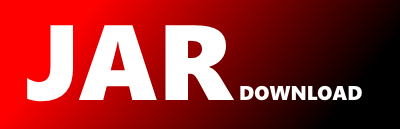
io.activej.serializer.stream.StreamCodecs Maven / Gradle / Ivy
package io.activej.serializer.stream;
import io.activej.common.annotation.StaticFactories;
import io.activej.common.builder.AbstractBuilder;
import io.activej.serializer.BinaryInput;
import io.activej.serializer.BinaryOutput;
import io.activej.serializer.BinarySerializer;
import io.activej.serializer.CorruptedDataException;
import org.jetbrains.annotations.Nullable;
import java.io.IOException;
import java.util.*;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.function.Function;
import java.util.function.IntFunction;
import java.util.function.Supplier;
import static io.activej.common.Checks.checkState;
import static io.activej.serializer.stream.SizedCollectors.*;
import static io.activej.serializer.stream.SizedCollectorsKV.*;
import static io.activej.serializer.util.ZeroArrayUtils.*;
import static java.util.Objects.requireNonNullElseGet;
@SuppressWarnings("unused")
@StaticFactories(StreamCodec.class)
public class StreamCodecs {
public static StreamCodec ofVoid() {
return new StreamCodec<>() {
@Override
public void encode(StreamOutput output, Void item) {
}
@Override
public Void decode(StreamInput input) {
return null;
}
};
}
public static StreamCodec ofBoolean() {
return new StreamCodec<>() {
@Override
public Boolean decode(StreamInput input) throws IOException {
return input.readBoolean();
}
@Override
public void encode(StreamOutput output, Boolean item) throws IOException {
output.writeBoolean(item);
}
};
}
public static StreamCodec ofChar() {
return new StreamCodec<>() {
@Override
public Character decode(StreamInput input) throws IOException {
return input.readChar();
}
@Override
public void encode(StreamOutput output, Character item) throws IOException {
output.writeChar(item);
}
};
}
public static StreamCodec ofByte() {
return new StreamCodec<>() {
@Override
public Byte decode(StreamInput input) throws IOException {
return input.readByte();
}
@Override
public void encode(StreamOutput output, Byte item) throws IOException {
output.writeByte(item);
}
};
}
public static StreamCodec ofShort() {
return new StreamCodec<>() {
@Override
public Short decode(StreamInput input) throws IOException {
return input.readShort();
}
@Override
public void encode(StreamOutput output, Short item) throws IOException {
output.writeShort(item);
}
};
}
public static StreamCodec ofInt() {
return new StreamCodec<>() {
@Override
public Integer decode(StreamInput input) throws IOException {
return input.readInt();
}
@Override
public void encode(StreamOutput output, Integer item) throws IOException {
output.writeInt(item);
}
};
}
public static StreamCodec ofVarInt() {
return new StreamCodec<>() {
@Override
public Integer decode(StreamInput input) throws IOException {
return input.readVarInt();
}
@Override
public void encode(StreamOutput output, Integer item) throws IOException {
output.writeVarInt(item);
}
};
}
public static StreamCodec ofLong() {
return new StreamCodec<>() {
@Override
public Long decode(StreamInput input) throws IOException {
return input.readLong();
}
@Override
public void encode(StreamOutput output, Long item) throws IOException {
output.writeLong(item);
}
};
}
public static StreamCodec ofVarLong() {
return new StreamCodec<>() {
@Override
public Long decode(StreamInput input) throws IOException {
return input.readVarLong();
}
@Override
public void encode(StreamOutput output, Long item) throws IOException {
output.writeVarLong(item);
}
};
}
public static StreamCodec ofFloat() {
return new StreamCodec<>() {
@Override
public Float decode(StreamInput input) throws IOException {
return input.readFloat();
}
@Override
public void encode(StreamOutput output, Float item) throws IOException {
output.writeFloat(item);
}
};
}
public static StreamCodec ofDouble() {
return new StreamCodec<>() {
@Override
public Double decode(StreamInput input) throws IOException {
return input.readDouble();
}
@Override
public void encode(StreamOutput output, Double item) throws IOException {
output.writeDouble(item);
}
};
}
public static StreamCodec ofString() {
return new StreamCodec<>() {
@Override
public String decode(StreamInput input) throws IOException {
return input.readString();
}
@Override
public void encode(StreamOutput output, String item) throws IOException {
output.writeString(item);
}
};
}
public static StreamCodec ofBooleanArray() {
return new AbstractArbitraryLengthArrayStreamCodec<>(ZERO_ARRAY_BOOLEANS, 1) {
@Override
protected int getArrayLength(boolean[] array) {
return array.length;
}
@Override
protected void doWrite(BinaryOutput output, boolean[] array, int offset, int limit) {
for (; offset < limit; offset++) {
output.writeBoolean(array[offset]);
}
}
@Override
protected boolean[] createArray(int length) {
return new boolean[length];
}
@Override
protected void doRead(BinaryInput input, boolean[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[i + offset] = input.readBoolean();
}
}
@Override
protected void doReadRemaining(StreamInput input, boolean[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readBoolean();
}
}
};
}
public static StreamCodec ofCharArray() {
return new AbstractArbitraryLengthArrayStreamCodec<>(ZERO_ARRAY_CHARS, 2) {
@Override
protected int getArrayLength(char[] array) {
return array.length;
}
@Override
protected void doWrite(BinaryOutput output, char[] array, int offset, int limit) {
for (; offset < limit; offset++) {
output.writeChar(array[offset]);
}
}
@Override
protected char[] createArray(int length) {
return new char[length];
}
@Override
protected void doRead(BinaryInput input, char[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[i + offset] = input.readChar();
}
}
@Override
protected void doReadRemaining(StreamInput input, char[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readChar();
}
}
};
}
public static StreamCodec ofByteArray() {
return new StreamCodec<>() {
@Override
public void encode(StreamOutput output, byte[] item) throws IOException {
output.writeVarInt(item.length);
output.write(item);
}
@Override
public byte[] decode(StreamInput input) throws IOException {
int size = input.readVarInt();
if (size == 0) return ZERO_ARRAY_BYTES;
byte[] array = new byte[size];
input.read(array);
return array;
}
};
}
public static StreamCodec ofShortArray() {
return new AbstractArbitraryLengthArrayStreamCodec<>(ZERO_ARRAY_SHORTS, 2) {
@Override
protected int getArrayLength(short[] array) {
return array.length;
}
@Override
protected void doWrite(BinaryOutput output, short[] array, int offset, int limit) {
for (; offset < limit; offset++) {
output.writeShort(array[offset]);
}
}
@Override
protected short[] createArray(int length) {
return new short[length];
}
@Override
protected void doRead(BinaryInput input, short[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[i + offset] = input.readShort();
}
}
@Override
protected void doReadRemaining(StreamInput input, short[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readShort();
}
}
};
}
public static StreamCodec ofIntArray() {
return new AbstractArbitraryLengthArrayStreamCodec<>(ZERO_ARRAY_INTS, 4) {
@Override
protected int getArrayLength(int[] array) {
return array.length;
}
@Override
protected void doWrite(BinaryOutput output, int[] array, int offset, int limit) {
for (; offset < limit; offset++) {
output.writeInt(array[offset]);
}
}
@Override
protected int[] createArray(int length) {
return new int[length];
}
@Override
protected void doRead(BinaryInput input, int[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[i + offset] = input.readInt();
}
}
@Override
protected void doReadRemaining(StreamInput input, int[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readInt();
}
}
};
}
public static StreamCodec ofLongArray() {
return new AbstractArbitraryLengthArrayStreamCodec<>(ZERO_ARRAY_LONGS, 8) {
@Override
protected int getArrayLength(long[] array) {
return array.length;
}
@Override
protected void doWrite(BinaryOutput output, long[] array, int offset, int limit) {
for (; offset < limit; offset++) {
output.writeLong(array[offset]);
}
}
@Override
protected long[] createArray(int length) {
return new long[length];
}
@Override
protected void doRead(BinaryInput input, long[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[i + offset] = input.readLong();
}
}
@Override
protected void doReadRemaining(StreamInput input, long[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readLong();
}
}
};
}
public static StreamCodec ofFloatArray() {
return new AbstractArbitraryLengthArrayStreamCodec<>(ZERO_ARRAY_FLOATS, 4) {
@Override
protected int getArrayLength(float[] array) {
return array.length;
}
@Override
protected void doWrite(BinaryOutput output, float[] array, int offset, int limit) {
for (; offset < limit; offset++) {
output.writeFloat(array[offset]);
}
}
@Override
protected float[] createArray(int length) {
return new float[length];
}
@Override
protected void doRead(BinaryInput input, float[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[i + offset] = input.readFloat();
}
}
@Override
protected void doReadRemaining(StreamInput input, float[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readFloat();
}
}
};
}
public static StreamCodec ofDoubleArray() {
return new AbstractArbitraryLengthArrayStreamCodec<>(ZERO_ARRAY_DOUBLES, 8) {
@Override
protected int getArrayLength(double[] array) {
return array.length;
}
@Override
protected void doWrite(BinaryOutput output, double[] array, int offset, int limit) {
for (; offset < limit; offset++) {
output.writeDouble(array[offset]);
}
}
@Override
protected double[] createArray(int length) {
return new double[length];
}
@Override
protected void doRead(BinaryInput input, double[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[i + offset] = input.readDouble();
}
}
@Override
protected void doReadRemaining(StreamInput input, double[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readDouble();
}
}
};
}
public static StreamCodec ofVarIntArray() {
return new AbstractArbitraryLengthArrayStreamCodec<>(ZERO_ARRAY_INTS, 1, 5) {
@Override
protected int getArrayLength(int[] array) {
return array.length;
}
@Override
protected void doWrite(BinaryOutput output, int[] array, int offset, int limit) {
for (int i = offset; i < limit; i++) {
output.writeVarInt(array[i]);
}
}
@Override
protected int[] createArray(int length) {
return new int[length];
}
@Override
protected void doRead(BinaryInput input, int[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[offset++] = input.readVarInt();
}
}
@Override
protected void doReadRemaining(StreamInput input, int[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readVarInt();
}
}
};
}
public static StreamCodec ofVarLongArray() {
return new AbstractArbitraryLengthArrayStreamCodec<>(ZERO_ARRAY_LONGS, 1, 10) {
@Override
protected int getArrayLength(long[] array) {
return array.length;
}
@Override
protected void doWrite(BinaryOutput output, long[] array, int offset, int limit) {
for (int i = offset; i < limit; i++) {
output.writeVarLong(array[i]);
}
}
@Override
protected long[] createArray(int length) {
return new long[length];
}
@Override
protected void doRead(BinaryInput input, long[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[offset++] = input.readVarLong();
}
}
@Override
protected void doReadRemaining(StreamInput input, long[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readVarLong();
}
}
};
}
public static StreamCodec ofFixedLengthBooleanArray(int length) {
if (length == 0) return StreamCodec.of(($1, $2) -> {}, $ -> ZERO_ARRAY_BOOLEANS);
return new AbstractFixedLengthArrayStreamCodec<>(1, length) {
@Override
protected void doWrite(BinaryOutput output, boolean[] array, int offset, int limit) {
for (; offset < limit; offset++) {
output.writeBoolean(array[offset]);
}
}
@Override
protected boolean[] createArray(int length) {
return new boolean[length];
}
@Override
protected void doRead(BinaryInput input, boolean[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[i + offset] = input.readBoolean();
}
}
@Override
protected void doReadRemaining(StreamInput input, boolean[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readBoolean();
}
}
};
}
public static StreamCodec ofFixedLengthCharArray(int length) {
if (length == 0) return StreamCodec.of(($1, $2) -> {}, $ -> ZERO_ARRAY_CHARS);
return new AbstractFixedLengthArrayStreamCodec<>(2, length) {
@Override
protected void doWrite(BinaryOutput output, char[] array, int offset, int limit) {
for (; offset < limit; offset++) {
output.writeChar(array[offset]);
}
}
@Override
protected char[] createArray(int length) {
return new char[length];
}
@Override
protected void doRead(BinaryInput input, char[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[i + offset] = input.readChar();
}
}
@Override
protected void doReadRemaining(StreamInput input, char[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readChar();
}
}
};
}
public static StreamCodec ofFixedLengthByteArray(int length) {
if (length == 0) return StreamCodec.of(($1, $2) -> {}, $ -> ZERO_ARRAY_BYTES);
return new StreamCodec<>() {
@Override
public void encode(StreamOutput output, byte[] array) throws IOException {
output.write(array);
}
@Override
public byte[] decode(StreamInput input) throws IOException {
byte[] array = new byte[length];
input.read(array);
return array;
}
};
}
public static StreamCodec ofFixedLengthShortArray(int length) {
if (length == 0) return StreamCodec.of(($1, $2) -> {}, $ -> ZERO_ARRAY_SHORTS);
return new AbstractFixedLengthArrayStreamCodec<>(2, length) {
@Override
protected void doWrite(BinaryOutput output, short[] array, int offset, int limit) {
for (; offset < limit; offset++) {
output.writeShort(array[offset]);
}
}
@Override
protected short[] createArray(int length) {
return new short[length];
}
@Override
protected void doRead(BinaryInput input, short[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[i + offset] = input.readShort();
}
}
@Override
protected void doReadRemaining(StreamInput input, short[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readShort();
}
}
};
}
public static StreamCodec ofFixedLengthIntArray(int length) {
if (length == 0) return StreamCodec.of(($1, $2) -> {}, $ -> ZERO_ARRAY_INTS);
return new AbstractFixedLengthArrayStreamCodec<>(4, length) {
@Override
protected void doWrite(BinaryOutput output, int[] array, int offset, int limit) {
for (; offset < limit; offset++) {
output.writeInt(array[offset]);
}
}
@Override
protected int[] createArray(int length) {
return new int[length];
}
@Override
protected void doRead(BinaryInput input, int[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[i + offset] = input.readInt();
}
}
@Override
protected void doReadRemaining(StreamInput input, int[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readInt();
}
}
};
}
public static StreamCodec ofFixedLengthLongArray(int length) {
if (length == 0) return StreamCodec.of(($1, $2) -> {}, $ -> ZERO_ARRAY_LONGS);
return new AbstractFixedLengthArrayStreamCodec<>(8, length) {
@Override
protected void doWrite(BinaryOutput output, long[] array, int offset, int limit) {
for (; offset < limit; offset++) {
output.writeLong(array[offset]);
}
}
@Override
protected long[] createArray(int length) {
return new long[length];
}
@Override
protected void doRead(BinaryInput input, long[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[i + offset] = input.readLong();
}
}
@Override
protected void doReadRemaining(StreamInput input, long[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readLong();
}
}
};
}
public static StreamCodec ofFixedLengthFloatArray(int length) {
if (length == 0) return StreamCodec.of(($1, $2) -> {}, $ -> ZERO_ARRAY_FLOATS);
return new AbstractFixedLengthArrayStreamCodec<>(4, length) {
@Override
protected void doWrite(BinaryOutput output, float[] array, int offset, int limit) {
for (; offset < limit; offset++) {
output.writeFloat(array[offset]);
}
}
@Override
protected float[] createArray(int length) {
return new float[length];
}
@Override
protected void doRead(BinaryInput input, float[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[i + offset] = input.readFloat();
}
}
@Override
protected void doReadRemaining(StreamInput input, float[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readFloat();
}
}
};
}
public static StreamCodec ofFixedLengthDoubleArray(int length) {
if (length == 0) return StreamCodec.of(($1, $2) -> {}, $ -> ZERO_ARRAY_DOUBLES);
return new AbstractFixedLengthArrayStreamCodec<>(8, length) {
@Override
protected void doWrite(BinaryOutput output, double[] array, int offset, int limit) {
for (; offset < limit; offset++) {
output.writeDouble(array[offset]);
}
}
@Override
protected double[] createArray(int length) {
return new double[length];
}
@Override
protected void doRead(BinaryInput input, double[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[i + offset] = input.readDouble();
}
}
@Override
protected void doReadRemaining(StreamInput input, double[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readDouble();
}
}
};
}
public static StreamCodec ofFixedLengthVarIntArray(int length) {
if (length == 0) return StreamCodec.of(($1, $2) -> {}, $ -> ZERO_ARRAY_INTS);
return new AbstractFixedLengthArrayStreamCodec<>(1, 5, length) {
@Override
protected void doWrite(BinaryOutput output, int[] array, int offset, int limit) {
for (int i = offset; i < limit; i++) {
output.writeVarInt(array[i]);
}
}
@Override
protected int[] createArray(int length) {
return new int[length];
}
@Override
protected void doRead(BinaryInput input, int[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[offset++] = input.readVarInt();
}
}
@Override
protected void doReadRemaining(StreamInput input, int[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readVarInt();
}
}
};
}
public static StreamCodec ofFixedLengthVarLongArray(int length) {
if (length == 0) return StreamCodec.of(($1, $2) -> {}, $ -> ZERO_ARRAY_LONGS);
return new AbstractFixedLengthArrayStreamCodec<>(1, 10, length) {
@Override
protected void doWrite(BinaryOutput output, long[] array, int offset, int limit) {
for (int i = offset; i < limit; i++) {
output.writeVarLong(array[i]);
}
}
@Override
protected long[] createArray(int length) {
return new long[length];
}
@Override
protected void doRead(BinaryInput input, long[] array, int offset, int count) {
for (int i = 0; i < count; i++) {
array[offset++] = input.readVarLong();
}
}
@Override
protected void doReadRemaining(StreamInput input, long[] array, int offset, int limit) throws IOException {
for (; offset < limit; offset++) {
array[offset] = input.readVarLong();
}
}
};
}
public static StreamCodec ofArray(StreamCodec itemCodec, IntFunction factory) {
return ofArray($ -> itemCodec, factory);
}
public static StreamCodec ofArray(IntFunction extends StreamCodec extends T>> itemCodecFn, IntFunction factory) {
return new StreamCodec<>() {
@Override
public void encode(StreamOutput output, T[] array) throws IOException {
output.writeVarInt(array.length);
for (int i = 0; i < array.length; i++) {
//noinspection unchecked
StreamCodec codec = (StreamCodec) itemCodecFn.apply(i);
codec.encode(output, array[i]);
}
}
@Override
public T[] decode(StreamInput input) throws IOException {
int size = input.readVarInt();
T[] array = factory.apply(size);
for (int i = 0; i < size; i++) {
array[i] = itemCodecFn.apply(i).decode(input);
}
return array;
}
};
}
public static StreamCodec ofFixedLengthArray(StreamCodec itemCodec, Supplier factory, int length) {
return ofFixedLengthArray($ -> itemCodec, factory, length);
}
public static StreamCodec ofFixedLengthArray(IntFunction extends StreamCodec extends T>> itemCodecFn, Supplier factory, int length) {
return new StreamCodec<>() {
@Override
public void encode(StreamOutput output, T[] array) throws IOException {
for (int i = 0; i < length; i++) {
//noinspection unchecked
StreamCodec codec = (StreamCodec) itemCodecFn.apply(i);
codec.encode(output, array[i]);
}
}
@Override
public T[] decode(StreamInput input) throws IOException {
T[] array = factory.get();
for (int i = 0; i < length; i++) {
array[i] = itemCodecFn.apply(i).decode(input);
}
return array;
}
};
}
public static > StreamCodec ofEnum(Class enumType) {
return new StreamCodec<>() {
@Override
public void encode(StreamOutput output, E value) throws IOException {
output.writeVarInt(value.ordinal());
}
@Override
public E decode(StreamInput input) throws IOException {
return enumType.getEnumConstants()[input.readVarInt()];
}
};
}
public static StreamCodec> ofOptional(StreamCodec codec) {
return new StreamCodec<>() {
@Override
public void encode(StreamOutput output, Optional item) throws IOException {
if (item.isEmpty()) {
output.writeByte((byte) 0);
} else {
output.writeByte((byte) 1);
codec.encode(output, item.get());
}
}
@Override
public Optional decode(StreamInput input) throws IOException {
if (input.readByte() == 0) return Optional.empty();
return Optional.of(codec.decode(input));
}
};
}
public static > StreamCodec ofCollection(StreamCodec itemCodec, SizedCollector collector) {
return ofCollection($ -> itemCodec, collector);
}
public static > StreamCodec ofCollection(IntFunction extends StreamCodec extends T>> itemCodecFn, SizedCollector collector) {
return new StreamCodec<>() {
@Override
public void encode(StreamOutput output, C collection) throws IOException {
output.writeVarInt(collection.size());
int i = 0;
for (T item : collection) {
//noinspection unchecked
StreamCodec codec = (StreamCodec) itemCodecFn.apply(i++);
codec.encode(output, item);
}
}
@Override
public C decode(StreamInput input) throws IOException {
int size = input.readVarInt();
if (size == 0) {
return collector.create0();
} else if (size == 1) {
return collector.create1(itemCodecFn.apply(0).decode(input));
} else {
Object acc = collector.accumulator(size);
for (int i = 0; i < size; i++) {
T item = itemCodecFn.apply(i).decode(input);
//noinspection unchecked
((SizedCollector) collector).accumulate(acc, i, item);
}
//noinspection unchecked
return ((SizedCollector) collector).result(acc);
}
}
};
}
public static StreamCodec> ofCollection(StreamCodec itemCodec) {
return ofCollection(itemCodec, toCollection());
}
public static > StreamCodec> ofEnumSet(Class type) {
return ofCollection(StreamCodecs.ofEnum(type), toEnumSet(type));
}
public static StreamCodec> ofList(StreamCodec itemCodec) {
return ofCollection(itemCodec, toList());
}
public static StreamCodec> ofList(IntFunction extends StreamCodec extends T>> itemCodecFn) {
return ofCollection(itemCodecFn, toList());
}
public static StreamCodec> ofArrayList(StreamCodec itemCodec) {
return ofCollection(itemCodec, toArrayList());
}
public static StreamCodec> ofLinkedList(StreamCodec itemCodec) {
return ofCollection(itemCodec, toLinkedList());
}
public static StreamCodec> ofSet(StreamCodec itemCodec) {
return ofCollection(itemCodec, toSet());
}
public static StreamCodec> ofHashSet(StreamCodec itemCodec) {
return ofCollection(itemCodec, toHashSet());
}
public static StreamCodec> ofLinkedHashSet(StreamCodec itemCodec) {
return ofCollection(itemCodec, toLinkedHashSet());
}
public static StreamCodec
© 2015 - 2025 Weber Informatics LLC | Privacy Policy