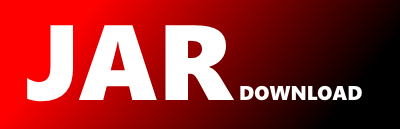
types.Blockchain Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: blockchain.proto
package types;
public final class Blockchain {
private Blockchain() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code types.TxType}
*/
public enum TxType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* NORMAL = 0;
*/
NORMAL(0),
/**
* GOVERNANCE = 1;
*/
GOVERNANCE(1),
/**
* REDEPLOY = 2;
*/
REDEPLOY(2),
/**
* FEEDELEGATION = 3;
*/
FEEDELEGATION(3),
/**
* TRANSFER = 4;
*/
TRANSFER(4),
/**
* CALL = 5;
*/
CALL(5),
/**
* DEPLOY = 6;
*/
DEPLOY(6),
UNRECOGNIZED(-1),
;
/**
* NORMAL = 0;
*/
public static final int NORMAL_VALUE = 0;
/**
* GOVERNANCE = 1;
*/
public static final int GOVERNANCE_VALUE = 1;
/**
* REDEPLOY = 2;
*/
public static final int REDEPLOY_VALUE = 2;
/**
* FEEDELEGATION = 3;
*/
public static final int FEEDELEGATION_VALUE = 3;
/**
* TRANSFER = 4;
*/
public static final int TRANSFER_VALUE = 4;
/**
* CALL = 5;
*/
public static final int CALL_VALUE = 5;
/**
* DEPLOY = 6;
*/
public static final int DEPLOY_VALUE = 6;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static TxType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static TxType forNumber(int value) {
switch (value) {
case 0: return NORMAL;
case 1: return GOVERNANCE;
case 2: return REDEPLOY;
case 3: return FEEDELEGATION;
case 4: return TRANSFER;
case 5: return CALL;
case 6: return DEPLOY;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
TxType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TxType findValueByNumber(int number) {
return TxType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return types.Blockchain.getDescriptor().getEnumTypes().get(0);
}
private static final TxType[] VALUES = values();
public static TxType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private TxType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:types.TxType)
}
public interface BlockOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.Block)
com.google.protobuf.MessageOrBuilder {
/**
* bytes hash = 1;
* @return The hash.
*/
com.google.protobuf.ByteString getHash();
/**
* .types.BlockHeader header = 2;
* @return Whether the header field is set.
*/
boolean hasHeader();
/**
* .types.BlockHeader header = 2;
* @return The header.
*/
types.Blockchain.BlockHeader getHeader();
/**
* .types.BlockHeader header = 2;
*/
types.Blockchain.BlockHeaderOrBuilder getHeaderOrBuilder();
/**
* .types.BlockBody body = 3;
* @return Whether the body field is set.
*/
boolean hasBody();
/**
* .types.BlockBody body = 3;
* @return The body.
*/
types.Blockchain.BlockBody getBody();
/**
* .types.BlockBody body = 3;
*/
types.Blockchain.BlockBodyOrBuilder getBodyOrBuilder();
}
/**
* Protobuf type {@code types.Block}
*/
public static final class Block extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.Block)
BlockOrBuilder {
private static final long serialVersionUID = 0L;
// Use Block.newBuilder() to construct.
private Block(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Block() {
hash_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Block();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Block(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
hash_ = input.readBytes();
break;
}
case 18: {
types.Blockchain.BlockHeader.Builder subBuilder = null;
if (header_ != null) {
subBuilder = header_.toBuilder();
}
header_ = input.readMessage(types.Blockchain.BlockHeader.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(header_);
header_ = subBuilder.buildPartial();
}
break;
}
case 26: {
types.Blockchain.BlockBody.Builder subBuilder = null;
if (body_ != null) {
subBuilder = body_.toBuilder();
}
body_ = input.readMessage(types.Blockchain.BlockBody.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(body_);
body_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_Block_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_Block_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.Block.class, types.Blockchain.Block.Builder.class);
}
public static final int HASH_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString hash_;
/**
* bytes hash = 1;
* @return The hash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getHash() {
return hash_;
}
public static final int HEADER_FIELD_NUMBER = 2;
private types.Blockchain.BlockHeader header_;
/**
* .types.BlockHeader header = 2;
* @return Whether the header field is set.
*/
@java.lang.Override
public boolean hasHeader() {
return header_ != null;
}
/**
* .types.BlockHeader header = 2;
* @return The header.
*/
@java.lang.Override
public types.Blockchain.BlockHeader getHeader() {
return header_ == null ? types.Blockchain.BlockHeader.getDefaultInstance() : header_;
}
/**
* .types.BlockHeader header = 2;
*/
@java.lang.Override
public types.Blockchain.BlockHeaderOrBuilder getHeaderOrBuilder() {
return getHeader();
}
public static final int BODY_FIELD_NUMBER = 3;
private types.Blockchain.BlockBody body_;
/**
* .types.BlockBody body = 3;
* @return Whether the body field is set.
*/
@java.lang.Override
public boolean hasBody() {
return body_ != null;
}
/**
* .types.BlockBody body = 3;
* @return The body.
*/
@java.lang.Override
public types.Blockchain.BlockBody getBody() {
return body_ == null ? types.Blockchain.BlockBody.getDefaultInstance() : body_;
}
/**
* .types.BlockBody body = 3;
*/
@java.lang.Override
public types.Blockchain.BlockBodyOrBuilder getBodyOrBuilder() {
return getBody();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!hash_.isEmpty()) {
output.writeBytes(1, hash_);
}
if (header_ != null) {
output.writeMessage(2, getHeader());
}
if (body_ != null) {
output.writeMessage(3, getBody());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!hash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, hash_);
}
if (header_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getHeader());
}
if (body_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getBody());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.Block)) {
return super.equals(obj);
}
types.Blockchain.Block other = (types.Blockchain.Block) obj;
if (!getHash()
.equals(other.getHash())) return false;
if (hasHeader() != other.hasHeader()) return false;
if (hasHeader()) {
if (!getHeader()
.equals(other.getHeader())) return false;
}
if (hasBody() != other.hasBody()) return false;
if (hasBody()) {
if (!getBody()
.equals(other.getBody())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + HASH_FIELD_NUMBER;
hash = (53 * hash) + getHash().hashCode();
if (hasHeader()) {
hash = (37 * hash) + HEADER_FIELD_NUMBER;
hash = (53 * hash) + getHeader().hashCode();
}
if (hasBody()) {
hash = (37 * hash) + BODY_FIELD_NUMBER;
hash = (53 * hash) + getBody().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.Block parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Block parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Block parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Block parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Block parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Block parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Block parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.Block parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.Block parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.Block parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.Block parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.Block parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.Block prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.Block}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.Block)
types.Blockchain.BlockOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_Block_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_Block_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.Block.class, types.Blockchain.Block.Builder.class);
}
// Construct using types.Blockchain.Block.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
hash_ = com.google.protobuf.ByteString.EMPTY;
if (headerBuilder_ == null) {
header_ = null;
} else {
header_ = null;
headerBuilder_ = null;
}
if (bodyBuilder_ == null) {
body_ = null;
} else {
body_ = null;
bodyBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_Block_descriptor;
}
@java.lang.Override
public types.Blockchain.Block getDefaultInstanceForType() {
return types.Blockchain.Block.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.Block build() {
types.Blockchain.Block result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.Block buildPartial() {
types.Blockchain.Block result = new types.Blockchain.Block(this);
result.hash_ = hash_;
if (headerBuilder_ == null) {
result.header_ = header_;
} else {
result.header_ = headerBuilder_.build();
}
if (bodyBuilder_ == null) {
result.body_ = body_;
} else {
result.body_ = bodyBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.Block) {
return mergeFrom((types.Blockchain.Block)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.Block other) {
if (other == types.Blockchain.Block.getDefaultInstance()) return this;
if (other.getHash() != com.google.protobuf.ByteString.EMPTY) {
setHash(other.getHash());
}
if (other.hasHeader()) {
mergeHeader(other.getHeader());
}
if (other.hasBody()) {
mergeBody(other.getBody());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.Block parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.Block) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString hash_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes hash = 1;
* @return The hash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getHash() {
return hash_;
}
/**
* bytes hash = 1;
* @param value The hash to set.
* @return This builder for chaining.
*/
public Builder setHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
hash_ = value;
onChanged();
return this;
}
/**
* bytes hash = 1;
* @return This builder for chaining.
*/
public Builder clearHash() {
hash_ = getDefaultInstance().getHash();
onChanged();
return this;
}
private types.Blockchain.BlockHeader header_;
private com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.BlockHeader, types.Blockchain.BlockHeader.Builder, types.Blockchain.BlockHeaderOrBuilder> headerBuilder_;
/**
* .types.BlockHeader header = 2;
* @return Whether the header field is set.
*/
public boolean hasHeader() {
return headerBuilder_ != null || header_ != null;
}
/**
* .types.BlockHeader header = 2;
* @return The header.
*/
public types.Blockchain.BlockHeader getHeader() {
if (headerBuilder_ == null) {
return header_ == null ? types.Blockchain.BlockHeader.getDefaultInstance() : header_;
} else {
return headerBuilder_.getMessage();
}
}
/**
* .types.BlockHeader header = 2;
*/
public Builder setHeader(types.Blockchain.BlockHeader value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
header_ = value;
onChanged();
} else {
headerBuilder_.setMessage(value);
}
return this;
}
/**
* .types.BlockHeader header = 2;
*/
public Builder setHeader(
types.Blockchain.BlockHeader.Builder builderForValue) {
if (headerBuilder_ == null) {
header_ = builderForValue.build();
onChanged();
} else {
headerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .types.BlockHeader header = 2;
*/
public Builder mergeHeader(types.Blockchain.BlockHeader value) {
if (headerBuilder_ == null) {
if (header_ != null) {
header_ =
types.Blockchain.BlockHeader.newBuilder(header_).mergeFrom(value).buildPartial();
} else {
header_ = value;
}
onChanged();
} else {
headerBuilder_.mergeFrom(value);
}
return this;
}
/**
* .types.BlockHeader header = 2;
*/
public Builder clearHeader() {
if (headerBuilder_ == null) {
header_ = null;
onChanged();
} else {
header_ = null;
headerBuilder_ = null;
}
return this;
}
/**
* .types.BlockHeader header = 2;
*/
public types.Blockchain.BlockHeader.Builder getHeaderBuilder() {
onChanged();
return getHeaderFieldBuilder().getBuilder();
}
/**
* .types.BlockHeader header = 2;
*/
public types.Blockchain.BlockHeaderOrBuilder getHeaderOrBuilder() {
if (headerBuilder_ != null) {
return headerBuilder_.getMessageOrBuilder();
} else {
return header_ == null ?
types.Blockchain.BlockHeader.getDefaultInstance() : header_;
}
}
/**
* .types.BlockHeader header = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.BlockHeader, types.Blockchain.BlockHeader.Builder, types.Blockchain.BlockHeaderOrBuilder>
getHeaderFieldBuilder() {
if (headerBuilder_ == null) {
headerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.BlockHeader, types.Blockchain.BlockHeader.Builder, types.Blockchain.BlockHeaderOrBuilder>(
getHeader(),
getParentForChildren(),
isClean());
header_ = null;
}
return headerBuilder_;
}
private types.Blockchain.BlockBody body_;
private com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.BlockBody, types.Blockchain.BlockBody.Builder, types.Blockchain.BlockBodyOrBuilder> bodyBuilder_;
/**
* .types.BlockBody body = 3;
* @return Whether the body field is set.
*/
public boolean hasBody() {
return bodyBuilder_ != null || body_ != null;
}
/**
* .types.BlockBody body = 3;
* @return The body.
*/
public types.Blockchain.BlockBody getBody() {
if (bodyBuilder_ == null) {
return body_ == null ? types.Blockchain.BlockBody.getDefaultInstance() : body_;
} else {
return bodyBuilder_.getMessage();
}
}
/**
* .types.BlockBody body = 3;
*/
public Builder setBody(types.Blockchain.BlockBody value) {
if (bodyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
body_ = value;
onChanged();
} else {
bodyBuilder_.setMessage(value);
}
return this;
}
/**
* .types.BlockBody body = 3;
*/
public Builder setBody(
types.Blockchain.BlockBody.Builder builderForValue) {
if (bodyBuilder_ == null) {
body_ = builderForValue.build();
onChanged();
} else {
bodyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .types.BlockBody body = 3;
*/
public Builder mergeBody(types.Blockchain.BlockBody value) {
if (bodyBuilder_ == null) {
if (body_ != null) {
body_ =
types.Blockchain.BlockBody.newBuilder(body_).mergeFrom(value).buildPartial();
} else {
body_ = value;
}
onChanged();
} else {
bodyBuilder_.mergeFrom(value);
}
return this;
}
/**
* .types.BlockBody body = 3;
*/
public Builder clearBody() {
if (bodyBuilder_ == null) {
body_ = null;
onChanged();
} else {
body_ = null;
bodyBuilder_ = null;
}
return this;
}
/**
* .types.BlockBody body = 3;
*/
public types.Blockchain.BlockBody.Builder getBodyBuilder() {
onChanged();
return getBodyFieldBuilder().getBuilder();
}
/**
* .types.BlockBody body = 3;
*/
public types.Blockchain.BlockBodyOrBuilder getBodyOrBuilder() {
if (bodyBuilder_ != null) {
return bodyBuilder_.getMessageOrBuilder();
} else {
return body_ == null ?
types.Blockchain.BlockBody.getDefaultInstance() : body_;
}
}
/**
* .types.BlockBody body = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.BlockBody, types.Blockchain.BlockBody.Builder, types.Blockchain.BlockBodyOrBuilder>
getBodyFieldBuilder() {
if (bodyBuilder_ == null) {
bodyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.BlockBody, types.Blockchain.BlockBody.Builder, types.Blockchain.BlockBodyOrBuilder>(
getBody(),
getParentForChildren(),
isClean());
body_ = null;
}
return bodyBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.Block)
}
// @@protoc_insertion_point(class_scope:types.Block)
private static final types.Blockchain.Block DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.Block();
}
public static types.Blockchain.Block getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Block parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Block(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.Block getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BlockHeaderOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.BlockHeader)
com.google.protobuf.MessageOrBuilder {
/**
*
* chain identifier
*
*
* bytes chainID = 1;
* @return The chainID.
*/
com.google.protobuf.ByteString getChainID();
/**
*
* hash of previous block
*
*
* bytes prevBlockHash = 2;
* @return The prevBlockHash.
*/
com.google.protobuf.ByteString getPrevBlockHash();
/**
*
* block number
*
*
* uint64 blockNo = 3;
* @return The blockNo.
*/
long getBlockNo();
/**
*
* block creation time stamp
*
*
* int64 timestamp = 4;
* @return The timestamp.
*/
long getTimestamp();
/**
*
* hash of root of block merkle tree
*
*
* bytes blocksRootHash = 5;
* @return The blocksRootHash.
*/
com.google.protobuf.ByteString getBlocksRootHash();
/**
*
* hash of root of transaction merkle tree
*
*
* bytes txsRootHash = 6;
* @return The txsRootHash.
*/
com.google.protobuf.ByteString getTxsRootHash();
/**
*
* hash of root of receipt merkle tree
*
*
* bytes receiptsRootHash = 7;
* @return The receiptsRootHash.
*/
com.google.protobuf.ByteString getReceiptsRootHash();
/**
*
* number of blocks this block is able to confirm
*
*
* uint64 confirms = 8;
* @return The confirms.
*/
long getConfirms();
/**
*
* block producer's public key
*
*
* bytes pubKey = 9;
* @return The pubKey.
*/
com.google.protobuf.ByteString getPubKey();
/**
*
* address of account to receive fees
*
*
* bytes coinbaseAccount = 10;
* @return The coinbaseAccount.
*/
com.google.protobuf.ByteString getCoinbaseAccount();
/**
*
* block producer's signature of BlockHeader
*
*
* bytes sign = 11;
* @return The sign.
*/
com.google.protobuf.ByteString getSign();
/**
*
* consensus meta
*
*
* bytes consensus = 12;
* @return The consensus.
*/
com.google.protobuf.ByteString getConsensus();
}
/**
* Protobuf type {@code types.BlockHeader}
*/
public static final class BlockHeader extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.BlockHeader)
BlockHeaderOrBuilder {
private static final long serialVersionUID = 0L;
// Use BlockHeader.newBuilder() to construct.
private BlockHeader(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BlockHeader() {
chainID_ = com.google.protobuf.ByteString.EMPTY;
prevBlockHash_ = com.google.protobuf.ByteString.EMPTY;
blocksRootHash_ = com.google.protobuf.ByteString.EMPTY;
txsRootHash_ = com.google.protobuf.ByteString.EMPTY;
receiptsRootHash_ = com.google.protobuf.ByteString.EMPTY;
pubKey_ = com.google.protobuf.ByteString.EMPTY;
coinbaseAccount_ = com.google.protobuf.ByteString.EMPTY;
sign_ = com.google.protobuf.ByteString.EMPTY;
consensus_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new BlockHeader();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BlockHeader(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
chainID_ = input.readBytes();
break;
}
case 18: {
prevBlockHash_ = input.readBytes();
break;
}
case 24: {
blockNo_ = input.readUInt64();
break;
}
case 32: {
timestamp_ = input.readInt64();
break;
}
case 42: {
blocksRootHash_ = input.readBytes();
break;
}
case 50: {
txsRootHash_ = input.readBytes();
break;
}
case 58: {
receiptsRootHash_ = input.readBytes();
break;
}
case 64: {
confirms_ = input.readUInt64();
break;
}
case 74: {
pubKey_ = input.readBytes();
break;
}
case 82: {
coinbaseAccount_ = input.readBytes();
break;
}
case 90: {
sign_ = input.readBytes();
break;
}
case 98: {
consensus_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_BlockHeader_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_BlockHeader_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.BlockHeader.class, types.Blockchain.BlockHeader.Builder.class);
}
public static final int CHAINID_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString chainID_;
/**
*
* chain identifier
*
*
* bytes chainID = 1;
* @return The chainID.
*/
@java.lang.Override
public com.google.protobuf.ByteString getChainID() {
return chainID_;
}
public static final int PREVBLOCKHASH_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString prevBlockHash_;
/**
*
* hash of previous block
*
*
* bytes prevBlockHash = 2;
* @return The prevBlockHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPrevBlockHash() {
return prevBlockHash_;
}
public static final int BLOCKNO_FIELD_NUMBER = 3;
private long blockNo_;
/**
*
* block number
*
*
* uint64 blockNo = 3;
* @return The blockNo.
*/
@java.lang.Override
public long getBlockNo() {
return blockNo_;
}
public static final int TIMESTAMP_FIELD_NUMBER = 4;
private long timestamp_;
/**
*
* block creation time stamp
*
*
* int64 timestamp = 4;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
return timestamp_;
}
public static final int BLOCKSROOTHASH_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString blocksRootHash_;
/**
*
* hash of root of block merkle tree
*
*
* bytes blocksRootHash = 5;
* @return The blocksRootHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBlocksRootHash() {
return blocksRootHash_;
}
public static final int TXSROOTHASH_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString txsRootHash_;
/**
*
* hash of root of transaction merkle tree
*
*
* bytes txsRootHash = 6;
* @return The txsRootHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTxsRootHash() {
return txsRootHash_;
}
public static final int RECEIPTSROOTHASH_FIELD_NUMBER = 7;
private com.google.protobuf.ByteString receiptsRootHash_;
/**
*
* hash of root of receipt merkle tree
*
*
* bytes receiptsRootHash = 7;
* @return The receiptsRootHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getReceiptsRootHash() {
return receiptsRootHash_;
}
public static final int CONFIRMS_FIELD_NUMBER = 8;
private long confirms_;
/**
*
* number of blocks this block is able to confirm
*
*
* uint64 confirms = 8;
* @return The confirms.
*/
@java.lang.Override
public long getConfirms() {
return confirms_;
}
public static final int PUBKEY_FIELD_NUMBER = 9;
private com.google.protobuf.ByteString pubKey_;
/**
*
* block producer's public key
*
*
* bytes pubKey = 9;
* @return The pubKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPubKey() {
return pubKey_;
}
public static final int COINBASEACCOUNT_FIELD_NUMBER = 10;
private com.google.protobuf.ByteString coinbaseAccount_;
/**
*
* address of account to receive fees
*
*
* bytes coinbaseAccount = 10;
* @return The coinbaseAccount.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCoinbaseAccount() {
return coinbaseAccount_;
}
public static final int SIGN_FIELD_NUMBER = 11;
private com.google.protobuf.ByteString sign_;
/**
*
* block producer's signature of BlockHeader
*
*
* bytes sign = 11;
* @return The sign.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSign() {
return sign_;
}
public static final int CONSENSUS_FIELD_NUMBER = 12;
private com.google.protobuf.ByteString consensus_;
/**
*
* consensus meta
*
*
* bytes consensus = 12;
* @return The consensus.
*/
@java.lang.Override
public com.google.protobuf.ByteString getConsensus() {
return consensus_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!chainID_.isEmpty()) {
output.writeBytes(1, chainID_);
}
if (!prevBlockHash_.isEmpty()) {
output.writeBytes(2, prevBlockHash_);
}
if (blockNo_ != 0L) {
output.writeUInt64(3, blockNo_);
}
if (timestamp_ != 0L) {
output.writeInt64(4, timestamp_);
}
if (!blocksRootHash_.isEmpty()) {
output.writeBytes(5, blocksRootHash_);
}
if (!txsRootHash_.isEmpty()) {
output.writeBytes(6, txsRootHash_);
}
if (!receiptsRootHash_.isEmpty()) {
output.writeBytes(7, receiptsRootHash_);
}
if (confirms_ != 0L) {
output.writeUInt64(8, confirms_);
}
if (!pubKey_.isEmpty()) {
output.writeBytes(9, pubKey_);
}
if (!coinbaseAccount_.isEmpty()) {
output.writeBytes(10, coinbaseAccount_);
}
if (!sign_.isEmpty()) {
output.writeBytes(11, sign_);
}
if (!consensus_.isEmpty()) {
output.writeBytes(12, consensus_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!chainID_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, chainID_);
}
if (!prevBlockHash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, prevBlockHash_);
}
if (blockNo_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, blockNo_);
}
if (timestamp_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, timestamp_);
}
if (!blocksRootHash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, blocksRootHash_);
}
if (!txsRootHash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, txsRootHash_);
}
if (!receiptsRootHash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, receiptsRootHash_);
}
if (confirms_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(8, confirms_);
}
if (!pubKey_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(9, pubKey_);
}
if (!coinbaseAccount_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(10, coinbaseAccount_);
}
if (!sign_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(11, sign_);
}
if (!consensus_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(12, consensus_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.BlockHeader)) {
return super.equals(obj);
}
types.Blockchain.BlockHeader other = (types.Blockchain.BlockHeader) obj;
if (!getChainID()
.equals(other.getChainID())) return false;
if (!getPrevBlockHash()
.equals(other.getPrevBlockHash())) return false;
if (getBlockNo()
!= other.getBlockNo()) return false;
if (getTimestamp()
!= other.getTimestamp()) return false;
if (!getBlocksRootHash()
.equals(other.getBlocksRootHash())) return false;
if (!getTxsRootHash()
.equals(other.getTxsRootHash())) return false;
if (!getReceiptsRootHash()
.equals(other.getReceiptsRootHash())) return false;
if (getConfirms()
!= other.getConfirms()) return false;
if (!getPubKey()
.equals(other.getPubKey())) return false;
if (!getCoinbaseAccount()
.equals(other.getCoinbaseAccount())) return false;
if (!getSign()
.equals(other.getSign())) return false;
if (!getConsensus()
.equals(other.getConsensus())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CHAINID_FIELD_NUMBER;
hash = (53 * hash) + getChainID().hashCode();
hash = (37 * hash) + PREVBLOCKHASH_FIELD_NUMBER;
hash = (53 * hash) + getPrevBlockHash().hashCode();
hash = (37 * hash) + BLOCKNO_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBlockNo());
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
hash = (37 * hash) + BLOCKSROOTHASH_FIELD_NUMBER;
hash = (53 * hash) + getBlocksRootHash().hashCode();
hash = (37 * hash) + TXSROOTHASH_FIELD_NUMBER;
hash = (53 * hash) + getTxsRootHash().hashCode();
hash = (37 * hash) + RECEIPTSROOTHASH_FIELD_NUMBER;
hash = (53 * hash) + getReceiptsRootHash().hashCode();
hash = (37 * hash) + CONFIRMS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getConfirms());
hash = (37 * hash) + PUBKEY_FIELD_NUMBER;
hash = (53 * hash) + getPubKey().hashCode();
hash = (37 * hash) + COINBASEACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getCoinbaseAccount().hashCode();
hash = (37 * hash) + SIGN_FIELD_NUMBER;
hash = (53 * hash) + getSign().hashCode();
hash = (37 * hash) + CONSENSUS_FIELD_NUMBER;
hash = (53 * hash) + getConsensus().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.BlockHeader parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.BlockHeader parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.BlockHeader parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.BlockHeader parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.BlockHeader parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.BlockHeader parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.BlockHeader parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.BlockHeader parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.BlockHeader parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.BlockHeader parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.BlockHeader parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.BlockHeader parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.BlockHeader prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.BlockHeader}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.BlockHeader)
types.Blockchain.BlockHeaderOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_BlockHeader_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_BlockHeader_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.BlockHeader.class, types.Blockchain.BlockHeader.Builder.class);
}
// Construct using types.Blockchain.BlockHeader.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
chainID_ = com.google.protobuf.ByteString.EMPTY;
prevBlockHash_ = com.google.protobuf.ByteString.EMPTY;
blockNo_ = 0L;
timestamp_ = 0L;
blocksRootHash_ = com.google.protobuf.ByteString.EMPTY;
txsRootHash_ = com.google.protobuf.ByteString.EMPTY;
receiptsRootHash_ = com.google.protobuf.ByteString.EMPTY;
confirms_ = 0L;
pubKey_ = com.google.protobuf.ByteString.EMPTY;
coinbaseAccount_ = com.google.protobuf.ByteString.EMPTY;
sign_ = com.google.protobuf.ByteString.EMPTY;
consensus_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_BlockHeader_descriptor;
}
@java.lang.Override
public types.Blockchain.BlockHeader getDefaultInstanceForType() {
return types.Blockchain.BlockHeader.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.BlockHeader build() {
types.Blockchain.BlockHeader result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.BlockHeader buildPartial() {
types.Blockchain.BlockHeader result = new types.Blockchain.BlockHeader(this);
result.chainID_ = chainID_;
result.prevBlockHash_ = prevBlockHash_;
result.blockNo_ = blockNo_;
result.timestamp_ = timestamp_;
result.blocksRootHash_ = blocksRootHash_;
result.txsRootHash_ = txsRootHash_;
result.receiptsRootHash_ = receiptsRootHash_;
result.confirms_ = confirms_;
result.pubKey_ = pubKey_;
result.coinbaseAccount_ = coinbaseAccount_;
result.sign_ = sign_;
result.consensus_ = consensus_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.BlockHeader) {
return mergeFrom((types.Blockchain.BlockHeader)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.BlockHeader other) {
if (other == types.Blockchain.BlockHeader.getDefaultInstance()) return this;
if (other.getChainID() != com.google.protobuf.ByteString.EMPTY) {
setChainID(other.getChainID());
}
if (other.getPrevBlockHash() != com.google.protobuf.ByteString.EMPTY) {
setPrevBlockHash(other.getPrevBlockHash());
}
if (other.getBlockNo() != 0L) {
setBlockNo(other.getBlockNo());
}
if (other.getTimestamp() != 0L) {
setTimestamp(other.getTimestamp());
}
if (other.getBlocksRootHash() != com.google.protobuf.ByteString.EMPTY) {
setBlocksRootHash(other.getBlocksRootHash());
}
if (other.getTxsRootHash() != com.google.protobuf.ByteString.EMPTY) {
setTxsRootHash(other.getTxsRootHash());
}
if (other.getReceiptsRootHash() != com.google.protobuf.ByteString.EMPTY) {
setReceiptsRootHash(other.getReceiptsRootHash());
}
if (other.getConfirms() != 0L) {
setConfirms(other.getConfirms());
}
if (other.getPubKey() != com.google.protobuf.ByteString.EMPTY) {
setPubKey(other.getPubKey());
}
if (other.getCoinbaseAccount() != com.google.protobuf.ByteString.EMPTY) {
setCoinbaseAccount(other.getCoinbaseAccount());
}
if (other.getSign() != com.google.protobuf.ByteString.EMPTY) {
setSign(other.getSign());
}
if (other.getConsensus() != com.google.protobuf.ByteString.EMPTY) {
setConsensus(other.getConsensus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.BlockHeader parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.BlockHeader) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString chainID_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* chain identifier
*
*
* bytes chainID = 1;
* @return The chainID.
*/
@java.lang.Override
public com.google.protobuf.ByteString getChainID() {
return chainID_;
}
/**
*
* chain identifier
*
*
* bytes chainID = 1;
* @param value The chainID to set.
* @return This builder for chaining.
*/
public Builder setChainID(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
chainID_ = value;
onChanged();
return this;
}
/**
*
* chain identifier
*
*
* bytes chainID = 1;
* @return This builder for chaining.
*/
public Builder clearChainID() {
chainID_ = getDefaultInstance().getChainID();
onChanged();
return this;
}
private com.google.protobuf.ByteString prevBlockHash_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* hash of previous block
*
*
* bytes prevBlockHash = 2;
* @return The prevBlockHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPrevBlockHash() {
return prevBlockHash_;
}
/**
*
* hash of previous block
*
*
* bytes prevBlockHash = 2;
* @param value The prevBlockHash to set.
* @return This builder for chaining.
*/
public Builder setPrevBlockHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
prevBlockHash_ = value;
onChanged();
return this;
}
/**
*
* hash of previous block
*
*
* bytes prevBlockHash = 2;
* @return This builder for chaining.
*/
public Builder clearPrevBlockHash() {
prevBlockHash_ = getDefaultInstance().getPrevBlockHash();
onChanged();
return this;
}
private long blockNo_ ;
/**
*
* block number
*
*
* uint64 blockNo = 3;
* @return The blockNo.
*/
@java.lang.Override
public long getBlockNo() {
return blockNo_;
}
/**
*
* block number
*
*
* uint64 blockNo = 3;
* @param value The blockNo to set.
* @return This builder for chaining.
*/
public Builder setBlockNo(long value) {
blockNo_ = value;
onChanged();
return this;
}
/**
*
* block number
*
*
* uint64 blockNo = 3;
* @return This builder for chaining.
*/
public Builder clearBlockNo() {
blockNo_ = 0L;
onChanged();
return this;
}
private long timestamp_ ;
/**
*
* block creation time stamp
*
*
* int64 timestamp = 4;
* @return The timestamp.
*/
@java.lang.Override
public long getTimestamp() {
return timestamp_;
}
/**
*
* block creation time stamp
*
*
* int64 timestamp = 4;
* @param value The timestamp to set.
* @return This builder for chaining.
*/
public Builder setTimestamp(long value) {
timestamp_ = value;
onChanged();
return this;
}
/**
*
* block creation time stamp
*
*
* int64 timestamp = 4;
* @return This builder for chaining.
*/
public Builder clearTimestamp() {
timestamp_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString blocksRootHash_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* hash of root of block merkle tree
*
*
* bytes blocksRootHash = 5;
* @return The blocksRootHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBlocksRootHash() {
return blocksRootHash_;
}
/**
*
* hash of root of block merkle tree
*
*
* bytes blocksRootHash = 5;
* @param value The blocksRootHash to set.
* @return This builder for chaining.
*/
public Builder setBlocksRootHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
blocksRootHash_ = value;
onChanged();
return this;
}
/**
*
* hash of root of block merkle tree
*
*
* bytes blocksRootHash = 5;
* @return This builder for chaining.
*/
public Builder clearBlocksRootHash() {
blocksRootHash_ = getDefaultInstance().getBlocksRootHash();
onChanged();
return this;
}
private com.google.protobuf.ByteString txsRootHash_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* hash of root of transaction merkle tree
*
*
* bytes txsRootHash = 6;
* @return The txsRootHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTxsRootHash() {
return txsRootHash_;
}
/**
*
* hash of root of transaction merkle tree
*
*
* bytes txsRootHash = 6;
* @param value The txsRootHash to set.
* @return This builder for chaining.
*/
public Builder setTxsRootHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
txsRootHash_ = value;
onChanged();
return this;
}
/**
*
* hash of root of transaction merkle tree
*
*
* bytes txsRootHash = 6;
* @return This builder for chaining.
*/
public Builder clearTxsRootHash() {
txsRootHash_ = getDefaultInstance().getTxsRootHash();
onChanged();
return this;
}
private com.google.protobuf.ByteString receiptsRootHash_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* hash of root of receipt merkle tree
*
*
* bytes receiptsRootHash = 7;
* @return The receiptsRootHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getReceiptsRootHash() {
return receiptsRootHash_;
}
/**
*
* hash of root of receipt merkle tree
*
*
* bytes receiptsRootHash = 7;
* @param value The receiptsRootHash to set.
* @return This builder for chaining.
*/
public Builder setReceiptsRootHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
receiptsRootHash_ = value;
onChanged();
return this;
}
/**
*
* hash of root of receipt merkle tree
*
*
* bytes receiptsRootHash = 7;
* @return This builder for chaining.
*/
public Builder clearReceiptsRootHash() {
receiptsRootHash_ = getDefaultInstance().getReceiptsRootHash();
onChanged();
return this;
}
private long confirms_ ;
/**
*
* number of blocks this block is able to confirm
*
*
* uint64 confirms = 8;
* @return The confirms.
*/
@java.lang.Override
public long getConfirms() {
return confirms_;
}
/**
*
* number of blocks this block is able to confirm
*
*
* uint64 confirms = 8;
* @param value The confirms to set.
* @return This builder for chaining.
*/
public Builder setConfirms(long value) {
confirms_ = value;
onChanged();
return this;
}
/**
*
* number of blocks this block is able to confirm
*
*
* uint64 confirms = 8;
* @return This builder for chaining.
*/
public Builder clearConfirms() {
confirms_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString pubKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* block producer's public key
*
*
* bytes pubKey = 9;
* @return The pubKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPubKey() {
return pubKey_;
}
/**
*
* block producer's public key
*
*
* bytes pubKey = 9;
* @param value The pubKey to set.
* @return This builder for chaining.
*/
public Builder setPubKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
pubKey_ = value;
onChanged();
return this;
}
/**
*
* block producer's public key
*
*
* bytes pubKey = 9;
* @return This builder for chaining.
*/
public Builder clearPubKey() {
pubKey_ = getDefaultInstance().getPubKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString coinbaseAccount_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* address of account to receive fees
*
*
* bytes coinbaseAccount = 10;
* @return The coinbaseAccount.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCoinbaseAccount() {
return coinbaseAccount_;
}
/**
*
* address of account to receive fees
*
*
* bytes coinbaseAccount = 10;
* @param value The coinbaseAccount to set.
* @return This builder for chaining.
*/
public Builder setCoinbaseAccount(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
coinbaseAccount_ = value;
onChanged();
return this;
}
/**
*
* address of account to receive fees
*
*
* bytes coinbaseAccount = 10;
* @return This builder for chaining.
*/
public Builder clearCoinbaseAccount() {
coinbaseAccount_ = getDefaultInstance().getCoinbaseAccount();
onChanged();
return this;
}
private com.google.protobuf.ByteString sign_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* block producer's signature of BlockHeader
*
*
* bytes sign = 11;
* @return The sign.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSign() {
return sign_;
}
/**
*
* block producer's signature of BlockHeader
*
*
* bytes sign = 11;
* @param value The sign to set.
* @return This builder for chaining.
*/
public Builder setSign(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
sign_ = value;
onChanged();
return this;
}
/**
*
* block producer's signature of BlockHeader
*
*
* bytes sign = 11;
* @return This builder for chaining.
*/
public Builder clearSign() {
sign_ = getDefaultInstance().getSign();
onChanged();
return this;
}
private com.google.protobuf.ByteString consensus_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* consensus meta
*
*
* bytes consensus = 12;
* @return The consensus.
*/
@java.lang.Override
public com.google.protobuf.ByteString getConsensus() {
return consensus_;
}
/**
*
* consensus meta
*
*
* bytes consensus = 12;
* @param value The consensus to set.
* @return This builder for chaining.
*/
public Builder setConsensus(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
consensus_ = value;
onChanged();
return this;
}
/**
*
* consensus meta
*
*
* bytes consensus = 12;
* @return This builder for chaining.
*/
public Builder clearConsensus() {
consensus_ = getDefaultInstance().getConsensus();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.BlockHeader)
}
// @@protoc_insertion_point(class_scope:types.BlockHeader)
private static final types.Blockchain.BlockHeader DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.BlockHeader();
}
public static types.Blockchain.BlockHeader getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BlockHeader parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BlockHeader(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.BlockHeader getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BlockBodyOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.BlockBody)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .types.Tx txs = 1;
*/
java.util.List
getTxsList();
/**
* repeated .types.Tx txs = 1;
*/
types.Blockchain.Tx getTxs(int index);
/**
* repeated .types.Tx txs = 1;
*/
int getTxsCount();
/**
* repeated .types.Tx txs = 1;
*/
java.util.List extends types.Blockchain.TxOrBuilder>
getTxsOrBuilderList();
/**
* repeated .types.Tx txs = 1;
*/
types.Blockchain.TxOrBuilder getTxsOrBuilder(
int index);
}
/**
* Protobuf type {@code types.BlockBody}
*/
public static final class BlockBody extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.BlockBody)
BlockBodyOrBuilder {
private static final long serialVersionUID = 0L;
// Use BlockBody.newBuilder() to construct.
private BlockBody(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BlockBody() {
txs_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new BlockBody();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BlockBody(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
txs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
txs_.add(
input.readMessage(types.Blockchain.Tx.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
txs_ = java.util.Collections.unmodifiableList(txs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_BlockBody_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_BlockBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.BlockBody.class, types.Blockchain.BlockBody.Builder.class);
}
public static final int TXS_FIELD_NUMBER = 1;
private java.util.List txs_;
/**
* repeated .types.Tx txs = 1;
*/
@java.lang.Override
public java.util.List getTxsList() {
return txs_;
}
/**
* repeated .types.Tx txs = 1;
*/
@java.lang.Override
public java.util.List extends types.Blockchain.TxOrBuilder>
getTxsOrBuilderList() {
return txs_;
}
/**
* repeated .types.Tx txs = 1;
*/
@java.lang.Override
public int getTxsCount() {
return txs_.size();
}
/**
* repeated .types.Tx txs = 1;
*/
@java.lang.Override
public types.Blockchain.Tx getTxs(int index) {
return txs_.get(index);
}
/**
* repeated .types.Tx txs = 1;
*/
@java.lang.Override
public types.Blockchain.TxOrBuilder getTxsOrBuilder(
int index) {
return txs_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < txs_.size(); i++) {
output.writeMessage(1, txs_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < txs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, txs_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.BlockBody)) {
return super.equals(obj);
}
types.Blockchain.BlockBody other = (types.Blockchain.BlockBody) obj;
if (!getTxsList()
.equals(other.getTxsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getTxsCount() > 0) {
hash = (37 * hash) + TXS_FIELD_NUMBER;
hash = (53 * hash) + getTxsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.BlockBody parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.BlockBody parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.BlockBody parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.BlockBody parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.BlockBody parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.BlockBody parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.BlockBody parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.BlockBody parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.BlockBody parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.BlockBody parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.BlockBody parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.BlockBody parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.BlockBody prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.BlockBody}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.BlockBody)
types.Blockchain.BlockBodyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_BlockBody_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_BlockBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.BlockBody.class, types.Blockchain.BlockBody.Builder.class);
}
// Construct using types.Blockchain.BlockBody.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTxsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (txsBuilder_ == null) {
txs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
txsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_BlockBody_descriptor;
}
@java.lang.Override
public types.Blockchain.BlockBody getDefaultInstanceForType() {
return types.Blockchain.BlockBody.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.BlockBody build() {
types.Blockchain.BlockBody result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.BlockBody buildPartial() {
types.Blockchain.BlockBody result = new types.Blockchain.BlockBody(this);
int from_bitField0_ = bitField0_;
if (txsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
txs_ = java.util.Collections.unmodifiableList(txs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.txs_ = txs_;
} else {
result.txs_ = txsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.BlockBody) {
return mergeFrom((types.Blockchain.BlockBody)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.BlockBody other) {
if (other == types.Blockchain.BlockBody.getDefaultInstance()) return this;
if (txsBuilder_ == null) {
if (!other.txs_.isEmpty()) {
if (txs_.isEmpty()) {
txs_ = other.txs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTxsIsMutable();
txs_.addAll(other.txs_);
}
onChanged();
}
} else {
if (!other.txs_.isEmpty()) {
if (txsBuilder_.isEmpty()) {
txsBuilder_.dispose();
txsBuilder_ = null;
txs_ = other.txs_;
bitField0_ = (bitField0_ & ~0x00000001);
txsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getTxsFieldBuilder() : null;
} else {
txsBuilder_.addAllMessages(other.txs_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.BlockBody parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.BlockBody) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List txs_ =
java.util.Collections.emptyList();
private void ensureTxsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
txs_ = new java.util.ArrayList(txs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.Tx, types.Blockchain.Tx.Builder, types.Blockchain.TxOrBuilder> txsBuilder_;
/**
* repeated .types.Tx txs = 1;
*/
public java.util.List getTxsList() {
if (txsBuilder_ == null) {
return java.util.Collections.unmodifiableList(txs_);
} else {
return txsBuilder_.getMessageList();
}
}
/**
* repeated .types.Tx txs = 1;
*/
public int getTxsCount() {
if (txsBuilder_ == null) {
return txs_.size();
} else {
return txsBuilder_.getCount();
}
}
/**
* repeated .types.Tx txs = 1;
*/
public types.Blockchain.Tx getTxs(int index) {
if (txsBuilder_ == null) {
return txs_.get(index);
} else {
return txsBuilder_.getMessage(index);
}
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder setTxs(
int index, types.Blockchain.Tx value) {
if (txsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTxsIsMutable();
txs_.set(index, value);
onChanged();
} else {
txsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder setTxs(
int index, types.Blockchain.Tx.Builder builderForValue) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
txs_.set(index, builderForValue.build());
onChanged();
} else {
txsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder addTxs(types.Blockchain.Tx value) {
if (txsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTxsIsMutable();
txs_.add(value);
onChanged();
} else {
txsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder addTxs(
int index, types.Blockchain.Tx value) {
if (txsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTxsIsMutable();
txs_.add(index, value);
onChanged();
} else {
txsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder addTxs(
types.Blockchain.Tx.Builder builderForValue) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
txs_.add(builderForValue.build());
onChanged();
} else {
txsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder addTxs(
int index, types.Blockchain.Tx.Builder builderForValue) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
txs_.add(index, builderForValue.build());
onChanged();
} else {
txsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder addAllTxs(
java.lang.Iterable extends types.Blockchain.Tx> values) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, txs_);
onChanged();
} else {
txsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder clearTxs() {
if (txsBuilder_ == null) {
txs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
txsBuilder_.clear();
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder removeTxs(int index) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
txs_.remove(index);
onChanged();
} else {
txsBuilder_.remove(index);
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public types.Blockchain.Tx.Builder getTxsBuilder(
int index) {
return getTxsFieldBuilder().getBuilder(index);
}
/**
* repeated .types.Tx txs = 1;
*/
public types.Blockchain.TxOrBuilder getTxsOrBuilder(
int index) {
if (txsBuilder_ == null) {
return txs_.get(index); } else {
return txsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .types.Tx txs = 1;
*/
public java.util.List extends types.Blockchain.TxOrBuilder>
getTxsOrBuilderList() {
if (txsBuilder_ != null) {
return txsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(txs_);
}
}
/**
* repeated .types.Tx txs = 1;
*/
public types.Blockchain.Tx.Builder addTxsBuilder() {
return getTxsFieldBuilder().addBuilder(
types.Blockchain.Tx.getDefaultInstance());
}
/**
* repeated .types.Tx txs = 1;
*/
public types.Blockchain.Tx.Builder addTxsBuilder(
int index) {
return getTxsFieldBuilder().addBuilder(
index, types.Blockchain.Tx.getDefaultInstance());
}
/**
* repeated .types.Tx txs = 1;
*/
public java.util.List
getTxsBuilderList() {
return getTxsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.Tx, types.Blockchain.Tx.Builder, types.Blockchain.TxOrBuilder>
getTxsFieldBuilder() {
if (txsBuilder_ == null) {
txsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.Tx, types.Blockchain.Tx.Builder, types.Blockchain.TxOrBuilder>(
txs_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
txs_ = null;
}
return txsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.BlockBody)
}
// @@protoc_insertion_point(class_scope:types.BlockBody)
private static final types.Blockchain.BlockBody DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.BlockBody();
}
public static types.Blockchain.BlockBody getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BlockBody parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BlockBody(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.BlockBody getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TxListOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.TxList)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .types.Tx txs = 1;
*/
java.util.List
getTxsList();
/**
* repeated .types.Tx txs = 1;
*/
types.Blockchain.Tx getTxs(int index);
/**
* repeated .types.Tx txs = 1;
*/
int getTxsCount();
/**
* repeated .types.Tx txs = 1;
*/
java.util.List extends types.Blockchain.TxOrBuilder>
getTxsOrBuilderList();
/**
* repeated .types.Tx txs = 1;
*/
types.Blockchain.TxOrBuilder getTxsOrBuilder(
int index);
}
/**
* Protobuf type {@code types.TxList}
*/
public static final class TxList extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.TxList)
TxListOrBuilder {
private static final long serialVersionUID = 0L;
// Use TxList.newBuilder() to construct.
private TxList(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TxList() {
txs_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TxList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TxList(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
txs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
txs_.add(
input.readMessage(types.Blockchain.Tx.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
txs_ = java.util.Collections.unmodifiableList(txs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_TxList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_TxList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.TxList.class, types.Blockchain.TxList.Builder.class);
}
public static final int TXS_FIELD_NUMBER = 1;
private java.util.List txs_;
/**
* repeated .types.Tx txs = 1;
*/
@java.lang.Override
public java.util.List getTxsList() {
return txs_;
}
/**
* repeated .types.Tx txs = 1;
*/
@java.lang.Override
public java.util.List extends types.Blockchain.TxOrBuilder>
getTxsOrBuilderList() {
return txs_;
}
/**
* repeated .types.Tx txs = 1;
*/
@java.lang.Override
public int getTxsCount() {
return txs_.size();
}
/**
* repeated .types.Tx txs = 1;
*/
@java.lang.Override
public types.Blockchain.Tx getTxs(int index) {
return txs_.get(index);
}
/**
* repeated .types.Tx txs = 1;
*/
@java.lang.Override
public types.Blockchain.TxOrBuilder getTxsOrBuilder(
int index) {
return txs_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < txs_.size(); i++) {
output.writeMessage(1, txs_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < txs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, txs_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.TxList)) {
return super.equals(obj);
}
types.Blockchain.TxList other = (types.Blockchain.TxList) obj;
if (!getTxsList()
.equals(other.getTxsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getTxsCount() > 0) {
hash = (37 * hash) + TXS_FIELD_NUMBER;
hash = (53 * hash) + getTxsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.TxList parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.TxList parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.TxList parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.TxList parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.TxList parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.TxList parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.TxList parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.TxList parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.TxList parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.TxList parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.TxList parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.TxList parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.TxList prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.TxList}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.TxList)
types.Blockchain.TxListOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_TxList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_TxList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.TxList.class, types.Blockchain.TxList.Builder.class);
}
// Construct using types.Blockchain.TxList.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTxsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (txsBuilder_ == null) {
txs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
txsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_TxList_descriptor;
}
@java.lang.Override
public types.Blockchain.TxList getDefaultInstanceForType() {
return types.Blockchain.TxList.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.TxList build() {
types.Blockchain.TxList result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.TxList buildPartial() {
types.Blockchain.TxList result = new types.Blockchain.TxList(this);
int from_bitField0_ = bitField0_;
if (txsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
txs_ = java.util.Collections.unmodifiableList(txs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.txs_ = txs_;
} else {
result.txs_ = txsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.TxList) {
return mergeFrom((types.Blockchain.TxList)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.TxList other) {
if (other == types.Blockchain.TxList.getDefaultInstance()) return this;
if (txsBuilder_ == null) {
if (!other.txs_.isEmpty()) {
if (txs_.isEmpty()) {
txs_ = other.txs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTxsIsMutable();
txs_.addAll(other.txs_);
}
onChanged();
}
} else {
if (!other.txs_.isEmpty()) {
if (txsBuilder_.isEmpty()) {
txsBuilder_.dispose();
txsBuilder_ = null;
txs_ = other.txs_;
bitField0_ = (bitField0_ & ~0x00000001);
txsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getTxsFieldBuilder() : null;
} else {
txsBuilder_.addAllMessages(other.txs_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.TxList parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.TxList) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List txs_ =
java.util.Collections.emptyList();
private void ensureTxsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
txs_ = new java.util.ArrayList(txs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.Tx, types.Blockchain.Tx.Builder, types.Blockchain.TxOrBuilder> txsBuilder_;
/**
* repeated .types.Tx txs = 1;
*/
public java.util.List getTxsList() {
if (txsBuilder_ == null) {
return java.util.Collections.unmodifiableList(txs_);
} else {
return txsBuilder_.getMessageList();
}
}
/**
* repeated .types.Tx txs = 1;
*/
public int getTxsCount() {
if (txsBuilder_ == null) {
return txs_.size();
} else {
return txsBuilder_.getCount();
}
}
/**
* repeated .types.Tx txs = 1;
*/
public types.Blockchain.Tx getTxs(int index) {
if (txsBuilder_ == null) {
return txs_.get(index);
} else {
return txsBuilder_.getMessage(index);
}
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder setTxs(
int index, types.Blockchain.Tx value) {
if (txsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTxsIsMutable();
txs_.set(index, value);
onChanged();
} else {
txsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder setTxs(
int index, types.Blockchain.Tx.Builder builderForValue) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
txs_.set(index, builderForValue.build());
onChanged();
} else {
txsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder addTxs(types.Blockchain.Tx value) {
if (txsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTxsIsMutable();
txs_.add(value);
onChanged();
} else {
txsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder addTxs(
int index, types.Blockchain.Tx value) {
if (txsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTxsIsMutable();
txs_.add(index, value);
onChanged();
} else {
txsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder addTxs(
types.Blockchain.Tx.Builder builderForValue) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
txs_.add(builderForValue.build());
onChanged();
} else {
txsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder addTxs(
int index, types.Blockchain.Tx.Builder builderForValue) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
txs_.add(index, builderForValue.build());
onChanged();
} else {
txsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder addAllTxs(
java.lang.Iterable extends types.Blockchain.Tx> values) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, txs_);
onChanged();
} else {
txsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder clearTxs() {
if (txsBuilder_ == null) {
txs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
txsBuilder_.clear();
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public Builder removeTxs(int index) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
txs_.remove(index);
onChanged();
} else {
txsBuilder_.remove(index);
}
return this;
}
/**
* repeated .types.Tx txs = 1;
*/
public types.Blockchain.Tx.Builder getTxsBuilder(
int index) {
return getTxsFieldBuilder().getBuilder(index);
}
/**
* repeated .types.Tx txs = 1;
*/
public types.Blockchain.TxOrBuilder getTxsOrBuilder(
int index) {
if (txsBuilder_ == null) {
return txs_.get(index); } else {
return txsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .types.Tx txs = 1;
*/
public java.util.List extends types.Blockchain.TxOrBuilder>
getTxsOrBuilderList() {
if (txsBuilder_ != null) {
return txsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(txs_);
}
}
/**
* repeated .types.Tx txs = 1;
*/
public types.Blockchain.Tx.Builder addTxsBuilder() {
return getTxsFieldBuilder().addBuilder(
types.Blockchain.Tx.getDefaultInstance());
}
/**
* repeated .types.Tx txs = 1;
*/
public types.Blockchain.Tx.Builder addTxsBuilder(
int index) {
return getTxsFieldBuilder().addBuilder(
index, types.Blockchain.Tx.getDefaultInstance());
}
/**
* repeated .types.Tx txs = 1;
*/
public java.util.List
getTxsBuilderList() {
return getTxsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.Tx, types.Blockchain.Tx.Builder, types.Blockchain.TxOrBuilder>
getTxsFieldBuilder() {
if (txsBuilder_ == null) {
txsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.Tx, types.Blockchain.Tx.Builder, types.Blockchain.TxOrBuilder>(
txs_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
txs_ = null;
}
return txsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.TxList)
}
// @@protoc_insertion_point(class_scope:types.TxList)
private static final types.Blockchain.TxList DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.TxList();
}
public static types.Blockchain.TxList getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TxList parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TxList(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.TxList getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TxOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.Tx)
com.google.protobuf.MessageOrBuilder {
/**
* bytes hash = 1;
* @return The hash.
*/
com.google.protobuf.ByteString getHash();
/**
* .types.TxBody body = 2;
* @return Whether the body field is set.
*/
boolean hasBody();
/**
* .types.TxBody body = 2;
* @return The body.
*/
types.Blockchain.TxBody getBody();
/**
* .types.TxBody body = 2;
*/
types.Blockchain.TxBodyOrBuilder getBodyOrBuilder();
}
/**
* Protobuf type {@code types.Tx}
*/
public static final class Tx extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.Tx)
TxOrBuilder {
private static final long serialVersionUID = 0L;
// Use Tx.newBuilder() to construct.
private Tx(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Tx() {
hash_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Tx();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Tx(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
hash_ = input.readBytes();
break;
}
case 18: {
types.Blockchain.TxBody.Builder subBuilder = null;
if (body_ != null) {
subBuilder = body_.toBuilder();
}
body_ = input.readMessage(types.Blockchain.TxBody.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(body_);
body_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_Tx_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_Tx_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.Tx.class, types.Blockchain.Tx.Builder.class);
}
public static final int HASH_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString hash_;
/**
* bytes hash = 1;
* @return The hash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getHash() {
return hash_;
}
public static final int BODY_FIELD_NUMBER = 2;
private types.Blockchain.TxBody body_;
/**
* .types.TxBody body = 2;
* @return Whether the body field is set.
*/
@java.lang.Override
public boolean hasBody() {
return body_ != null;
}
/**
* .types.TxBody body = 2;
* @return The body.
*/
@java.lang.Override
public types.Blockchain.TxBody getBody() {
return body_ == null ? types.Blockchain.TxBody.getDefaultInstance() : body_;
}
/**
* .types.TxBody body = 2;
*/
@java.lang.Override
public types.Blockchain.TxBodyOrBuilder getBodyOrBuilder() {
return getBody();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!hash_.isEmpty()) {
output.writeBytes(1, hash_);
}
if (body_ != null) {
output.writeMessage(2, getBody());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!hash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, hash_);
}
if (body_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getBody());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.Tx)) {
return super.equals(obj);
}
types.Blockchain.Tx other = (types.Blockchain.Tx) obj;
if (!getHash()
.equals(other.getHash())) return false;
if (hasBody() != other.hasBody()) return false;
if (hasBody()) {
if (!getBody()
.equals(other.getBody())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + HASH_FIELD_NUMBER;
hash = (53 * hash) + getHash().hashCode();
if (hasBody()) {
hash = (37 * hash) + BODY_FIELD_NUMBER;
hash = (53 * hash) + getBody().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.Tx parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Tx parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Tx parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Tx parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Tx parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Tx parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Tx parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.Tx parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.Tx parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.Tx parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.Tx parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.Tx parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.Tx prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.Tx}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.Tx)
types.Blockchain.TxOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_Tx_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_Tx_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.Tx.class, types.Blockchain.Tx.Builder.class);
}
// Construct using types.Blockchain.Tx.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
hash_ = com.google.protobuf.ByteString.EMPTY;
if (bodyBuilder_ == null) {
body_ = null;
} else {
body_ = null;
bodyBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_Tx_descriptor;
}
@java.lang.Override
public types.Blockchain.Tx getDefaultInstanceForType() {
return types.Blockchain.Tx.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.Tx build() {
types.Blockchain.Tx result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.Tx buildPartial() {
types.Blockchain.Tx result = new types.Blockchain.Tx(this);
result.hash_ = hash_;
if (bodyBuilder_ == null) {
result.body_ = body_;
} else {
result.body_ = bodyBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.Tx) {
return mergeFrom((types.Blockchain.Tx)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.Tx other) {
if (other == types.Blockchain.Tx.getDefaultInstance()) return this;
if (other.getHash() != com.google.protobuf.ByteString.EMPTY) {
setHash(other.getHash());
}
if (other.hasBody()) {
mergeBody(other.getBody());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.Tx parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.Tx) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString hash_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes hash = 1;
* @return The hash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getHash() {
return hash_;
}
/**
* bytes hash = 1;
* @param value The hash to set.
* @return This builder for chaining.
*/
public Builder setHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
hash_ = value;
onChanged();
return this;
}
/**
* bytes hash = 1;
* @return This builder for chaining.
*/
public Builder clearHash() {
hash_ = getDefaultInstance().getHash();
onChanged();
return this;
}
private types.Blockchain.TxBody body_;
private com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.TxBody, types.Blockchain.TxBody.Builder, types.Blockchain.TxBodyOrBuilder> bodyBuilder_;
/**
* .types.TxBody body = 2;
* @return Whether the body field is set.
*/
public boolean hasBody() {
return bodyBuilder_ != null || body_ != null;
}
/**
* .types.TxBody body = 2;
* @return The body.
*/
public types.Blockchain.TxBody getBody() {
if (bodyBuilder_ == null) {
return body_ == null ? types.Blockchain.TxBody.getDefaultInstance() : body_;
} else {
return bodyBuilder_.getMessage();
}
}
/**
* .types.TxBody body = 2;
*/
public Builder setBody(types.Blockchain.TxBody value) {
if (bodyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
body_ = value;
onChanged();
} else {
bodyBuilder_.setMessage(value);
}
return this;
}
/**
* .types.TxBody body = 2;
*/
public Builder setBody(
types.Blockchain.TxBody.Builder builderForValue) {
if (bodyBuilder_ == null) {
body_ = builderForValue.build();
onChanged();
} else {
bodyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .types.TxBody body = 2;
*/
public Builder mergeBody(types.Blockchain.TxBody value) {
if (bodyBuilder_ == null) {
if (body_ != null) {
body_ =
types.Blockchain.TxBody.newBuilder(body_).mergeFrom(value).buildPartial();
} else {
body_ = value;
}
onChanged();
} else {
bodyBuilder_.mergeFrom(value);
}
return this;
}
/**
* .types.TxBody body = 2;
*/
public Builder clearBody() {
if (bodyBuilder_ == null) {
body_ = null;
onChanged();
} else {
body_ = null;
bodyBuilder_ = null;
}
return this;
}
/**
* .types.TxBody body = 2;
*/
public types.Blockchain.TxBody.Builder getBodyBuilder() {
onChanged();
return getBodyFieldBuilder().getBuilder();
}
/**
* .types.TxBody body = 2;
*/
public types.Blockchain.TxBodyOrBuilder getBodyOrBuilder() {
if (bodyBuilder_ != null) {
return bodyBuilder_.getMessageOrBuilder();
} else {
return body_ == null ?
types.Blockchain.TxBody.getDefaultInstance() : body_;
}
}
/**
* .types.TxBody body = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.TxBody, types.Blockchain.TxBody.Builder, types.Blockchain.TxBodyOrBuilder>
getBodyFieldBuilder() {
if (bodyBuilder_ == null) {
bodyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.TxBody, types.Blockchain.TxBody.Builder, types.Blockchain.TxBodyOrBuilder>(
getBody(),
getParentForChildren(),
isClean());
body_ = null;
}
return bodyBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.Tx)
}
// @@protoc_insertion_point(class_scope:types.Tx)
private static final types.Blockchain.Tx DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.Tx();
}
public static types.Blockchain.Tx getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Tx parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Tx(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.Tx getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TxBodyOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.TxBody)
com.google.protobuf.MessageOrBuilder {
/**
*
* increasing number used only once per sender account
*
*
* uint64 nonce = 1;
* @return The nonce.
*/
long getNonce();
/**
*
* decoded account address
*
*
* bytes account = 2;
* @return The account.
*/
com.google.protobuf.ByteString getAccount();
/**
*
* decoded account address
*
*
* bytes recipient = 3;
* @return The recipient.
*/
com.google.protobuf.ByteString getRecipient();
/**
*
* variable-length big integer
*
*
* bytes amount = 4;
* @return The amount.
*/
com.google.protobuf.ByteString getAmount();
/**
* bytes payload = 5;
* @return The payload.
*/
com.google.protobuf.ByteString getPayload();
/**
*
* maximum gas used for this transaction. 0 = no limit
*
*
* uint64 gasLimit = 6;
* @return The gasLimit.
*/
long getGasLimit();
/**
*
* variable-length big integer. currently not used
*
*
* bytes gasPrice = 7;
* @return The gasPrice.
*/
com.google.protobuf.ByteString getGasPrice();
/**
* .types.TxType type = 8;
* @return The enum numeric value on the wire for type.
*/
int getTypeValue();
/**
* .types.TxType type = 8;
* @return The type.
*/
types.Blockchain.TxType getType();
/**
*
* hash value of chain identifier in the block
*
*
* bytes chainIdHash = 9;
* @return The chainIdHash.
*/
com.google.protobuf.ByteString getChainIdHash();
/**
*
* sender's signature for this TxBody
*
*
* bytes sign = 10;
* @return The sign.
*/
com.google.protobuf.ByteString getSign();
}
/**
* Protobuf type {@code types.TxBody}
*/
public static final class TxBody extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.TxBody)
TxBodyOrBuilder {
private static final long serialVersionUID = 0L;
// Use TxBody.newBuilder() to construct.
private TxBody(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TxBody() {
account_ = com.google.protobuf.ByteString.EMPTY;
recipient_ = com.google.protobuf.ByteString.EMPTY;
amount_ = com.google.protobuf.ByteString.EMPTY;
payload_ = com.google.protobuf.ByteString.EMPTY;
gasPrice_ = com.google.protobuf.ByteString.EMPTY;
type_ = 0;
chainIdHash_ = com.google.protobuf.ByteString.EMPTY;
sign_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TxBody();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TxBody(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
nonce_ = input.readUInt64();
break;
}
case 18: {
account_ = input.readBytes();
break;
}
case 26: {
recipient_ = input.readBytes();
break;
}
case 34: {
amount_ = input.readBytes();
break;
}
case 42: {
payload_ = input.readBytes();
break;
}
case 48: {
gasLimit_ = input.readUInt64();
break;
}
case 58: {
gasPrice_ = input.readBytes();
break;
}
case 64: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 74: {
chainIdHash_ = input.readBytes();
break;
}
case 82: {
sign_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_TxBody_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_TxBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.TxBody.class, types.Blockchain.TxBody.Builder.class);
}
public static final int NONCE_FIELD_NUMBER = 1;
private long nonce_;
/**
*
* increasing number used only once per sender account
*
*
* uint64 nonce = 1;
* @return The nonce.
*/
@java.lang.Override
public long getNonce() {
return nonce_;
}
public static final int ACCOUNT_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString account_;
/**
*
* decoded account address
*
*
* bytes account = 2;
* @return The account.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAccount() {
return account_;
}
public static final int RECIPIENT_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString recipient_;
/**
*
* decoded account address
*
*
* bytes recipient = 3;
* @return The recipient.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRecipient() {
return recipient_;
}
public static final int AMOUNT_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString amount_;
/**
*
* variable-length big integer
*
*
* bytes amount = 4;
* @return The amount.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAmount() {
return amount_;
}
public static final int PAYLOAD_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString payload_;
/**
* bytes payload = 5;
* @return The payload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPayload() {
return payload_;
}
public static final int GASLIMIT_FIELD_NUMBER = 6;
private long gasLimit_;
/**
*
* maximum gas used for this transaction. 0 = no limit
*
*
* uint64 gasLimit = 6;
* @return The gasLimit.
*/
@java.lang.Override
public long getGasLimit() {
return gasLimit_;
}
public static final int GASPRICE_FIELD_NUMBER = 7;
private com.google.protobuf.ByteString gasPrice_;
/**
*
* variable-length big integer. currently not used
*
*
* bytes gasPrice = 7;
* @return The gasPrice.
*/
@java.lang.Override
public com.google.protobuf.ByteString getGasPrice() {
return gasPrice_;
}
public static final int TYPE_FIELD_NUMBER = 8;
private int type_;
/**
* .types.TxType type = 8;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .types.TxType type = 8;
* @return The type.
*/
@java.lang.Override public types.Blockchain.TxType getType() {
@SuppressWarnings("deprecation")
types.Blockchain.TxType result = types.Blockchain.TxType.valueOf(type_);
return result == null ? types.Blockchain.TxType.UNRECOGNIZED : result;
}
public static final int CHAINIDHASH_FIELD_NUMBER = 9;
private com.google.protobuf.ByteString chainIdHash_;
/**
*
* hash value of chain identifier in the block
*
*
* bytes chainIdHash = 9;
* @return The chainIdHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getChainIdHash() {
return chainIdHash_;
}
public static final int SIGN_FIELD_NUMBER = 10;
private com.google.protobuf.ByteString sign_;
/**
*
* sender's signature for this TxBody
*
*
* bytes sign = 10;
* @return The sign.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSign() {
return sign_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (nonce_ != 0L) {
output.writeUInt64(1, nonce_);
}
if (!account_.isEmpty()) {
output.writeBytes(2, account_);
}
if (!recipient_.isEmpty()) {
output.writeBytes(3, recipient_);
}
if (!amount_.isEmpty()) {
output.writeBytes(4, amount_);
}
if (!payload_.isEmpty()) {
output.writeBytes(5, payload_);
}
if (gasLimit_ != 0L) {
output.writeUInt64(6, gasLimit_);
}
if (!gasPrice_.isEmpty()) {
output.writeBytes(7, gasPrice_);
}
if (type_ != types.Blockchain.TxType.NORMAL.getNumber()) {
output.writeEnum(8, type_);
}
if (!chainIdHash_.isEmpty()) {
output.writeBytes(9, chainIdHash_);
}
if (!sign_.isEmpty()) {
output.writeBytes(10, sign_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (nonce_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, nonce_);
}
if (!account_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, account_);
}
if (!recipient_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, recipient_);
}
if (!amount_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, amount_);
}
if (!payload_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, payload_);
}
if (gasLimit_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(6, gasLimit_);
}
if (!gasPrice_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, gasPrice_);
}
if (type_ != types.Blockchain.TxType.NORMAL.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(8, type_);
}
if (!chainIdHash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(9, chainIdHash_);
}
if (!sign_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(10, sign_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.TxBody)) {
return super.equals(obj);
}
types.Blockchain.TxBody other = (types.Blockchain.TxBody) obj;
if (getNonce()
!= other.getNonce()) return false;
if (!getAccount()
.equals(other.getAccount())) return false;
if (!getRecipient()
.equals(other.getRecipient())) return false;
if (!getAmount()
.equals(other.getAmount())) return false;
if (!getPayload()
.equals(other.getPayload())) return false;
if (getGasLimit()
!= other.getGasLimit()) return false;
if (!getGasPrice()
.equals(other.getGasPrice())) return false;
if (type_ != other.type_) return false;
if (!getChainIdHash()
.equals(other.getChainIdHash())) return false;
if (!getSign()
.equals(other.getSign())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NONCE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNonce());
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
hash = (37 * hash) + RECIPIENT_FIELD_NUMBER;
hash = (53 * hash) + getRecipient().hashCode();
hash = (37 * hash) + AMOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAmount().hashCode();
hash = (37 * hash) + PAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getPayload().hashCode();
hash = (37 * hash) + GASLIMIT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getGasLimit());
hash = (37 * hash) + GASPRICE_FIELD_NUMBER;
hash = (53 * hash) + getGasPrice().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
hash = (37 * hash) + CHAINIDHASH_FIELD_NUMBER;
hash = (53 * hash) + getChainIdHash().hashCode();
hash = (37 * hash) + SIGN_FIELD_NUMBER;
hash = (53 * hash) + getSign().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.TxBody parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.TxBody parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.TxBody parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.TxBody parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.TxBody parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.TxBody parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.TxBody parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.TxBody parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.TxBody parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.TxBody parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.TxBody parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.TxBody parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.TxBody prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.TxBody}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.TxBody)
types.Blockchain.TxBodyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_TxBody_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_TxBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.TxBody.class, types.Blockchain.TxBody.Builder.class);
}
// Construct using types.Blockchain.TxBody.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
nonce_ = 0L;
account_ = com.google.protobuf.ByteString.EMPTY;
recipient_ = com.google.protobuf.ByteString.EMPTY;
amount_ = com.google.protobuf.ByteString.EMPTY;
payload_ = com.google.protobuf.ByteString.EMPTY;
gasLimit_ = 0L;
gasPrice_ = com.google.protobuf.ByteString.EMPTY;
type_ = 0;
chainIdHash_ = com.google.protobuf.ByteString.EMPTY;
sign_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_TxBody_descriptor;
}
@java.lang.Override
public types.Blockchain.TxBody getDefaultInstanceForType() {
return types.Blockchain.TxBody.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.TxBody build() {
types.Blockchain.TxBody result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.TxBody buildPartial() {
types.Blockchain.TxBody result = new types.Blockchain.TxBody(this);
result.nonce_ = nonce_;
result.account_ = account_;
result.recipient_ = recipient_;
result.amount_ = amount_;
result.payload_ = payload_;
result.gasLimit_ = gasLimit_;
result.gasPrice_ = gasPrice_;
result.type_ = type_;
result.chainIdHash_ = chainIdHash_;
result.sign_ = sign_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.TxBody) {
return mergeFrom((types.Blockchain.TxBody)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.TxBody other) {
if (other == types.Blockchain.TxBody.getDefaultInstance()) return this;
if (other.getNonce() != 0L) {
setNonce(other.getNonce());
}
if (other.getAccount() != com.google.protobuf.ByteString.EMPTY) {
setAccount(other.getAccount());
}
if (other.getRecipient() != com.google.protobuf.ByteString.EMPTY) {
setRecipient(other.getRecipient());
}
if (other.getAmount() != com.google.protobuf.ByteString.EMPTY) {
setAmount(other.getAmount());
}
if (other.getPayload() != com.google.protobuf.ByteString.EMPTY) {
setPayload(other.getPayload());
}
if (other.getGasLimit() != 0L) {
setGasLimit(other.getGasLimit());
}
if (other.getGasPrice() != com.google.protobuf.ByteString.EMPTY) {
setGasPrice(other.getGasPrice());
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.getChainIdHash() != com.google.protobuf.ByteString.EMPTY) {
setChainIdHash(other.getChainIdHash());
}
if (other.getSign() != com.google.protobuf.ByteString.EMPTY) {
setSign(other.getSign());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.TxBody parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.TxBody) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long nonce_ ;
/**
*
* increasing number used only once per sender account
*
*
* uint64 nonce = 1;
* @return The nonce.
*/
@java.lang.Override
public long getNonce() {
return nonce_;
}
/**
*
* increasing number used only once per sender account
*
*
* uint64 nonce = 1;
* @param value The nonce to set.
* @return This builder for chaining.
*/
public Builder setNonce(long value) {
nonce_ = value;
onChanged();
return this;
}
/**
*
* increasing number used only once per sender account
*
*
* uint64 nonce = 1;
* @return This builder for chaining.
*/
public Builder clearNonce() {
nonce_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString account_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* decoded account address
*
*
* bytes account = 2;
* @return The account.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAccount() {
return account_;
}
/**
*
* decoded account address
*
*
* bytes account = 2;
* @param value The account to set.
* @return This builder for chaining.
*/
public Builder setAccount(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
return this;
}
/**
*
* decoded account address
*
*
* bytes account = 2;
* @return This builder for chaining.
*/
public Builder clearAccount() {
account_ = getDefaultInstance().getAccount();
onChanged();
return this;
}
private com.google.protobuf.ByteString recipient_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* decoded account address
*
*
* bytes recipient = 3;
* @return The recipient.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRecipient() {
return recipient_;
}
/**
*
* decoded account address
*
*
* bytes recipient = 3;
* @param value The recipient to set.
* @return This builder for chaining.
*/
public Builder setRecipient(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
recipient_ = value;
onChanged();
return this;
}
/**
*
* decoded account address
*
*
* bytes recipient = 3;
* @return This builder for chaining.
*/
public Builder clearRecipient() {
recipient_ = getDefaultInstance().getRecipient();
onChanged();
return this;
}
private com.google.protobuf.ByteString amount_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* variable-length big integer
*
*
* bytes amount = 4;
* @return The amount.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAmount() {
return amount_;
}
/**
*
* variable-length big integer
*
*
* bytes amount = 4;
* @param value The amount to set.
* @return This builder for chaining.
*/
public Builder setAmount(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
amount_ = value;
onChanged();
return this;
}
/**
*
* variable-length big integer
*
*
* bytes amount = 4;
* @return This builder for chaining.
*/
public Builder clearAmount() {
amount_ = getDefaultInstance().getAmount();
onChanged();
return this;
}
private com.google.protobuf.ByteString payload_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes payload = 5;
* @return The payload.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPayload() {
return payload_;
}
/**
* bytes payload = 5;
* @param value The payload to set.
* @return This builder for chaining.
*/
public Builder setPayload(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
payload_ = value;
onChanged();
return this;
}
/**
* bytes payload = 5;
* @return This builder for chaining.
*/
public Builder clearPayload() {
payload_ = getDefaultInstance().getPayload();
onChanged();
return this;
}
private long gasLimit_ ;
/**
*
* maximum gas used for this transaction. 0 = no limit
*
*
* uint64 gasLimit = 6;
* @return The gasLimit.
*/
@java.lang.Override
public long getGasLimit() {
return gasLimit_;
}
/**
*
* maximum gas used for this transaction. 0 = no limit
*
*
* uint64 gasLimit = 6;
* @param value The gasLimit to set.
* @return This builder for chaining.
*/
public Builder setGasLimit(long value) {
gasLimit_ = value;
onChanged();
return this;
}
/**
*
* maximum gas used for this transaction. 0 = no limit
*
*
* uint64 gasLimit = 6;
* @return This builder for chaining.
*/
public Builder clearGasLimit() {
gasLimit_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString gasPrice_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* variable-length big integer. currently not used
*
*
* bytes gasPrice = 7;
* @return The gasPrice.
*/
@java.lang.Override
public com.google.protobuf.ByteString getGasPrice() {
return gasPrice_;
}
/**
*
* variable-length big integer. currently not used
*
*
* bytes gasPrice = 7;
* @param value The gasPrice to set.
* @return This builder for chaining.
*/
public Builder setGasPrice(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
gasPrice_ = value;
onChanged();
return this;
}
/**
*
* variable-length big integer. currently not used
*
*
* bytes gasPrice = 7;
* @return This builder for chaining.
*/
public Builder clearGasPrice() {
gasPrice_ = getDefaultInstance().getGasPrice();
onChanged();
return this;
}
private int type_ = 0;
/**
* .types.TxType type = 8;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .types.TxType type = 8;
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
* .types.TxType type = 8;
* @return The type.
*/
@java.lang.Override
public types.Blockchain.TxType getType() {
@SuppressWarnings("deprecation")
types.Blockchain.TxType result = types.Blockchain.TxType.valueOf(type_);
return result == null ? types.Blockchain.TxType.UNRECOGNIZED : result;
}
/**
* .types.TxType type = 8;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(types.Blockchain.TxType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
* .types.TxType type = 8;
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString chainIdHash_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* hash value of chain identifier in the block
*
*
* bytes chainIdHash = 9;
* @return The chainIdHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getChainIdHash() {
return chainIdHash_;
}
/**
*
* hash value of chain identifier in the block
*
*
* bytes chainIdHash = 9;
* @param value The chainIdHash to set.
* @return This builder for chaining.
*/
public Builder setChainIdHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
chainIdHash_ = value;
onChanged();
return this;
}
/**
*
* hash value of chain identifier in the block
*
*
* bytes chainIdHash = 9;
* @return This builder for chaining.
*/
public Builder clearChainIdHash() {
chainIdHash_ = getDefaultInstance().getChainIdHash();
onChanged();
return this;
}
private com.google.protobuf.ByteString sign_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* sender's signature for this TxBody
*
*
* bytes sign = 10;
* @return The sign.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSign() {
return sign_;
}
/**
*
* sender's signature for this TxBody
*
*
* bytes sign = 10;
* @param value The sign to set.
* @return This builder for chaining.
*/
public Builder setSign(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
sign_ = value;
onChanged();
return this;
}
/**
*
* sender's signature for this TxBody
*
*
* bytes sign = 10;
* @return This builder for chaining.
*/
public Builder clearSign() {
sign_ = getDefaultInstance().getSign();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.TxBody)
}
// @@protoc_insertion_point(class_scope:types.TxBody)
private static final types.Blockchain.TxBody DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.TxBody();
}
public static types.Blockchain.TxBody getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TxBody parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TxBody(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.TxBody getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TxIdxOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.TxIdx)
com.google.protobuf.MessageOrBuilder {
/**
* bytes blockHash = 1;
* @return The blockHash.
*/
com.google.protobuf.ByteString getBlockHash();
/**
* int32 idx = 2;
* @return The idx.
*/
int getIdx();
}
/**
*
* TxIdx specifies a transaction's block hash and index within the block body
*
*
* Protobuf type {@code types.TxIdx}
*/
public static final class TxIdx extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.TxIdx)
TxIdxOrBuilder {
private static final long serialVersionUID = 0L;
// Use TxIdx.newBuilder() to construct.
private TxIdx(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TxIdx() {
blockHash_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TxIdx();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TxIdx(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
blockHash_ = input.readBytes();
break;
}
case 16: {
idx_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_TxIdx_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_TxIdx_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.TxIdx.class, types.Blockchain.TxIdx.Builder.class);
}
public static final int BLOCKHASH_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString blockHash_;
/**
* bytes blockHash = 1;
* @return The blockHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBlockHash() {
return blockHash_;
}
public static final int IDX_FIELD_NUMBER = 2;
private int idx_;
/**
* int32 idx = 2;
* @return The idx.
*/
@java.lang.Override
public int getIdx() {
return idx_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!blockHash_.isEmpty()) {
output.writeBytes(1, blockHash_);
}
if (idx_ != 0) {
output.writeInt32(2, idx_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!blockHash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, blockHash_);
}
if (idx_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, idx_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.TxIdx)) {
return super.equals(obj);
}
types.Blockchain.TxIdx other = (types.Blockchain.TxIdx) obj;
if (!getBlockHash()
.equals(other.getBlockHash())) return false;
if (getIdx()
!= other.getIdx()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + BLOCKHASH_FIELD_NUMBER;
hash = (53 * hash) + getBlockHash().hashCode();
hash = (37 * hash) + IDX_FIELD_NUMBER;
hash = (53 * hash) + getIdx();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.TxIdx parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.TxIdx parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.TxIdx parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.TxIdx parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.TxIdx parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.TxIdx parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.TxIdx parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.TxIdx parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.TxIdx parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.TxIdx parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.TxIdx parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.TxIdx parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.TxIdx prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* TxIdx specifies a transaction's block hash and index within the block body
*
*
* Protobuf type {@code types.TxIdx}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.TxIdx)
types.Blockchain.TxIdxOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_TxIdx_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_TxIdx_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.TxIdx.class, types.Blockchain.TxIdx.Builder.class);
}
// Construct using types.Blockchain.TxIdx.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
blockHash_ = com.google.protobuf.ByteString.EMPTY;
idx_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_TxIdx_descriptor;
}
@java.lang.Override
public types.Blockchain.TxIdx getDefaultInstanceForType() {
return types.Blockchain.TxIdx.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.TxIdx build() {
types.Blockchain.TxIdx result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.TxIdx buildPartial() {
types.Blockchain.TxIdx result = new types.Blockchain.TxIdx(this);
result.blockHash_ = blockHash_;
result.idx_ = idx_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.TxIdx) {
return mergeFrom((types.Blockchain.TxIdx)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.TxIdx other) {
if (other == types.Blockchain.TxIdx.getDefaultInstance()) return this;
if (other.getBlockHash() != com.google.protobuf.ByteString.EMPTY) {
setBlockHash(other.getBlockHash());
}
if (other.getIdx() != 0) {
setIdx(other.getIdx());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.TxIdx parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.TxIdx) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString blockHash_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes blockHash = 1;
* @return The blockHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBlockHash() {
return blockHash_;
}
/**
* bytes blockHash = 1;
* @param value The blockHash to set.
* @return This builder for chaining.
*/
public Builder setBlockHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
blockHash_ = value;
onChanged();
return this;
}
/**
* bytes blockHash = 1;
* @return This builder for chaining.
*/
public Builder clearBlockHash() {
blockHash_ = getDefaultInstance().getBlockHash();
onChanged();
return this;
}
private int idx_ ;
/**
* int32 idx = 2;
* @return The idx.
*/
@java.lang.Override
public int getIdx() {
return idx_;
}
/**
* int32 idx = 2;
* @param value The idx to set.
* @return This builder for chaining.
*/
public Builder setIdx(int value) {
idx_ = value;
onChanged();
return this;
}
/**
* int32 idx = 2;
* @return This builder for chaining.
*/
public Builder clearIdx() {
idx_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.TxIdx)
}
// @@protoc_insertion_point(class_scope:types.TxIdx)
private static final types.Blockchain.TxIdx DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.TxIdx();
}
public static types.Blockchain.TxIdx getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TxIdx parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TxIdx(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.TxIdx getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TxInBlockOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.TxInBlock)
com.google.protobuf.MessageOrBuilder {
/**
* .types.TxIdx txIdx = 1;
* @return Whether the txIdx field is set.
*/
boolean hasTxIdx();
/**
* .types.TxIdx txIdx = 1;
* @return The txIdx.
*/
types.Blockchain.TxIdx getTxIdx();
/**
* .types.TxIdx txIdx = 1;
*/
types.Blockchain.TxIdxOrBuilder getTxIdxOrBuilder();
/**
* .types.Tx tx = 2;
* @return Whether the tx field is set.
*/
boolean hasTx();
/**
* .types.Tx tx = 2;
* @return The tx.
*/
types.Blockchain.Tx getTx();
/**
* .types.Tx tx = 2;
*/
types.Blockchain.TxOrBuilder getTxOrBuilder();
}
/**
* Protobuf type {@code types.TxInBlock}
*/
public static final class TxInBlock extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.TxInBlock)
TxInBlockOrBuilder {
private static final long serialVersionUID = 0L;
// Use TxInBlock.newBuilder() to construct.
private TxInBlock(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TxInBlock() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TxInBlock();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TxInBlock(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
types.Blockchain.TxIdx.Builder subBuilder = null;
if (txIdx_ != null) {
subBuilder = txIdx_.toBuilder();
}
txIdx_ = input.readMessage(types.Blockchain.TxIdx.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(txIdx_);
txIdx_ = subBuilder.buildPartial();
}
break;
}
case 18: {
types.Blockchain.Tx.Builder subBuilder = null;
if (tx_ != null) {
subBuilder = tx_.toBuilder();
}
tx_ = input.readMessage(types.Blockchain.Tx.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tx_);
tx_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_TxInBlock_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_TxInBlock_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.TxInBlock.class, types.Blockchain.TxInBlock.Builder.class);
}
public static final int TXIDX_FIELD_NUMBER = 1;
private types.Blockchain.TxIdx txIdx_;
/**
* .types.TxIdx txIdx = 1;
* @return Whether the txIdx field is set.
*/
@java.lang.Override
public boolean hasTxIdx() {
return txIdx_ != null;
}
/**
* .types.TxIdx txIdx = 1;
* @return The txIdx.
*/
@java.lang.Override
public types.Blockchain.TxIdx getTxIdx() {
return txIdx_ == null ? types.Blockchain.TxIdx.getDefaultInstance() : txIdx_;
}
/**
* .types.TxIdx txIdx = 1;
*/
@java.lang.Override
public types.Blockchain.TxIdxOrBuilder getTxIdxOrBuilder() {
return getTxIdx();
}
public static final int TX_FIELD_NUMBER = 2;
private types.Blockchain.Tx tx_;
/**
* .types.Tx tx = 2;
* @return Whether the tx field is set.
*/
@java.lang.Override
public boolean hasTx() {
return tx_ != null;
}
/**
* .types.Tx tx = 2;
* @return The tx.
*/
@java.lang.Override
public types.Blockchain.Tx getTx() {
return tx_ == null ? types.Blockchain.Tx.getDefaultInstance() : tx_;
}
/**
* .types.Tx tx = 2;
*/
@java.lang.Override
public types.Blockchain.TxOrBuilder getTxOrBuilder() {
return getTx();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (txIdx_ != null) {
output.writeMessage(1, getTxIdx());
}
if (tx_ != null) {
output.writeMessage(2, getTx());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (txIdx_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getTxIdx());
}
if (tx_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getTx());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.TxInBlock)) {
return super.equals(obj);
}
types.Blockchain.TxInBlock other = (types.Blockchain.TxInBlock) obj;
if (hasTxIdx() != other.hasTxIdx()) return false;
if (hasTxIdx()) {
if (!getTxIdx()
.equals(other.getTxIdx())) return false;
}
if (hasTx() != other.hasTx()) return false;
if (hasTx()) {
if (!getTx()
.equals(other.getTx())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTxIdx()) {
hash = (37 * hash) + TXIDX_FIELD_NUMBER;
hash = (53 * hash) + getTxIdx().hashCode();
}
if (hasTx()) {
hash = (37 * hash) + TX_FIELD_NUMBER;
hash = (53 * hash) + getTx().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.TxInBlock parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.TxInBlock parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.TxInBlock parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.TxInBlock parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.TxInBlock parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.TxInBlock parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.TxInBlock parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.TxInBlock parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.TxInBlock parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.TxInBlock parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.TxInBlock parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.TxInBlock parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.TxInBlock prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.TxInBlock}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.TxInBlock)
types.Blockchain.TxInBlockOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_TxInBlock_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_TxInBlock_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.TxInBlock.class, types.Blockchain.TxInBlock.Builder.class);
}
// Construct using types.Blockchain.TxInBlock.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (txIdxBuilder_ == null) {
txIdx_ = null;
} else {
txIdx_ = null;
txIdxBuilder_ = null;
}
if (txBuilder_ == null) {
tx_ = null;
} else {
tx_ = null;
txBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_TxInBlock_descriptor;
}
@java.lang.Override
public types.Blockchain.TxInBlock getDefaultInstanceForType() {
return types.Blockchain.TxInBlock.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.TxInBlock build() {
types.Blockchain.TxInBlock result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.TxInBlock buildPartial() {
types.Blockchain.TxInBlock result = new types.Blockchain.TxInBlock(this);
if (txIdxBuilder_ == null) {
result.txIdx_ = txIdx_;
} else {
result.txIdx_ = txIdxBuilder_.build();
}
if (txBuilder_ == null) {
result.tx_ = tx_;
} else {
result.tx_ = txBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.TxInBlock) {
return mergeFrom((types.Blockchain.TxInBlock)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.TxInBlock other) {
if (other == types.Blockchain.TxInBlock.getDefaultInstance()) return this;
if (other.hasTxIdx()) {
mergeTxIdx(other.getTxIdx());
}
if (other.hasTx()) {
mergeTx(other.getTx());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.TxInBlock parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.TxInBlock) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private types.Blockchain.TxIdx txIdx_;
private com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.TxIdx, types.Blockchain.TxIdx.Builder, types.Blockchain.TxIdxOrBuilder> txIdxBuilder_;
/**
* .types.TxIdx txIdx = 1;
* @return Whether the txIdx field is set.
*/
public boolean hasTxIdx() {
return txIdxBuilder_ != null || txIdx_ != null;
}
/**
* .types.TxIdx txIdx = 1;
* @return The txIdx.
*/
public types.Blockchain.TxIdx getTxIdx() {
if (txIdxBuilder_ == null) {
return txIdx_ == null ? types.Blockchain.TxIdx.getDefaultInstance() : txIdx_;
} else {
return txIdxBuilder_.getMessage();
}
}
/**
* .types.TxIdx txIdx = 1;
*/
public Builder setTxIdx(types.Blockchain.TxIdx value) {
if (txIdxBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
txIdx_ = value;
onChanged();
} else {
txIdxBuilder_.setMessage(value);
}
return this;
}
/**
* .types.TxIdx txIdx = 1;
*/
public Builder setTxIdx(
types.Blockchain.TxIdx.Builder builderForValue) {
if (txIdxBuilder_ == null) {
txIdx_ = builderForValue.build();
onChanged();
} else {
txIdxBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .types.TxIdx txIdx = 1;
*/
public Builder mergeTxIdx(types.Blockchain.TxIdx value) {
if (txIdxBuilder_ == null) {
if (txIdx_ != null) {
txIdx_ =
types.Blockchain.TxIdx.newBuilder(txIdx_).mergeFrom(value).buildPartial();
} else {
txIdx_ = value;
}
onChanged();
} else {
txIdxBuilder_.mergeFrom(value);
}
return this;
}
/**
* .types.TxIdx txIdx = 1;
*/
public Builder clearTxIdx() {
if (txIdxBuilder_ == null) {
txIdx_ = null;
onChanged();
} else {
txIdx_ = null;
txIdxBuilder_ = null;
}
return this;
}
/**
* .types.TxIdx txIdx = 1;
*/
public types.Blockchain.TxIdx.Builder getTxIdxBuilder() {
onChanged();
return getTxIdxFieldBuilder().getBuilder();
}
/**
* .types.TxIdx txIdx = 1;
*/
public types.Blockchain.TxIdxOrBuilder getTxIdxOrBuilder() {
if (txIdxBuilder_ != null) {
return txIdxBuilder_.getMessageOrBuilder();
} else {
return txIdx_ == null ?
types.Blockchain.TxIdx.getDefaultInstance() : txIdx_;
}
}
/**
* .types.TxIdx txIdx = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.TxIdx, types.Blockchain.TxIdx.Builder, types.Blockchain.TxIdxOrBuilder>
getTxIdxFieldBuilder() {
if (txIdxBuilder_ == null) {
txIdxBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.TxIdx, types.Blockchain.TxIdx.Builder, types.Blockchain.TxIdxOrBuilder>(
getTxIdx(),
getParentForChildren(),
isClean());
txIdx_ = null;
}
return txIdxBuilder_;
}
private types.Blockchain.Tx tx_;
private com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.Tx, types.Blockchain.Tx.Builder, types.Blockchain.TxOrBuilder> txBuilder_;
/**
* .types.Tx tx = 2;
* @return Whether the tx field is set.
*/
public boolean hasTx() {
return txBuilder_ != null || tx_ != null;
}
/**
* .types.Tx tx = 2;
* @return The tx.
*/
public types.Blockchain.Tx getTx() {
if (txBuilder_ == null) {
return tx_ == null ? types.Blockchain.Tx.getDefaultInstance() : tx_;
} else {
return txBuilder_.getMessage();
}
}
/**
* .types.Tx tx = 2;
*/
public Builder setTx(types.Blockchain.Tx value) {
if (txBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tx_ = value;
onChanged();
} else {
txBuilder_.setMessage(value);
}
return this;
}
/**
* .types.Tx tx = 2;
*/
public Builder setTx(
types.Blockchain.Tx.Builder builderForValue) {
if (txBuilder_ == null) {
tx_ = builderForValue.build();
onChanged();
} else {
txBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .types.Tx tx = 2;
*/
public Builder mergeTx(types.Blockchain.Tx value) {
if (txBuilder_ == null) {
if (tx_ != null) {
tx_ =
types.Blockchain.Tx.newBuilder(tx_).mergeFrom(value).buildPartial();
} else {
tx_ = value;
}
onChanged();
} else {
txBuilder_.mergeFrom(value);
}
return this;
}
/**
* .types.Tx tx = 2;
*/
public Builder clearTx() {
if (txBuilder_ == null) {
tx_ = null;
onChanged();
} else {
tx_ = null;
txBuilder_ = null;
}
return this;
}
/**
* .types.Tx tx = 2;
*/
public types.Blockchain.Tx.Builder getTxBuilder() {
onChanged();
return getTxFieldBuilder().getBuilder();
}
/**
* .types.Tx tx = 2;
*/
public types.Blockchain.TxOrBuilder getTxOrBuilder() {
if (txBuilder_ != null) {
return txBuilder_.getMessageOrBuilder();
} else {
return tx_ == null ?
types.Blockchain.Tx.getDefaultInstance() : tx_;
}
}
/**
* .types.Tx tx = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.Tx, types.Blockchain.Tx.Builder, types.Blockchain.TxOrBuilder>
getTxFieldBuilder() {
if (txBuilder_ == null) {
txBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.Tx, types.Blockchain.Tx.Builder, types.Blockchain.TxOrBuilder>(
getTx(),
getParentForChildren(),
isClean());
tx_ = null;
}
return txBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.TxInBlock)
}
// @@protoc_insertion_point(class_scope:types.TxInBlock)
private static final types.Blockchain.TxInBlock DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.TxInBlock();
}
public static types.Blockchain.TxInBlock getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TxInBlock parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TxInBlock(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.TxInBlock getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StateOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.State)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 nonce = 1;
* @return The nonce.
*/
long getNonce();
/**
* bytes balance = 2;
* @return The balance.
*/
com.google.protobuf.ByteString getBalance();
/**
* bytes codeHash = 3;
* @return The codeHash.
*/
com.google.protobuf.ByteString getCodeHash();
/**
* bytes storageRoot = 4;
* @return The storageRoot.
*/
com.google.protobuf.ByteString getStorageRoot();
/**
* uint64 sqlRecoveryPoint = 5;
* @return The sqlRecoveryPoint.
*/
long getSqlRecoveryPoint();
}
/**
* Protobuf type {@code types.State}
*/
public static final class State extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.State)
StateOrBuilder {
private static final long serialVersionUID = 0L;
// Use State.newBuilder() to construct.
private State(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private State() {
balance_ = com.google.protobuf.ByteString.EMPTY;
codeHash_ = com.google.protobuf.ByteString.EMPTY;
storageRoot_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new State();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private State(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
nonce_ = input.readUInt64();
break;
}
case 18: {
balance_ = input.readBytes();
break;
}
case 26: {
codeHash_ = input.readBytes();
break;
}
case 34: {
storageRoot_ = input.readBytes();
break;
}
case 40: {
sqlRecoveryPoint_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_State_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_State_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.State.class, types.Blockchain.State.Builder.class);
}
public static final int NONCE_FIELD_NUMBER = 1;
private long nonce_;
/**
* uint64 nonce = 1;
* @return The nonce.
*/
@java.lang.Override
public long getNonce() {
return nonce_;
}
public static final int BALANCE_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString balance_;
/**
* bytes balance = 2;
* @return The balance.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBalance() {
return balance_;
}
public static final int CODEHASH_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString codeHash_;
/**
* bytes codeHash = 3;
* @return The codeHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCodeHash() {
return codeHash_;
}
public static final int STORAGEROOT_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString storageRoot_;
/**
* bytes storageRoot = 4;
* @return The storageRoot.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStorageRoot() {
return storageRoot_;
}
public static final int SQLRECOVERYPOINT_FIELD_NUMBER = 5;
private long sqlRecoveryPoint_;
/**
* uint64 sqlRecoveryPoint = 5;
* @return The sqlRecoveryPoint.
*/
@java.lang.Override
public long getSqlRecoveryPoint() {
return sqlRecoveryPoint_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (nonce_ != 0L) {
output.writeUInt64(1, nonce_);
}
if (!balance_.isEmpty()) {
output.writeBytes(2, balance_);
}
if (!codeHash_.isEmpty()) {
output.writeBytes(3, codeHash_);
}
if (!storageRoot_.isEmpty()) {
output.writeBytes(4, storageRoot_);
}
if (sqlRecoveryPoint_ != 0L) {
output.writeUInt64(5, sqlRecoveryPoint_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (nonce_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, nonce_);
}
if (!balance_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, balance_);
}
if (!codeHash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, codeHash_);
}
if (!storageRoot_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, storageRoot_);
}
if (sqlRecoveryPoint_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(5, sqlRecoveryPoint_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.State)) {
return super.equals(obj);
}
types.Blockchain.State other = (types.Blockchain.State) obj;
if (getNonce()
!= other.getNonce()) return false;
if (!getBalance()
.equals(other.getBalance())) return false;
if (!getCodeHash()
.equals(other.getCodeHash())) return false;
if (!getStorageRoot()
.equals(other.getStorageRoot())) return false;
if (getSqlRecoveryPoint()
!= other.getSqlRecoveryPoint()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NONCE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNonce());
hash = (37 * hash) + BALANCE_FIELD_NUMBER;
hash = (53 * hash) + getBalance().hashCode();
hash = (37 * hash) + CODEHASH_FIELD_NUMBER;
hash = (53 * hash) + getCodeHash().hashCode();
hash = (37 * hash) + STORAGEROOT_FIELD_NUMBER;
hash = (53 * hash) + getStorageRoot().hashCode();
hash = (37 * hash) + SQLRECOVERYPOINT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSqlRecoveryPoint());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.State parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.State parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.State parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.State parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.State parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.State parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.State parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.State parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.State parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.State parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.State parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.State parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.State prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.State}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.State)
types.Blockchain.StateOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_State_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_State_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.State.class, types.Blockchain.State.Builder.class);
}
// Construct using types.Blockchain.State.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
nonce_ = 0L;
balance_ = com.google.protobuf.ByteString.EMPTY;
codeHash_ = com.google.protobuf.ByteString.EMPTY;
storageRoot_ = com.google.protobuf.ByteString.EMPTY;
sqlRecoveryPoint_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_State_descriptor;
}
@java.lang.Override
public types.Blockchain.State getDefaultInstanceForType() {
return types.Blockchain.State.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.State build() {
types.Blockchain.State result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.State buildPartial() {
types.Blockchain.State result = new types.Blockchain.State(this);
result.nonce_ = nonce_;
result.balance_ = balance_;
result.codeHash_ = codeHash_;
result.storageRoot_ = storageRoot_;
result.sqlRecoveryPoint_ = sqlRecoveryPoint_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.State) {
return mergeFrom((types.Blockchain.State)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.State other) {
if (other == types.Blockchain.State.getDefaultInstance()) return this;
if (other.getNonce() != 0L) {
setNonce(other.getNonce());
}
if (other.getBalance() != com.google.protobuf.ByteString.EMPTY) {
setBalance(other.getBalance());
}
if (other.getCodeHash() != com.google.protobuf.ByteString.EMPTY) {
setCodeHash(other.getCodeHash());
}
if (other.getStorageRoot() != com.google.protobuf.ByteString.EMPTY) {
setStorageRoot(other.getStorageRoot());
}
if (other.getSqlRecoveryPoint() != 0L) {
setSqlRecoveryPoint(other.getSqlRecoveryPoint());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.State parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.State) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long nonce_ ;
/**
* uint64 nonce = 1;
* @return The nonce.
*/
@java.lang.Override
public long getNonce() {
return nonce_;
}
/**
* uint64 nonce = 1;
* @param value The nonce to set.
* @return This builder for chaining.
*/
public Builder setNonce(long value) {
nonce_ = value;
onChanged();
return this;
}
/**
* uint64 nonce = 1;
* @return This builder for chaining.
*/
public Builder clearNonce() {
nonce_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString balance_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes balance = 2;
* @return The balance.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBalance() {
return balance_;
}
/**
* bytes balance = 2;
* @param value The balance to set.
* @return This builder for chaining.
*/
public Builder setBalance(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
balance_ = value;
onChanged();
return this;
}
/**
* bytes balance = 2;
* @return This builder for chaining.
*/
public Builder clearBalance() {
balance_ = getDefaultInstance().getBalance();
onChanged();
return this;
}
private com.google.protobuf.ByteString codeHash_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes codeHash = 3;
* @return The codeHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCodeHash() {
return codeHash_;
}
/**
* bytes codeHash = 3;
* @param value The codeHash to set.
* @return This builder for chaining.
*/
public Builder setCodeHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
codeHash_ = value;
onChanged();
return this;
}
/**
* bytes codeHash = 3;
* @return This builder for chaining.
*/
public Builder clearCodeHash() {
codeHash_ = getDefaultInstance().getCodeHash();
onChanged();
return this;
}
private com.google.protobuf.ByteString storageRoot_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes storageRoot = 4;
* @return The storageRoot.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStorageRoot() {
return storageRoot_;
}
/**
* bytes storageRoot = 4;
* @param value The storageRoot to set.
* @return This builder for chaining.
*/
public Builder setStorageRoot(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
storageRoot_ = value;
onChanged();
return this;
}
/**
* bytes storageRoot = 4;
* @return This builder for chaining.
*/
public Builder clearStorageRoot() {
storageRoot_ = getDefaultInstance().getStorageRoot();
onChanged();
return this;
}
private long sqlRecoveryPoint_ ;
/**
* uint64 sqlRecoveryPoint = 5;
* @return The sqlRecoveryPoint.
*/
@java.lang.Override
public long getSqlRecoveryPoint() {
return sqlRecoveryPoint_;
}
/**
* uint64 sqlRecoveryPoint = 5;
* @param value The sqlRecoveryPoint to set.
* @return This builder for chaining.
*/
public Builder setSqlRecoveryPoint(long value) {
sqlRecoveryPoint_ = value;
onChanged();
return this;
}
/**
* uint64 sqlRecoveryPoint = 5;
* @return This builder for chaining.
*/
public Builder clearSqlRecoveryPoint() {
sqlRecoveryPoint_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.State)
}
// @@protoc_insertion_point(class_scope:types.State)
private static final types.Blockchain.State DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.State();
}
public static types.Blockchain.State getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public State parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new State(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.State getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AccountProofOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.AccountProof)
com.google.protobuf.MessageOrBuilder {
/**
* .types.State state = 1;
* @return Whether the state field is set.
*/
boolean hasState();
/**
* .types.State state = 1;
* @return The state.
*/
types.Blockchain.State getState();
/**
* .types.State state = 1;
*/
types.Blockchain.StateOrBuilder getStateOrBuilder();
/**
* bool inclusion = 2;
* @return The inclusion.
*/
boolean getInclusion();
/**
* bytes key = 3;
* @return The key.
*/
com.google.protobuf.ByteString getKey();
/**
* bytes proofKey = 4;
* @return The proofKey.
*/
com.google.protobuf.ByteString getProofKey();
/**
* bytes proofVal = 5;
* @return The proofVal.
*/
com.google.protobuf.ByteString getProofVal();
/**
* bytes bitmap = 6;
* @return The bitmap.
*/
com.google.protobuf.ByteString getBitmap();
/**
* uint32 height = 7;
* @return The height.
*/
int getHeight();
/**
* repeated bytes auditPath = 8;
* @return A list containing the auditPath.
*/
java.util.List getAuditPathList();
/**
* repeated bytes auditPath = 8;
* @return The count of auditPath.
*/
int getAuditPathCount();
/**
* repeated bytes auditPath = 8;
* @param index The index of the element to return.
* @return The auditPath at the given index.
*/
com.google.protobuf.ByteString getAuditPath(int index);
}
/**
* Protobuf type {@code types.AccountProof}
*/
public static final class AccountProof extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.AccountProof)
AccountProofOrBuilder {
private static final long serialVersionUID = 0L;
// Use AccountProof.newBuilder() to construct.
private AccountProof(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AccountProof() {
key_ = com.google.protobuf.ByteString.EMPTY;
proofKey_ = com.google.protobuf.ByteString.EMPTY;
proofVal_ = com.google.protobuf.ByteString.EMPTY;
bitmap_ = com.google.protobuf.ByteString.EMPTY;
auditPath_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AccountProof();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AccountProof(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
types.Blockchain.State.Builder subBuilder = null;
if (state_ != null) {
subBuilder = state_.toBuilder();
}
state_ = input.readMessage(types.Blockchain.State.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(state_);
state_ = subBuilder.buildPartial();
}
break;
}
case 16: {
inclusion_ = input.readBool();
break;
}
case 26: {
key_ = input.readBytes();
break;
}
case 34: {
proofKey_ = input.readBytes();
break;
}
case 42: {
proofVal_ = input.readBytes();
break;
}
case 50: {
bitmap_ = input.readBytes();
break;
}
case 56: {
height_ = input.readUInt32();
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
auditPath_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
auditPath_.add(input.readBytes());
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
auditPath_ = java.util.Collections.unmodifiableList(auditPath_); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_AccountProof_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_AccountProof_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.AccountProof.class, types.Blockchain.AccountProof.Builder.class);
}
public static final int STATE_FIELD_NUMBER = 1;
private types.Blockchain.State state_;
/**
* .types.State state = 1;
* @return Whether the state field is set.
*/
@java.lang.Override
public boolean hasState() {
return state_ != null;
}
/**
* .types.State state = 1;
* @return The state.
*/
@java.lang.Override
public types.Blockchain.State getState() {
return state_ == null ? types.Blockchain.State.getDefaultInstance() : state_;
}
/**
* .types.State state = 1;
*/
@java.lang.Override
public types.Blockchain.StateOrBuilder getStateOrBuilder() {
return getState();
}
public static final int INCLUSION_FIELD_NUMBER = 2;
private boolean inclusion_;
/**
* bool inclusion = 2;
* @return The inclusion.
*/
@java.lang.Override
public boolean getInclusion() {
return inclusion_;
}
public static final int KEY_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString key_;
/**
* bytes key = 3;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int PROOFKEY_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString proofKey_;
/**
* bytes proofKey = 4;
* @return The proofKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getProofKey() {
return proofKey_;
}
public static final int PROOFVAL_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString proofVal_;
/**
* bytes proofVal = 5;
* @return The proofVal.
*/
@java.lang.Override
public com.google.protobuf.ByteString getProofVal() {
return proofVal_;
}
public static final int BITMAP_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString bitmap_;
/**
* bytes bitmap = 6;
* @return The bitmap.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBitmap() {
return bitmap_;
}
public static final int HEIGHT_FIELD_NUMBER = 7;
private int height_;
/**
* uint32 height = 7;
* @return The height.
*/
@java.lang.Override
public int getHeight() {
return height_;
}
public static final int AUDITPATH_FIELD_NUMBER = 8;
private java.util.List auditPath_;
/**
* repeated bytes auditPath = 8;
* @return A list containing the auditPath.
*/
@java.lang.Override
public java.util.List
getAuditPathList() {
return auditPath_;
}
/**
* repeated bytes auditPath = 8;
* @return The count of auditPath.
*/
public int getAuditPathCount() {
return auditPath_.size();
}
/**
* repeated bytes auditPath = 8;
* @param index The index of the element to return.
* @return The auditPath at the given index.
*/
public com.google.protobuf.ByteString getAuditPath(int index) {
return auditPath_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (state_ != null) {
output.writeMessage(1, getState());
}
if (inclusion_ != false) {
output.writeBool(2, inclusion_);
}
if (!key_.isEmpty()) {
output.writeBytes(3, key_);
}
if (!proofKey_.isEmpty()) {
output.writeBytes(4, proofKey_);
}
if (!proofVal_.isEmpty()) {
output.writeBytes(5, proofVal_);
}
if (!bitmap_.isEmpty()) {
output.writeBytes(6, bitmap_);
}
if (height_ != 0) {
output.writeUInt32(7, height_);
}
for (int i = 0; i < auditPath_.size(); i++) {
output.writeBytes(8, auditPath_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (state_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getState());
}
if (inclusion_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, inclusion_);
}
if (!key_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, key_);
}
if (!proofKey_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, proofKey_);
}
if (!proofVal_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, proofVal_);
}
if (!bitmap_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, bitmap_);
}
if (height_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(7, height_);
}
{
int dataSize = 0;
for (int i = 0; i < auditPath_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(auditPath_.get(i));
}
size += dataSize;
size += 1 * getAuditPathList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.AccountProof)) {
return super.equals(obj);
}
types.Blockchain.AccountProof other = (types.Blockchain.AccountProof) obj;
if (hasState() != other.hasState()) return false;
if (hasState()) {
if (!getState()
.equals(other.getState())) return false;
}
if (getInclusion()
!= other.getInclusion()) return false;
if (!getKey()
.equals(other.getKey())) return false;
if (!getProofKey()
.equals(other.getProofKey())) return false;
if (!getProofVal()
.equals(other.getProofVal())) return false;
if (!getBitmap()
.equals(other.getBitmap())) return false;
if (getHeight()
!= other.getHeight()) return false;
if (!getAuditPathList()
.equals(other.getAuditPathList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasState()) {
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + getState().hashCode();
}
hash = (37 * hash) + INCLUSION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getInclusion());
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
hash = (37 * hash) + PROOFKEY_FIELD_NUMBER;
hash = (53 * hash) + getProofKey().hashCode();
hash = (37 * hash) + PROOFVAL_FIELD_NUMBER;
hash = (53 * hash) + getProofVal().hashCode();
hash = (37 * hash) + BITMAP_FIELD_NUMBER;
hash = (53 * hash) + getBitmap().hashCode();
hash = (37 * hash) + HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getHeight();
if (getAuditPathCount() > 0) {
hash = (37 * hash) + AUDITPATH_FIELD_NUMBER;
hash = (53 * hash) + getAuditPathList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.AccountProof parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.AccountProof parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.AccountProof parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.AccountProof parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.AccountProof parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.AccountProof parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.AccountProof parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.AccountProof parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.AccountProof parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.AccountProof parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.AccountProof parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.AccountProof parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.AccountProof prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.AccountProof}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.AccountProof)
types.Blockchain.AccountProofOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_AccountProof_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_AccountProof_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.AccountProof.class, types.Blockchain.AccountProof.Builder.class);
}
// Construct using types.Blockchain.AccountProof.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (stateBuilder_ == null) {
state_ = null;
} else {
state_ = null;
stateBuilder_ = null;
}
inclusion_ = false;
key_ = com.google.protobuf.ByteString.EMPTY;
proofKey_ = com.google.protobuf.ByteString.EMPTY;
proofVal_ = com.google.protobuf.ByteString.EMPTY;
bitmap_ = com.google.protobuf.ByteString.EMPTY;
height_ = 0;
auditPath_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_AccountProof_descriptor;
}
@java.lang.Override
public types.Blockchain.AccountProof getDefaultInstanceForType() {
return types.Blockchain.AccountProof.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.AccountProof build() {
types.Blockchain.AccountProof result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.AccountProof buildPartial() {
types.Blockchain.AccountProof result = new types.Blockchain.AccountProof(this);
int from_bitField0_ = bitField0_;
if (stateBuilder_ == null) {
result.state_ = state_;
} else {
result.state_ = stateBuilder_.build();
}
result.inclusion_ = inclusion_;
result.key_ = key_;
result.proofKey_ = proofKey_;
result.proofVal_ = proofVal_;
result.bitmap_ = bitmap_;
result.height_ = height_;
if (((bitField0_ & 0x00000001) != 0)) {
auditPath_ = java.util.Collections.unmodifiableList(auditPath_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.auditPath_ = auditPath_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.AccountProof) {
return mergeFrom((types.Blockchain.AccountProof)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.AccountProof other) {
if (other == types.Blockchain.AccountProof.getDefaultInstance()) return this;
if (other.hasState()) {
mergeState(other.getState());
}
if (other.getInclusion() != false) {
setInclusion(other.getInclusion());
}
if (other.getKey() != com.google.protobuf.ByteString.EMPTY) {
setKey(other.getKey());
}
if (other.getProofKey() != com.google.protobuf.ByteString.EMPTY) {
setProofKey(other.getProofKey());
}
if (other.getProofVal() != com.google.protobuf.ByteString.EMPTY) {
setProofVal(other.getProofVal());
}
if (other.getBitmap() != com.google.protobuf.ByteString.EMPTY) {
setBitmap(other.getBitmap());
}
if (other.getHeight() != 0) {
setHeight(other.getHeight());
}
if (!other.auditPath_.isEmpty()) {
if (auditPath_.isEmpty()) {
auditPath_ = other.auditPath_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureAuditPathIsMutable();
auditPath_.addAll(other.auditPath_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.AccountProof parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.AccountProof) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private types.Blockchain.State state_;
private com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.State, types.Blockchain.State.Builder, types.Blockchain.StateOrBuilder> stateBuilder_;
/**
* .types.State state = 1;
* @return Whether the state field is set.
*/
public boolean hasState() {
return stateBuilder_ != null || state_ != null;
}
/**
* .types.State state = 1;
* @return The state.
*/
public types.Blockchain.State getState() {
if (stateBuilder_ == null) {
return state_ == null ? types.Blockchain.State.getDefaultInstance() : state_;
} else {
return stateBuilder_.getMessage();
}
}
/**
* .types.State state = 1;
*/
public Builder setState(types.Blockchain.State value) {
if (stateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
state_ = value;
onChanged();
} else {
stateBuilder_.setMessage(value);
}
return this;
}
/**
* .types.State state = 1;
*/
public Builder setState(
types.Blockchain.State.Builder builderForValue) {
if (stateBuilder_ == null) {
state_ = builderForValue.build();
onChanged();
} else {
stateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .types.State state = 1;
*/
public Builder mergeState(types.Blockchain.State value) {
if (stateBuilder_ == null) {
if (state_ != null) {
state_ =
types.Blockchain.State.newBuilder(state_).mergeFrom(value).buildPartial();
} else {
state_ = value;
}
onChanged();
} else {
stateBuilder_.mergeFrom(value);
}
return this;
}
/**
* .types.State state = 1;
*/
public Builder clearState() {
if (stateBuilder_ == null) {
state_ = null;
onChanged();
} else {
state_ = null;
stateBuilder_ = null;
}
return this;
}
/**
* .types.State state = 1;
*/
public types.Blockchain.State.Builder getStateBuilder() {
onChanged();
return getStateFieldBuilder().getBuilder();
}
/**
* .types.State state = 1;
*/
public types.Blockchain.StateOrBuilder getStateOrBuilder() {
if (stateBuilder_ != null) {
return stateBuilder_.getMessageOrBuilder();
} else {
return state_ == null ?
types.Blockchain.State.getDefaultInstance() : state_;
}
}
/**
* .types.State state = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.State, types.Blockchain.State.Builder, types.Blockchain.StateOrBuilder>
getStateFieldBuilder() {
if (stateBuilder_ == null) {
stateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.State, types.Blockchain.State.Builder, types.Blockchain.StateOrBuilder>(
getState(),
getParentForChildren(),
isClean());
state_ = null;
}
return stateBuilder_;
}
private boolean inclusion_ ;
/**
* bool inclusion = 2;
* @return The inclusion.
*/
@java.lang.Override
public boolean getInclusion() {
return inclusion_;
}
/**
* bool inclusion = 2;
* @param value The inclusion to set.
* @return This builder for chaining.
*/
public Builder setInclusion(boolean value) {
inclusion_ = value;
onChanged();
return this;
}
/**
* bool inclusion = 2;
* @return This builder for chaining.
*/
public Builder clearInclusion() {
inclusion_ = false;
onChanged();
return this;
}
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes key = 3;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
* bytes key = 3;
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
return this;
}
/**
* bytes key = 3;
* @return This builder for chaining.
*/
public Builder clearKey() {
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString proofKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes proofKey = 4;
* @return The proofKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getProofKey() {
return proofKey_;
}
/**
* bytes proofKey = 4;
* @param value The proofKey to set.
* @return This builder for chaining.
*/
public Builder setProofKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proofKey_ = value;
onChanged();
return this;
}
/**
* bytes proofKey = 4;
* @return This builder for chaining.
*/
public Builder clearProofKey() {
proofKey_ = getDefaultInstance().getProofKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString proofVal_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes proofVal = 5;
* @return The proofVal.
*/
@java.lang.Override
public com.google.protobuf.ByteString getProofVal() {
return proofVal_;
}
/**
* bytes proofVal = 5;
* @param value The proofVal to set.
* @return This builder for chaining.
*/
public Builder setProofVal(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proofVal_ = value;
onChanged();
return this;
}
/**
* bytes proofVal = 5;
* @return This builder for chaining.
*/
public Builder clearProofVal() {
proofVal_ = getDefaultInstance().getProofVal();
onChanged();
return this;
}
private com.google.protobuf.ByteString bitmap_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes bitmap = 6;
* @return The bitmap.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBitmap() {
return bitmap_;
}
/**
* bytes bitmap = 6;
* @param value The bitmap to set.
* @return This builder for chaining.
*/
public Builder setBitmap(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitmap_ = value;
onChanged();
return this;
}
/**
* bytes bitmap = 6;
* @return This builder for chaining.
*/
public Builder clearBitmap() {
bitmap_ = getDefaultInstance().getBitmap();
onChanged();
return this;
}
private int height_ ;
/**
* uint32 height = 7;
* @return The height.
*/
@java.lang.Override
public int getHeight() {
return height_;
}
/**
* uint32 height = 7;
* @param value The height to set.
* @return This builder for chaining.
*/
public Builder setHeight(int value) {
height_ = value;
onChanged();
return this;
}
/**
* uint32 height = 7;
* @return This builder for chaining.
*/
public Builder clearHeight() {
height_ = 0;
onChanged();
return this;
}
private java.util.List auditPath_ = java.util.Collections.emptyList();
private void ensureAuditPathIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
auditPath_ = new java.util.ArrayList(auditPath_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated bytes auditPath = 8;
* @return A list containing the auditPath.
*/
public java.util.List
getAuditPathList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(auditPath_) : auditPath_;
}
/**
* repeated bytes auditPath = 8;
* @return The count of auditPath.
*/
public int getAuditPathCount() {
return auditPath_.size();
}
/**
* repeated bytes auditPath = 8;
* @param index The index of the element to return.
* @return The auditPath at the given index.
*/
public com.google.protobuf.ByteString getAuditPath(int index) {
return auditPath_.get(index);
}
/**
* repeated bytes auditPath = 8;
* @param index The index to set the value at.
* @param value The auditPath to set.
* @return This builder for chaining.
*/
public Builder setAuditPath(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureAuditPathIsMutable();
auditPath_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes auditPath = 8;
* @param value The auditPath to add.
* @return This builder for chaining.
*/
public Builder addAuditPath(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureAuditPathIsMutable();
auditPath_.add(value);
onChanged();
return this;
}
/**
* repeated bytes auditPath = 8;
* @param values The auditPath to add.
* @return This builder for chaining.
*/
public Builder addAllAuditPath(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureAuditPathIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, auditPath_);
onChanged();
return this;
}
/**
* repeated bytes auditPath = 8;
* @return This builder for chaining.
*/
public Builder clearAuditPath() {
auditPath_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.AccountProof)
}
// @@protoc_insertion_point(class_scope:types.AccountProof)
private static final types.Blockchain.AccountProof DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.AccountProof();
}
public static types.Blockchain.AccountProof getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AccountProof parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AccountProof(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.AccountProof getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ContractVarProofOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.ContractVarProof)
com.google.protobuf.MessageOrBuilder {
/**
* bytes value = 1;
* @return The value.
*/
com.google.protobuf.ByteString getValue();
/**
* bool inclusion = 2;
* @return The inclusion.
*/
boolean getInclusion();
/**
* bytes proofKey = 4;
* @return The proofKey.
*/
com.google.protobuf.ByteString getProofKey();
/**
* bytes proofVal = 5;
* @return The proofVal.
*/
com.google.protobuf.ByteString getProofVal();
/**
* bytes bitmap = 6;
* @return The bitmap.
*/
com.google.protobuf.ByteString getBitmap();
/**
* uint32 height = 7;
* @return The height.
*/
int getHeight();
/**
* repeated bytes auditPath = 8;
* @return A list containing the auditPath.
*/
java.util.List getAuditPathList();
/**
* repeated bytes auditPath = 8;
* @return The count of auditPath.
*/
int getAuditPathCount();
/**
* repeated bytes auditPath = 8;
* @param index The index of the element to return.
* @return The auditPath at the given index.
*/
com.google.protobuf.ByteString getAuditPath(int index);
/**
* bytes key = 9;
* @return The key.
*/
com.google.protobuf.ByteString getKey();
}
/**
* Protobuf type {@code types.ContractVarProof}
*/
public static final class ContractVarProof extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.ContractVarProof)
ContractVarProofOrBuilder {
private static final long serialVersionUID = 0L;
// Use ContractVarProof.newBuilder() to construct.
private ContractVarProof(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ContractVarProof() {
value_ = com.google.protobuf.ByteString.EMPTY;
proofKey_ = com.google.protobuf.ByteString.EMPTY;
proofVal_ = com.google.protobuf.ByteString.EMPTY;
bitmap_ = com.google.protobuf.ByteString.EMPTY;
auditPath_ = java.util.Collections.emptyList();
key_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ContractVarProof();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ContractVarProof(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
value_ = input.readBytes();
break;
}
case 16: {
inclusion_ = input.readBool();
break;
}
case 34: {
proofKey_ = input.readBytes();
break;
}
case 42: {
proofVal_ = input.readBytes();
break;
}
case 50: {
bitmap_ = input.readBytes();
break;
}
case 56: {
height_ = input.readUInt32();
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
auditPath_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
auditPath_.add(input.readBytes());
break;
}
case 74: {
key_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
auditPath_ = java.util.Collections.unmodifiableList(auditPath_); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_ContractVarProof_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_ContractVarProof_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.ContractVarProof.class, types.Blockchain.ContractVarProof.Builder.class);
}
public static final int VALUE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString value_;
/**
* bytes value = 1;
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
public static final int INCLUSION_FIELD_NUMBER = 2;
private boolean inclusion_;
/**
* bool inclusion = 2;
* @return The inclusion.
*/
@java.lang.Override
public boolean getInclusion() {
return inclusion_;
}
public static final int PROOFKEY_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString proofKey_;
/**
* bytes proofKey = 4;
* @return The proofKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getProofKey() {
return proofKey_;
}
public static final int PROOFVAL_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString proofVal_;
/**
* bytes proofVal = 5;
* @return The proofVal.
*/
@java.lang.Override
public com.google.protobuf.ByteString getProofVal() {
return proofVal_;
}
public static final int BITMAP_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString bitmap_;
/**
* bytes bitmap = 6;
* @return The bitmap.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBitmap() {
return bitmap_;
}
public static final int HEIGHT_FIELD_NUMBER = 7;
private int height_;
/**
* uint32 height = 7;
* @return The height.
*/
@java.lang.Override
public int getHeight() {
return height_;
}
public static final int AUDITPATH_FIELD_NUMBER = 8;
private java.util.List auditPath_;
/**
* repeated bytes auditPath = 8;
* @return A list containing the auditPath.
*/
@java.lang.Override
public java.util.List
getAuditPathList() {
return auditPath_;
}
/**
* repeated bytes auditPath = 8;
* @return The count of auditPath.
*/
public int getAuditPathCount() {
return auditPath_.size();
}
/**
* repeated bytes auditPath = 8;
* @param index The index of the element to return.
* @return The auditPath at the given index.
*/
public com.google.protobuf.ByteString getAuditPath(int index) {
return auditPath_.get(index);
}
public static final int KEY_FIELD_NUMBER = 9;
private com.google.protobuf.ByteString key_;
/**
* bytes key = 9;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!value_.isEmpty()) {
output.writeBytes(1, value_);
}
if (inclusion_ != false) {
output.writeBool(2, inclusion_);
}
if (!proofKey_.isEmpty()) {
output.writeBytes(4, proofKey_);
}
if (!proofVal_.isEmpty()) {
output.writeBytes(5, proofVal_);
}
if (!bitmap_.isEmpty()) {
output.writeBytes(6, bitmap_);
}
if (height_ != 0) {
output.writeUInt32(7, height_);
}
for (int i = 0; i < auditPath_.size(); i++) {
output.writeBytes(8, auditPath_.get(i));
}
if (!key_.isEmpty()) {
output.writeBytes(9, key_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!value_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, value_);
}
if (inclusion_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, inclusion_);
}
if (!proofKey_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, proofKey_);
}
if (!proofVal_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, proofVal_);
}
if (!bitmap_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, bitmap_);
}
if (height_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(7, height_);
}
{
int dataSize = 0;
for (int i = 0; i < auditPath_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(auditPath_.get(i));
}
size += dataSize;
size += 1 * getAuditPathList().size();
}
if (!key_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(9, key_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.ContractVarProof)) {
return super.equals(obj);
}
types.Blockchain.ContractVarProof other = (types.Blockchain.ContractVarProof) obj;
if (!getValue()
.equals(other.getValue())) return false;
if (getInclusion()
!= other.getInclusion()) return false;
if (!getProofKey()
.equals(other.getProofKey())) return false;
if (!getProofVal()
.equals(other.getProofVal())) return false;
if (!getBitmap()
.equals(other.getBitmap())) return false;
if (getHeight()
!= other.getHeight()) return false;
if (!getAuditPathList()
.equals(other.getAuditPathList())) return false;
if (!getKey()
.equals(other.getKey())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
hash = (37 * hash) + INCLUSION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getInclusion());
hash = (37 * hash) + PROOFKEY_FIELD_NUMBER;
hash = (53 * hash) + getProofKey().hashCode();
hash = (37 * hash) + PROOFVAL_FIELD_NUMBER;
hash = (53 * hash) + getProofVal().hashCode();
hash = (37 * hash) + BITMAP_FIELD_NUMBER;
hash = (53 * hash) + getBitmap().hashCode();
hash = (37 * hash) + HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getHeight();
if (getAuditPathCount() > 0) {
hash = (37 * hash) + AUDITPATH_FIELD_NUMBER;
hash = (53 * hash) + getAuditPathList().hashCode();
}
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.ContractVarProof parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.ContractVarProof parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.ContractVarProof parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.ContractVarProof parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.ContractVarProof parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.ContractVarProof parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.ContractVarProof parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.ContractVarProof parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.ContractVarProof parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.ContractVarProof parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.ContractVarProof parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.ContractVarProof parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.ContractVarProof prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.ContractVarProof}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.ContractVarProof)
types.Blockchain.ContractVarProofOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_ContractVarProof_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_ContractVarProof_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.ContractVarProof.class, types.Blockchain.ContractVarProof.Builder.class);
}
// Construct using types.Blockchain.ContractVarProof.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
value_ = com.google.protobuf.ByteString.EMPTY;
inclusion_ = false;
proofKey_ = com.google.protobuf.ByteString.EMPTY;
proofVal_ = com.google.protobuf.ByteString.EMPTY;
bitmap_ = com.google.protobuf.ByteString.EMPTY;
height_ = 0;
auditPath_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
key_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_ContractVarProof_descriptor;
}
@java.lang.Override
public types.Blockchain.ContractVarProof getDefaultInstanceForType() {
return types.Blockchain.ContractVarProof.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.ContractVarProof build() {
types.Blockchain.ContractVarProof result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.ContractVarProof buildPartial() {
types.Blockchain.ContractVarProof result = new types.Blockchain.ContractVarProof(this);
int from_bitField0_ = bitField0_;
result.value_ = value_;
result.inclusion_ = inclusion_;
result.proofKey_ = proofKey_;
result.proofVal_ = proofVal_;
result.bitmap_ = bitmap_;
result.height_ = height_;
if (((bitField0_ & 0x00000001) != 0)) {
auditPath_ = java.util.Collections.unmodifiableList(auditPath_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.auditPath_ = auditPath_;
result.key_ = key_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.ContractVarProof) {
return mergeFrom((types.Blockchain.ContractVarProof)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.ContractVarProof other) {
if (other == types.Blockchain.ContractVarProof.getDefaultInstance()) return this;
if (other.getValue() != com.google.protobuf.ByteString.EMPTY) {
setValue(other.getValue());
}
if (other.getInclusion() != false) {
setInclusion(other.getInclusion());
}
if (other.getProofKey() != com.google.protobuf.ByteString.EMPTY) {
setProofKey(other.getProofKey());
}
if (other.getProofVal() != com.google.protobuf.ByteString.EMPTY) {
setProofVal(other.getProofVal());
}
if (other.getBitmap() != com.google.protobuf.ByteString.EMPTY) {
setBitmap(other.getBitmap());
}
if (other.getHeight() != 0) {
setHeight(other.getHeight());
}
if (!other.auditPath_.isEmpty()) {
if (auditPath_.isEmpty()) {
auditPath_ = other.auditPath_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureAuditPathIsMutable();
auditPath_.addAll(other.auditPath_);
}
onChanged();
}
if (other.getKey() != com.google.protobuf.ByteString.EMPTY) {
setKey(other.getKey());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.ContractVarProof parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.ContractVarProof) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes value = 1;
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
/**
* bytes value = 1;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
return this;
}
/**
* bytes value = 1;
* @return This builder for chaining.
*/
public Builder clearValue() {
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
private boolean inclusion_ ;
/**
* bool inclusion = 2;
* @return The inclusion.
*/
@java.lang.Override
public boolean getInclusion() {
return inclusion_;
}
/**
* bool inclusion = 2;
* @param value The inclusion to set.
* @return This builder for chaining.
*/
public Builder setInclusion(boolean value) {
inclusion_ = value;
onChanged();
return this;
}
/**
* bool inclusion = 2;
* @return This builder for chaining.
*/
public Builder clearInclusion() {
inclusion_ = false;
onChanged();
return this;
}
private com.google.protobuf.ByteString proofKey_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes proofKey = 4;
* @return The proofKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getProofKey() {
return proofKey_;
}
/**
* bytes proofKey = 4;
* @param value The proofKey to set.
* @return This builder for chaining.
*/
public Builder setProofKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proofKey_ = value;
onChanged();
return this;
}
/**
* bytes proofKey = 4;
* @return This builder for chaining.
*/
public Builder clearProofKey() {
proofKey_ = getDefaultInstance().getProofKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString proofVal_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes proofVal = 5;
* @return The proofVal.
*/
@java.lang.Override
public com.google.protobuf.ByteString getProofVal() {
return proofVal_;
}
/**
* bytes proofVal = 5;
* @param value The proofVal to set.
* @return This builder for chaining.
*/
public Builder setProofVal(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proofVal_ = value;
onChanged();
return this;
}
/**
* bytes proofVal = 5;
* @return This builder for chaining.
*/
public Builder clearProofVal() {
proofVal_ = getDefaultInstance().getProofVal();
onChanged();
return this;
}
private com.google.protobuf.ByteString bitmap_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes bitmap = 6;
* @return The bitmap.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBitmap() {
return bitmap_;
}
/**
* bytes bitmap = 6;
* @param value The bitmap to set.
* @return This builder for chaining.
*/
public Builder setBitmap(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitmap_ = value;
onChanged();
return this;
}
/**
* bytes bitmap = 6;
* @return This builder for chaining.
*/
public Builder clearBitmap() {
bitmap_ = getDefaultInstance().getBitmap();
onChanged();
return this;
}
private int height_ ;
/**
* uint32 height = 7;
* @return The height.
*/
@java.lang.Override
public int getHeight() {
return height_;
}
/**
* uint32 height = 7;
* @param value The height to set.
* @return This builder for chaining.
*/
public Builder setHeight(int value) {
height_ = value;
onChanged();
return this;
}
/**
* uint32 height = 7;
* @return This builder for chaining.
*/
public Builder clearHeight() {
height_ = 0;
onChanged();
return this;
}
private java.util.List auditPath_ = java.util.Collections.emptyList();
private void ensureAuditPathIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
auditPath_ = new java.util.ArrayList(auditPath_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated bytes auditPath = 8;
* @return A list containing the auditPath.
*/
public java.util.List
getAuditPathList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(auditPath_) : auditPath_;
}
/**
* repeated bytes auditPath = 8;
* @return The count of auditPath.
*/
public int getAuditPathCount() {
return auditPath_.size();
}
/**
* repeated bytes auditPath = 8;
* @param index The index of the element to return.
* @return The auditPath at the given index.
*/
public com.google.protobuf.ByteString getAuditPath(int index) {
return auditPath_.get(index);
}
/**
* repeated bytes auditPath = 8;
* @param index The index to set the value at.
* @param value The auditPath to set.
* @return This builder for chaining.
*/
public Builder setAuditPath(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureAuditPathIsMutable();
auditPath_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes auditPath = 8;
* @param value The auditPath to add.
* @return This builder for chaining.
*/
public Builder addAuditPath(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureAuditPathIsMutable();
auditPath_.add(value);
onChanged();
return this;
}
/**
* repeated bytes auditPath = 8;
* @param values The auditPath to add.
* @return This builder for chaining.
*/
public Builder addAllAuditPath(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureAuditPathIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, auditPath_);
onChanged();
return this;
}
/**
* repeated bytes auditPath = 8;
* @return This builder for chaining.
*/
public Builder clearAuditPath() {
auditPath_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes key = 9;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
* bytes key = 9;
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
return this;
}
/**
* bytes key = 9;
* @return This builder for chaining.
*/
public Builder clearKey() {
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.ContractVarProof)
}
// @@protoc_insertion_point(class_scope:types.ContractVarProof)
private static final types.Blockchain.ContractVarProof DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.ContractVarProof();
}
public static types.Blockchain.ContractVarProof getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ContractVarProof parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ContractVarProof(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.ContractVarProof getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StateQueryProofOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.StateQueryProof)
com.google.protobuf.MessageOrBuilder {
/**
* .types.AccountProof contractProof = 1;
* @return Whether the contractProof field is set.
*/
boolean hasContractProof();
/**
* .types.AccountProof contractProof = 1;
* @return The contractProof.
*/
types.Blockchain.AccountProof getContractProof();
/**
* .types.AccountProof contractProof = 1;
*/
types.Blockchain.AccountProofOrBuilder getContractProofOrBuilder();
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
java.util.List
getVarProofsList();
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
types.Blockchain.ContractVarProof getVarProofs(int index);
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
int getVarProofsCount();
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
java.util.List extends types.Blockchain.ContractVarProofOrBuilder>
getVarProofsOrBuilderList();
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
types.Blockchain.ContractVarProofOrBuilder getVarProofsOrBuilder(
int index);
}
/**
* Protobuf type {@code types.StateQueryProof}
*/
public static final class StateQueryProof extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.StateQueryProof)
StateQueryProofOrBuilder {
private static final long serialVersionUID = 0L;
// Use StateQueryProof.newBuilder() to construct.
private StateQueryProof(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StateQueryProof() {
varProofs_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StateQueryProof();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StateQueryProof(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
types.Blockchain.AccountProof.Builder subBuilder = null;
if (contractProof_ != null) {
subBuilder = contractProof_.toBuilder();
}
contractProof_ = input.readMessage(types.Blockchain.AccountProof.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(contractProof_);
contractProof_ = subBuilder.buildPartial();
}
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
varProofs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
varProofs_.add(
input.readMessage(types.Blockchain.ContractVarProof.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
varProofs_ = java.util.Collections.unmodifiableList(varProofs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_StateQueryProof_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_StateQueryProof_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.StateQueryProof.class, types.Blockchain.StateQueryProof.Builder.class);
}
public static final int CONTRACTPROOF_FIELD_NUMBER = 1;
private types.Blockchain.AccountProof contractProof_;
/**
* .types.AccountProof contractProof = 1;
* @return Whether the contractProof field is set.
*/
@java.lang.Override
public boolean hasContractProof() {
return contractProof_ != null;
}
/**
* .types.AccountProof contractProof = 1;
* @return The contractProof.
*/
@java.lang.Override
public types.Blockchain.AccountProof getContractProof() {
return contractProof_ == null ? types.Blockchain.AccountProof.getDefaultInstance() : contractProof_;
}
/**
* .types.AccountProof contractProof = 1;
*/
@java.lang.Override
public types.Blockchain.AccountProofOrBuilder getContractProofOrBuilder() {
return getContractProof();
}
public static final int VARPROOFS_FIELD_NUMBER = 2;
private java.util.List varProofs_;
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
@java.lang.Override
public java.util.List getVarProofsList() {
return varProofs_;
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
@java.lang.Override
public java.util.List extends types.Blockchain.ContractVarProofOrBuilder>
getVarProofsOrBuilderList() {
return varProofs_;
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
@java.lang.Override
public int getVarProofsCount() {
return varProofs_.size();
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
@java.lang.Override
public types.Blockchain.ContractVarProof getVarProofs(int index) {
return varProofs_.get(index);
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
@java.lang.Override
public types.Blockchain.ContractVarProofOrBuilder getVarProofsOrBuilder(
int index) {
return varProofs_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (contractProof_ != null) {
output.writeMessage(1, getContractProof());
}
for (int i = 0; i < varProofs_.size(); i++) {
output.writeMessage(2, varProofs_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (contractProof_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getContractProof());
}
for (int i = 0; i < varProofs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, varProofs_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.StateQueryProof)) {
return super.equals(obj);
}
types.Blockchain.StateQueryProof other = (types.Blockchain.StateQueryProof) obj;
if (hasContractProof() != other.hasContractProof()) return false;
if (hasContractProof()) {
if (!getContractProof()
.equals(other.getContractProof())) return false;
}
if (!getVarProofsList()
.equals(other.getVarProofsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasContractProof()) {
hash = (37 * hash) + CONTRACTPROOF_FIELD_NUMBER;
hash = (53 * hash) + getContractProof().hashCode();
}
if (getVarProofsCount() > 0) {
hash = (37 * hash) + VARPROOFS_FIELD_NUMBER;
hash = (53 * hash) + getVarProofsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.StateQueryProof parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.StateQueryProof parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.StateQueryProof parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.StateQueryProof parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.StateQueryProof parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.StateQueryProof parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.StateQueryProof parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.StateQueryProof parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.StateQueryProof parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.StateQueryProof parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.StateQueryProof parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.StateQueryProof parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.StateQueryProof prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.StateQueryProof}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.StateQueryProof)
types.Blockchain.StateQueryProofOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_StateQueryProof_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_StateQueryProof_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.StateQueryProof.class, types.Blockchain.StateQueryProof.Builder.class);
}
// Construct using types.Blockchain.StateQueryProof.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getVarProofsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (contractProofBuilder_ == null) {
contractProof_ = null;
} else {
contractProof_ = null;
contractProofBuilder_ = null;
}
if (varProofsBuilder_ == null) {
varProofs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
varProofsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_StateQueryProof_descriptor;
}
@java.lang.Override
public types.Blockchain.StateQueryProof getDefaultInstanceForType() {
return types.Blockchain.StateQueryProof.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.StateQueryProof build() {
types.Blockchain.StateQueryProof result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.StateQueryProof buildPartial() {
types.Blockchain.StateQueryProof result = new types.Blockchain.StateQueryProof(this);
int from_bitField0_ = bitField0_;
if (contractProofBuilder_ == null) {
result.contractProof_ = contractProof_;
} else {
result.contractProof_ = contractProofBuilder_.build();
}
if (varProofsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
varProofs_ = java.util.Collections.unmodifiableList(varProofs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.varProofs_ = varProofs_;
} else {
result.varProofs_ = varProofsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.StateQueryProof) {
return mergeFrom((types.Blockchain.StateQueryProof)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.StateQueryProof other) {
if (other == types.Blockchain.StateQueryProof.getDefaultInstance()) return this;
if (other.hasContractProof()) {
mergeContractProof(other.getContractProof());
}
if (varProofsBuilder_ == null) {
if (!other.varProofs_.isEmpty()) {
if (varProofs_.isEmpty()) {
varProofs_ = other.varProofs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureVarProofsIsMutable();
varProofs_.addAll(other.varProofs_);
}
onChanged();
}
} else {
if (!other.varProofs_.isEmpty()) {
if (varProofsBuilder_.isEmpty()) {
varProofsBuilder_.dispose();
varProofsBuilder_ = null;
varProofs_ = other.varProofs_;
bitField0_ = (bitField0_ & ~0x00000001);
varProofsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getVarProofsFieldBuilder() : null;
} else {
varProofsBuilder_.addAllMessages(other.varProofs_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.StateQueryProof parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.StateQueryProof) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private types.Blockchain.AccountProof contractProof_;
private com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.AccountProof, types.Blockchain.AccountProof.Builder, types.Blockchain.AccountProofOrBuilder> contractProofBuilder_;
/**
* .types.AccountProof contractProof = 1;
* @return Whether the contractProof field is set.
*/
public boolean hasContractProof() {
return contractProofBuilder_ != null || contractProof_ != null;
}
/**
* .types.AccountProof contractProof = 1;
* @return The contractProof.
*/
public types.Blockchain.AccountProof getContractProof() {
if (contractProofBuilder_ == null) {
return contractProof_ == null ? types.Blockchain.AccountProof.getDefaultInstance() : contractProof_;
} else {
return contractProofBuilder_.getMessage();
}
}
/**
* .types.AccountProof contractProof = 1;
*/
public Builder setContractProof(types.Blockchain.AccountProof value) {
if (contractProofBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
contractProof_ = value;
onChanged();
} else {
contractProofBuilder_.setMessage(value);
}
return this;
}
/**
* .types.AccountProof contractProof = 1;
*/
public Builder setContractProof(
types.Blockchain.AccountProof.Builder builderForValue) {
if (contractProofBuilder_ == null) {
contractProof_ = builderForValue.build();
onChanged();
} else {
contractProofBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .types.AccountProof contractProof = 1;
*/
public Builder mergeContractProof(types.Blockchain.AccountProof value) {
if (contractProofBuilder_ == null) {
if (contractProof_ != null) {
contractProof_ =
types.Blockchain.AccountProof.newBuilder(contractProof_).mergeFrom(value).buildPartial();
} else {
contractProof_ = value;
}
onChanged();
} else {
contractProofBuilder_.mergeFrom(value);
}
return this;
}
/**
* .types.AccountProof contractProof = 1;
*/
public Builder clearContractProof() {
if (contractProofBuilder_ == null) {
contractProof_ = null;
onChanged();
} else {
contractProof_ = null;
contractProofBuilder_ = null;
}
return this;
}
/**
* .types.AccountProof contractProof = 1;
*/
public types.Blockchain.AccountProof.Builder getContractProofBuilder() {
onChanged();
return getContractProofFieldBuilder().getBuilder();
}
/**
* .types.AccountProof contractProof = 1;
*/
public types.Blockchain.AccountProofOrBuilder getContractProofOrBuilder() {
if (contractProofBuilder_ != null) {
return contractProofBuilder_.getMessageOrBuilder();
} else {
return contractProof_ == null ?
types.Blockchain.AccountProof.getDefaultInstance() : contractProof_;
}
}
/**
* .types.AccountProof contractProof = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.AccountProof, types.Blockchain.AccountProof.Builder, types.Blockchain.AccountProofOrBuilder>
getContractProofFieldBuilder() {
if (contractProofBuilder_ == null) {
contractProofBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
types.Blockchain.AccountProof, types.Blockchain.AccountProof.Builder, types.Blockchain.AccountProofOrBuilder>(
getContractProof(),
getParentForChildren(),
isClean());
contractProof_ = null;
}
return contractProofBuilder_;
}
private java.util.List varProofs_ =
java.util.Collections.emptyList();
private void ensureVarProofsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
varProofs_ = new java.util.ArrayList(varProofs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.ContractVarProof, types.Blockchain.ContractVarProof.Builder, types.Blockchain.ContractVarProofOrBuilder> varProofsBuilder_;
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public java.util.List getVarProofsList() {
if (varProofsBuilder_ == null) {
return java.util.Collections.unmodifiableList(varProofs_);
} else {
return varProofsBuilder_.getMessageList();
}
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public int getVarProofsCount() {
if (varProofsBuilder_ == null) {
return varProofs_.size();
} else {
return varProofsBuilder_.getCount();
}
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public types.Blockchain.ContractVarProof getVarProofs(int index) {
if (varProofsBuilder_ == null) {
return varProofs_.get(index);
} else {
return varProofsBuilder_.getMessage(index);
}
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public Builder setVarProofs(
int index, types.Blockchain.ContractVarProof value) {
if (varProofsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVarProofsIsMutable();
varProofs_.set(index, value);
onChanged();
} else {
varProofsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public Builder setVarProofs(
int index, types.Blockchain.ContractVarProof.Builder builderForValue) {
if (varProofsBuilder_ == null) {
ensureVarProofsIsMutable();
varProofs_.set(index, builderForValue.build());
onChanged();
} else {
varProofsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public Builder addVarProofs(types.Blockchain.ContractVarProof value) {
if (varProofsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVarProofsIsMutable();
varProofs_.add(value);
onChanged();
} else {
varProofsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public Builder addVarProofs(
int index, types.Blockchain.ContractVarProof value) {
if (varProofsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVarProofsIsMutable();
varProofs_.add(index, value);
onChanged();
} else {
varProofsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public Builder addVarProofs(
types.Blockchain.ContractVarProof.Builder builderForValue) {
if (varProofsBuilder_ == null) {
ensureVarProofsIsMutable();
varProofs_.add(builderForValue.build());
onChanged();
} else {
varProofsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public Builder addVarProofs(
int index, types.Blockchain.ContractVarProof.Builder builderForValue) {
if (varProofsBuilder_ == null) {
ensureVarProofsIsMutable();
varProofs_.add(index, builderForValue.build());
onChanged();
} else {
varProofsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public Builder addAllVarProofs(
java.lang.Iterable extends types.Blockchain.ContractVarProof> values) {
if (varProofsBuilder_ == null) {
ensureVarProofsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, varProofs_);
onChanged();
} else {
varProofsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public Builder clearVarProofs() {
if (varProofsBuilder_ == null) {
varProofs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
varProofsBuilder_.clear();
}
return this;
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public Builder removeVarProofs(int index) {
if (varProofsBuilder_ == null) {
ensureVarProofsIsMutable();
varProofs_.remove(index);
onChanged();
} else {
varProofsBuilder_.remove(index);
}
return this;
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public types.Blockchain.ContractVarProof.Builder getVarProofsBuilder(
int index) {
return getVarProofsFieldBuilder().getBuilder(index);
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public types.Blockchain.ContractVarProofOrBuilder getVarProofsOrBuilder(
int index) {
if (varProofsBuilder_ == null) {
return varProofs_.get(index); } else {
return varProofsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public java.util.List extends types.Blockchain.ContractVarProofOrBuilder>
getVarProofsOrBuilderList() {
if (varProofsBuilder_ != null) {
return varProofsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(varProofs_);
}
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public types.Blockchain.ContractVarProof.Builder addVarProofsBuilder() {
return getVarProofsFieldBuilder().addBuilder(
types.Blockchain.ContractVarProof.getDefaultInstance());
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public types.Blockchain.ContractVarProof.Builder addVarProofsBuilder(
int index) {
return getVarProofsFieldBuilder().addBuilder(
index, types.Blockchain.ContractVarProof.getDefaultInstance());
}
/**
* repeated .types.ContractVarProof varProofs = 2;
*/
public java.util.List
getVarProofsBuilderList() {
return getVarProofsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.ContractVarProof, types.Blockchain.ContractVarProof.Builder, types.Blockchain.ContractVarProofOrBuilder>
getVarProofsFieldBuilder() {
if (varProofsBuilder_ == null) {
varProofsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.ContractVarProof, types.Blockchain.ContractVarProof.Builder, types.Blockchain.ContractVarProofOrBuilder>(
varProofs_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
varProofs_ = null;
}
return varProofsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.StateQueryProof)
}
// @@protoc_insertion_point(class_scope:types.StateQueryProof)
private static final types.Blockchain.StateQueryProof DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.StateQueryProof();
}
public static types.Blockchain.StateQueryProof getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StateQueryProof parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StateQueryProof(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.StateQueryProof getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ReceiptOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.Receipt)
com.google.protobuf.MessageOrBuilder {
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
com.google.protobuf.ByteString getContractAddress();
/**
* string status = 2;
* @return The status.
*/
java.lang.String getStatus();
/**
* string status = 2;
* @return The bytes for status.
*/
com.google.protobuf.ByteString
getStatusBytes();
/**
* string ret = 3;
* @return The ret.
*/
java.lang.String getRet();
/**
* string ret = 3;
* @return The bytes for ret.
*/
com.google.protobuf.ByteString
getRetBytes();
/**
* bytes txHash = 4;
* @return The txHash.
*/
com.google.protobuf.ByteString getTxHash();
/**
* bytes feeUsed = 5;
* @return The feeUsed.
*/
com.google.protobuf.ByteString getFeeUsed();
/**
* bytes cumulativeFeeUsed = 6;
* @return The cumulativeFeeUsed.
*/
com.google.protobuf.ByteString getCumulativeFeeUsed();
/**
* bytes bloom = 7;
* @return The bloom.
*/
com.google.protobuf.ByteString getBloom();
/**
* repeated .types.Event events = 8;
*/
java.util.List
getEventsList();
/**
* repeated .types.Event events = 8;
*/
types.Blockchain.Event getEvents(int index);
/**
* repeated .types.Event events = 8;
*/
int getEventsCount();
/**
* repeated .types.Event events = 8;
*/
java.util.List extends types.Blockchain.EventOrBuilder>
getEventsOrBuilderList();
/**
* repeated .types.Event events = 8;
*/
types.Blockchain.EventOrBuilder getEventsOrBuilder(
int index);
/**
* uint64 blockNo = 9;
* @return The blockNo.
*/
long getBlockNo();
/**
* bytes blockHash = 10;
* @return The blockHash.
*/
com.google.protobuf.ByteString getBlockHash();
/**
* int32 txIndex = 11;
* @return The txIndex.
*/
int getTxIndex();
/**
* bytes from = 12;
* @return The from.
*/
com.google.protobuf.ByteString getFrom();
/**
* bytes to = 13;
* @return The to.
*/
com.google.protobuf.ByteString getTo();
/**
* bool feeDelegation = 14;
* @return The feeDelegation.
*/
boolean getFeeDelegation();
/**
* uint64 gasUsed = 15;
* @return The gasUsed.
*/
long getGasUsed();
}
/**
* Protobuf type {@code types.Receipt}
*/
public static final class Receipt extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.Receipt)
ReceiptOrBuilder {
private static final long serialVersionUID = 0L;
// Use Receipt.newBuilder() to construct.
private Receipt(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Receipt() {
contractAddress_ = com.google.protobuf.ByteString.EMPTY;
status_ = "";
ret_ = "";
txHash_ = com.google.protobuf.ByteString.EMPTY;
feeUsed_ = com.google.protobuf.ByteString.EMPTY;
cumulativeFeeUsed_ = com.google.protobuf.ByteString.EMPTY;
bloom_ = com.google.protobuf.ByteString.EMPTY;
events_ = java.util.Collections.emptyList();
blockHash_ = com.google.protobuf.ByteString.EMPTY;
from_ = com.google.protobuf.ByteString.EMPTY;
to_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Receipt();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Receipt(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
contractAddress_ = input.readBytes();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
status_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
ret_ = s;
break;
}
case 34: {
txHash_ = input.readBytes();
break;
}
case 42: {
feeUsed_ = input.readBytes();
break;
}
case 50: {
cumulativeFeeUsed_ = input.readBytes();
break;
}
case 58: {
bloom_ = input.readBytes();
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
events_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
events_.add(
input.readMessage(types.Blockchain.Event.parser(), extensionRegistry));
break;
}
case 72: {
blockNo_ = input.readUInt64();
break;
}
case 82: {
blockHash_ = input.readBytes();
break;
}
case 88: {
txIndex_ = input.readInt32();
break;
}
case 98: {
from_ = input.readBytes();
break;
}
case 106: {
to_ = input.readBytes();
break;
}
case 112: {
feeDelegation_ = input.readBool();
break;
}
case 120: {
gasUsed_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
events_ = java.util.Collections.unmodifiableList(events_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_Receipt_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_Receipt_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.Receipt.class, types.Blockchain.Receipt.Builder.class);
}
public static final int CONTRACTADDRESS_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString contractAddress_;
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
@java.lang.Override
public com.google.protobuf.ByteString getContractAddress() {
return contractAddress_;
}
public static final int STATUS_FIELD_NUMBER = 2;
private volatile java.lang.Object status_;
/**
* string status = 2;
* @return The status.
*/
@java.lang.Override
public java.lang.String getStatus() {
java.lang.Object ref = status_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
status_ = s;
return s;
}
}
/**
* string status = 2;
* @return The bytes for status.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStatusBytes() {
java.lang.Object ref = status_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
status_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RET_FIELD_NUMBER = 3;
private volatile java.lang.Object ret_;
/**
* string ret = 3;
* @return The ret.
*/
@java.lang.Override
public java.lang.String getRet() {
java.lang.Object ref = ret_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ret_ = s;
return s;
}
}
/**
* string ret = 3;
* @return The bytes for ret.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRetBytes() {
java.lang.Object ref = ret_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ret_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TXHASH_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString txHash_;
/**
* bytes txHash = 4;
* @return The txHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTxHash() {
return txHash_;
}
public static final int FEEUSED_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString feeUsed_;
/**
* bytes feeUsed = 5;
* @return The feeUsed.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFeeUsed() {
return feeUsed_;
}
public static final int CUMULATIVEFEEUSED_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString cumulativeFeeUsed_;
/**
* bytes cumulativeFeeUsed = 6;
* @return The cumulativeFeeUsed.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCumulativeFeeUsed() {
return cumulativeFeeUsed_;
}
public static final int BLOOM_FIELD_NUMBER = 7;
private com.google.protobuf.ByteString bloom_;
/**
* bytes bloom = 7;
* @return The bloom.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBloom() {
return bloom_;
}
public static final int EVENTS_FIELD_NUMBER = 8;
private java.util.List events_;
/**
* repeated .types.Event events = 8;
*/
@java.lang.Override
public java.util.List getEventsList() {
return events_;
}
/**
* repeated .types.Event events = 8;
*/
@java.lang.Override
public java.util.List extends types.Blockchain.EventOrBuilder>
getEventsOrBuilderList() {
return events_;
}
/**
* repeated .types.Event events = 8;
*/
@java.lang.Override
public int getEventsCount() {
return events_.size();
}
/**
* repeated .types.Event events = 8;
*/
@java.lang.Override
public types.Blockchain.Event getEvents(int index) {
return events_.get(index);
}
/**
* repeated .types.Event events = 8;
*/
@java.lang.Override
public types.Blockchain.EventOrBuilder getEventsOrBuilder(
int index) {
return events_.get(index);
}
public static final int BLOCKNO_FIELD_NUMBER = 9;
private long blockNo_;
/**
* uint64 blockNo = 9;
* @return The blockNo.
*/
@java.lang.Override
public long getBlockNo() {
return blockNo_;
}
public static final int BLOCKHASH_FIELD_NUMBER = 10;
private com.google.protobuf.ByteString blockHash_;
/**
* bytes blockHash = 10;
* @return The blockHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBlockHash() {
return blockHash_;
}
public static final int TXINDEX_FIELD_NUMBER = 11;
private int txIndex_;
/**
* int32 txIndex = 11;
* @return The txIndex.
*/
@java.lang.Override
public int getTxIndex() {
return txIndex_;
}
public static final int FROM_FIELD_NUMBER = 12;
private com.google.protobuf.ByteString from_;
/**
* bytes from = 12;
* @return The from.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFrom() {
return from_;
}
public static final int TO_FIELD_NUMBER = 13;
private com.google.protobuf.ByteString to_;
/**
* bytes to = 13;
* @return The to.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTo() {
return to_;
}
public static final int FEEDELEGATION_FIELD_NUMBER = 14;
private boolean feeDelegation_;
/**
* bool feeDelegation = 14;
* @return The feeDelegation.
*/
@java.lang.Override
public boolean getFeeDelegation() {
return feeDelegation_;
}
public static final int GASUSED_FIELD_NUMBER = 15;
private long gasUsed_;
/**
* uint64 gasUsed = 15;
* @return The gasUsed.
*/
@java.lang.Override
public long getGasUsed() {
return gasUsed_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!contractAddress_.isEmpty()) {
output.writeBytes(1, contractAddress_);
}
if (!getStatusBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, status_);
}
if (!getRetBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, ret_);
}
if (!txHash_.isEmpty()) {
output.writeBytes(4, txHash_);
}
if (!feeUsed_.isEmpty()) {
output.writeBytes(5, feeUsed_);
}
if (!cumulativeFeeUsed_.isEmpty()) {
output.writeBytes(6, cumulativeFeeUsed_);
}
if (!bloom_.isEmpty()) {
output.writeBytes(7, bloom_);
}
for (int i = 0; i < events_.size(); i++) {
output.writeMessage(8, events_.get(i));
}
if (blockNo_ != 0L) {
output.writeUInt64(9, blockNo_);
}
if (!blockHash_.isEmpty()) {
output.writeBytes(10, blockHash_);
}
if (txIndex_ != 0) {
output.writeInt32(11, txIndex_);
}
if (!from_.isEmpty()) {
output.writeBytes(12, from_);
}
if (!to_.isEmpty()) {
output.writeBytes(13, to_);
}
if (feeDelegation_ != false) {
output.writeBool(14, feeDelegation_);
}
if (gasUsed_ != 0L) {
output.writeUInt64(15, gasUsed_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!contractAddress_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, contractAddress_);
}
if (!getStatusBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, status_);
}
if (!getRetBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, ret_);
}
if (!txHash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, txHash_);
}
if (!feeUsed_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, feeUsed_);
}
if (!cumulativeFeeUsed_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, cumulativeFeeUsed_);
}
if (!bloom_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, bloom_);
}
for (int i = 0; i < events_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, events_.get(i));
}
if (blockNo_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(9, blockNo_);
}
if (!blockHash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(10, blockHash_);
}
if (txIndex_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(11, txIndex_);
}
if (!from_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(12, from_);
}
if (!to_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(13, to_);
}
if (feeDelegation_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(14, feeDelegation_);
}
if (gasUsed_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(15, gasUsed_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.Receipt)) {
return super.equals(obj);
}
types.Blockchain.Receipt other = (types.Blockchain.Receipt) obj;
if (!getContractAddress()
.equals(other.getContractAddress())) return false;
if (!getStatus()
.equals(other.getStatus())) return false;
if (!getRet()
.equals(other.getRet())) return false;
if (!getTxHash()
.equals(other.getTxHash())) return false;
if (!getFeeUsed()
.equals(other.getFeeUsed())) return false;
if (!getCumulativeFeeUsed()
.equals(other.getCumulativeFeeUsed())) return false;
if (!getBloom()
.equals(other.getBloom())) return false;
if (!getEventsList()
.equals(other.getEventsList())) return false;
if (getBlockNo()
!= other.getBlockNo()) return false;
if (!getBlockHash()
.equals(other.getBlockHash())) return false;
if (getTxIndex()
!= other.getTxIndex()) return false;
if (!getFrom()
.equals(other.getFrom())) return false;
if (!getTo()
.equals(other.getTo())) return false;
if (getFeeDelegation()
!= other.getFeeDelegation()) return false;
if (getGasUsed()
!= other.getGasUsed()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTRACTADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getContractAddress().hashCode();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
hash = (37 * hash) + RET_FIELD_NUMBER;
hash = (53 * hash) + getRet().hashCode();
hash = (37 * hash) + TXHASH_FIELD_NUMBER;
hash = (53 * hash) + getTxHash().hashCode();
hash = (37 * hash) + FEEUSED_FIELD_NUMBER;
hash = (53 * hash) + getFeeUsed().hashCode();
hash = (37 * hash) + CUMULATIVEFEEUSED_FIELD_NUMBER;
hash = (53 * hash) + getCumulativeFeeUsed().hashCode();
hash = (37 * hash) + BLOOM_FIELD_NUMBER;
hash = (53 * hash) + getBloom().hashCode();
if (getEventsCount() > 0) {
hash = (37 * hash) + EVENTS_FIELD_NUMBER;
hash = (53 * hash) + getEventsList().hashCode();
}
hash = (37 * hash) + BLOCKNO_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBlockNo());
hash = (37 * hash) + BLOCKHASH_FIELD_NUMBER;
hash = (53 * hash) + getBlockHash().hashCode();
hash = (37 * hash) + TXINDEX_FIELD_NUMBER;
hash = (53 * hash) + getTxIndex();
hash = (37 * hash) + FROM_FIELD_NUMBER;
hash = (53 * hash) + getFrom().hashCode();
hash = (37 * hash) + TO_FIELD_NUMBER;
hash = (53 * hash) + getTo().hashCode();
hash = (37 * hash) + FEEDELEGATION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getFeeDelegation());
hash = (37 * hash) + GASUSED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getGasUsed());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.Receipt parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Receipt parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Receipt parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Receipt parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Receipt parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Receipt parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Receipt parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.Receipt parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.Receipt parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.Receipt parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.Receipt parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.Receipt parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.Receipt prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.Receipt}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.Receipt)
types.Blockchain.ReceiptOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_Receipt_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_Receipt_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.Receipt.class, types.Blockchain.Receipt.Builder.class);
}
// Construct using types.Blockchain.Receipt.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getEventsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
contractAddress_ = com.google.protobuf.ByteString.EMPTY;
status_ = "";
ret_ = "";
txHash_ = com.google.protobuf.ByteString.EMPTY;
feeUsed_ = com.google.protobuf.ByteString.EMPTY;
cumulativeFeeUsed_ = com.google.protobuf.ByteString.EMPTY;
bloom_ = com.google.protobuf.ByteString.EMPTY;
if (eventsBuilder_ == null) {
events_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
eventsBuilder_.clear();
}
blockNo_ = 0L;
blockHash_ = com.google.protobuf.ByteString.EMPTY;
txIndex_ = 0;
from_ = com.google.protobuf.ByteString.EMPTY;
to_ = com.google.protobuf.ByteString.EMPTY;
feeDelegation_ = false;
gasUsed_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_Receipt_descriptor;
}
@java.lang.Override
public types.Blockchain.Receipt getDefaultInstanceForType() {
return types.Blockchain.Receipt.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.Receipt build() {
types.Blockchain.Receipt result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.Receipt buildPartial() {
types.Blockchain.Receipt result = new types.Blockchain.Receipt(this);
int from_bitField0_ = bitField0_;
result.contractAddress_ = contractAddress_;
result.status_ = status_;
result.ret_ = ret_;
result.txHash_ = txHash_;
result.feeUsed_ = feeUsed_;
result.cumulativeFeeUsed_ = cumulativeFeeUsed_;
result.bloom_ = bloom_;
if (eventsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
events_ = java.util.Collections.unmodifiableList(events_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.events_ = events_;
} else {
result.events_ = eventsBuilder_.build();
}
result.blockNo_ = blockNo_;
result.blockHash_ = blockHash_;
result.txIndex_ = txIndex_;
result.from_ = from_;
result.to_ = to_;
result.feeDelegation_ = feeDelegation_;
result.gasUsed_ = gasUsed_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.Receipt) {
return mergeFrom((types.Blockchain.Receipt)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.Receipt other) {
if (other == types.Blockchain.Receipt.getDefaultInstance()) return this;
if (other.getContractAddress() != com.google.protobuf.ByteString.EMPTY) {
setContractAddress(other.getContractAddress());
}
if (!other.getStatus().isEmpty()) {
status_ = other.status_;
onChanged();
}
if (!other.getRet().isEmpty()) {
ret_ = other.ret_;
onChanged();
}
if (other.getTxHash() != com.google.protobuf.ByteString.EMPTY) {
setTxHash(other.getTxHash());
}
if (other.getFeeUsed() != com.google.protobuf.ByteString.EMPTY) {
setFeeUsed(other.getFeeUsed());
}
if (other.getCumulativeFeeUsed() != com.google.protobuf.ByteString.EMPTY) {
setCumulativeFeeUsed(other.getCumulativeFeeUsed());
}
if (other.getBloom() != com.google.protobuf.ByteString.EMPTY) {
setBloom(other.getBloom());
}
if (eventsBuilder_ == null) {
if (!other.events_.isEmpty()) {
if (events_.isEmpty()) {
events_ = other.events_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureEventsIsMutable();
events_.addAll(other.events_);
}
onChanged();
}
} else {
if (!other.events_.isEmpty()) {
if (eventsBuilder_.isEmpty()) {
eventsBuilder_.dispose();
eventsBuilder_ = null;
events_ = other.events_;
bitField0_ = (bitField0_ & ~0x00000001);
eventsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getEventsFieldBuilder() : null;
} else {
eventsBuilder_.addAllMessages(other.events_);
}
}
}
if (other.getBlockNo() != 0L) {
setBlockNo(other.getBlockNo());
}
if (other.getBlockHash() != com.google.protobuf.ByteString.EMPTY) {
setBlockHash(other.getBlockHash());
}
if (other.getTxIndex() != 0) {
setTxIndex(other.getTxIndex());
}
if (other.getFrom() != com.google.protobuf.ByteString.EMPTY) {
setFrom(other.getFrom());
}
if (other.getTo() != com.google.protobuf.ByteString.EMPTY) {
setTo(other.getTo());
}
if (other.getFeeDelegation() != false) {
setFeeDelegation(other.getFeeDelegation());
}
if (other.getGasUsed() != 0L) {
setGasUsed(other.getGasUsed());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.Receipt parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.Receipt) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString contractAddress_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
@java.lang.Override
public com.google.protobuf.ByteString getContractAddress() {
return contractAddress_;
}
/**
* bytes contractAddress = 1;
* @param value The contractAddress to set.
* @return This builder for chaining.
*/
public Builder setContractAddress(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
contractAddress_ = value;
onChanged();
return this;
}
/**
* bytes contractAddress = 1;
* @return This builder for chaining.
*/
public Builder clearContractAddress() {
contractAddress_ = getDefaultInstance().getContractAddress();
onChanged();
return this;
}
private java.lang.Object status_ = "";
/**
* string status = 2;
* @return The status.
*/
public java.lang.String getStatus() {
java.lang.Object ref = status_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
status_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string status = 2;
* @return The bytes for status.
*/
public com.google.protobuf.ByteString
getStatusBytes() {
java.lang.Object ref = status_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
status_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string status = 2;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
return this;
}
/**
* string status = 2;
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = getDefaultInstance().getStatus();
onChanged();
return this;
}
/**
* string status = 2;
* @param value The bytes for status to set.
* @return This builder for chaining.
*/
public Builder setStatusBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
status_ = value;
onChanged();
return this;
}
private java.lang.Object ret_ = "";
/**
* string ret = 3;
* @return The ret.
*/
public java.lang.String getRet() {
java.lang.Object ref = ret_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ret_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string ret = 3;
* @return The bytes for ret.
*/
public com.google.protobuf.ByteString
getRetBytes() {
java.lang.Object ref = ret_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ret_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string ret = 3;
* @param value The ret to set.
* @return This builder for chaining.
*/
public Builder setRet(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ret_ = value;
onChanged();
return this;
}
/**
* string ret = 3;
* @return This builder for chaining.
*/
public Builder clearRet() {
ret_ = getDefaultInstance().getRet();
onChanged();
return this;
}
/**
* string ret = 3;
* @param value The bytes for ret to set.
* @return This builder for chaining.
*/
public Builder setRetBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ret_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString txHash_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes txHash = 4;
* @return The txHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTxHash() {
return txHash_;
}
/**
* bytes txHash = 4;
* @param value The txHash to set.
* @return This builder for chaining.
*/
public Builder setTxHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
txHash_ = value;
onChanged();
return this;
}
/**
* bytes txHash = 4;
* @return This builder for chaining.
*/
public Builder clearTxHash() {
txHash_ = getDefaultInstance().getTxHash();
onChanged();
return this;
}
private com.google.protobuf.ByteString feeUsed_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes feeUsed = 5;
* @return The feeUsed.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFeeUsed() {
return feeUsed_;
}
/**
* bytes feeUsed = 5;
* @param value The feeUsed to set.
* @return This builder for chaining.
*/
public Builder setFeeUsed(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
feeUsed_ = value;
onChanged();
return this;
}
/**
* bytes feeUsed = 5;
* @return This builder for chaining.
*/
public Builder clearFeeUsed() {
feeUsed_ = getDefaultInstance().getFeeUsed();
onChanged();
return this;
}
private com.google.protobuf.ByteString cumulativeFeeUsed_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes cumulativeFeeUsed = 6;
* @return The cumulativeFeeUsed.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCumulativeFeeUsed() {
return cumulativeFeeUsed_;
}
/**
* bytes cumulativeFeeUsed = 6;
* @param value The cumulativeFeeUsed to set.
* @return This builder for chaining.
*/
public Builder setCumulativeFeeUsed(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
cumulativeFeeUsed_ = value;
onChanged();
return this;
}
/**
* bytes cumulativeFeeUsed = 6;
* @return This builder for chaining.
*/
public Builder clearCumulativeFeeUsed() {
cumulativeFeeUsed_ = getDefaultInstance().getCumulativeFeeUsed();
onChanged();
return this;
}
private com.google.protobuf.ByteString bloom_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes bloom = 7;
* @return The bloom.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBloom() {
return bloom_;
}
/**
* bytes bloom = 7;
* @param value The bloom to set.
* @return This builder for chaining.
*/
public Builder setBloom(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bloom_ = value;
onChanged();
return this;
}
/**
* bytes bloom = 7;
* @return This builder for chaining.
*/
public Builder clearBloom() {
bloom_ = getDefaultInstance().getBloom();
onChanged();
return this;
}
private java.util.List events_ =
java.util.Collections.emptyList();
private void ensureEventsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
events_ = new java.util.ArrayList(events_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.Event, types.Blockchain.Event.Builder, types.Blockchain.EventOrBuilder> eventsBuilder_;
/**
* repeated .types.Event events = 8;
*/
public java.util.List getEventsList() {
if (eventsBuilder_ == null) {
return java.util.Collections.unmodifiableList(events_);
} else {
return eventsBuilder_.getMessageList();
}
}
/**
* repeated .types.Event events = 8;
*/
public int getEventsCount() {
if (eventsBuilder_ == null) {
return events_.size();
} else {
return eventsBuilder_.getCount();
}
}
/**
* repeated .types.Event events = 8;
*/
public types.Blockchain.Event getEvents(int index) {
if (eventsBuilder_ == null) {
return events_.get(index);
} else {
return eventsBuilder_.getMessage(index);
}
}
/**
* repeated .types.Event events = 8;
*/
public Builder setEvents(
int index, types.Blockchain.Event value) {
if (eventsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEventsIsMutable();
events_.set(index, value);
onChanged();
} else {
eventsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .types.Event events = 8;
*/
public Builder setEvents(
int index, types.Blockchain.Event.Builder builderForValue) {
if (eventsBuilder_ == null) {
ensureEventsIsMutable();
events_.set(index, builderForValue.build());
onChanged();
} else {
eventsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .types.Event events = 8;
*/
public Builder addEvents(types.Blockchain.Event value) {
if (eventsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEventsIsMutable();
events_.add(value);
onChanged();
} else {
eventsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .types.Event events = 8;
*/
public Builder addEvents(
int index, types.Blockchain.Event value) {
if (eventsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEventsIsMutable();
events_.add(index, value);
onChanged();
} else {
eventsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .types.Event events = 8;
*/
public Builder addEvents(
types.Blockchain.Event.Builder builderForValue) {
if (eventsBuilder_ == null) {
ensureEventsIsMutable();
events_.add(builderForValue.build());
onChanged();
} else {
eventsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .types.Event events = 8;
*/
public Builder addEvents(
int index, types.Blockchain.Event.Builder builderForValue) {
if (eventsBuilder_ == null) {
ensureEventsIsMutable();
events_.add(index, builderForValue.build());
onChanged();
} else {
eventsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .types.Event events = 8;
*/
public Builder addAllEvents(
java.lang.Iterable extends types.Blockchain.Event> values) {
if (eventsBuilder_ == null) {
ensureEventsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, events_);
onChanged();
} else {
eventsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .types.Event events = 8;
*/
public Builder clearEvents() {
if (eventsBuilder_ == null) {
events_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
eventsBuilder_.clear();
}
return this;
}
/**
* repeated .types.Event events = 8;
*/
public Builder removeEvents(int index) {
if (eventsBuilder_ == null) {
ensureEventsIsMutable();
events_.remove(index);
onChanged();
} else {
eventsBuilder_.remove(index);
}
return this;
}
/**
* repeated .types.Event events = 8;
*/
public types.Blockchain.Event.Builder getEventsBuilder(
int index) {
return getEventsFieldBuilder().getBuilder(index);
}
/**
* repeated .types.Event events = 8;
*/
public types.Blockchain.EventOrBuilder getEventsOrBuilder(
int index) {
if (eventsBuilder_ == null) {
return events_.get(index); } else {
return eventsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .types.Event events = 8;
*/
public java.util.List extends types.Blockchain.EventOrBuilder>
getEventsOrBuilderList() {
if (eventsBuilder_ != null) {
return eventsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(events_);
}
}
/**
* repeated .types.Event events = 8;
*/
public types.Blockchain.Event.Builder addEventsBuilder() {
return getEventsFieldBuilder().addBuilder(
types.Blockchain.Event.getDefaultInstance());
}
/**
* repeated .types.Event events = 8;
*/
public types.Blockchain.Event.Builder addEventsBuilder(
int index) {
return getEventsFieldBuilder().addBuilder(
index, types.Blockchain.Event.getDefaultInstance());
}
/**
* repeated .types.Event events = 8;
*/
public java.util.List
getEventsBuilderList() {
return getEventsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.Event, types.Blockchain.Event.Builder, types.Blockchain.EventOrBuilder>
getEventsFieldBuilder() {
if (eventsBuilder_ == null) {
eventsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.Event, types.Blockchain.Event.Builder, types.Blockchain.EventOrBuilder>(
events_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
events_ = null;
}
return eventsBuilder_;
}
private long blockNo_ ;
/**
* uint64 blockNo = 9;
* @return The blockNo.
*/
@java.lang.Override
public long getBlockNo() {
return blockNo_;
}
/**
* uint64 blockNo = 9;
* @param value The blockNo to set.
* @return This builder for chaining.
*/
public Builder setBlockNo(long value) {
blockNo_ = value;
onChanged();
return this;
}
/**
* uint64 blockNo = 9;
* @return This builder for chaining.
*/
public Builder clearBlockNo() {
blockNo_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString blockHash_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes blockHash = 10;
* @return The blockHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBlockHash() {
return blockHash_;
}
/**
* bytes blockHash = 10;
* @param value The blockHash to set.
* @return This builder for chaining.
*/
public Builder setBlockHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
blockHash_ = value;
onChanged();
return this;
}
/**
* bytes blockHash = 10;
* @return This builder for chaining.
*/
public Builder clearBlockHash() {
blockHash_ = getDefaultInstance().getBlockHash();
onChanged();
return this;
}
private int txIndex_ ;
/**
* int32 txIndex = 11;
* @return The txIndex.
*/
@java.lang.Override
public int getTxIndex() {
return txIndex_;
}
/**
* int32 txIndex = 11;
* @param value The txIndex to set.
* @return This builder for chaining.
*/
public Builder setTxIndex(int value) {
txIndex_ = value;
onChanged();
return this;
}
/**
* int32 txIndex = 11;
* @return This builder for chaining.
*/
public Builder clearTxIndex() {
txIndex_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString from_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes from = 12;
* @return The from.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFrom() {
return from_;
}
/**
* bytes from = 12;
* @param value The from to set.
* @return This builder for chaining.
*/
public Builder setFrom(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
from_ = value;
onChanged();
return this;
}
/**
* bytes from = 12;
* @return This builder for chaining.
*/
public Builder clearFrom() {
from_ = getDefaultInstance().getFrom();
onChanged();
return this;
}
private com.google.protobuf.ByteString to_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes to = 13;
* @return The to.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTo() {
return to_;
}
/**
* bytes to = 13;
* @param value The to to set.
* @return This builder for chaining.
*/
public Builder setTo(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
to_ = value;
onChanged();
return this;
}
/**
* bytes to = 13;
* @return This builder for chaining.
*/
public Builder clearTo() {
to_ = getDefaultInstance().getTo();
onChanged();
return this;
}
private boolean feeDelegation_ ;
/**
* bool feeDelegation = 14;
* @return The feeDelegation.
*/
@java.lang.Override
public boolean getFeeDelegation() {
return feeDelegation_;
}
/**
* bool feeDelegation = 14;
* @param value The feeDelegation to set.
* @return This builder for chaining.
*/
public Builder setFeeDelegation(boolean value) {
feeDelegation_ = value;
onChanged();
return this;
}
/**
* bool feeDelegation = 14;
* @return This builder for chaining.
*/
public Builder clearFeeDelegation() {
feeDelegation_ = false;
onChanged();
return this;
}
private long gasUsed_ ;
/**
* uint64 gasUsed = 15;
* @return The gasUsed.
*/
@java.lang.Override
public long getGasUsed() {
return gasUsed_;
}
/**
* uint64 gasUsed = 15;
* @param value The gasUsed to set.
* @return This builder for chaining.
*/
public Builder setGasUsed(long value) {
gasUsed_ = value;
onChanged();
return this;
}
/**
* uint64 gasUsed = 15;
* @return This builder for chaining.
*/
public Builder clearGasUsed() {
gasUsed_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.Receipt)
}
// @@protoc_insertion_point(class_scope:types.Receipt)
private static final types.Blockchain.Receipt DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.Receipt();
}
public static types.Blockchain.Receipt getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Receipt parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Receipt(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.Receipt getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EventOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.Event)
com.google.protobuf.MessageOrBuilder {
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
com.google.protobuf.ByteString getContractAddress();
/**
* string eventName = 2;
* @return The eventName.
*/
java.lang.String getEventName();
/**
* string eventName = 2;
* @return The bytes for eventName.
*/
com.google.protobuf.ByteString
getEventNameBytes();
/**
* string jsonArgs = 3;
* @return The jsonArgs.
*/
java.lang.String getJsonArgs();
/**
* string jsonArgs = 3;
* @return The bytes for jsonArgs.
*/
com.google.protobuf.ByteString
getJsonArgsBytes();
/**
* int32 eventIdx = 4;
* @return The eventIdx.
*/
int getEventIdx();
/**
* bytes txHash = 5;
* @return The txHash.
*/
com.google.protobuf.ByteString getTxHash();
/**
* bytes blockHash = 6;
* @return The blockHash.
*/
com.google.protobuf.ByteString getBlockHash();
/**
* uint64 blockNo = 7;
* @return The blockNo.
*/
long getBlockNo();
/**
* int32 txIndex = 8;
* @return The txIndex.
*/
int getTxIndex();
}
/**
* Protobuf type {@code types.Event}
*/
public static final class Event extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.Event)
EventOrBuilder {
private static final long serialVersionUID = 0L;
// Use Event.newBuilder() to construct.
private Event(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Event() {
contractAddress_ = com.google.protobuf.ByteString.EMPTY;
eventName_ = "";
jsonArgs_ = "";
txHash_ = com.google.protobuf.ByteString.EMPTY;
blockHash_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Event();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Event(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
contractAddress_ = input.readBytes();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
eventName_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
jsonArgs_ = s;
break;
}
case 32: {
eventIdx_ = input.readInt32();
break;
}
case 42: {
txHash_ = input.readBytes();
break;
}
case 50: {
blockHash_ = input.readBytes();
break;
}
case 56: {
blockNo_ = input.readUInt64();
break;
}
case 64: {
txIndex_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_Event_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_Event_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.Event.class, types.Blockchain.Event.Builder.class);
}
public static final int CONTRACTADDRESS_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString contractAddress_;
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
@java.lang.Override
public com.google.protobuf.ByteString getContractAddress() {
return contractAddress_;
}
public static final int EVENTNAME_FIELD_NUMBER = 2;
private volatile java.lang.Object eventName_;
/**
* string eventName = 2;
* @return The eventName.
*/
@java.lang.Override
public java.lang.String getEventName() {
java.lang.Object ref = eventName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
eventName_ = s;
return s;
}
}
/**
* string eventName = 2;
* @return The bytes for eventName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getEventNameBytes() {
java.lang.Object ref = eventName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
eventName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JSONARGS_FIELD_NUMBER = 3;
private volatile java.lang.Object jsonArgs_;
/**
* string jsonArgs = 3;
* @return The jsonArgs.
*/
@java.lang.Override
public java.lang.String getJsonArgs() {
java.lang.Object ref = jsonArgs_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jsonArgs_ = s;
return s;
}
}
/**
* string jsonArgs = 3;
* @return The bytes for jsonArgs.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getJsonArgsBytes() {
java.lang.Object ref = jsonArgs_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jsonArgs_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EVENTIDX_FIELD_NUMBER = 4;
private int eventIdx_;
/**
* int32 eventIdx = 4;
* @return The eventIdx.
*/
@java.lang.Override
public int getEventIdx() {
return eventIdx_;
}
public static final int TXHASH_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString txHash_;
/**
* bytes txHash = 5;
* @return The txHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTxHash() {
return txHash_;
}
public static final int BLOCKHASH_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString blockHash_;
/**
* bytes blockHash = 6;
* @return The blockHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBlockHash() {
return blockHash_;
}
public static final int BLOCKNO_FIELD_NUMBER = 7;
private long blockNo_;
/**
* uint64 blockNo = 7;
* @return The blockNo.
*/
@java.lang.Override
public long getBlockNo() {
return blockNo_;
}
public static final int TXINDEX_FIELD_NUMBER = 8;
private int txIndex_;
/**
* int32 txIndex = 8;
* @return The txIndex.
*/
@java.lang.Override
public int getTxIndex() {
return txIndex_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!contractAddress_.isEmpty()) {
output.writeBytes(1, contractAddress_);
}
if (!getEventNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, eventName_);
}
if (!getJsonArgsBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, jsonArgs_);
}
if (eventIdx_ != 0) {
output.writeInt32(4, eventIdx_);
}
if (!txHash_.isEmpty()) {
output.writeBytes(5, txHash_);
}
if (!blockHash_.isEmpty()) {
output.writeBytes(6, blockHash_);
}
if (blockNo_ != 0L) {
output.writeUInt64(7, blockNo_);
}
if (txIndex_ != 0) {
output.writeInt32(8, txIndex_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!contractAddress_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, contractAddress_);
}
if (!getEventNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, eventName_);
}
if (!getJsonArgsBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, jsonArgs_);
}
if (eventIdx_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, eventIdx_);
}
if (!txHash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, txHash_);
}
if (!blockHash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, blockHash_);
}
if (blockNo_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(7, blockNo_);
}
if (txIndex_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, txIndex_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.Event)) {
return super.equals(obj);
}
types.Blockchain.Event other = (types.Blockchain.Event) obj;
if (!getContractAddress()
.equals(other.getContractAddress())) return false;
if (!getEventName()
.equals(other.getEventName())) return false;
if (!getJsonArgs()
.equals(other.getJsonArgs())) return false;
if (getEventIdx()
!= other.getEventIdx()) return false;
if (!getTxHash()
.equals(other.getTxHash())) return false;
if (!getBlockHash()
.equals(other.getBlockHash())) return false;
if (getBlockNo()
!= other.getBlockNo()) return false;
if (getTxIndex()
!= other.getTxIndex()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTRACTADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getContractAddress().hashCode();
hash = (37 * hash) + EVENTNAME_FIELD_NUMBER;
hash = (53 * hash) + getEventName().hashCode();
hash = (37 * hash) + JSONARGS_FIELD_NUMBER;
hash = (53 * hash) + getJsonArgs().hashCode();
hash = (37 * hash) + EVENTIDX_FIELD_NUMBER;
hash = (53 * hash) + getEventIdx();
hash = (37 * hash) + TXHASH_FIELD_NUMBER;
hash = (53 * hash) + getTxHash().hashCode();
hash = (37 * hash) + BLOCKHASH_FIELD_NUMBER;
hash = (53 * hash) + getBlockHash().hashCode();
hash = (37 * hash) + BLOCKNO_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBlockNo());
hash = (37 * hash) + TXINDEX_FIELD_NUMBER;
hash = (53 * hash) + getTxIndex();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.Event parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Event parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Event parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Event parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Event parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Event parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Event parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.Event parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.Event parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.Event parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.Event parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.Event parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.Event prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.Event}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.Event)
types.Blockchain.EventOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_Event_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_Event_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.Event.class, types.Blockchain.Event.Builder.class);
}
// Construct using types.Blockchain.Event.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
contractAddress_ = com.google.protobuf.ByteString.EMPTY;
eventName_ = "";
jsonArgs_ = "";
eventIdx_ = 0;
txHash_ = com.google.protobuf.ByteString.EMPTY;
blockHash_ = com.google.protobuf.ByteString.EMPTY;
blockNo_ = 0L;
txIndex_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_Event_descriptor;
}
@java.lang.Override
public types.Blockchain.Event getDefaultInstanceForType() {
return types.Blockchain.Event.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.Event build() {
types.Blockchain.Event result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.Event buildPartial() {
types.Blockchain.Event result = new types.Blockchain.Event(this);
result.contractAddress_ = contractAddress_;
result.eventName_ = eventName_;
result.jsonArgs_ = jsonArgs_;
result.eventIdx_ = eventIdx_;
result.txHash_ = txHash_;
result.blockHash_ = blockHash_;
result.blockNo_ = blockNo_;
result.txIndex_ = txIndex_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.Event) {
return mergeFrom((types.Blockchain.Event)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.Event other) {
if (other == types.Blockchain.Event.getDefaultInstance()) return this;
if (other.getContractAddress() != com.google.protobuf.ByteString.EMPTY) {
setContractAddress(other.getContractAddress());
}
if (!other.getEventName().isEmpty()) {
eventName_ = other.eventName_;
onChanged();
}
if (!other.getJsonArgs().isEmpty()) {
jsonArgs_ = other.jsonArgs_;
onChanged();
}
if (other.getEventIdx() != 0) {
setEventIdx(other.getEventIdx());
}
if (other.getTxHash() != com.google.protobuf.ByteString.EMPTY) {
setTxHash(other.getTxHash());
}
if (other.getBlockHash() != com.google.protobuf.ByteString.EMPTY) {
setBlockHash(other.getBlockHash());
}
if (other.getBlockNo() != 0L) {
setBlockNo(other.getBlockNo());
}
if (other.getTxIndex() != 0) {
setTxIndex(other.getTxIndex());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.Event parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.Event) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString contractAddress_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
@java.lang.Override
public com.google.protobuf.ByteString getContractAddress() {
return contractAddress_;
}
/**
* bytes contractAddress = 1;
* @param value The contractAddress to set.
* @return This builder for chaining.
*/
public Builder setContractAddress(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
contractAddress_ = value;
onChanged();
return this;
}
/**
* bytes contractAddress = 1;
* @return This builder for chaining.
*/
public Builder clearContractAddress() {
contractAddress_ = getDefaultInstance().getContractAddress();
onChanged();
return this;
}
private java.lang.Object eventName_ = "";
/**
* string eventName = 2;
* @return The eventName.
*/
public java.lang.String getEventName() {
java.lang.Object ref = eventName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
eventName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string eventName = 2;
* @return The bytes for eventName.
*/
public com.google.protobuf.ByteString
getEventNameBytes() {
java.lang.Object ref = eventName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
eventName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string eventName = 2;
* @param value The eventName to set.
* @return This builder for chaining.
*/
public Builder setEventName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
eventName_ = value;
onChanged();
return this;
}
/**
* string eventName = 2;
* @return This builder for chaining.
*/
public Builder clearEventName() {
eventName_ = getDefaultInstance().getEventName();
onChanged();
return this;
}
/**
* string eventName = 2;
* @param value The bytes for eventName to set.
* @return This builder for chaining.
*/
public Builder setEventNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
eventName_ = value;
onChanged();
return this;
}
private java.lang.Object jsonArgs_ = "";
/**
* string jsonArgs = 3;
* @return The jsonArgs.
*/
public java.lang.String getJsonArgs() {
java.lang.Object ref = jsonArgs_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jsonArgs_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string jsonArgs = 3;
* @return The bytes for jsonArgs.
*/
public com.google.protobuf.ByteString
getJsonArgsBytes() {
java.lang.Object ref = jsonArgs_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jsonArgs_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string jsonArgs = 3;
* @param value The jsonArgs to set.
* @return This builder for chaining.
*/
public Builder setJsonArgs(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
jsonArgs_ = value;
onChanged();
return this;
}
/**
* string jsonArgs = 3;
* @return This builder for chaining.
*/
public Builder clearJsonArgs() {
jsonArgs_ = getDefaultInstance().getJsonArgs();
onChanged();
return this;
}
/**
* string jsonArgs = 3;
* @param value The bytes for jsonArgs to set.
* @return This builder for chaining.
*/
public Builder setJsonArgsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
jsonArgs_ = value;
onChanged();
return this;
}
private int eventIdx_ ;
/**
* int32 eventIdx = 4;
* @return The eventIdx.
*/
@java.lang.Override
public int getEventIdx() {
return eventIdx_;
}
/**
* int32 eventIdx = 4;
* @param value The eventIdx to set.
* @return This builder for chaining.
*/
public Builder setEventIdx(int value) {
eventIdx_ = value;
onChanged();
return this;
}
/**
* int32 eventIdx = 4;
* @return This builder for chaining.
*/
public Builder clearEventIdx() {
eventIdx_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString txHash_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes txHash = 5;
* @return The txHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTxHash() {
return txHash_;
}
/**
* bytes txHash = 5;
* @param value The txHash to set.
* @return This builder for chaining.
*/
public Builder setTxHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
txHash_ = value;
onChanged();
return this;
}
/**
* bytes txHash = 5;
* @return This builder for chaining.
*/
public Builder clearTxHash() {
txHash_ = getDefaultInstance().getTxHash();
onChanged();
return this;
}
private com.google.protobuf.ByteString blockHash_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes blockHash = 6;
* @return The blockHash.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBlockHash() {
return blockHash_;
}
/**
* bytes blockHash = 6;
* @param value The blockHash to set.
* @return This builder for chaining.
*/
public Builder setBlockHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
blockHash_ = value;
onChanged();
return this;
}
/**
* bytes blockHash = 6;
* @return This builder for chaining.
*/
public Builder clearBlockHash() {
blockHash_ = getDefaultInstance().getBlockHash();
onChanged();
return this;
}
private long blockNo_ ;
/**
* uint64 blockNo = 7;
* @return The blockNo.
*/
@java.lang.Override
public long getBlockNo() {
return blockNo_;
}
/**
* uint64 blockNo = 7;
* @param value The blockNo to set.
* @return This builder for chaining.
*/
public Builder setBlockNo(long value) {
blockNo_ = value;
onChanged();
return this;
}
/**
* uint64 blockNo = 7;
* @return This builder for chaining.
*/
public Builder clearBlockNo() {
blockNo_ = 0L;
onChanged();
return this;
}
private int txIndex_ ;
/**
* int32 txIndex = 8;
* @return The txIndex.
*/
@java.lang.Override
public int getTxIndex() {
return txIndex_;
}
/**
* int32 txIndex = 8;
* @param value The txIndex to set.
* @return This builder for chaining.
*/
public Builder setTxIndex(int value) {
txIndex_ = value;
onChanged();
return this;
}
/**
* int32 txIndex = 8;
* @return This builder for chaining.
*/
public Builder clearTxIndex() {
txIndex_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.Event)
}
// @@protoc_insertion_point(class_scope:types.Event)
private static final types.Blockchain.Event DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.Event();
}
public static types.Blockchain.Event getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Event parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Event(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.Event getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FnArgumentOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.FnArgument)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
}
/**
* Protobuf type {@code types.FnArgument}
*/
public static final class FnArgument extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.FnArgument)
FnArgumentOrBuilder {
private static final long serialVersionUID = 0L;
// Use FnArgument.newBuilder() to construct.
private FnArgument(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FnArgument() {
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new FnArgument();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FnArgument(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_FnArgument_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_FnArgument_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.FnArgument.class, types.Blockchain.FnArgument.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.FnArgument)) {
return super.equals(obj);
}
types.Blockchain.FnArgument other = (types.Blockchain.FnArgument) obj;
if (!getName()
.equals(other.getName())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.FnArgument parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.FnArgument parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.FnArgument parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.FnArgument parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.FnArgument parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.FnArgument parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.FnArgument parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.FnArgument parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.FnArgument parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.FnArgument parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.FnArgument parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.FnArgument parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.FnArgument prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.FnArgument}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.FnArgument)
types.Blockchain.FnArgumentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_FnArgument_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_FnArgument_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.FnArgument.class, types.Blockchain.FnArgument.Builder.class);
}
// Construct using types.Blockchain.FnArgument.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_FnArgument_descriptor;
}
@java.lang.Override
public types.Blockchain.FnArgument getDefaultInstanceForType() {
return types.Blockchain.FnArgument.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.FnArgument build() {
types.Blockchain.FnArgument result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.FnArgument buildPartial() {
types.Blockchain.FnArgument result = new types.Blockchain.FnArgument(this);
result.name_ = name_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.FnArgument) {
return mergeFrom((types.Blockchain.FnArgument)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.FnArgument other) {
if (other == types.Blockchain.FnArgument.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.FnArgument parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.FnArgument) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.FnArgument)
}
// @@protoc_insertion_point(class_scope:types.FnArgument)
private static final types.Blockchain.FnArgument DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.FnArgument();
}
public static types.Blockchain.FnArgument getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FnArgument parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FnArgument(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.FnArgument getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FunctionOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.Function)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* repeated .types.FnArgument arguments = 2;
*/
java.util.List
getArgumentsList();
/**
* repeated .types.FnArgument arguments = 2;
*/
types.Blockchain.FnArgument getArguments(int index);
/**
* repeated .types.FnArgument arguments = 2;
*/
int getArgumentsCount();
/**
* repeated .types.FnArgument arguments = 2;
*/
java.util.List extends types.Blockchain.FnArgumentOrBuilder>
getArgumentsOrBuilderList();
/**
* repeated .types.FnArgument arguments = 2;
*/
types.Blockchain.FnArgumentOrBuilder getArgumentsOrBuilder(
int index);
/**
* bool payable = 3;
* @return The payable.
*/
boolean getPayable();
/**
* bool view = 4;
* @return The view.
*/
boolean getView();
/**
* bool fee_delegation = 5;
* @return The feeDelegation.
*/
boolean getFeeDelegation();
}
/**
* Protobuf type {@code types.Function}
*/
public static final class Function extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.Function)
FunctionOrBuilder {
private static final long serialVersionUID = 0L;
// Use Function.newBuilder() to construct.
private Function(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Function() {
name_ = "";
arguments_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Function();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Function(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
arguments_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
arguments_.add(
input.readMessage(types.Blockchain.FnArgument.parser(), extensionRegistry));
break;
}
case 24: {
payable_ = input.readBool();
break;
}
case 32: {
view_ = input.readBool();
break;
}
case 40: {
feeDelegation_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
arguments_ = java.util.Collections.unmodifiableList(arguments_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_Function_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_Function_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.Function.class, types.Blockchain.Function.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ARGUMENTS_FIELD_NUMBER = 2;
private java.util.List arguments_;
/**
* repeated .types.FnArgument arguments = 2;
*/
@java.lang.Override
public java.util.List getArgumentsList() {
return arguments_;
}
/**
* repeated .types.FnArgument arguments = 2;
*/
@java.lang.Override
public java.util.List extends types.Blockchain.FnArgumentOrBuilder>
getArgumentsOrBuilderList() {
return arguments_;
}
/**
* repeated .types.FnArgument arguments = 2;
*/
@java.lang.Override
public int getArgumentsCount() {
return arguments_.size();
}
/**
* repeated .types.FnArgument arguments = 2;
*/
@java.lang.Override
public types.Blockchain.FnArgument getArguments(int index) {
return arguments_.get(index);
}
/**
* repeated .types.FnArgument arguments = 2;
*/
@java.lang.Override
public types.Blockchain.FnArgumentOrBuilder getArgumentsOrBuilder(
int index) {
return arguments_.get(index);
}
public static final int PAYABLE_FIELD_NUMBER = 3;
private boolean payable_;
/**
* bool payable = 3;
* @return The payable.
*/
@java.lang.Override
public boolean getPayable() {
return payable_;
}
public static final int VIEW_FIELD_NUMBER = 4;
private boolean view_;
/**
* bool view = 4;
* @return The view.
*/
@java.lang.Override
public boolean getView() {
return view_;
}
public static final int FEE_DELEGATION_FIELD_NUMBER = 5;
private boolean feeDelegation_;
/**
* bool fee_delegation = 5;
* @return The feeDelegation.
*/
@java.lang.Override
public boolean getFeeDelegation() {
return feeDelegation_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
for (int i = 0; i < arguments_.size(); i++) {
output.writeMessage(2, arguments_.get(i));
}
if (payable_ != false) {
output.writeBool(3, payable_);
}
if (view_ != false) {
output.writeBool(4, view_);
}
if (feeDelegation_ != false) {
output.writeBool(5, feeDelegation_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
for (int i = 0; i < arguments_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, arguments_.get(i));
}
if (payable_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, payable_);
}
if (view_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, view_);
}
if (feeDelegation_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, feeDelegation_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.Function)) {
return super.equals(obj);
}
types.Blockchain.Function other = (types.Blockchain.Function) obj;
if (!getName()
.equals(other.getName())) return false;
if (!getArgumentsList()
.equals(other.getArgumentsList())) return false;
if (getPayable()
!= other.getPayable()) return false;
if (getView()
!= other.getView()) return false;
if (getFeeDelegation()
!= other.getFeeDelegation()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
if (getArgumentsCount() > 0) {
hash = (37 * hash) + ARGUMENTS_FIELD_NUMBER;
hash = (53 * hash) + getArgumentsList().hashCode();
}
hash = (37 * hash) + PAYABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getPayable());
hash = (37 * hash) + VIEW_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getView());
hash = (37 * hash) + FEE_DELEGATION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getFeeDelegation());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.Function parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Function parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Function parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Function parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Function parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Function parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Function parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.Function parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.Function parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.Function parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.Function parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.Function parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.Function prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.Function}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.Function)
types.Blockchain.FunctionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_Function_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_Function_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.Function.class, types.Blockchain.Function.Builder.class);
}
// Construct using types.Blockchain.Function.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getArgumentsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
if (argumentsBuilder_ == null) {
arguments_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
argumentsBuilder_.clear();
}
payable_ = false;
view_ = false;
feeDelegation_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_Function_descriptor;
}
@java.lang.Override
public types.Blockchain.Function getDefaultInstanceForType() {
return types.Blockchain.Function.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.Function build() {
types.Blockchain.Function result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.Function buildPartial() {
types.Blockchain.Function result = new types.Blockchain.Function(this);
int from_bitField0_ = bitField0_;
result.name_ = name_;
if (argumentsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
arguments_ = java.util.Collections.unmodifiableList(arguments_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.arguments_ = arguments_;
} else {
result.arguments_ = argumentsBuilder_.build();
}
result.payable_ = payable_;
result.view_ = view_;
result.feeDelegation_ = feeDelegation_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.Function) {
return mergeFrom((types.Blockchain.Function)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.Function other) {
if (other == types.Blockchain.Function.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (argumentsBuilder_ == null) {
if (!other.arguments_.isEmpty()) {
if (arguments_.isEmpty()) {
arguments_ = other.arguments_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureArgumentsIsMutable();
arguments_.addAll(other.arguments_);
}
onChanged();
}
} else {
if (!other.arguments_.isEmpty()) {
if (argumentsBuilder_.isEmpty()) {
argumentsBuilder_.dispose();
argumentsBuilder_ = null;
arguments_ = other.arguments_;
bitField0_ = (bitField0_ & ~0x00000001);
argumentsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getArgumentsFieldBuilder() : null;
} else {
argumentsBuilder_.addAllMessages(other.arguments_);
}
}
}
if (other.getPayable() != false) {
setPayable(other.getPayable());
}
if (other.getView() != false) {
setView(other.getView());
}
if (other.getFeeDelegation() != false) {
setFeeDelegation(other.getFeeDelegation());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.Function parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.Function) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private java.util.List arguments_ =
java.util.Collections.emptyList();
private void ensureArgumentsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
arguments_ = new java.util.ArrayList(arguments_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.FnArgument, types.Blockchain.FnArgument.Builder, types.Blockchain.FnArgumentOrBuilder> argumentsBuilder_;
/**
* repeated .types.FnArgument arguments = 2;
*/
public java.util.List getArgumentsList() {
if (argumentsBuilder_ == null) {
return java.util.Collections.unmodifiableList(arguments_);
} else {
return argumentsBuilder_.getMessageList();
}
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public int getArgumentsCount() {
if (argumentsBuilder_ == null) {
return arguments_.size();
} else {
return argumentsBuilder_.getCount();
}
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public types.Blockchain.FnArgument getArguments(int index) {
if (argumentsBuilder_ == null) {
return arguments_.get(index);
} else {
return argumentsBuilder_.getMessage(index);
}
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public Builder setArguments(
int index, types.Blockchain.FnArgument value) {
if (argumentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentsIsMutable();
arguments_.set(index, value);
onChanged();
} else {
argumentsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public Builder setArguments(
int index, types.Blockchain.FnArgument.Builder builderForValue) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
arguments_.set(index, builderForValue.build());
onChanged();
} else {
argumentsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public Builder addArguments(types.Blockchain.FnArgument value) {
if (argumentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentsIsMutable();
arguments_.add(value);
onChanged();
} else {
argumentsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public Builder addArguments(
int index, types.Blockchain.FnArgument value) {
if (argumentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentsIsMutable();
arguments_.add(index, value);
onChanged();
} else {
argumentsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public Builder addArguments(
types.Blockchain.FnArgument.Builder builderForValue) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
arguments_.add(builderForValue.build());
onChanged();
} else {
argumentsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public Builder addArguments(
int index, types.Blockchain.FnArgument.Builder builderForValue) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
arguments_.add(index, builderForValue.build());
onChanged();
} else {
argumentsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public Builder addAllArguments(
java.lang.Iterable extends types.Blockchain.FnArgument> values) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, arguments_);
onChanged();
} else {
argumentsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public Builder clearArguments() {
if (argumentsBuilder_ == null) {
arguments_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
argumentsBuilder_.clear();
}
return this;
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public Builder removeArguments(int index) {
if (argumentsBuilder_ == null) {
ensureArgumentsIsMutable();
arguments_.remove(index);
onChanged();
} else {
argumentsBuilder_.remove(index);
}
return this;
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public types.Blockchain.FnArgument.Builder getArgumentsBuilder(
int index) {
return getArgumentsFieldBuilder().getBuilder(index);
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public types.Blockchain.FnArgumentOrBuilder getArgumentsOrBuilder(
int index) {
if (argumentsBuilder_ == null) {
return arguments_.get(index); } else {
return argumentsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public java.util.List extends types.Blockchain.FnArgumentOrBuilder>
getArgumentsOrBuilderList() {
if (argumentsBuilder_ != null) {
return argumentsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(arguments_);
}
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public types.Blockchain.FnArgument.Builder addArgumentsBuilder() {
return getArgumentsFieldBuilder().addBuilder(
types.Blockchain.FnArgument.getDefaultInstance());
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public types.Blockchain.FnArgument.Builder addArgumentsBuilder(
int index) {
return getArgumentsFieldBuilder().addBuilder(
index, types.Blockchain.FnArgument.getDefaultInstance());
}
/**
* repeated .types.FnArgument arguments = 2;
*/
public java.util.List
getArgumentsBuilderList() {
return getArgumentsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.FnArgument, types.Blockchain.FnArgument.Builder, types.Blockchain.FnArgumentOrBuilder>
getArgumentsFieldBuilder() {
if (argumentsBuilder_ == null) {
argumentsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.FnArgument, types.Blockchain.FnArgument.Builder, types.Blockchain.FnArgumentOrBuilder>(
arguments_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
arguments_ = null;
}
return argumentsBuilder_;
}
private boolean payable_ ;
/**
* bool payable = 3;
* @return The payable.
*/
@java.lang.Override
public boolean getPayable() {
return payable_;
}
/**
* bool payable = 3;
* @param value The payable to set.
* @return This builder for chaining.
*/
public Builder setPayable(boolean value) {
payable_ = value;
onChanged();
return this;
}
/**
* bool payable = 3;
* @return This builder for chaining.
*/
public Builder clearPayable() {
payable_ = false;
onChanged();
return this;
}
private boolean view_ ;
/**
* bool view = 4;
* @return The view.
*/
@java.lang.Override
public boolean getView() {
return view_;
}
/**
* bool view = 4;
* @param value The view to set.
* @return This builder for chaining.
*/
public Builder setView(boolean value) {
view_ = value;
onChanged();
return this;
}
/**
* bool view = 4;
* @return This builder for chaining.
*/
public Builder clearView() {
view_ = false;
onChanged();
return this;
}
private boolean feeDelegation_ ;
/**
* bool fee_delegation = 5;
* @return The feeDelegation.
*/
@java.lang.Override
public boolean getFeeDelegation() {
return feeDelegation_;
}
/**
* bool fee_delegation = 5;
* @param value The feeDelegation to set.
* @return This builder for chaining.
*/
public Builder setFeeDelegation(boolean value) {
feeDelegation_ = value;
onChanged();
return this;
}
/**
* bool fee_delegation = 5;
* @return This builder for chaining.
*/
public Builder clearFeeDelegation() {
feeDelegation_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.Function)
}
// @@protoc_insertion_point(class_scope:types.Function)
private static final types.Blockchain.Function DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.Function();
}
public static types.Blockchain.Function getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Function parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Function(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.Function getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StateVarOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.StateVar)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* string type = 2;
* @return The type.
*/
java.lang.String getType();
/**
* string type = 2;
* @return The bytes for type.
*/
com.google.protobuf.ByteString
getTypeBytes();
/**
* int32 len = 3;
* @return The len.
*/
int getLen();
}
/**
* Protobuf type {@code types.StateVar}
*/
public static final class StateVar extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.StateVar)
StateVarOrBuilder {
private static final long serialVersionUID = 0L;
// Use StateVar.newBuilder() to construct.
private StateVar(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StateVar() {
name_ = "";
type_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StateVar();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StateVar(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
type_ = s;
break;
}
case 24: {
len_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_StateVar_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_StateVar_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.StateVar.class, types.Blockchain.StateVar.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 2;
private volatile java.lang.Object type_;
/**
* string type = 2;
* @return The type.
*/
@java.lang.Override
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
}
}
/**
* string type = 2;
* @return The bytes for type.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LEN_FIELD_NUMBER = 3;
private int len_;
/**
* int32 len = 3;
* @return The len.
*/
@java.lang.Override
public int getLen() {
return len_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!getTypeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, type_);
}
if (len_ != 0) {
output.writeInt32(3, len_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!getTypeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, type_);
}
if (len_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, len_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.StateVar)) {
return super.equals(obj);
}
types.Blockchain.StateVar other = (types.Blockchain.StateVar) obj;
if (!getName()
.equals(other.getName())) return false;
if (!getType()
.equals(other.getType())) return false;
if (getLen()
!= other.getLen()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
hash = (37 * hash) + LEN_FIELD_NUMBER;
hash = (53 * hash) + getLen();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.StateVar parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.StateVar parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.StateVar parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.StateVar parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.StateVar parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.StateVar parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.StateVar parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.StateVar parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.StateVar parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.StateVar parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.StateVar parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.StateVar parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.StateVar prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.StateVar}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.StateVar)
types.Blockchain.StateVarOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_StateVar_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_StateVar_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.StateVar.class, types.Blockchain.StateVar.Builder.class);
}
// Construct using types.Blockchain.StateVar.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
type_ = "";
len_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_StateVar_descriptor;
}
@java.lang.Override
public types.Blockchain.StateVar getDefaultInstanceForType() {
return types.Blockchain.StateVar.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.StateVar build() {
types.Blockchain.StateVar result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.StateVar buildPartial() {
types.Blockchain.StateVar result = new types.Blockchain.StateVar(this);
result.name_ = name_;
result.type_ = type_;
result.len_ = len_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.StateVar) {
return mergeFrom((types.Blockchain.StateVar)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.StateVar other) {
if (other == types.Blockchain.StateVar.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (!other.getType().isEmpty()) {
type_ = other.type_;
onChanged();
}
if (other.getLen() != 0) {
setLen(other.getLen());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.StateVar parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.StateVar) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private java.lang.Object type_ = "";
/**
* string type = 2;
* @return The type.
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string type = 2;
* @return The bytes for type.
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string type = 2;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value;
onChanged();
return this;
}
/**
* string type = 2;
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
/**
* string type = 2;
* @param value The bytes for type to set.
* @return This builder for chaining.
*/
public Builder setTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
type_ = value;
onChanged();
return this;
}
private int len_ ;
/**
* int32 len = 3;
* @return The len.
*/
@java.lang.Override
public int getLen() {
return len_;
}
/**
* int32 len = 3;
* @param value The len to set.
* @return This builder for chaining.
*/
public Builder setLen(int value) {
len_ = value;
onChanged();
return this;
}
/**
* int32 len = 3;
* @return This builder for chaining.
*/
public Builder clearLen() {
len_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.StateVar)
}
// @@protoc_insertion_point(class_scope:types.StateVar)
private static final types.Blockchain.StateVar DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.StateVar();
}
public static types.Blockchain.StateVar getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StateVar parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StateVar(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.StateVar getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ABIOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.ABI)
com.google.protobuf.MessageOrBuilder {
/**
* string version = 1;
* @return The version.
*/
java.lang.String getVersion();
/**
* string version = 1;
* @return The bytes for version.
*/
com.google.protobuf.ByteString
getVersionBytes();
/**
* string language = 2;
* @return The language.
*/
java.lang.String getLanguage();
/**
* string language = 2;
* @return The bytes for language.
*/
com.google.protobuf.ByteString
getLanguageBytes();
/**
* repeated .types.Function functions = 3;
*/
java.util.List
getFunctionsList();
/**
* repeated .types.Function functions = 3;
*/
types.Blockchain.Function getFunctions(int index);
/**
* repeated .types.Function functions = 3;
*/
int getFunctionsCount();
/**
* repeated .types.Function functions = 3;
*/
java.util.List extends types.Blockchain.FunctionOrBuilder>
getFunctionsOrBuilderList();
/**
* repeated .types.Function functions = 3;
*/
types.Blockchain.FunctionOrBuilder getFunctionsOrBuilder(
int index);
/**
* repeated .types.StateVar state_variables = 4;
*/
java.util.List
getStateVariablesList();
/**
* repeated .types.StateVar state_variables = 4;
*/
types.Blockchain.StateVar getStateVariables(int index);
/**
* repeated .types.StateVar state_variables = 4;
*/
int getStateVariablesCount();
/**
* repeated .types.StateVar state_variables = 4;
*/
java.util.List extends types.Blockchain.StateVarOrBuilder>
getStateVariablesOrBuilderList();
/**
* repeated .types.StateVar state_variables = 4;
*/
types.Blockchain.StateVarOrBuilder getStateVariablesOrBuilder(
int index);
}
/**
* Protobuf type {@code types.ABI}
*/
public static final class ABI extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.ABI)
ABIOrBuilder {
private static final long serialVersionUID = 0L;
// Use ABI.newBuilder() to construct.
private ABI(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ABI() {
version_ = "";
language_ = "";
functions_ = java.util.Collections.emptyList();
stateVariables_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ABI();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ABI(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
version_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
language_ = s;
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
functions_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
functions_.add(
input.readMessage(types.Blockchain.Function.parser(), extensionRegistry));
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
stateVariables_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
stateVariables_.add(
input.readMessage(types.Blockchain.StateVar.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
functions_ = java.util.Collections.unmodifiableList(functions_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
stateVariables_ = java.util.Collections.unmodifiableList(stateVariables_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_ABI_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_ABI_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.ABI.class, types.Blockchain.ABI.Builder.class);
}
public static final int VERSION_FIELD_NUMBER = 1;
private volatile java.lang.Object version_;
/**
* string version = 1;
* @return The version.
*/
@java.lang.Override
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
version_ = s;
return s;
}
}
/**
* string version = 1;
* @return The bytes for version.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LANGUAGE_FIELD_NUMBER = 2;
private volatile java.lang.Object language_;
/**
* string language = 2;
* @return The language.
*/
@java.lang.Override
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
language_ = s;
return s;
}
}
/**
* string language = 2;
* @return The bytes for language.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FUNCTIONS_FIELD_NUMBER = 3;
private java.util.List functions_;
/**
* repeated .types.Function functions = 3;
*/
@java.lang.Override
public java.util.List getFunctionsList() {
return functions_;
}
/**
* repeated .types.Function functions = 3;
*/
@java.lang.Override
public java.util.List extends types.Blockchain.FunctionOrBuilder>
getFunctionsOrBuilderList() {
return functions_;
}
/**
* repeated .types.Function functions = 3;
*/
@java.lang.Override
public int getFunctionsCount() {
return functions_.size();
}
/**
* repeated .types.Function functions = 3;
*/
@java.lang.Override
public types.Blockchain.Function getFunctions(int index) {
return functions_.get(index);
}
/**
* repeated .types.Function functions = 3;
*/
@java.lang.Override
public types.Blockchain.FunctionOrBuilder getFunctionsOrBuilder(
int index) {
return functions_.get(index);
}
public static final int STATE_VARIABLES_FIELD_NUMBER = 4;
private java.util.List stateVariables_;
/**
* repeated .types.StateVar state_variables = 4;
*/
@java.lang.Override
public java.util.List getStateVariablesList() {
return stateVariables_;
}
/**
* repeated .types.StateVar state_variables = 4;
*/
@java.lang.Override
public java.util.List extends types.Blockchain.StateVarOrBuilder>
getStateVariablesOrBuilderList() {
return stateVariables_;
}
/**
* repeated .types.StateVar state_variables = 4;
*/
@java.lang.Override
public int getStateVariablesCount() {
return stateVariables_.size();
}
/**
* repeated .types.StateVar state_variables = 4;
*/
@java.lang.Override
public types.Blockchain.StateVar getStateVariables(int index) {
return stateVariables_.get(index);
}
/**
* repeated .types.StateVar state_variables = 4;
*/
@java.lang.Override
public types.Blockchain.StateVarOrBuilder getStateVariablesOrBuilder(
int index) {
return stateVariables_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getVersionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, version_);
}
if (!getLanguageBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, language_);
}
for (int i = 0; i < functions_.size(); i++) {
output.writeMessage(3, functions_.get(i));
}
for (int i = 0; i < stateVariables_.size(); i++) {
output.writeMessage(4, stateVariables_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getVersionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, version_);
}
if (!getLanguageBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, language_);
}
for (int i = 0; i < functions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, functions_.get(i));
}
for (int i = 0; i < stateVariables_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, stateVariables_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.ABI)) {
return super.equals(obj);
}
types.Blockchain.ABI other = (types.Blockchain.ABI) obj;
if (!getVersion()
.equals(other.getVersion())) return false;
if (!getLanguage()
.equals(other.getLanguage())) return false;
if (!getFunctionsList()
.equals(other.getFunctionsList())) return false;
if (!getStateVariablesList()
.equals(other.getStateVariablesList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion().hashCode();
hash = (37 * hash) + LANGUAGE_FIELD_NUMBER;
hash = (53 * hash) + getLanguage().hashCode();
if (getFunctionsCount() > 0) {
hash = (37 * hash) + FUNCTIONS_FIELD_NUMBER;
hash = (53 * hash) + getFunctionsList().hashCode();
}
if (getStateVariablesCount() > 0) {
hash = (37 * hash) + STATE_VARIABLES_FIELD_NUMBER;
hash = (53 * hash) + getStateVariablesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.ABI parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.ABI parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.ABI parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.ABI parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.ABI parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.ABI parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.ABI parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.ABI parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.ABI parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.ABI parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.ABI parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.ABI parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.ABI prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.ABI}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.ABI)
types.Blockchain.ABIOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_ABI_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_ABI_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.ABI.class, types.Blockchain.ABI.Builder.class);
}
// Construct using types.Blockchain.ABI.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getFunctionsFieldBuilder();
getStateVariablesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
version_ = "";
language_ = "";
if (functionsBuilder_ == null) {
functions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
functionsBuilder_.clear();
}
if (stateVariablesBuilder_ == null) {
stateVariables_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
stateVariablesBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_ABI_descriptor;
}
@java.lang.Override
public types.Blockchain.ABI getDefaultInstanceForType() {
return types.Blockchain.ABI.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.ABI build() {
types.Blockchain.ABI result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.ABI buildPartial() {
types.Blockchain.ABI result = new types.Blockchain.ABI(this);
int from_bitField0_ = bitField0_;
result.version_ = version_;
result.language_ = language_;
if (functionsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
functions_ = java.util.Collections.unmodifiableList(functions_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.functions_ = functions_;
} else {
result.functions_ = functionsBuilder_.build();
}
if (stateVariablesBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
stateVariables_ = java.util.Collections.unmodifiableList(stateVariables_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.stateVariables_ = stateVariables_;
} else {
result.stateVariables_ = stateVariablesBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.ABI) {
return mergeFrom((types.Blockchain.ABI)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.ABI other) {
if (other == types.Blockchain.ABI.getDefaultInstance()) return this;
if (!other.getVersion().isEmpty()) {
version_ = other.version_;
onChanged();
}
if (!other.getLanguage().isEmpty()) {
language_ = other.language_;
onChanged();
}
if (functionsBuilder_ == null) {
if (!other.functions_.isEmpty()) {
if (functions_.isEmpty()) {
functions_ = other.functions_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFunctionsIsMutable();
functions_.addAll(other.functions_);
}
onChanged();
}
} else {
if (!other.functions_.isEmpty()) {
if (functionsBuilder_.isEmpty()) {
functionsBuilder_.dispose();
functionsBuilder_ = null;
functions_ = other.functions_;
bitField0_ = (bitField0_ & ~0x00000001);
functionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFunctionsFieldBuilder() : null;
} else {
functionsBuilder_.addAllMessages(other.functions_);
}
}
}
if (stateVariablesBuilder_ == null) {
if (!other.stateVariables_.isEmpty()) {
if (stateVariables_.isEmpty()) {
stateVariables_ = other.stateVariables_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureStateVariablesIsMutable();
stateVariables_.addAll(other.stateVariables_);
}
onChanged();
}
} else {
if (!other.stateVariables_.isEmpty()) {
if (stateVariablesBuilder_.isEmpty()) {
stateVariablesBuilder_.dispose();
stateVariablesBuilder_ = null;
stateVariables_ = other.stateVariables_;
bitField0_ = (bitField0_ & ~0x00000002);
stateVariablesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getStateVariablesFieldBuilder() : null;
} else {
stateVariablesBuilder_.addAllMessages(other.stateVariables_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.ABI parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.ABI) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object version_ = "";
/**
* string version = 1;
* @return The version.
*/
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
version_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string version = 1;
* @return The bytes for version.
*/
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string version = 1;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
version_ = value;
onChanged();
return this;
}
/**
* string version = 1;
* @return This builder for chaining.
*/
public Builder clearVersion() {
version_ = getDefaultInstance().getVersion();
onChanged();
return this;
}
/**
* string version = 1;
* @param value The bytes for version to set.
* @return This builder for chaining.
*/
public Builder setVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
version_ = value;
onChanged();
return this;
}
private java.lang.Object language_ = "";
/**
* string language = 2;
* @return The language.
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
language_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string language = 2;
* @return The bytes for language.
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string language = 2;
* @param value The language to set.
* @return This builder for chaining.
*/
public Builder setLanguage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
language_ = value;
onChanged();
return this;
}
/**
* string language = 2;
* @return This builder for chaining.
*/
public Builder clearLanguage() {
language_ = getDefaultInstance().getLanguage();
onChanged();
return this;
}
/**
* string language = 2;
* @param value The bytes for language to set.
* @return This builder for chaining.
*/
public Builder setLanguageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
language_ = value;
onChanged();
return this;
}
private java.util.List functions_ =
java.util.Collections.emptyList();
private void ensureFunctionsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
functions_ = new java.util.ArrayList(functions_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.Function, types.Blockchain.Function.Builder, types.Blockchain.FunctionOrBuilder> functionsBuilder_;
/**
* repeated .types.Function functions = 3;
*/
public java.util.List getFunctionsList() {
if (functionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(functions_);
} else {
return functionsBuilder_.getMessageList();
}
}
/**
* repeated .types.Function functions = 3;
*/
public int getFunctionsCount() {
if (functionsBuilder_ == null) {
return functions_.size();
} else {
return functionsBuilder_.getCount();
}
}
/**
* repeated .types.Function functions = 3;
*/
public types.Blockchain.Function getFunctions(int index) {
if (functionsBuilder_ == null) {
return functions_.get(index);
} else {
return functionsBuilder_.getMessage(index);
}
}
/**
* repeated .types.Function functions = 3;
*/
public Builder setFunctions(
int index, types.Blockchain.Function value) {
if (functionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFunctionsIsMutable();
functions_.set(index, value);
onChanged();
} else {
functionsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .types.Function functions = 3;
*/
public Builder setFunctions(
int index, types.Blockchain.Function.Builder builderForValue) {
if (functionsBuilder_ == null) {
ensureFunctionsIsMutable();
functions_.set(index, builderForValue.build());
onChanged();
} else {
functionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .types.Function functions = 3;
*/
public Builder addFunctions(types.Blockchain.Function value) {
if (functionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFunctionsIsMutable();
functions_.add(value);
onChanged();
} else {
functionsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .types.Function functions = 3;
*/
public Builder addFunctions(
int index, types.Blockchain.Function value) {
if (functionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFunctionsIsMutable();
functions_.add(index, value);
onChanged();
} else {
functionsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .types.Function functions = 3;
*/
public Builder addFunctions(
types.Blockchain.Function.Builder builderForValue) {
if (functionsBuilder_ == null) {
ensureFunctionsIsMutable();
functions_.add(builderForValue.build());
onChanged();
} else {
functionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .types.Function functions = 3;
*/
public Builder addFunctions(
int index, types.Blockchain.Function.Builder builderForValue) {
if (functionsBuilder_ == null) {
ensureFunctionsIsMutable();
functions_.add(index, builderForValue.build());
onChanged();
} else {
functionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .types.Function functions = 3;
*/
public Builder addAllFunctions(
java.lang.Iterable extends types.Blockchain.Function> values) {
if (functionsBuilder_ == null) {
ensureFunctionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, functions_);
onChanged();
} else {
functionsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .types.Function functions = 3;
*/
public Builder clearFunctions() {
if (functionsBuilder_ == null) {
functions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
functionsBuilder_.clear();
}
return this;
}
/**
* repeated .types.Function functions = 3;
*/
public Builder removeFunctions(int index) {
if (functionsBuilder_ == null) {
ensureFunctionsIsMutable();
functions_.remove(index);
onChanged();
} else {
functionsBuilder_.remove(index);
}
return this;
}
/**
* repeated .types.Function functions = 3;
*/
public types.Blockchain.Function.Builder getFunctionsBuilder(
int index) {
return getFunctionsFieldBuilder().getBuilder(index);
}
/**
* repeated .types.Function functions = 3;
*/
public types.Blockchain.FunctionOrBuilder getFunctionsOrBuilder(
int index) {
if (functionsBuilder_ == null) {
return functions_.get(index); } else {
return functionsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .types.Function functions = 3;
*/
public java.util.List extends types.Blockchain.FunctionOrBuilder>
getFunctionsOrBuilderList() {
if (functionsBuilder_ != null) {
return functionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(functions_);
}
}
/**
* repeated .types.Function functions = 3;
*/
public types.Blockchain.Function.Builder addFunctionsBuilder() {
return getFunctionsFieldBuilder().addBuilder(
types.Blockchain.Function.getDefaultInstance());
}
/**
* repeated .types.Function functions = 3;
*/
public types.Blockchain.Function.Builder addFunctionsBuilder(
int index) {
return getFunctionsFieldBuilder().addBuilder(
index, types.Blockchain.Function.getDefaultInstance());
}
/**
* repeated .types.Function functions = 3;
*/
public java.util.List
getFunctionsBuilderList() {
return getFunctionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.Function, types.Blockchain.Function.Builder, types.Blockchain.FunctionOrBuilder>
getFunctionsFieldBuilder() {
if (functionsBuilder_ == null) {
functionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.Function, types.Blockchain.Function.Builder, types.Blockchain.FunctionOrBuilder>(
functions_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
functions_ = null;
}
return functionsBuilder_;
}
private java.util.List stateVariables_ =
java.util.Collections.emptyList();
private void ensureStateVariablesIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
stateVariables_ = new java.util.ArrayList(stateVariables_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.StateVar, types.Blockchain.StateVar.Builder, types.Blockchain.StateVarOrBuilder> stateVariablesBuilder_;
/**
* repeated .types.StateVar state_variables = 4;
*/
public java.util.List getStateVariablesList() {
if (stateVariablesBuilder_ == null) {
return java.util.Collections.unmodifiableList(stateVariables_);
} else {
return stateVariablesBuilder_.getMessageList();
}
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public int getStateVariablesCount() {
if (stateVariablesBuilder_ == null) {
return stateVariables_.size();
} else {
return stateVariablesBuilder_.getCount();
}
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public types.Blockchain.StateVar getStateVariables(int index) {
if (stateVariablesBuilder_ == null) {
return stateVariables_.get(index);
} else {
return stateVariablesBuilder_.getMessage(index);
}
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public Builder setStateVariables(
int index, types.Blockchain.StateVar value) {
if (stateVariablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStateVariablesIsMutable();
stateVariables_.set(index, value);
onChanged();
} else {
stateVariablesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public Builder setStateVariables(
int index, types.Blockchain.StateVar.Builder builderForValue) {
if (stateVariablesBuilder_ == null) {
ensureStateVariablesIsMutable();
stateVariables_.set(index, builderForValue.build());
onChanged();
} else {
stateVariablesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public Builder addStateVariables(types.Blockchain.StateVar value) {
if (stateVariablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStateVariablesIsMutable();
stateVariables_.add(value);
onChanged();
} else {
stateVariablesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public Builder addStateVariables(
int index, types.Blockchain.StateVar value) {
if (stateVariablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStateVariablesIsMutable();
stateVariables_.add(index, value);
onChanged();
} else {
stateVariablesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public Builder addStateVariables(
types.Blockchain.StateVar.Builder builderForValue) {
if (stateVariablesBuilder_ == null) {
ensureStateVariablesIsMutable();
stateVariables_.add(builderForValue.build());
onChanged();
} else {
stateVariablesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public Builder addStateVariables(
int index, types.Blockchain.StateVar.Builder builderForValue) {
if (stateVariablesBuilder_ == null) {
ensureStateVariablesIsMutable();
stateVariables_.add(index, builderForValue.build());
onChanged();
} else {
stateVariablesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public Builder addAllStateVariables(
java.lang.Iterable extends types.Blockchain.StateVar> values) {
if (stateVariablesBuilder_ == null) {
ensureStateVariablesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, stateVariables_);
onChanged();
} else {
stateVariablesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public Builder clearStateVariables() {
if (stateVariablesBuilder_ == null) {
stateVariables_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
stateVariablesBuilder_.clear();
}
return this;
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public Builder removeStateVariables(int index) {
if (stateVariablesBuilder_ == null) {
ensureStateVariablesIsMutable();
stateVariables_.remove(index);
onChanged();
} else {
stateVariablesBuilder_.remove(index);
}
return this;
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public types.Blockchain.StateVar.Builder getStateVariablesBuilder(
int index) {
return getStateVariablesFieldBuilder().getBuilder(index);
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public types.Blockchain.StateVarOrBuilder getStateVariablesOrBuilder(
int index) {
if (stateVariablesBuilder_ == null) {
return stateVariables_.get(index); } else {
return stateVariablesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public java.util.List extends types.Blockchain.StateVarOrBuilder>
getStateVariablesOrBuilderList() {
if (stateVariablesBuilder_ != null) {
return stateVariablesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(stateVariables_);
}
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public types.Blockchain.StateVar.Builder addStateVariablesBuilder() {
return getStateVariablesFieldBuilder().addBuilder(
types.Blockchain.StateVar.getDefaultInstance());
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public types.Blockchain.StateVar.Builder addStateVariablesBuilder(
int index) {
return getStateVariablesFieldBuilder().addBuilder(
index, types.Blockchain.StateVar.getDefaultInstance());
}
/**
* repeated .types.StateVar state_variables = 4;
*/
public java.util.List
getStateVariablesBuilderList() {
return getStateVariablesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.StateVar, types.Blockchain.StateVar.Builder, types.Blockchain.StateVarOrBuilder>
getStateVariablesFieldBuilder() {
if (stateVariablesBuilder_ == null) {
stateVariablesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
types.Blockchain.StateVar, types.Blockchain.StateVar.Builder, types.Blockchain.StateVarOrBuilder>(
stateVariables_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
stateVariables_ = null;
}
return stateVariablesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.ABI)
}
// @@protoc_insertion_point(class_scope:types.ABI)
private static final types.Blockchain.ABI DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.ABI();
}
public static types.Blockchain.ABI getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ABI parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ABI(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.ABI getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.Query)
com.google.protobuf.MessageOrBuilder {
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
com.google.protobuf.ByteString getContractAddress();
/**
* bytes queryinfo = 2;
* @return The queryinfo.
*/
com.google.protobuf.ByteString getQueryinfo();
}
/**
* Protobuf type {@code types.Query}
*/
public static final class Query extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.Query)
QueryOrBuilder {
private static final long serialVersionUID = 0L;
// Use Query.newBuilder() to construct.
private Query(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Query() {
contractAddress_ = com.google.protobuf.ByteString.EMPTY;
queryinfo_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Query();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Query(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
contractAddress_ = input.readBytes();
break;
}
case 18: {
queryinfo_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_Query_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_Query_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.Query.class, types.Blockchain.Query.Builder.class);
}
public static final int CONTRACTADDRESS_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString contractAddress_;
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
@java.lang.Override
public com.google.protobuf.ByteString getContractAddress() {
return contractAddress_;
}
public static final int QUERYINFO_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString queryinfo_;
/**
* bytes queryinfo = 2;
* @return The queryinfo.
*/
@java.lang.Override
public com.google.protobuf.ByteString getQueryinfo() {
return queryinfo_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!contractAddress_.isEmpty()) {
output.writeBytes(1, contractAddress_);
}
if (!queryinfo_.isEmpty()) {
output.writeBytes(2, queryinfo_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!contractAddress_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, contractAddress_);
}
if (!queryinfo_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, queryinfo_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.Query)) {
return super.equals(obj);
}
types.Blockchain.Query other = (types.Blockchain.Query) obj;
if (!getContractAddress()
.equals(other.getContractAddress())) return false;
if (!getQueryinfo()
.equals(other.getQueryinfo())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTRACTADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getContractAddress().hashCode();
hash = (37 * hash) + QUERYINFO_FIELD_NUMBER;
hash = (53 * hash) + getQueryinfo().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.Query parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Query parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Query parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Query parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Query parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Query parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Query parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.Query parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.Query parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.Query parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.Query parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.Query parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.Query prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.Query}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.Query)
types.Blockchain.QueryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_Query_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_Query_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.Query.class, types.Blockchain.Query.Builder.class);
}
// Construct using types.Blockchain.Query.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
contractAddress_ = com.google.protobuf.ByteString.EMPTY;
queryinfo_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_Query_descriptor;
}
@java.lang.Override
public types.Blockchain.Query getDefaultInstanceForType() {
return types.Blockchain.Query.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.Query build() {
types.Blockchain.Query result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.Query buildPartial() {
types.Blockchain.Query result = new types.Blockchain.Query(this);
result.contractAddress_ = contractAddress_;
result.queryinfo_ = queryinfo_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.Query) {
return mergeFrom((types.Blockchain.Query)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.Query other) {
if (other == types.Blockchain.Query.getDefaultInstance()) return this;
if (other.getContractAddress() != com.google.protobuf.ByteString.EMPTY) {
setContractAddress(other.getContractAddress());
}
if (other.getQueryinfo() != com.google.protobuf.ByteString.EMPTY) {
setQueryinfo(other.getQueryinfo());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.Query parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.Query) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString contractAddress_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
@java.lang.Override
public com.google.protobuf.ByteString getContractAddress() {
return contractAddress_;
}
/**
* bytes contractAddress = 1;
* @param value The contractAddress to set.
* @return This builder for chaining.
*/
public Builder setContractAddress(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
contractAddress_ = value;
onChanged();
return this;
}
/**
* bytes contractAddress = 1;
* @return This builder for chaining.
*/
public Builder clearContractAddress() {
contractAddress_ = getDefaultInstance().getContractAddress();
onChanged();
return this;
}
private com.google.protobuf.ByteString queryinfo_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes queryinfo = 2;
* @return The queryinfo.
*/
@java.lang.Override
public com.google.protobuf.ByteString getQueryinfo() {
return queryinfo_;
}
/**
* bytes queryinfo = 2;
* @param value The queryinfo to set.
* @return This builder for chaining.
*/
public Builder setQueryinfo(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
queryinfo_ = value;
onChanged();
return this;
}
/**
* bytes queryinfo = 2;
* @return This builder for chaining.
*/
public Builder clearQueryinfo() {
queryinfo_ = getDefaultInstance().getQueryinfo();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.Query)
}
// @@protoc_insertion_point(class_scope:types.Query)
private static final types.Blockchain.Query DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.Query();
}
public static types.Blockchain.Query getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Query parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Query(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.Query getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StateQueryOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.StateQuery)
com.google.protobuf.MessageOrBuilder {
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
com.google.protobuf.ByteString getContractAddress();
/**
* bytes root = 3;
* @return The root.
*/
com.google.protobuf.ByteString getRoot();
/**
* bool compressed = 4;
* @return The compressed.
*/
boolean getCompressed();
/**
* repeated bytes storageKeys = 5;
* @return A list containing the storageKeys.
*/
java.util.List getStorageKeysList();
/**
* repeated bytes storageKeys = 5;
* @return The count of storageKeys.
*/
int getStorageKeysCount();
/**
* repeated bytes storageKeys = 5;
* @param index The index of the element to return.
* @return The storageKeys at the given index.
*/
com.google.protobuf.ByteString getStorageKeys(int index);
}
/**
* Protobuf type {@code types.StateQuery}
*/
public static final class StateQuery extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.StateQuery)
StateQueryOrBuilder {
private static final long serialVersionUID = 0L;
// Use StateQuery.newBuilder() to construct.
private StateQuery(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StateQuery() {
contractAddress_ = com.google.protobuf.ByteString.EMPTY;
root_ = com.google.protobuf.ByteString.EMPTY;
storageKeys_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StateQuery();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StateQuery(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
contractAddress_ = input.readBytes();
break;
}
case 26: {
root_ = input.readBytes();
break;
}
case 32: {
compressed_ = input.readBool();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
storageKeys_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
storageKeys_.add(input.readBytes());
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
storageKeys_ = java.util.Collections.unmodifiableList(storageKeys_); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_StateQuery_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_StateQuery_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.StateQuery.class, types.Blockchain.StateQuery.Builder.class);
}
public static final int CONTRACTADDRESS_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString contractAddress_;
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
@java.lang.Override
public com.google.protobuf.ByteString getContractAddress() {
return contractAddress_;
}
public static final int ROOT_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString root_;
/**
* bytes root = 3;
* @return The root.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRoot() {
return root_;
}
public static final int COMPRESSED_FIELD_NUMBER = 4;
private boolean compressed_;
/**
* bool compressed = 4;
* @return The compressed.
*/
@java.lang.Override
public boolean getCompressed() {
return compressed_;
}
public static final int STORAGEKEYS_FIELD_NUMBER = 5;
private java.util.List storageKeys_;
/**
* repeated bytes storageKeys = 5;
* @return A list containing the storageKeys.
*/
@java.lang.Override
public java.util.List
getStorageKeysList() {
return storageKeys_;
}
/**
* repeated bytes storageKeys = 5;
* @return The count of storageKeys.
*/
public int getStorageKeysCount() {
return storageKeys_.size();
}
/**
* repeated bytes storageKeys = 5;
* @param index The index of the element to return.
* @return The storageKeys at the given index.
*/
public com.google.protobuf.ByteString getStorageKeys(int index) {
return storageKeys_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!contractAddress_.isEmpty()) {
output.writeBytes(1, contractAddress_);
}
if (!root_.isEmpty()) {
output.writeBytes(3, root_);
}
if (compressed_ != false) {
output.writeBool(4, compressed_);
}
for (int i = 0; i < storageKeys_.size(); i++) {
output.writeBytes(5, storageKeys_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!contractAddress_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, contractAddress_);
}
if (!root_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, root_);
}
if (compressed_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, compressed_);
}
{
int dataSize = 0;
for (int i = 0; i < storageKeys_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(storageKeys_.get(i));
}
size += dataSize;
size += 1 * getStorageKeysList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.StateQuery)) {
return super.equals(obj);
}
types.Blockchain.StateQuery other = (types.Blockchain.StateQuery) obj;
if (!getContractAddress()
.equals(other.getContractAddress())) return false;
if (!getRoot()
.equals(other.getRoot())) return false;
if (getCompressed()
!= other.getCompressed()) return false;
if (!getStorageKeysList()
.equals(other.getStorageKeysList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTRACTADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getContractAddress().hashCode();
hash = (37 * hash) + ROOT_FIELD_NUMBER;
hash = (53 * hash) + getRoot().hashCode();
hash = (37 * hash) + COMPRESSED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getCompressed());
if (getStorageKeysCount() > 0) {
hash = (37 * hash) + STORAGEKEYS_FIELD_NUMBER;
hash = (53 * hash) + getStorageKeysList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.StateQuery parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.StateQuery parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.StateQuery parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.StateQuery parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.StateQuery parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.StateQuery parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.StateQuery parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.StateQuery parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.StateQuery parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.StateQuery parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.StateQuery parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.StateQuery parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.StateQuery prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.StateQuery}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.StateQuery)
types.Blockchain.StateQueryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_StateQuery_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_StateQuery_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.StateQuery.class, types.Blockchain.StateQuery.Builder.class);
}
// Construct using types.Blockchain.StateQuery.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
contractAddress_ = com.google.protobuf.ByteString.EMPTY;
root_ = com.google.protobuf.ByteString.EMPTY;
compressed_ = false;
storageKeys_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_StateQuery_descriptor;
}
@java.lang.Override
public types.Blockchain.StateQuery getDefaultInstanceForType() {
return types.Blockchain.StateQuery.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.StateQuery build() {
types.Blockchain.StateQuery result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.StateQuery buildPartial() {
types.Blockchain.StateQuery result = new types.Blockchain.StateQuery(this);
int from_bitField0_ = bitField0_;
result.contractAddress_ = contractAddress_;
result.root_ = root_;
result.compressed_ = compressed_;
if (((bitField0_ & 0x00000001) != 0)) {
storageKeys_ = java.util.Collections.unmodifiableList(storageKeys_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.storageKeys_ = storageKeys_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.StateQuery) {
return mergeFrom((types.Blockchain.StateQuery)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.StateQuery other) {
if (other == types.Blockchain.StateQuery.getDefaultInstance()) return this;
if (other.getContractAddress() != com.google.protobuf.ByteString.EMPTY) {
setContractAddress(other.getContractAddress());
}
if (other.getRoot() != com.google.protobuf.ByteString.EMPTY) {
setRoot(other.getRoot());
}
if (other.getCompressed() != false) {
setCompressed(other.getCompressed());
}
if (!other.storageKeys_.isEmpty()) {
if (storageKeys_.isEmpty()) {
storageKeys_ = other.storageKeys_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureStorageKeysIsMutable();
storageKeys_.addAll(other.storageKeys_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.StateQuery parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.StateQuery) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString contractAddress_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
@java.lang.Override
public com.google.protobuf.ByteString getContractAddress() {
return contractAddress_;
}
/**
* bytes contractAddress = 1;
* @param value The contractAddress to set.
* @return This builder for chaining.
*/
public Builder setContractAddress(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
contractAddress_ = value;
onChanged();
return this;
}
/**
* bytes contractAddress = 1;
* @return This builder for chaining.
*/
public Builder clearContractAddress() {
contractAddress_ = getDefaultInstance().getContractAddress();
onChanged();
return this;
}
private com.google.protobuf.ByteString root_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes root = 3;
* @return The root.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRoot() {
return root_;
}
/**
* bytes root = 3;
* @param value The root to set.
* @return This builder for chaining.
*/
public Builder setRoot(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
root_ = value;
onChanged();
return this;
}
/**
* bytes root = 3;
* @return This builder for chaining.
*/
public Builder clearRoot() {
root_ = getDefaultInstance().getRoot();
onChanged();
return this;
}
private boolean compressed_ ;
/**
* bool compressed = 4;
* @return The compressed.
*/
@java.lang.Override
public boolean getCompressed() {
return compressed_;
}
/**
* bool compressed = 4;
* @param value The compressed to set.
* @return This builder for chaining.
*/
public Builder setCompressed(boolean value) {
compressed_ = value;
onChanged();
return this;
}
/**
* bool compressed = 4;
* @return This builder for chaining.
*/
public Builder clearCompressed() {
compressed_ = false;
onChanged();
return this;
}
private java.util.List storageKeys_ = java.util.Collections.emptyList();
private void ensureStorageKeysIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
storageKeys_ = new java.util.ArrayList(storageKeys_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated bytes storageKeys = 5;
* @return A list containing the storageKeys.
*/
public java.util.List
getStorageKeysList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(storageKeys_) : storageKeys_;
}
/**
* repeated bytes storageKeys = 5;
* @return The count of storageKeys.
*/
public int getStorageKeysCount() {
return storageKeys_.size();
}
/**
* repeated bytes storageKeys = 5;
* @param index The index of the element to return.
* @return The storageKeys at the given index.
*/
public com.google.protobuf.ByteString getStorageKeys(int index) {
return storageKeys_.get(index);
}
/**
* repeated bytes storageKeys = 5;
* @param index The index to set the value at.
* @param value The storageKeys to set.
* @return This builder for chaining.
*/
public Builder setStorageKeys(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureStorageKeysIsMutable();
storageKeys_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes storageKeys = 5;
* @param value The storageKeys to add.
* @return This builder for chaining.
*/
public Builder addStorageKeys(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureStorageKeysIsMutable();
storageKeys_.add(value);
onChanged();
return this;
}
/**
* repeated bytes storageKeys = 5;
* @param values The storageKeys to add.
* @return This builder for chaining.
*/
public Builder addAllStorageKeys(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureStorageKeysIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, storageKeys_);
onChanged();
return this;
}
/**
* repeated bytes storageKeys = 5;
* @return This builder for chaining.
*/
public Builder clearStorageKeys() {
storageKeys_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.StateQuery)
}
// @@protoc_insertion_point(class_scope:types.StateQuery)
private static final types.Blockchain.StateQuery DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.StateQuery();
}
public static types.Blockchain.StateQuery getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StateQuery parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StateQuery(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.StateQuery getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FilterInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.FilterInfo)
com.google.protobuf.MessageOrBuilder {
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
com.google.protobuf.ByteString getContractAddress();
/**
* string eventName = 2;
* @return The eventName.
*/
java.lang.String getEventName();
/**
* string eventName = 2;
* @return The bytes for eventName.
*/
com.google.protobuf.ByteString
getEventNameBytes();
/**
* uint64 blockfrom = 3;
* @return The blockfrom.
*/
long getBlockfrom();
/**
* uint64 blockto = 4;
* @return The blockto.
*/
long getBlockto();
/**
* bool desc = 5;
* @return The desc.
*/
boolean getDesc();
/**
* bytes argFilter = 6;
* @return The argFilter.
*/
com.google.protobuf.ByteString getArgFilter();
/**
* int32 recentBlockCnt = 7;
* @return The recentBlockCnt.
*/
int getRecentBlockCnt();
}
/**
* Protobuf type {@code types.FilterInfo}
*/
public static final class FilterInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.FilterInfo)
FilterInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use FilterInfo.newBuilder() to construct.
private FilterInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FilterInfo() {
contractAddress_ = com.google.protobuf.ByteString.EMPTY;
eventName_ = "";
argFilter_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new FilterInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FilterInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
contractAddress_ = input.readBytes();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
eventName_ = s;
break;
}
case 24: {
blockfrom_ = input.readUInt64();
break;
}
case 32: {
blockto_ = input.readUInt64();
break;
}
case 40: {
desc_ = input.readBool();
break;
}
case 50: {
argFilter_ = input.readBytes();
break;
}
case 56: {
recentBlockCnt_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_FilterInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_FilterInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.FilterInfo.class, types.Blockchain.FilterInfo.Builder.class);
}
public static final int CONTRACTADDRESS_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString contractAddress_;
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
@java.lang.Override
public com.google.protobuf.ByteString getContractAddress() {
return contractAddress_;
}
public static final int EVENTNAME_FIELD_NUMBER = 2;
private volatile java.lang.Object eventName_;
/**
* string eventName = 2;
* @return The eventName.
*/
@java.lang.Override
public java.lang.String getEventName() {
java.lang.Object ref = eventName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
eventName_ = s;
return s;
}
}
/**
* string eventName = 2;
* @return The bytes for eventName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getEventNameBytes() {
java.lang.Object ref = eventName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
eventName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BLOCKFROM_FIELD_NUMBER = 3;
private long blockfrom_;
/**
* uint64 blockfrom = 3;
* @return The blockfrom.
*/
@java.lang.Override
public long getBlockfrom() {
return blockfrom_;
}
public static final int BLOCKTO_FIELD_NUMBER = 4;
private long blockto_;
/**
* uint64 blockto = 4;
* @return The blockto.
*/
@java.lang.Override
public long getBlockto() {
return blockto_;
}
public static final int DESC_FIELD_NUMBER = 5;
private boolean desc_;
/**
* bool desc = 5;
* @return The desc.
*/
@java.lang.Override
public boolean getDesc() {
return desc_;
}
public static final int ARGFILTER_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString argFilter_;
/**
* bytes argFilter = 6;
* @return The argFilter.
*/
@java.lang.Override
public com.google.protobuf.ByteString getArgFilter() {
return argFilter_;
}
public static final int RECENTBLOCKCNT_FIELD_NUMBER = 7;
private int recentBlockCnt_;
/**
* int32 recentBlockCnt = 7;
* @return The recentBlockCnt.
*/
@java.lang.Override
public int getRecentBlockCnt() {
return recentBlockCnt_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!contractAddress_.isEmpty()) {
output.writeBytes(1, contractAddress_);
}
if (!getEventNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, eventName_);
}
if (blockfrom_ != 0L) {
output.writeUInt64(3, blockfrom_);
}
if (blockto_ != 0L) {
output.writeUInt64(4, blockto_);
}
if (desc_ != false) {
output.writeBool(5, desc_);
}
if (!argFilter_.isEmpty()) {
output.writeBytes(6, argFilter_);
}
if (recentBlockCnt_ != 0) {
output.writeInt32(7, recentBlockCnt_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!contractAddress_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, contractAddress_);
}
if (!getEventNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, eventName_);
}
if (blockfrom_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, blockfrom_);
}
if (blockto_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, blockto_);
}
if (desc_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, desc_);
}
if (!argFilter_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, argFilter_);
}
if (recentBlockCnt_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(7, recentBlockCnt_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.FilterInfo)) {
return super.equals(obj);
}
types.Blockchain.FilterInfo other = (types.Blockchain.FilterInfo) obj;
if (!getContractAddress()
.equals(other.getContractAddress())) return false;
if (!getEventName()
.equals(other.getEventName())) return false;
if (getBlockfrom()
!= other.getBlockfrom()) return false;
if (getBlockto()
!= other.getBlockto()) return false;
if (getDesc()
!= other.getDesc()) return false;
if (!getArgFilter()
.equals(other.getArgFilter())) return false;
if (getRecentBlockCnt()
!= other.getRecentBlockCnt()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTRACTADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getContractAddress().hashCode();
hash = (37 * hash) + EVENTNAME_FIELD_NUMBER;
hash = (53 * hash) + getEventName().hashCode();
hash = (37 * hash) + BLOCKFROM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBlockfrom());
hash = (37 * hash) + BLOCKTO_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBlockto());
hash = (37 * hash) + DESC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getDesc());
hash = (37 * hash) + ARGFILTER_FIELD_NUMBER;
hash = (53 * hash) + getArgFilter().hashCode();
hash = (37 * hash) + RECENTBLOCKCNT_FIELD_NUMBER;
hash = (53 * hash) + getRecentBlockCnt();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.FilterInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.FilterInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.FilterInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.FilterInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.FilterInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.FilterInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.FilterInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.FilterInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.FilterInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.FilterInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.FilterInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.FilterInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.FilterInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.FilterInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.FilterInfo)
types.Blockchain.FilterInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_FilterInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_FilterInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.FilterInfo.class, types.Blockchain.FilterInfo.Builder.class);
}
// Construct using types.Blockchain.FilterInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
contractAddress_ = com.google.protobuf.ByteString.EMPTY;
eventName_ = "";
blockfrom_ = 0L;
blockto_ = 0L;
desc_ = false;
argFilter_ = com.google.protobuf.ByteString.EMPTY;
recentBlockCnt_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_FilterInfo_descriptor;
}
@java.lang.Override
public types.Blockchain.FilterInfo getDefaultInstanceForType() {
return types.Blockchain.FilterInfo.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.FilterInfo build() {
types.Blockchain.FilterInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.FilterInfo buildPartial() {
types.Blockchain.FilterInfo result = new types.Blockchain.FilterInfo(this);
result.contractAddress_ = contractAddress_;
result.eventName_ = eventName_;
result.blockfrom_ = blockfrom_;
result.blockto_ = blockto_;
result.desc_ = desc_;
result.argFilter_ = argFilter_;
result.recentBlockCnt_ = recentBlockCnt_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.FilterInfo) {
return mergeFrom((types.Blockchain.FilterInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.FilterInfo other) {
if (other == types.Blockchain.FilterInfo.getDefaultInstance()) return this;
if (other.getContractAddress() != com.google.protobuf.ByteString.EMPTY) {
setContractAddress(other.getContractAddress());
}
if (!other.getEventName().isEmpty()) {
eventName_ = other.eventName_;
onChanged();
}
if (other.getBlockfrom() != 0L) {
setBlockfrom(other.getBlockfrom());
}
if (other.getBlockto() != 0L) {
setBlockto(other.getBlockto());
}
if (other.getDesc() != false) {
setDesc(other.getDesc());
}
if (other.getArgFilter() != com.google.protobuf.ByteString.EMPTY) {
setArgFilter(other.getArgFilter());
}
if (other.getRecentBlockCnt() != 0) {
setRecentBlockCnt(other.getRecentBlockCnt());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.FilterInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.FilterInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString contractAddress_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes contractAddress = 1;
* @return The contractAddress.
*/
@java.lang.Override
public com.google.protobuf.ByteString getContractAddress() {
return contractAddress_;
}
/**
* bytes contractAddress = 1;
* @param value The contractAddress to set.
* @return This builder for chaining.
*/
public Builder setContractAddress(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
contractAddress_ = value;
onChanged();
return this;
}
/**
* bytes contractAddress = 1;
* @return This builder for chaining.
*/
public Builder clearContractAddress() {
contractAddress_ = getDefaultInstance().getContractAddress();
onChanged();
return this;
}
private java.lang.Object eventName_ = "";
/**
* string eventName = 2;
* @return The eventName.
*/
public java.lang.String getEventName() {
java.lang.Object ref = eventName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
eventName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string eventName = 2;
* @return The bytes for eventName.
*/
public com.google.protobuf.ByteString
getEventNameBytes() {
java.lang.Object ref = eventName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
eventName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string eventName = 2;
* @param value The eventName to set.
* @return This builder for chaining.
*/
public Builder setEventName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
eventName_ = value;
onChanged();
return this;
}
/**
* string eventName = 2;
* @return This builder for chaining.
*/
public Builder clearEventName() {
eventName_ = getDefaultInstance().getEventName();
onChanged();
return this;
}
/**
* string eventName = 2;
* @param value The bytes for eventName to set.
* @return This builder for chaining.
*/
public Builder setEventNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
eventName_ = value;
onChanged();
return this;
}
private long blockfrom_ ;
/**
* uint64 blockfrom = 3;
* @return The blockfrom.
*/
@java.lang.Override
public long getBlockfrom() {
return blockfrom_;
}
/**
* uint64 blockfrom = 3;
* @param value The blockfrom to set.
* @return This builder for chaining.
*/
public Builder setBlockfrom(long value) {
blockfrom_ = value;
onChanged();
return this;
}
/**
* uint64 blockfrom = 3;
* @return This builder for chaining.
*/
public Builder clearBlockfrom() {
blockfrom_ = 0L;
onChanged();
return this;
}
private long blockto_ ;
/**
* uint64 blockto = 4;
* @return The blockto.
*/
@java.lang.Override
public long getBlockto() {
return blockto_;
}
/**
* uint64 blockto = 4;
* @param value The blockto to set.
* @return This builder for chaining.
*/
public Builder setBlockto(long value) {
blockto_ = value;
onChanged();
return this;
}
/**
* uint64 blockto = 4;
* @return This builder for chaining.
*/
public Builder clearBlockto() {
blockto_ = 0L;
onChanged();
return this;
}
private boolean desc_ ;
/**
* bool desc = 5;
* @return The desc.
*/
@java.lang.Override
public boolean getDesc() {
return desc_;
}
/**
* bool desc = 5;
* @param value The desc to set.
* @return This builder for chaining.
*/
public Builder setDesc(boolean value) {
desc_ = value;
onChanged();
return this;
}
/**
* bool desc = 5;
* @return This builder for chaining.
*/
public Builder clearDesc() {
desc_ = false;
onChanged();
return this;
}
private com.google.protobuf.ByteString argFilter_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes argFilter = 6;
* @return The argFilter.
*/
@java.lang.Override
public com.google.protobuf.ByteString getArgFilter() {
return argFilter_;
}
/**
* bytes argFilter = 6;
* @param value The argFilter to set.
* @return This builder for chaining.
*/
public Builder setArgFilter(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
argFilter_ = value;
onChanged();
return this;
}
/**
* bytes argFilter = 6;
* @return This builder for chaining.
*/
public Builder clearArgFilter() {
argFilter_ = getDefaultInstance().getArgFilter();
onChanged();
return this;
}
private int recentBlockCnt_ ;
/**
* int32 recentBlockCnt = 7;
* @return The recentBlockCnt.
*/
@java.lang.Override
public int getRecentBlockCnt() {
return recentBlockCnt_;
}
/**
* int32 recentBlockCnt = 7;
* @param value The recentBlockCnt to set.
* @return This builder for chaining.
*/
public Builder setRecentBlockCnt(int value) {
recentBlockCnt_ = value;
onChanged();
return this;
}
/**
* int32 recentBlockCnt = 7;
* @return This builder for chaining.
*/
public Builder clearRecentBlockCnt() {
recentBlockCnt_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.FilterInfo)
}
// @@protoc_insertion_point(class_scope:types.FilterInfo)
private static final types.Blockchain.FilterInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.FilterInfo();
}
public static types.Blockchain.FilterInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FilterInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FilterInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.FilterInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProposalOrBuilder extends
// @@protoc_insertion_point(interface_extends:types.Proposal)
com.google.protobuf.MessageOrBuilder {
/**
* string id = 1;
* @return The id.
*/
java.lang.String getId();
/**
* string id = 1;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
* string description = 3;
* @return The description.
*/
java.lang.String getDescription();
/**
* string description = 3;
* @return The bytes for description.
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
* uint32 multipleChoice = 6;
* @return The multipleChoice.
*/
int getMultipleChoice();
}
/**
* Protobuf type {@code types.Proposal}
*/
public static final class Proposal extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:types.Proposal)
ProposalOrBuilder {
private static final long serialVersionUID = 0L;
// Use Proposal.newBuilder() to construct.
private Proposal(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Proposal() {
id_ = "";
description_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Proposal();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Proposal(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
case 48: {
multipleChoice_ = input.readUInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_Proposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_Proposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.Proposal.class, types.Blockchain.Proposal.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
* string id = 1;
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
* string id = 1;
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 3;
private volatile java.lang.Object description_;
/**
* string description = 3;
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
* string description = 3;
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MULTIPLECHOICE_FIELD_NUMBER = 6;
private int multipleChoice_;
/**
* uint32 multipleChoice = 6;
* @return The multipleChoice.
*/
@java.lang.Override
public int getMultipleChoice() {
return multipleChoice_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, description_);
}
if (multipleChoice_ != 0) {
output.writeUInt32(6, multipleChoice_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, description_);
}
if (multipleChoice_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(6, multipleChoice_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof types.Blockchain.Proposal)) {
return super.equals(obj);
}
types.Blockchain.Proposal other = (types.Blockchain.Proposal) obj;
if (!getId()
.equals(other.getId())) return false;
if (!getDescription()
.equals(other.getDescription())) return false;
if (getMultipleChoice()
!= other.getMultipleChoice()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (37 * hash) + MULTIPLECHOICE_FIELD_NUMBER;
hash = (53 * hash) + getMultipleChoice();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static types.Blockchain.Proposal parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Proposal parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Proposal parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Proposal parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Proposal parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static types.Blockchain.Proposal parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static types.Blockchain.Proposal parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.Proposal parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.Proposal parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static types.Blockchain.Proposal parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static types.Blockchain.Proposal parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static types.Blockchain.Proposal parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(types.Blockchain.Proposal prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code types.Proposal}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:types.Proposal)
types.Blockchain.ProposalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return types.Blockchain.internal_static_types_Proposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return types.Blockchain.internal_static_types_Proposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
types.Blockchain.Proposal.class, types.Blockchain.Proposal.Builder.class);
}
// Construct using types.Blockchain.Proposal.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
description_ = "";
multipleChoice_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return types.Blockchain.internal_static_types_Proposal_descriptor;
}
@java.lang.Override
public types.Blockchain.Proposal getDefaultInstanceForType() {
return types.Blockchain.Proposal.getDefaultInstance();
}
@java.lang.Override
public types.Blockchain.Proposal build() {
types.Blockchain.Proposal result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public types.Blockchain.Proposal buildPartial() {
types.Blockchain.Proposal result = new types.Blockchain.Proposal(this);
result.id_ = id_;
result.description_ = description_;
result.multipleChoice_ = multipleChoice_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof types.Blockchain.Proposal) {
return mergeFrom((types.Blockchain.Proposal)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(types.Blockchain.Proposal other) {
if (other == types.Blockchain.Proposal.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
if (other.getMultipleChoice() != 0) {
setMultipleChoice(other.getMultipleChoice());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
types.Blockchain.Proposal parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (types.Blockchain.Proposal) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object id_ = "";
/**
* string id = 1;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string id = 1;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
* string id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* string id = 1;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
* string description = 3;
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string description = 3;
* @return The bytes for description.
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string description = 3;
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
* string description = 3;
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
* string description = 3;
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
private int multipleChoice_ ;
/**
* uint32 multipleChoice = 6;
* @return The multipleChoice.
*/
@java.lang.Override
public int getMultipleChoice() {
return multipleChoice_;
}
/**
* uint32 multipleChoice = 6;
* @param value The multipleChoice to set.
* @return This builder for chaining.
*/
public Builder setMultipleChoice(int value) {
multipleChoice_ = value;
onChanged();
return this;
}
/**
* uint32 multipleChoice = 6;
* @return This builder for chaining.
*/
public Builder clearMultipleChoice() {
multipleChoice_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:types.Proposal)
}
// @@protoc_insertion_point(class_scope:types.Proposal)
private static final types.Blockchain.Proposal DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new types.Blockchain.Proposal();
}
public static types.Blockchain.Proposal getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Proposal parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Proposal(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public types.Blockchain.Proposal getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_Block_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_Block_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_BlockHeader_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_BlockHeader_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_BlockBody_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_BlockBody_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_TxList_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_TxList_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_Tx_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_Tx_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_TxBody_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_TxBody_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_TxIdx_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_TxIdx_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_TxInBlock_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_TxInBlock_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_State_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_State_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_AccountProof_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_AccountProof_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_ContractVarProof_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_ContractVarProof_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_StateQueryProof_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_StateQueryProof_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_Receipt_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_Receipt_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_Event_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_Event_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_FnArgument_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_FnArgument_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_Function_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_Function_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_StateVar_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_StateVar_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_ABI_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_ABI_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_Query_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_Query_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_StateQuery_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_StateQuery_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_FilterInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_FilterInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_types_Proposal_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_types_Proposal_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\020blockchain.proto\022\005types\"Y\n\005Block\022\014\n\004ha" +
"sh\030\001 \001(\014\022\"\n\006header\030\002 \001(\0132\022.types.BlockHe" +
"ader\022\036\n\004body\030\003 \001(\0132\020.types.BlockBody\"\374\001\n" +
"\013BlockHeader\022\017\n\007chainID\030\001 \001(\014\022\025\n\rprevBlo" +
"ckHash\030\002 \001(\014\022\017\n\007blockNo\030\003 \001(\004\022\021\n\ttimesta" +
"mp\030\004 \001(\003\022\026\n\016blocksRootHash\030\005 \001(\014\022\023\n\013txsR" +
"ootHash\030\006 \001(\014\022\030\n\020receiptsRootHash\030\007 \001(\014\022" +
"\020\n\010confirms\030\010 \001(\004\022\016\n\006pubKey\030\t \001(\014\022\027\n\017coi" +
"nbaseAccount\030\n \001(\014\022\014\n\004sign\030\013 \001(\014\022\021\n\tcons" +
"ensus\030\014 \001(\014\"#\n\tBlockBody\022\026\n\003txs\030\001 \003(\0132\t." +
"types.Tx\" \n\006TxList\022\026\n\003txs\030\001 \003(\0132\t.types." +
"Tx\"/\n\002Tx\022\014\n\004hash\030\001 \001(\014\022\033\n\004body\030\002 \001(\0132\r.t" +
"ypes.TxBody\"\300\001\n\006TxBody\022\r\n\005nonce\030\001 \001(\004\022\017\n" +
"\007account\030\002 \001(\014\022\021\n\trecipient\030\003 \001(\014\022\016\n\006amo" +
"unt\030\004 \001(\014\022\017\n\007payload\030\005 \001(\014\022\020\n\010gasLimit\030\006" +
" \001(\004\022\020\n\010gasPrice\030\007 \001(\014\022\033\n\004type\030\010 \001(\0162\r.t" +
"ypes.TxType\022\023\n\013chainIdHash\030\t \001(\014\022\014\n\004sign" +
"\030\n \001(\014\"\'\n\005TxIdx\022\021\n\tblockHash\030\001 \001(\014\022\013\n\003id" +
"x\030\002 \001(\005\"?\n\tTxInBlock\022\033\n\005txIdx\030\001 \001(\0132\014.ty" +
"pes.TxIdx\022\025\n\002tx\030\002 \001(\0132\t.types.Tx\"h\n\005Stat" +
"e\022\r\n\005nonce\030\001 \001(\004\022\017\n\007balance\030\002 \001(\014\022\020\n\010cod" +
"eHash\030\003 \001(\014\022\023\n\013storageRoot\030\004 \001(\014\022\030\n\020sqlR" +
"ecoveryPoint\030\005 \001(\004\"\242\001\n\014AccountProof\022\033\n\005s" +
"tate\030\001 \001(\0132\014.types.State\022\021\n\tinclusion\030\002 " +
"\001(\010\022\013\n\003key\030\003 \001(\014\022\020\n\010proofKey\030\004 \001(\014\022\020\n\010pr" +
"oofVal\030\005 \001(\014\022\016\n\006bitmap\030\006 \001(\014\022\016\n\006height\030\007" +
" \001(\r\022\021\n\tauditPath\030\010 \003(\014\"\236\001\n\020ContractVarP" +
"roof\022\r\n\005value\030\001 \001(\014\022\021\n\tinclusion\030\002 \001(\010\022\020" +
"\n\010proofKey\030\004 \001(\014\022\020\n\010proofVal\030\005 \001(\014\022\016\n\006bi" +
"tmap\030\006 \001(\014\022\016\n\006height\030\007 \001(\r\022\021\n\tauditPath\030" +
"\010 \003(\014\022\013\n\003key\030\t \001(\014J\004\010\003\020\004\"i\n\017StateQueryPr" +
"oof\022*\n\rcontractProof\030\001 \001(\0132\023.types.Accou" +
"ntProof\022*\n\tvarProofs\030\002 \003(\0132\027.types.Contr" +
"actVarProof\"\237\002\n\007Receipt\022\027\n\017contractAddre" +
"ss\030\001 \001(\014\022\016\n\006status\030\002 \001(\t\022\013\n\003ret\030\003 \001(\t\022\016\n" +
"\006txHash\030\004 \001(\014\022\017\n\007feeUsed\030\005 \001(\014\022\031\n\021cumula" +
"tiveFeeUsed\030\006 \001(\014\022\r\n\005bloom\030\007 \001(\014\022\034\n\006even" +
"ts\030\010 \003(\0132\014.types.Event\022\017\n\007blockNo\030\t \001(\004\022" +
"\021\n\tblockHash\030\n \001(\014\022\017\n\007txIndex\030\013 \001(\005\022\014\n\004f" +
"rom\030\014 \001(\014\022\n\n\002to\030\r \001(\014\022\025\n\rfeeDelegation\030\016" +
" \001(\010\022\017\n\007gasUsed\030\017 \001(\004\"\234\001\n\005Event\022\027\n\017contr" +
"actAddress\030\001 \001(\014\022\021\n\teventName\030\002 \001(\t\022\020\n\010j" +
"sonArgs\030\003 \001(\t\022\020\n\010eventIdx\030\004 \001(\005\022\016\n\006txHas" +
"h\030\005 \001(\014\022\021\n\tblockHash\030\006 \001(\014\022\017\n\007blockNo\030\007 " +
"\001(\004\022\017\n\007txIndex\030\010 \001(\005\"\032\n\nFnArgument\022\014\n\004na" +
"me\030\001 \001(\t\"u\n\010Function\022\014\n\004name\030\001 \001(\t\022$\n\tar" +
"guments\030\002 \003(\0132\021.types.FnArgument\022\017\n\007paya" +
"ble\030\003 \001(\010\022\014\n\004view\030\004 \001(\010\022\026\n\016fee_delegatio" +
"n\030\005 \001(\010\"3\n\010StateVar\022\014\n\004name\030\001 \001(\t\022\014\n\004typ" +
"e\030\002 \001(\t\022\013\n\003len\030\003 \001(\005\"v\n\003ABI\022\017\n\007version\030\001" +
" \001(\t\022\020\n\010language\030\002 \001(\t\022\"\n\tfunctions\030\003 \003(" +
"\0132\017.types.Function\022(\n\017state_variables\030\004 " +
"\003(\0132\017.types.StateVar\"3\n\005Query\022\027\n\017contrac" +
"tAddress\030\001 \001(\014\022\021\n\tqueryinfo\030\002 \001(\014\"b\n\nSta" +
"teQuery\022\027\n\017contractAddress\030\001 \001(\014\022\014\n\004root" +
"\030\003 \001(\014\022\022\n\ncompressed\030\004 \001(\010\022\023\n\013storageKey" +
"s\030\005 \003(\014J\004\010\002\020\003\"\225\001\n\nFilterInfo\022\027\n\017contract" +
"Address\030\001 \001(\014\022\021\n\teventName\030\002 \001(\t\022\021\n\tbloc" +
"kfrom\030\003 \001(\004\022\017\n\007blockto\030\004 \001(\004\022\014\n\004desc\030\005 \001" +
"(\010\022\021\n\targFilter\030\006 \001(\014\022\026\n\016recentBlockCnt\030" +
"\007 \001(\005\"C\n\010Proposal\022\n\n\002id\030\001 \001(\t\022\023\n\013descrip" +
"tion\030\003 \001(\t\022\026\n\016multipleChoice\030\006 \001(\r*i\n\006Tx" +
"Type\022\n\n\006NORMAL\020\000\022\016\n\nGOVERNANCE\020\001\022\014\n\010REDE" +
"PLOY\020\002\022\021\n\rFEEDELEGATION\020\003\022\014\n\010TRANSFER\020\004\022" +
"\010\n\004CALL\020\005\022\n\n\006DEPLOY\020\006b\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_types_Block_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_types_Block_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_Block_descriptor,
new java.lang.String[] { "Hash", "Header", "Body", });
internal_static_types_BlockHeader_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_types_BlockHeader_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_BlockHeader_descriptor,
new java.lang.String[] { "ChainID", "PrevBlockHash", "BlockNo", "Timestamp", "BlocksRootHash", "TxsRootHash", "ReceiptsRootHash", "Confirms", "PubKey", "CoinbaseAccount", "Sign", "Consensus", });
internal_static_types_BlockBody_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_types_BlockBody_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_BlockBody_descriptor,
new java.lang.String[] { "Txs", });
internal_static_types_TxList_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_types_TxList_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_TxList_descriptor,
new java.lang.String[] { "Txs", });
internal_static_types_Tx_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_types_Tx_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_Tx_descriptor,
new java.lang.String[] { "Hash", "Body", });
internal_static_types_TxBody_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_types_TxBody_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_TxBody_descriptor,
new java.lang.String[] { "Nonce", "Account", "Recipient", "Amount", "Payload", "GasLimit", "GasPrice", "Type", "ChainIdHash", "Sign", });
internal_static_types_TxIdx_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_types_TxIdx_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_TxIdx_descriptor,
new java.lang.String[] { "BlockHash", "Idx", });
internal_static_types_TxInBlock_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_types_TxInBlock_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_TxInBlock_descriptor,
new java.lang.String[] { "TxIdx", "Tx", });
internal_static_types_State_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_types_State_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_State_descriptor,
new java.lang.String[] { "Nonce", "Balance", "CodeHash", "StorageRoot", "SqlRecoveryPoint", });
internal_static_types_AccountProof_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_types_AccountProof_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_AccountProof_descriptor,
new java.lang.String[] { "State", "Inclusion", "Key", "ProofKey", "ProofVal", "Bitmap", "Height", "AuditPath", });
internal_static_types_ContractVarProof_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_types_ContractVarProof_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_ContractVarProof_descriptor,
new java.lang.String[] { "Value", "Inclusion", "ProofKey", "ProofVal", "Bitmap", "Height", "AuditPath", "Key", });
internal_static_types_StateQueryProof_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_types_StateQueryProof_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_StateQueryProof_descriptor,
new java.lang.String[] { "ContractProof", "VarProofs", });
internal_static_types_Receipt_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_types_Receipt_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_Receipt_descriptor,
new java.lang.String[] { "ContractAddress", "Status", "Ret", "TxHash", "FeeUsed", "CumulativeFeeUsed", "Bloom", "Events", "BlockNo", "BlockHash", "TxIndex", "From", "To", "FeeDelegation", "GasUsed", });
internal_static_types_Event_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_types_Event_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_Event_descriptor,
new java.lang.String[] { "ContractAddress", "EventName", "JsonArgs", "EventIdx", "TxHash", "BlockHash", "BlockNo", "TxIndex", });
internal_static_types_FnArgument_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_types_FnArgument_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_FnArgument_descriptor,
new java.lang.String[] { "Name", });
internal_static_types_Function_descriptor =
getDescriptor().getMessageTypes().get(15);
internal_static_types_Function_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_Function_descriptor,
new java.lang.String[] { "Name", "Arguments", "Payable", "View", "FeeDelegation", });
internal_static_types_StateVar_descriptor =
getDescriptor().getMessageTypes().get(16);
internal_static_types_StateVar_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_StateVar_descriptor,
new java.lang.String[] { "Name", "Type", "Len", });
internal_static_types_ABI_descriptor =
getDescriptor().getMessageTypes().get(17);
internal_static_types_ABI_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_ABI_descriptor,
new java.lang.String[] { "Version", "Language", "Functions", "StateVariables", });
internal_static_types_Query_descriptor =
getDescriptor().getMessageTypes().get(18);
internal_static_types_Query_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_Query_descriptor,
new java.lang.String[] { "ContractAddress", "Queryinfo", });
internal_static_types_StateQuery_descriptor =
getDescriptor().getMessageTypes().get(19);
internal_static_types_StateQuery_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_StateQuery_descriptor,
new java.lang.String[] { "ContractAddress", "Root", "Compressed", "StorageKeys", });
internal_static_types_FilterInfo_descriptor =
getDescriptor().getMessageTypes().get(20);
internal_static_types_FilterInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_FilterInfo_descriptor,
new java.lang.String[] { "ContractAddress", "EventName", "Blockfrom", "Blockto", "Desc", "ArgFilter", "RecentBlockCnt", });
internal_static_types_Proposal_descriptor =
getDescriptor().getMessageTypes().get(21);
internal_static_types_Proposal_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_types_Proposal_descriptor,
new java.lang.String[] { "Id", "Description", "MultipleChoice", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy