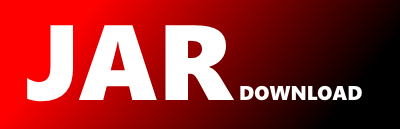
src.main.java.io.agora.rtc.ExternalVideoFrame Maven / Gradle / Ivy
//
//
// Agora Media SDK
// Copyright (c) 2020 Agora IO. All rights reserved.
// This file is generated by tool, do NOT modify it
//
//
package io.agora.rtc;
import java.nio.ByteBuffer;
public class ExternalVideoFrame {
/**
* The buffer type. See #VIDEO_BUFFER_TYPE
*/
private int type;
/**
* The pixel format. See #VIDEO_PIXEL_FORMAT
*/
private int format;
/**
* The video buffer.Must be DirectByteBuffer
*/
private java.nio.ByteBuffer buffer;
/**
* Line spacing of the incoming video frame, which must be in pixels instead of
* bytes. For
* textures, it is the width of the texture.
*/
private int stride;
/**
* Height of the incoming video frame.
*/
private int height;
/**
* [Raw data related parameter] The number of pixels trimmed from the left. The
* default value is
* 0.
*/
private int cropLeft;
/**
* [Raw data related parameter] The number of pixels trimmed from the top. The
* default value is
* 0.
*/
private int cropTop;
/**
* [Raw data related parameter] The number of pixels trimmed from the right. The
* default value is
* 0.
*/
private int cropRight;
/**
* [Raw data related parameter] The number of pixels trimmed from the bottom.
* The default value
* is 0.
*/
private int cropBottom;
/**
* [Raw data related parameter] The clockwise rotation of the video frame. You
* can set the
* rotation angle as 0, 90, 180, or 270. The default value is 0.
*/
private int rotation;
/**
* Timestamp of the incoming video frame (ms). An incorrect timestamp results in
* frame loss or
* unsynchronized audio and video.
*/
private long timestamp;
/**
* [Texture-related parameter]
* When using the OpenGL interface (javax.microedition.khronos.egl.*) defined by
* Khronos, set EGLContext to this field.
* When using the OpenGL interface (android.opengl.*) defined by Android, set
* EGLContext to this field.
*/
private Object eglContext;
/**
* [Texture related parameter] Texture ID used by the video frame.
*/
private int eglType;
/**
* [Texture related parameter] Incoming 4 × 4 transformational matrix. The
* typical value is a unit matrix.
*/
private int textureId;
/**
* [Texture related parameter] Incoming 4 × 4 transformational matrix. The
* typical value is a unit matrix.
*/
private float matrix;
/**
* [Texture related parameter] The MetaData buffer.
* The default value is NULL
*/
private ByteBuffer metadataBuffer;
/**
* Portrait Segmentation meta buffer, dimension of which is the same as
* VideoFrame.
* Pixl value is between 0-255, 0 represents totally background, 255 represents
* totally foreground.
* The default value is NULL
*/
private ByteBuffer alphaBuffer;
/**
* [For bgra or rgba only] Extract alphaBuffer from bgra or rgba data. Set it
* true if you do not explicitly specify the alphabuffer.
* The default value is false
*/
private int fillAlphaBuffer;
/**
* The relative position between alphabuffer and the frame.
* 0: Normal frame;
* 1: Alphabuffer is above the frame;
* 2: Alphabuffer is below the frame;
* 3: Alphabuffer is on the left of frame;
* 4: Alphabuffer is on the right of frame;
* The default value is 0.
*/
private int alphaMode;
/*
* The color_space_type
*/
private ColorSpace colorSpace;
public ExternalVideoFrame() {
}
public ExternalVideoFrame(int type, int format, ByteBuffer buffer, int stride, int height, int cropLeft,
int cropTop, int cropRight, int cropBottom, int rotation, long timestamp, Object eglContext, int eglType,
int textureId, float matrix, ByteBuffer metadataBuffer, ByteBuffer alphaBuffer,
int fillAlphaBuffer, int alphaMode, ColorSpace colorSpace) {
this.type = type;
this.format = format;
this.buffer = buffer;
this.stride = stride;
this.height = height;
this.cropLeft = cropLeft;
this.cropTop = cropTop;
this.cropRight = cropRight;
this.cropBottom = cropBottom;
this.rotation = rotation;
this.timestamp = timestamp;
this.eglContext = eglContext;
this.eglType = eglType;
this.textureId = textureId;
this.matrix = matrix;
this.metadataBuffer = metadataBuffer;
this.alphaBuffer = alphaBuffer;
this.fillAlphaBuffer = fillAlphaBuffer;
this.alphaMode = alphaMode;
this.colorSpace = colorSpace;
}
public int getType() {
return this.type;
}
public void setType(int type) {
this.type = type;
}
public int getFormat() {
return this.format;
}
public void setFormat(int format) {
this.format = format;
}
public java.nio.ByteBuffer getBuffer() {
return this.buffer;
}
public void setBuffer(java.nio.ByteBuffer buffer) {
this.buffer = buffer;
}
public int getStride() {
return this.stride;
}
public void setStride(int stride) {
this.stride = stride;
}
public int getHeight() {
return this.height;
}
public void setHeight(int height) {
this.height = height;
}
public int getCropLeft() {
return this.cropLeft;
}
public void setCropLeft(int cropLeft) {
this.cropLeft = cropLeft;
}
public int getCropTop() {
return this.cropTop;
}
public void setCropTop(int cropTop) {
this.cropTop = cropTop;
}
public int getCropRight() {
return this.cropRight;
}
public void setCropRight(int cropRight) {
this.cropRight = cropRight;
}
public int getCropBottom() {
return this.cropBottom;
}
public void setCropBottom(int cropBottom) {
this.cropBottom = cropBottom;
}
public int getRotation() {
return this.rotation;
}
public void setRotation(int rotation) {
this.rotation = rotation;
}
public long getTimestamp() {
return this.timestamp;
}
public void setTimestamp(long timestamp) {
this.timestamp = timestamp;
}
public Object getEglContext() {
return this.eglContext;
}
public void setEglContext(Object eglContext) {
this.eglContext = eglContext;
}
public int getEglType() {
return this.eglType;
}
public void setEglType(int eglType) {
this.eglType = eglType;
}
public int getTextureId() {
return this.textureId;
}
public void setTextureId(int textureId) {
this.textureId = textureId;
}
public float getMatrix() {
return this.matrix;
}
public void setMatrix(float matrix) {
this.matrix = matrix;
}
public ByteBuffer getMetadataBuffer() {
return this.metadataBuffer;
}
public void setMetadataBuffer(ByteBuffer metadataBuffer) {
this.metadataBuffer = metadataBuffer;
}
public ByteBuffer getAlphaBuffer() {
return this.alphaBuffer;
}
public void setAlphaBuffer(ByteBuffer buffer) {
this.alphaBuffer = buffer;
}
public int getFillAlphaBuffer() {
return this.fillAlphaBuffer;
}
public void setFillAlphaBuffer(int fillAlphaBuffer) {
this.fillAlphaBuffer = fillAlphaBuffer;
}
public int getAlphaMode() {
return this.alphaMode;
}
public void setAlphaMode(int alphaMode) {
this.alphaMode = alphaMode;
}
public ColorSpace getColorSpace() {
return this.colorSpace;
}
public void setColorSpace(ColorSpace colorSpace) {
this.colorSpace = colorSpace;
}
@Override
public String toString() {
return "ExternalVideoFrame{" + "type=" + type + ", format=" + format + ", buffer=" + buffer + ", stride="
+ stride + ", height=" + height + ", cropLeft=" + cropLeft + ", cropTop=" + cropTop + ", cropRight="
+ cropRight + ", cropBottom=" + cropBottom + ", rotation=" + rotation + ", timestamp=" + timestamp
+ ", eglContext=" + eglContext + ", eglType=" + eglType + ", textureId=" + textureId + ", matrix="
+ matrix + ", metadataBuffer=" + metadataBuffer + ", alphaBuffer=" + alphaBuffer + ", fillAlphaBuffer="
+ fillAlphaBuffer + ", alphaMode=" + alphaMode + ", colorSpace=" + colorSpace + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy