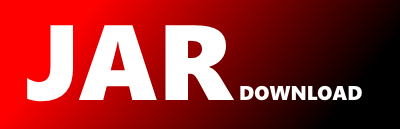
io.agora.rtm.internal.RtmLockImpl Maven / Gradle / Ivy
package io.agora.rtm.internal;
import io.agora.rtm.ErrorInfo;
import io.agora.rtm.LockDetail;
import io.agora.rtm.ResultCallback;
import io.agora.rtm.RtmConstants;
import io.agora.rtm.RtmConstants.RtmChannelType;
import io.agora.rtm.RtmConstants.RtmErrorCode;
import io.agora.rtm.RtmLock;
import java.util.ArrayList;
class RtmLockImpl extends RtmLock {
private static final String TAG = RtmLockImpl.class.getSimpleName();
private long mNativeLock = 0;
private RtmClientImpl mRtmClient;
public RtmLockImpl(long nativeHandle, RtmClientImpl rtmClient) {
mNativeLock = nativeHandle;
mRtmClient = rtmClient;
}
@Override
public synchronized void setLock(String channelName, RtmChannelType channelType, String lockName,
long ttl, ResultCallback resultCallback) {
if (mNativeLock == 0) {
mRtmClient.processFailureCallback(
resultCallback, RtmErrorCode.NOT_INITIALIZED, RtmConstants.SET_LOCK_API_STR);
return;
}
synchronized (mRtmClient.mRtmCallbackLock) {
RequestInfo requestInfo = new RequestInfo();
int ret = nativeSetLock(mNativeLock, channelName, RtmChannelType.getValue(channelType),
lockName, ttl, requestInfo);
RtmErrorCode errorCode = RtmErrorCode.getEnum(ret);
if (errorCode == RtmErrorCode.OK) {
mRtmClient.mModifyLockCallback.put(requestInfo.requestId, resultCallback);
} else {
mRtmClient.processFailureCallback(resultCallback, errorCode, RtmConstants.SET_LOCK_API_STR);
}
}
}
@Override
public synchronized void getLocks(String channelName, RtmChannelType channelType,
ResultCallback> resultCallback) {
if (resultCallback == null) {
return;
}
if (mNativeLock == 0) {
resultCallback.onFailure(new ErrorInfo(RtmErrorCode.NOT_INITIALIZED,
mRtmClient.getErrorReason(RtmErrorCode.NOT_INITIALIZED), RtmConstants.GET_LOCKS_API_STR));
return;
}
synchronized (mRtmClient.mRtmCallbackLock) {
RequestInfo requestInfo = new RequestInfo();
int ret = nativeGetLocks(
mNativeLock, channelName, RtmChannelType.getValue(channelType), requestInfo);
RtmErrorCode errorCode = RtmErrorCode.getEnum(ret);
if (errorCode == RtmErrorCode.OK) {
mRtmClient.mGetLocksCallback.put(requestInfo.requestId, resultCallback);
} else {
resultCallback.onFailure(new ErrorInfo(
errorCode, mRtmClient.getErrorReason(ret), RtmConstants.GET_LOCKS_API_STR));
}
}
}
@Override
public synchronized void removeLock(String channelName, RtmChannelType channelType,
String lockName, ResultCallback resultCallback) {
if (mNativeLock == 0) {
mRtmClient.processFailureCallback(
resultCallback, RtmErrorCode.NOT_INITIALIZED, RtmConstants.REMOVE_LOCK_API_STR);
return;
}
synchronized (mRtmClient.mRtmCallbackLock) {
RequestInfo requestInfo = new RequestInfo();
int ret = nativeRemoveLock(
mNativeLock, channelName, RtmChannelType.getValue(channelType), lockName, requestInfo);
RtmErrorCode errorCode = RtmErrorCode.getEnum(ret);
if (errorCode == RtmErrorCode.OK) {
mRtmClient.mModifyLockCallback.put(requestInfo.requestId, resultCallback);
} else {
mRtmClient.processFailureCallback(
resultCallback, errorCode, RtmConstants.REMOVE_LOCK_API_STR);
}
}
}
@Override
public synchronized void acquireLock(String channelName, RtmChannelType channelType,
String lockName, boolean retry, ResultCallback resultCallback) {
if (mNativeLock == 0) {
mRtmClient.processFailureCallback(
resultCallback, RtmErrorCode.NOT_INITIALIZED, RtmConstants.ACQUIRE_LOCK_API_STR);
return;
}
synchronized (mRtmClient.mRtmCallbackLock) {
RequestInfo requestInfo = new RequestInfo();
int ret = nativeAcquireLock(mNativeLock, channelName, RtmChannelType.getValue(channelType),
lockName, retry, requestInfo);
RtmErrorCode errorCode = RtmErrorCode.getEnum(ret);
if (errorCode == RtmErrorCode.OK) {
mRtmClient.mAcquireLockCallback.put(requestInfo.requestId, resultCallback);
} else {
mRtmClient.processFailureCallback(
resultCallback, errorCode, RtmConstants.ACQUIRE_LOCK_API_STR);
}
}
}
@Override
public synchronized void releaseLock(String channelName, RtmChannelType channelType,
String lockName, ResultCallback resultCallback) {
if (mNativeLock == 0) {
mRtmClient.processFailureCallback(
resultCallback, RtmErrorCode.NOT_INITIALIZED, RtmConstants.RELEASE_LOCK_API_STR);
return;
}
synchronized (mRtmClient.mRtmCallbackLock) {
RequestInfo requestInfo = new RequestInfo();
int ret = nativeReleaseLock(
mNativeLock, channelName, RtmChannelType.getValue(channelType), lockName, requestInfo);
RtmErrorCode errorCode = RtmErrorCode.getEnum(ret);
if (errorCode == RtmErrorCode.OK) {
mRtmClient.mModifyLockCallback.put(requestInfo.requestId, resultCallback);
} else {
mRtmClient.processFailureCallback(
resultCallback, errorCode, RtmConstants.RELEASE_LOCK_API_STR);
}
}
}
@Override
public synchronized void revokeLock(String channelName, RtmChannelType channelType,
String lockName, String owner, ResultCallback resultCallback) {
if (mNativeLock == 0) {
mRtmClient.processFailureCallback(
resultCallback, RtmErrorCode.NOT_INITIALIZED, RtmConstants.REVOKE_LOCK_API_STR);
return;
}
synchronized (mRtmClient.mRtmCallbackLock) {
RequestInfo requestInfo = new RequestInfo();
int ret = nativeRevokeLock(mNativeLock, channelName, RtmChannelType.getValue(channelType),
lockName, owner, requestInfo);
RtmErrorCode errorCode = RtmErrorCode.getEnum(ret);
if (errorCode == RtmErrorCode.OK) {
mRtmClient.mModifyLockCallback.put(requestInfo.requestId, resultCallback);
} else {
mRtmClient.processFailureCallback(
resultCallback, errorCode, RtmConstants.REVOKE_LOCK_API_STR);
}
}
}
private native int nativeSetLock(long nativeRtmLockAndroid, String channelName, int channelType,
String lockName, long ttl, RequestInfo requestInfo);
private native int nativeGetLocks(
long nativeRtmLockAndroid, String channelName, int channelType, RequestInfo requestInfo);
private native int nativeRemoveLock(long nativeRtmLockAndroid, String channelName,
int channelType, String lockName, RequestInfo requestInfo);
private native int nativeAcquireLock(long nativeRtmLockAndroid, String channelName,
int channelType, String lockName, boolean retry, RequestInfo requestInfo);
private native int nativeReleaseLock(long nativeRtmLockAndroid, String channelName,
int channelType, String lockName, RequestInfo requestInfo);
private native int nativeRevokeLock(long nativeRtmLockAndroid, String channelName,
int channelType, String lockName, String owner, RequestInfo requestInfo);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy