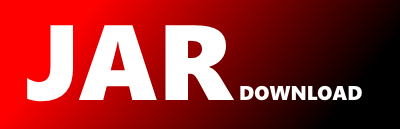
src.main.java.io.agora.rtc.VideoEncoderConfig Maven / Gradle / Ivy
The newest version!
//
//
// Agora Media SDK
// Copyright (c) 2020 Agora IO. All rights reserved.
// This file is generated by tool, do NOT modify it
//
//
package io.agora.rtc;
public class VideoEncoderConfig {
/**
* The video encoder code type: #VIDEO_CODEC_TYPE.
*/
private int codecType;
/**
* The video frame dimension used to specify the video quality and measured
* by the total number of pixels along a frame's width and height:
* VideoDimensions.
*/
private VideoDimensions dimensions;
/**
* Frame rate of the video: int type, but can accept #FRAME_RATE for backward
* compatibility.
*/
private int frameRate;
/**
* Video encoding target bitrate (Kbps).
*/
private int bitrate;
/**
* (For future use) The minimum encoding bitrate (Kbps).
*
* The Agora SDK automatically adjusts the encoding bitrate to adapt to the
* network conditions.
*
* Using a value greater than the default value forces the video encoder to
* output high-quality images but may cause more packet loss and hence
* sacrifice the smoothness of the video transmission. That said, unless you
* have special requirements for image quality, Agora does not recommend
* changing this value.
*
* @note
* This parameter applies to the Live Broadcast profile only.
*/
private int minBitrate;
/**
* (For future use) The video orientation mode of the video: #ORIENTATION_MODE.
*/
private int orientationMode;
/**
*
* The video degradation preference when the bandwidth is a constraint:
* #DEGRADATION_PREFERENCE. Currently, this member supports
* `MAINTAIN_QUALITY`(0)
* only.
*/
private int degradationPreference;
/**
* If mirror_type is set to VIDEO_MIRROR_MODE_ENABLED, then the video frame
* would be mirrored before encoding.
*/
private int mirrorMode;
/**
* Whether to encode and send the alpha data to the remote when alpha data is
* present.
* The default value is false.
*/
private int encodeAlpha;
public VideoEncoderConfig() {
}
public VideoEncoderConfig(int codecType, VideoDimensions dimensions, int frameRate, int bitrate, int minBitrate,
int orientationMode, int degradationPreference, int mirrorMode, int encodeAlpha) {
this.codecType = codecType;
this.dimensions = dimensions;
this.frameRate = frameRate;
this.bitrate = bitrate;
this.minBitrate = minBitrate;
this.orientationMode = orientationMode;
this.degradationPreference = degradationPreference;
this.mirrorMode = mirrorMode;
this.encodeAlpha = encodeAlpha;
}
public int getCodecType() {
return this.codecType;
}
public void setCodecType(int codecType) {
this.codecType = codecType;
}
public VideoDimensions getDimensions() {
return this.dimensions;
}
public void setDimensions(VideoDimensions dimensions) {
this.dimensions = dimensions;
}
public int getFrameRate() {
return this.frameRate;
}
public void setFrameRate(int frameRate) {
this.frameRate = frameRate;
}
public int getBitrate() {
return this.bitrate;
}
public void setBitrate(int bitrate) {
this.bitrate = bitrate;
}
public int getMinBitrate() {
return this.minBitrate;
}
public void setMinBitrate(int minBitrate) {
this.minBitrate = minBitrate;
}
public int getOrientationMode() {
return this.orientationMode;
}
public void setOrientationMode(int orientationMode) {
this.orientationMode = orientationMode;
}
public int getDegradationPreference() {
return this.degradationPreference;
}
public void setDegradationPreference(int degradationPreference) {
this.degradationPreference = degradationPreference;
}
public int getMirrorMode() {
return this.mirrorMode;
}
public void setMirrorMode(int mirrorMode) {
this.mirrorMode = mirrorMode;
}
public int getEncodeAlpha() {
return this.encodeAlpha;
}
public void setEncodeAlpha(int encodeAlpha) {
this.encodeAlpha = encodeAlpha;
}
@Override
public String toString() {
return "VideoEncoderConfig{" + "codecType=" + codecType + ", dimensions=" + dimensions + ", frameRate="
+ frameRate + ", bitrate=" + bitrate + ", minBitrate=" + minBitrate + ", orientationMode="
+ orientationMode
+ ", degradationPreference=" + degradationPreference + ", mirrorMode=" + mirrorMode + ", encodeAlpha="
+ encodeAlpha + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy