}
@Override
public Try getTry() {
return Try.ofException(exception);
}
@SuppressWarnings("unchecked")
@Override
public Promise map(FunctionEx super T, ? extends U> fn) {
return (Promise) this;
}
@Override
public Promise map(BiFunctionEx super T, Exception, ? extends U> fn) {
try {
return Promise.of(fn.apply(null, exception));
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise map(FunctionEx super T, ? extends U> fn, FunctionEx exceptionFn) {
try {
return Promise.of(exceptionFn.apply(exception));
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise mapException(FunctionEx exceptionFn) {
try {
return Promise.ofException(exceptionFn.apply(exception));
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise mapException(
Class clazz, FunctionEx super E, ? extends Exception> exceptionFn
) {
try {
return clazz.isAssignableFrom(exception.getClass()) ? Promise.ofException(exceptionFn.apply((E) exception)) : this;
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise then(AsyncFunctionEx super T, U> fn) {
return (Promise) this;
}
@Override
public Promise thenCallback(CallbackFunctionEx super T, U> fn) {
return (Promise) this;
}
@Override
public Promise then(AsyncSupplierEx fn) {
return (Promise) this;
}
@Override
public Promise thenCallback(CallbackSupplierEx fn) {
return (Promise) this;
}
@Override
public Promise then(AsyncBiFunctionEx super T, Exception, U> fn) {
try {
return fn.apply(null, exception);
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise thenCallback(CallbackBiFunctionEx super T, @Nullable Exception, U> fn) {
try {
return Promise.ofCallback(null, exception, fn);
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise then(AsyncFunctionEx super T, U> fn, AsyncFunctionEx exceptionFn) {
try {
return exceptionFn.apply(exception);
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise thenCallback(CallbackFunctionEx super T, U> fn, CallbackFunctionEx exceptionFn) {
try {
return Promise.ofCallback(null, exception, fn, exceptionFn);
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise whenComplete(BiConsumerEx super T, Exception> fn) {
try {
fn.accept(null, exception);
return this;
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise whenComplete(ConsumerEx super T> fn, ConsumerEx exceptionFn) {
try {
exceptionFn.accept(exception);
return this;
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise whenComplete(RunnableEx action) {
try {
action.run();
return this;
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise whenResult(ConsumerEx super T> fn) {
return this;
}
@Override
public Promise whenResult(RunnableEx action) {
return this;
}
@Override
public Promise whenException(ConsumerEx fn) {
try {
fn.accept(exception);
return this;
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise whenException(Class clazz, ConsumerEx super E> fn) {
try {
if (clazz.isAssignableFrom(exception.getClass())) {
fn.accept((E) exception);
}
return this;
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise whenException(RunnableEx action) {
try {
action.run();
return this;
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise whenException(Class extends Exception> clazz, RunnableEx action) {
try {
if (clazz.isAssignableFrom(exception.getClass())) {
action.run();
}
return this;
} catch (Exception ex) {
handleError(ex, this);
return Promise.ofException(ex);
}
}
@Override
public Promise combine(Promise extends U> other, BiFunctionEx super T, ? super U, ? extends V> fn) {
other.whenResult(Recyclers::recycle);
return (Promise) this;
}
@Override
public Promise both(Promise> other) {
other.whenResult(Recyclers::recycle);
return (Promise) this;
}
@Override
public Promise either(Promise extends T> other) {
return (Promise) other;
}
@Override
public Promise async() {
SettablePromise result = new SettablePromise<>();
getCurrentReactor().post(() -> result.setException(exception));
return result;
}
@Override
public Promise> toTry() {
return Promise.of(Try.ofException(exception));
}
@Override
public Promise toVoid() {
return (Promise) this;
}
@Override
public void next(NextPromise super T, ?> cb) {
cb.acceptNext(null, exception);
}
@Override
public Promise subscribe(Callback super T> cb) {
cb.accept(null, exception);
return this;
}
@Override
public CompletableFuture toCompletableFuture() {
CompletableFuture future = new CompletableFuture<>();
future.completeExceptionally(exception);
return future;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy