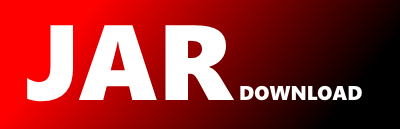
io.agora.rtm.RtmClient Maven / Gradle / Ivy
package io.agora.rtm;
import io.agora.rtm.ResultCallback;
import io.agora.rtm.RtmConfig;
import io.agora.rtm.RtmConstants.RtmErrorCode;
import io.agora.rtm.SubscribeOptions;
import io.agora.rtm.internal.RtmClientImpl;
/**
* The RtmClient class.
*
* This class provides the main methods that can be invoked by your app.
*
* RtmClient is the basic interface class of the Agora RTM SDK.
* Creating an RtmClient object and then calling the methods of
* this object enables you to use Agora RTM SDK's functionality.
*/
public abstract class RtmClient {
private static RtmClient mInstance = null;
protected abstract RtmErrorCode initialize(RtmConfig config);
protected abstract RtmErrorCode releaseClient();
/**
* create rtm client instance.
*
* @return If the method call succeeds, will return {@code RtmClient} instance,
* if the method call fails, will throw an exception.
*
* @exception If error occurs
*/
public static synchronized RtmClient create(RtmConfig config) throws Exception {
if (!RtmClientImpl.initializeNativeLibs()) {
throw new UnsatisfiedLinkError("load native libraries failed");
}
if (mInstance == null) {
mInstance = new RtmClientImpl();
}
if ((mInstance != null)) {
RtmErrorCode errorCode = mInstance.initialize(config);
if (errorCode != RtmErrorCode.OK) {
release();
throw new IllegalArgumentException(
"native rtm client init failed, error code: " + errorCode);
}
}
return mInstance;
}
/**
* get rtm client instance.
*
* @return The instance of RtmClient {@link RtmClient}.
*/
public static synchronized RtmClient getInstance() {
return mInstance;
}
/**
* Release the rtm client instance.
*/
public static synchronized void release() {
if (mInstance == null) {
return;
}
mInstance.releaseClient();
mInstance = null;
}
/**
* Adds an event listener for receiving rtm events.
*
* @apiNote The listener must implement the RtmEventListener interface or use
* RtmEventListener default implements if do not care about some event.
*
* @param listener the RtmEventListener object to be added
*/
public abstract void addEventListener(RtmEventListener listener);
/**
* Remove the specified event listener.
*
* @apiNote By removing the event listener, the listener will no longer receive
* notifications from rtm client.
*
* @param listener The event specified listener object
*/
public abstract void removeEventListener(RtmEventListener listener);
/**
* Login the Agora RTM service.
*
* @param token Token used to login RTM service.
* @param resultCallback A {@link ResultCallback} object.
* - Success: will receives the
* {@link ResultCallback.onSuccess()} callback.
* - Failure: will receives the
* {@link ResultCallback.onFailure()} callback.
*/
public abstract void login(String token, ResultCallback resultCallback);
/**
* Logout the Agora RTM service.
*
* @param resultCallback A {@link ResultCallback} object.
* - Success: will receives the
* {@link ResultCallback.onSuccess()} callback.
* - Failure: will receives the
* {@link ResultCallback.onFailure()} callback.
*/
public abstract void logout(ResultCallback resultCallback);
/**
* Get the storage instance.
*
* @return The instance of {@link RtmStorage}
*/
public abstract RtmStorage getStorage();
/**
* Get the lock instance.
*
* @return The instance of {@link RtmLock}
*/
public abstract RtmLock getLock();
/**
* Get the presence instance.
*
* @return The instance of {@link RtmPresence}
*/
public abstract RtmPresence getPresence();
/**
* Convert error code to error string.
*
* @param errorCode Received error code
* @return The error reason
*/
public abstract String getErrorReason(RtmErrorCode errorCode);
/**
* Renews the token. Once a token is enabled and used, it expires after a
* certain period of time.
* You should generate a new token on your server, call this method to renew it.
*
* @param token The new token
* @param resultCallback A {@link ResultCallback} object.
* - Success: will receives the
* {@link ResultCallback.onSuccess()} callback.
* - Failure: will receives the
* {@link ResultCallback.onFailure()} callback.
*/
public abstract void renewToken(String token, ResultCallback resultCallback);
/**
* Publish a string message in the channel.
*
* @param channelName The name of the channel.
* @param message The content of the string message.
* @param option The option of the message.
* @param resultCallback A {@link ResultCallback} object.
* - Success: will receives the
* {@link ResultCallback.onSuccess()} callback.
* - Failure: will receives the
* {@link ResultCallback.onFailure()} callback.
*/
public abstract void publish(String channelName, String message, PublishOptions options,
ResultCallback resultCallback);
/**
* Publish a binary message in the channel.
*
* @param channelName The name of the channel.
* @param message The content of the string message.
* @param option The option of the message.
* @param resultCallback A {@link ResultCallback} object.
* - Success: will receives the
* {@link ResultCallback.onSuccess()} callback.
* - Failure: will receives the
* {@link ResultCallback.onFailure()} callback.
*/
public abstract void publish(String channelName, byte[] message, PublishOptions options,
ResultCallback resultCallback);
/**
* Subscribe a channel.
*
* @param channelName The name of the channel.
* @param options The options of subscribe the channel.
* @param resultCallback A {@link ResultCallback} object.
* - Success: will receives the
* {@link ResultCallback.onSuccess()} callback.
* - Failure: will receives the
* {@link ResultCallback.onFailure()} callback.
*/
public abstract void subscribe(
String channelName, SubscribeOptions options, ResultCallback resultCallback);
/**
* Unsubscribe a channel.
*
* @param channelName The name of the channel.
* @param resultCallback A {@link ResultCallback} object.
* - Success: will receives the
* {@link ResultCallback.onSuccess()} callback.
* - Failure: will receives the
* {@link ResultCallback.onFailure()} callback.
*/
public abstract void unsubscribe(String channelName, ResultCallback resultCallback);
/**
* Set parameters of the sdk or engine
*
* @param parameters The parameters in json format
* @return {@code RtmErrorCode}
*/
public abstract RtmErrorCode setParameters(String parameters);
/**
* Create a stream channel instance.
*
* @param channelName The Name of the channel.
*
* @return If the method call succeeds, will return {@code StreamChannel}
* instance, if the method call fails, will throw an exception.
*
* @exception If error occurs
*/
public abstract StreamChannel createStreamChannel(String channelName) throws Exception;
/**
* Get the version info of the Agora RTM SDK.
*
* @return The version info of the Agora RTM SDK.
*/
public abstract String getVersion();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy