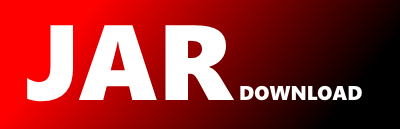
io.adminshell.aas.v3.model.builder.ViewBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of model Show documentation
Show all versions of model Show documentation
This project includes a Java representation of the classes defined in the Asset Administration Shell ontology.
/*
* Copyright (c) 2021 Fraunhofer-Gesellschaft zur Foerderung der angewandten Forschung e. V.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.adminshell.aas.v3.model.builder;
import java.util.List;
import io.adminshell.aas.v3.model.*;
import io.adminshell.aas.v3.model.impl.*;
public abstract class ViewBuilder> extends ExtendableBuilder {
/**
* This function allows setting a value for containedElements
*
* @param containedElements desired value to be set
* @return Builder object with new value for containedElements
*/
public B containedElements(List containedElements) {
getBuildingInstance().setContainedElements(containedElements);
return getSelf();
}
/**
* This function allows adding a value to the List containedElements
*
* @param containedElement desired value to be added
* @return Builder object with new value for containedElements
*/
public B containedElement(Reference containedElement) {
getBuildingInstance().getContainedElements().add(containedElement);
return getSelf();
}
/**
* This function allows setting a value for category
*
* @param category desired value to be set
* @return Builder object with new value for category
*/
public B category(String category) {
getBuildingInstance().setCategory(category);
return getSelf();
}
/**
* This function allows setting a value for descriptions
*
* @param descriptions desired value to be set
* @return Builder object with new value for descriptions
*/
public B descriptions(List descriptions) {
getBuildingInstance().setDescriptions(descriptions);
return getSelf();
}
/**
* This function allows adding a value to the List descriptions
*
* @param description desired value to be added
* @return Builder object with new value for descriptions
*/
public B description(LangString description) {
getBuildingInstance().getDescriptions().add(description);
return getSelf();
}
/**
* This function allows setting a value for displayNames
*
* @param displayNames desired value to be set
* @return Builder object with new value for displayNames
*/
public B displayNames(List displayNames) {
getBuildingInstance().setDisplayNames(displayNames);
return getSelf();
}
/**
* This function allows adding a value to the List displayNames
*
* @param displayName desired value to be added
* @return Builder object with new value for displayNames
*/
public B displayName(LangString displayName) {
getBuildingInstance().getDisplayNames().add(displayName);
return getSelf();
}
/**
* This function allows setting a value for idShort
*
* @param idShort desired value to be set
* @return Builder object with new value for idShort
*/
public B idShort(String idShort) {
getBuildingInstance().setIdShort(idShort);
return getSelf();
}
/**
* This function allows setting a value for embeddedDataSpecifications
*
* @param embeddedDataSpecifications desired value to be set
* @return Builder object with new value for embeddedDataSpecifications
*/
public B embeddedDataSpecifications(List embeddedDataSpecifications) {
getBuildingInstance().setEmbeddedDataSpecifications(embeddedDataSpecifications);
return getSelf();
}
/**
* This function allows adding a value to the List embeddedDataSpecifications
*
* @param embeddedDataSpecification desired value to be added
* @return Builder object with new value for embeddedDataSpecifications
*/
public B embeddedDataSpecification(EmbeddedDataSpecification embeddedDataSpecification) {
getBuildingInstance().getEmbeddedDataSpecifications().add(embeddedDataSpecification);
return getSelf();
}
/**
* This function allows setting a value for semanticId
*
* @param semanticId desired value to be set
* @return Builder object with new value for semanticId
*/
public B semanticId(Reference semanticId) {
getBuildingInstance().setSemanticId(semanticId);
return getSelf();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy