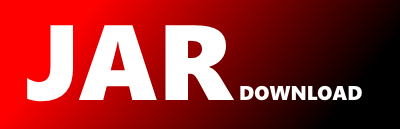
io.afu.utils.string.IdcardUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of utils Show documentation
Show all versions of utils Show documentation
RffanLAB Utils For Many Way use
package io.afu.utils.string;
import io.afu.utils.constant.CommonConstant;
import io.afu.utils.constant.ErrCodeConstant;
import io.afu.utils.constant.ErrMsgConstant;
import io.afu.utils.datetime.DateUtils;
import io.afu.utils.datetime.TimeUtils;
import io.afu.utils.exception.BaseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* 中国身份证号码助手
*/
public class IdcardUtils {
/**
* 18位身份证号识别助手,通过正则来的,该方法适用于中华人民共和95年起的方法,如果有所改变,需要自行修改。
* @param idcard 需要处理的身份证号
* @return Boolean true为是中国身份证,false为非中国身份证
*
*/
public static Boolean checkIfIsChineseIdcard(String idcard) throws BaseException{
int length = idcard.length();
if (length != 15 && length != 18){
throw new BaseException(ErrMsgConstant.IDCARD_ILLEGAL,ErrCodeConstant.IDCARD_ILLEGAL);
}
if (length == 15){
}
if (length ==18){
String patten = "^(11|12|13|14|15|21|22|23|31|32|33|34|35|36|37|41|42|43|44|45|46|51|52|52|54|50|61|62|63|64|65|71|81|82)\\d{4}([1-3][0-9])\\d{9}[\\d|X|x]$";
Pattern p = Pattern.compile(patten);
Matcher m = p.matcher(idcard);
if (m.find()){
System.out.println("idcard = [" + m.group() + "]");
return true;
}
}
return false;
}
/**
* 从身份证号上获取生日
* @param idcard 身份证号
* @return String 生日String
* @throws BaseException 抛出错误
*/
public static String getBirthFromIdcard(String idcard) throws BaseException{
if (checkIfIsChineseIdcard(idcard)){
return idcard.substring(6,14);
}
return null;
}
/**
* 获取身份证出生年份
* @param idcard 身份证
* @return String 出生年份
* @throws BaseException 抛出错误
*/
public static String getBirthYear(String idcard) throws BaseException{
if (idcard.length() == 18){
return idcard.substring(6,10);
}else if (idcard.length() == 15){
return "19"+idcard.substring(6,8);
}
throw new BaseException(ErrMsgConstant.IDCARD_ILLEGAL,ErrCodeConstant.IDCARD_ILLEGAL);
}
/**
* 判断年龄段
* @param idcard 身份证号
* @return 身份证所属年龄段
*/
public static String getAgeStage(String idcard) throws BaseException{
String thisYear = DateUtils.getThisYear();
String birthYear = getBirthYear(idcard);
if (thisYear != null && birthYear != null){
Integer disparity = Integer.valueOf(thisYear) - Integer.valueOf(birthYear);
if (disparity<0){
throw new BaseException(ErrMsgConstant.BIRTH_YEAR_ILLEGAL, ErrCodeConstant.BIRTH_YEAR_ILLEGAL);
}
if (disparity>0&&disparity<=10){
return "0-10";
}
if (disparity>10&&disparity<=20){
return "10-20";
}
if (disparity>20&&disparity<=30){
return "20-30";
}
if (disparity>30&&disparity<=40){
return "30-40";
}
if (disparity>40&&disparity<=50){
return "40-50";
}
if (disparity>50&&disparity<=60){
return "50-60";
}
if (disparity>60&&disparity<=70){
return "60-70";
}
if (disparity>70&&disparity<=80){
return "70-80";
}
if (disparity>80&&disparity<=90){
return "80-90";
}
return "other";
}
throw new BaseException(ErrMsgConstant.BIRTH_YEAR_ILLEGAL, ErrCodeConstant.BIRTH_YEAR_ILLEGAL);
}
/**
* 通过身份证判断男女
* @param idcard 身份证号码
* @return Integer 1为男2为女
* @throws BaseException 抛出错误
*/
public static Integer judgeGender(String idcard) throws BaseException {
String strGender = "";
if (idcard.length() == 18){
strGender = idcard.substring(16,17);
}else if (idcard.length() == 15){
strGender = idcard.substring(14,15);
}else {
throw new BaseException(ErrMsgConstant.IDCARD_ILLEGAL,ErrCodeConstant.IDCARD_ILLEGAL);
}
Integer intGender = Integer.valueOf(strGender);
if ((intGender % 2) == 0){
return CommonConstant.GENDER_MAN;
}else {
return CommonConstant.GENDER_WOMAN;
}
}
public static void main(String[] args){
try {
Boolean stat = checkIfIsChineseIdcard("");
System.out.println("args = [" + stat + "]");
System.out.println("args = [" + getBirthFromIdcard("") + "]");
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyyMMdd");
Date newDate = simpleDateFormat.parse("");
System.out.println(simpleDateFormat.format(newDate));
}catch (Exception e){
e.printStackTrace();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy