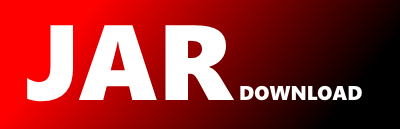
io.agora.rtm.MetadataItem Maven / Gradle / Ivy
package io.agora.rtm;
import io.agora.common.internal.CalledByNative;
public class MetadataItem {
/**
* The key of the metadata item.
*/
private String key = "";
/**
* The value of the metadata item.
*/
private String value = "";
/**
* The User ID of the user who makes the latest update to the metadata item.
*/
private String authorUserId = "";
/**
* The revision of the metadata item.
*/
private long revision;
/**
* The Timestamp when the metadata item was last updated.
*/
private long updateTs;
/**
* Creates a new instance of {@code MetadataItem} with default parameters.
*/
public MetadataItem() {
this.revision = -1;
this.updateTs = 0;
}
/**
* Creates a new instance of {@code MetadataItem} with key and value.
*
* @param key The key of the metadata item
* @param value The value of the metadata item
*/
public MetadataItem(String key, String value) {
this.key = key;
this.value = value;
}
/**
* Creates a new instance of {@code MetadataItem} with key, value and revision.
*
* @apiNote If set revision not equals -1, rtm server will check the revision
* when modify MetadataItem.
*
* @param key The key of the metadata item
* @param value The value of the metadata item
* @param revision The revision of the metadata item
*/
public MetadataItem(String key, String value, long revision) {
this.key = key;
this.value = value;
this.revision = revision;
}
@CalledByNative
public MetadataItem(String key, String value, String authorUserId, long revision, long updateTs) {
this.key = key;
this.value = value;
this.authorUserId = authorUserId;
this.revision = revision;
this.updateTs = updateTs;
}
public void setKey(String key) {
this.key = key;
}
public void setValue(String value) {
this.value = value;
}
public void setAuthorUserId(String authorUserId) {
this.authorUserId = authorUserId;
}
public void setRevision(long revision) {
this.revision = revision;
}
public void setUpdateTs(long updateTs) {
this.updateTs = updateTs;
}
@CalledByNative
public String getKey() {
return this.key;
}
@CalledByNative
public String getValue() {
return this.value;
}
@CalledByNative
public String getAuthorUserId() {
return this.authorUserId;
}
@CalledByNative
public long getRevision() {
return this.revision;
}
@CalledByNative
public long getUpdateTs() {
return this.updateTs;
}
@Override
public String toString() {
return "MetadataItem {key: " + key + ", value: " + value + ", authorUserId: " + authorUserId
+ ", revision: " + revision + ", updateTs: " + updateTs + "}";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy