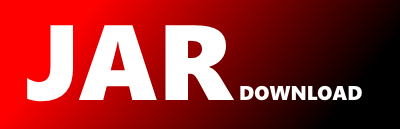
src.main.java.io.agora.rtc.RtcStats Maven / Gradle / Ivy
The newest version!
//
//
// Agora Media SDK
// Copyright (c) 2020 Agora IO. All rights reserved.
// This file is generated by tool, do NOT modify it
//
//
package io.agora.rtc;
public class RtcStats {
public RtcStats() {
}
public RtcStats(int connectionId, int duration, int txBytes, int rxBytes, int txAudioBytes, int txVideoBytes,
int rxAudioBytes, int rxVideoBytes, short txKBitRate, short rxKBitRate, short rxAudioKBitRate,
short txAudioKBitRate, short rxVideoKBitRate, short txVideoKBitRate, short lastmileDelay, int userCount,
double cpuAppUsage, double cpuTotalUsage, int gatewayRtt, double memoryAppUsageRatio,
double memoryTotalUsageRatio, int memoryAppUsageInKbytes, int connectTimeMs, int firstAudioPacketDuration,
int firstVideoPacketDuration, int firstVideoKeyFramePacketDuration, int packetsBeforeFirstKeyFramePacket,
int firstAudioPacketDurationAfterUnmute, int firstVideoPacketDurationAfterUnmute,
int firstVideoKeyFramePacketDurationAfterUnmute, int firstVideoKeyFrameDecodedDurationAfterUnmute,
int firstVideoKeyFrameRenderedDurationAfterUnmute, int txPacketLossRate, int rxPacketLossRate) {
this.connectionId = connectionId;
this.duration = duration;
this.txBytes = txBytes;
this.rxBytes = rxBytes;
this.txAudioBytes = txAudioBytes;
this.txVideoBytes = txVideoBytes;
this.rxAudioBytes = rxAudioBytes;
this.rxVideoBytes = rxVideoBytes;
this.txKBitRate = txKBitRate;
this.rxKBitRate = rxKBitRate;
this.rxAudioKBitRate = rxAudioKBitRate;
this.txAudioKBitRate = txAudioKBitRate;
this.rxVideoKBitRate = rxVideoKBitRate;
this.txVideoKBitRate = txVideoKBitRate;
this.lastmileDelay = lastmileDelay;
this.userCount = userCount;
this.cpuAppUsage = cpuAppUsage;
this.cpuTotalUsage = cpuTotalUsage;
this.gatewayRtt = gatewayRtt;
this.memoryAppUsageRatio = memoryAppUsageRatio;
this.memoryTotalUsageRatio = memoryTotalUsageRatio;
this.memoryAppUsageInKbytes = memoryAppUsageInKbytes;
this.connectTimeMs = connectTimeMs;
this.firstAudioPacketDuration = firstAudioPacketDuration;
this.firstVideoPacketDuration = firstVideoPacketDuration;
this.firstVideoKeyFramePacketDuration = firstVideoKeyFramePacketDuration;
this.packetsBeforeFirstKeyFramePacket = packetsBeforeFirstKeyFramePacket;
this.firstAudioPacketDurationAfterUnmute = firstAudioPacketDurationAfterUnmute;
this.firstVideoPacketDurationAfterUnmute = firstVideoPacketDurationAfterUnmute;
this.firstVideoKeyFramePacketDurationAfterUnmute = firstVideoKeyFramePacketDurationAfterUnmute;
this.firstVideoKeyFrameDecodedDurationAfterUnmute = firstVideoKeyFrameDecodedDurationAfterUnmute;
this.firstVideoKeyFrameRenderedDurationAfterUnmute = firstVideoKeyFrameRenderedDurationAfterUnmute;
this.txPacketLossRate = txPacketLossRate;
this.rxPacketLossRate = rxPacketLossRate;
}
private int connectionId;
public int getConnectionId() {
return this.connectionId;
}
public void setConnectionId(int connectionId) {
this.connectionId = connectionId;
}
private int duration;
public int getDuration() {
return this.duration;
}
public void setDuration(int duration) {
this.duration = duration;
}
private int txBytes;
public int getTxBytes() {
return this.txBytes;
}
public void setTxBytes(int txBytes) {
this.txBytes = txBytes;
}
private int rxBytes;
public int getRxBytes() {
return this.rxBytes;
}
public void setRxBytes(int rxBytes) {
this.rxBytes = rxBytes;
}
private int txAudioBytes;
public int getTxAudioBytes() {
return this.txAudioBytes;
}
public void setTxAudioBytes(int txAudioBytes) {
this.txAudioBytes = txAudioBytes;
}
private int txVideoBytes;
public int getTxVideoBytes() {
return this.txVideoBytes;
}
public void setTxVideoBytes(int txVideoBytes) {
this.txVideoBytes = txVideoBytes;
}
private int rxAudioBytes;
public int getRxAudioBytes() {
return this.rxAudioBytes;
}
public void setRxAudioBytes(int rxAudioBytes) {
this.rxAudioBytes = rxAudioBytes;
}
private int rxVideoBytes;
public int getRxVideoBytes() {
return this.rxVideoBytes;
}
public void setRxVideoBytes(int rxVideoBytes) {
this.rxVideoBytes = rxVideoBytes;
}
private short txKBitRate;
public short getTxKBitRate() {
return this.txKBitRate;
}
public void setTxKBitRate(short txKBitRate) {
this.txKBitRate = txKBitRate;
}
private short rxKBitRate;
public short getRxKBitRate() {
return this.rxKBitRate;
}
public void setRxKBitRate(short rxKBitRate) {
this.rxKBitRate = rxKBitRate;
}
private short rxAudioKBitRate;
public short getRxAudioKBitRate() {
return this.rxAudioKBitRate;
}
public void setRxAudioKBitRate(short rxAudioKBitRate) {
this.rxAudioKBitRate = rxAudioKBitRate;
}
private short txAudioKBitRate;
public short getTxAudioKBitRate() {
return this.txAudioKBitRate;
}
public void setTxAudioKBitRate(short txAudioKBitRate) {
this.txAudioKBitRate = txAudioKBitRate;
}
private short rxVideoKBitRate;
public short getRxVideoKBitRate() {
return this.rxVideoKBitRate;
}
public void setRxVideoKBitRate(short rxVideoKBitRate) {
this.rxVideoKBitRate = rxVideoKBitRate;
}
private short txVideoKBitRate;
public short getTxVideoKBitRate() {
return this.txVideoKBitRate;
}
public void setTxVideoKBitRate(short txVideoKBitRate) {
this.txVideoKBitRate = txVideoKBitRate;
}
private short lastmileDelay;
public short getLastmileDelay() {
return this.lastmileDelay;
}
public void setLastmileDelay(short lastmileDelay) {
this.lastmileDelay = lastmileDelay;
}
private int userCount;
public int getUserCount() {
return this.userCount;
}
public void setUserCount(int userCount) {
this.userCount = userCount;
}
private double cpuAppUsage;
public double getCpuAppUsage() {
return this.cpuAppUsage;
}
public void setCpuAppUsage(double cpuAppUsage) {
this.cpuAppUsage = cpuAppUsage;
}
private double cpuTotalUsage;
public double getCpuTotalUsage() {
return this.cpuTotalUsage;
}
public void setCpuTotalUsage(double cpuTotalUsage) {
this.cpuTotalUsage = cpuTotalUsage;
}
private int gatewayRtt;
public int getGatewayRtt() {
return this.gatewayRtt;
}
public void setGatewayRtt(int gatewayRtt) {
this.gatewayRtt = gatewayRtt;
}
private double memoryAppUsageRatio;
public double getMemoryAppUsageRatio() {
return this.memoryAppUsageRatio;
}
public void setMemoryAppUsageRatio(double memoryAppUsageRatio) {
this.memoryAppUsageRatio = memoryAppUsageRatio;
}
private double memoryTotalUsageRatio;
public double getMemoryTotalUsageRatio() {
return this.memoryTotalUsageRatio;
}
public void setMemoryTotalUsageRatio(double memoryTotalUsageRatio) {
this.memoryTotalUsageRatio = memoryTotalUsageRatio;
}
private int memoryAppUsageInKbytes;
public int getMemoryAppUsageInKbytes() {
return this.memoryAppUsageInKbytes;
}
public void setMemoryAppUsageInKbytes(int memoryAppUsageInKbytes) {
this.memoryAppUsageInKbytes = memoryAppUsageInKbytes;
}
private int connectTimeMs;
public int getConnectTimeMs() {
return this.connectTimeMs;
}
public void setConnectTimeMs(int connectTimeMs) {
this.connectTimeMs = connectTimeMs;
}
private int firstAudioPacketDuration;
public int getFirstAudioPacketDuration() {
return this.firstAudioPacketDuration;
}
public void setFirstAudioPacketDuration(int firstAudioPacketDuration) {
this.firstAudioPacketDuration = firstAudioPacketDuration;
}
private int firstVideoPacketDuration;
public int getFirstVideoPacketDuration() {
return this.firstVideoPacketDuration;
}
public void setFirstVideoPacketDuration(int firstVideoPacketDuration) {
this.firstVideoPacketDuration = firstVideoPacketDuration;
}
private int firstVideoKeyFramePacketDuration;
public int getFirstVideoKeyFramePacketDuration() {
return this.firstVideoKeyFramePacketDuration;
}
public void setFirstVideoKeyFramePacketDuration(int firstVideoKeyFramePacketDuration) {
this.firstVideoKeyFramePacketDuration = firstVideoKeyFramePacketDuration;
}
private int packetsBeforeFirstKeyFramePacket;
public int getPacketsBeforeFirstKeyFramePacket() {
return this.packetsBeforeFirstKeyFramePacket;
}
public void setPacketsBeforeFirstKeyFramePacket(int packetsBeforeFirstKeyFramePacket) {
this.packetsBeforeFirstKeyFramePacket = packetsBeforeFirstKeyFramePacket;
}
private int firstAudioPacketDurationAfterUnmute;
public int getFirstAudioPacketDurationAfterUnmute() {
return this.firstAudioPacketDurationAfterUnmute;
}
public void setFirstAudioPacketDurationAfterUnmute(int firstAudioPacketDurationAfterUnmute) {
this.firstAudioPacketDurationAfterUnmute = firstAudioPacketDurationAfterUnmute;
}
private int firstVideoPacketDurationAfterUnmute;
public int getFirstVideoPacketDurationAfterUnmute() {
return this.firstVideoPacketDurationAfterUnmute;
}
public void setFirstVideoPacketDurationAfterUnmute(int firstVideoPacketDurationAfterUnmute) {
this.firstVideoPacketDurationAfterUnmute = firstVideoPacketDurationAfterUnmute;
}
private int firstVideoKeyFramePacketDurationAfterUnmute;
public int getFirstVideoKeyFramePacketDurationAfterUnmute() {
return this.firstVideoKeyFramePacketDurationAfterUnmute;
}
public void setFirstVideoKeyFramePacketDurationAfterUnmute(int firstVideoKeyFramePacketDurationAfterUnmute) {
this.firstVideoKeyFramePacketDurationAfterUnmute = firstVideoKeyFramePacketDurationAfterUnmute;
}
private int firstVideoKeyFrameDecodedDurationAfterUnmute;
public int getFirstVideoKeyFrameDecodedDurationAfterUnmute() {
return this.firstVideoKeyFrameDecodedDurationAfterUnmute;
}
public void setFirstVideoKeyFrameDecodedDurationAfterUnmute(int firstVideoKeyFrameDecodedDurationAfterUnmute) {
this.firstVideoKeyFrameDecodedDurationAfterUnmute = firstVideoKeyFrameDecodedDurationAfterUnmute;
}
private int firstVideoKeyFrameRenderedDurationAfterUnmute;
public int getFirstVideoKeyFrameRenderedDurationAfterUnmute() {
return this.firstVideoKeyFrameRenderedDurationAfterUnmute;
}
public void setFirstVideoKeyFrameRenderedDurationAfterUnmute(int firstVideoKeyFrameRenderedDurationAfterUnmute) {
this.firstVideoKeyFrameRenderedDurationAfterUnmute = firstVideoKeyFrameRenderedDurationAfterUnmute;
}
private int txPacketLossRate;
public int getTxPacketLossRate() {
return this.txPacketLossRate;
}
public void setTxPacketLossRate(int txPacketLossRate) {
this.txPacketLossRate = txPacketLossRate;
}
private int rxPacketLossRate;
public int getRxPacketLossRate() {
return this.rxPacketLossRate;
}
public void setRxPacketLossRate(int rxPacketLossRate) {
this.rxPacketLossRate = rxPacketLossRate;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy