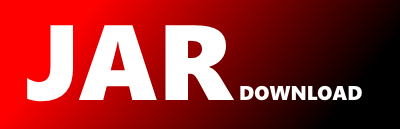
src.main.java.io.agora.rtc.VideoFrame Maven / Gradle / Ivy
The newest version!
//
//
// Agora Media SDK
// Copyright (c) 2020 Agora IO. All rights reserved.
// This file is generated by tool, do NOT modify it
//
//
package io.agora.rtc;
import java.nio.ByteBuffer;
public class VideoFrame {
private int type;
/**
* Video pixel width.
*/
private int width;
/**
* Video pixel height.
*/
private int height;
/**
* Line span of Y buffer in YUV data.
*/
private int yStride;
/**
* Line span of U buffer in YUV data.
*/
private int uStride;
/**
* Line span of V buffer in YUV data.
*/
private int vStride;
/**
* Y data buffer
*/
private ByteBuffer yBuffer;
/**
* U data buffer
*/
private ByteBuffer uBuffer;
/**
* V data buffer
*/
private ByteBuffer vBuffer;
/**
* rotation of this frame (0, 90, 180, 270)
*/
private int rotation;
/**
* Timestamp to render the video stream. It instructs the users to use this
* timestamp to
* synchronize the video stream render while rendering the video streams.
*
* Note: This timestamp is for rendering the video stream, not for capturing the
* video stream.
*/
private long renderTimeMs;
private int avsyncType;
/**
* [Texture related parameter] The MetaData buffer.
* The default value is NULL
*/
private ByteBuffer metadataBuffer;
/**
* [Texture related parameter], egl context.
*/
private Object sharedContext;
/**
* [Texture related parameter], Texture ID used by the video frame.
*/
private int textureId;
/**
* [Texture related parameter], Incoming 4 × 4 transformational matrix.
*/
private float[] matrix;
/**
* Portrait Segmentation meta buffer, dimension of which is the same as
* VideoFrame.
* Pixl value is between 0-255, 0 represents totally background, 255 represents
* totally foreground.
* The default value is NULL
*/
private ByteBuffer alphaBuffer;
/**
* The relative position between alphabuffer and the frame.
* 0: Normal frame;
* 1: Alphabuffer is above the frame;
* 2: Alphabuffer is below the frame;
* 3: Alphabuffer is on the left of frame;
* 4: Alphabuffer is on the right of frame;
* The default value is 0.
*/
private int alphaMode;
public VideoFrame() {
}
public VideoFrame(int type, int width, int height, int yStride, int uStride, int vStride,
ByteBuffer yBuffer, ByteBuffer uBuffer, ByteBuffer vBuffer, int rotation,
long renderTimeMs, int avsyncType, ByteBuffer metadataBuffer, Object sharedContext,
int textureId, float[] matrix, ByteBuffer alphaBuffer, int alphaMode) {
this.type = type;
this.width = width;
this.height = height;
this.yStride = yStride;
this.uStride = uStride;
this.vStride = vStride;
this.yBuffer = yBuffer;
this.uBuffer = uBuffer;
this.vBuffer = vBuffer;
this.rotation = rotation;
this.renderTimeMs = renderTimeMs;
this.avsyncType = avsyncType;
this.metadataBuffer = metadataBuffer;
this.sharedContext = sharedContext;
this.textureId = textureId;
this.matrix = matrix;
this.alphaBuffer = alphaBuffer;
this.alphaMode = alphaMode;
}
public int getType() {
return this.type;
}
public void setType(int type) {
this.type = type;
}
public int getWidth() {
return this.width;
}
public void setWidth(int width) {
this.width = width;
}
public int getHeight() {
return this.height;
}
public void setHeight(int height) {
this.height = height;
}
public int getYStride() {
return this.yStride;
}
public void setYStride(int yStride) {
this.yStride = yStride;
}
public int getUStride() {
return this.uStride;
}
public void setUStride(int uStride) {
this.uStride = uStride;
}
public int getVStride() {
return this.vStride;
}
public void setVStride(int vStride) {
this.vStride = vStride;
}
public ByteBuffer getYBuffer() {
return this.yBuffer;
}
public void setYBuffer(ByteBuffer yBuffer) {
this.yBuffer = yBuffer;
}
public ByteBuffer getUBuffer() {
return this.uBuffer;
}
public void setUBuffer(ByteBuffer uBuffer) {
this.uBuffer = uBuffer;
}
public ByteBuffer getVBuffer() {
return this.vBuffer;
}
public void setVBuffer(ByteBuffer vBuffer) {
this.vBuffer = vBuffer;
}
public int getRotation() {
return this.rotation;
}
public void setRotation(int rotation) {
this.rotation = rotation;
}
public long getRenderTimeMs() {
return this.renderTimeMs;
}
public void setRenderTimeMs(long renderTimeMs) {
this.renderTimeMs = renderTimeMs;
}
public int getAvsyncType() {
return this.avsyncType;
}
public void setAvsyncType(int avsyncType) {
this.avsyncType = avsyncType;
}
public ByteBuffer getMetadataBuffer() {
return this.metadataBuffer;
}
public void setMetadataBuffer(ByteBuffer metadataBuffer) {
this.metadataBuffer = metadataBuffer;
}
public Object getSharedContext() {
return this.sharedContext;
}
public void setSharedContext(Object sharedContext) {
this.sharedContext = sharedContext;
}
public int getTextureId() {
return this.textureId;
}
public void setTextureId(int textureId) {
this.textureId = textureId;
}
public float[] getMatrix() {
return this.matrix;
}
public void setMatrix(float[] matrix) {
this.matrix = matrix;
}
public ByteBuffer getAlphaBuffer() {
return this.alphaBuffer;
}
public void setAlphaBuffer(ByteBuffer alphaBuffer) {
this.alphaBuffer = alphaBuffer;
}
public int getAlphaMode() {
return this.alphaMode;
}
public void setAlphaMode(int alphaMode) {
this.alphaMode = alphaMode;
}
@Override
public String toString() {
return "VideoFrame{" + "type=" + type + ", width=" + width + ", height=" + height + ", yStride=" + yStride
+ ", uStride=" + uStride + ", vStride=" + vStride + ", yBuffer=" + yBuffer + ", uBuffer=" + uBuffer
+ ", vBuffer=" + vBuffer + ", rotation=" + rotation + ", renderTimeMs=" + renderTimeMs + ", avsyncType="
+ avsyncType + ", metadataBuffer=" + metadataBuffer
+ ", sharedContext=" + sharedContext + ", textureId=" + textureId + ", matrix=" + matrix
+ ", alphaBuffer=" + alphaBuffer + ", alphaMode=" + alphaMode + '}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy