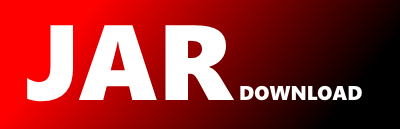
io.alauda.client.AlaudaClient Maven / Gradle / Ivy
package io.alauda.client;
import io.alauda.http.AlaudaHttp;
import net.sf.json.JSONObject;
import okhttp3.HttpUrl;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
public class AlaudaClient implements IAlaudaClient {
private IServiceClient serviceClient;
private IBuildClient buildClient;
private INotifactionClient notifactionClient;
private static Logger log = LoggerFactory.getLogger(AlaudaClient.class);
private String endPoint;
private String namespace;
private String spaceName;
private String token;
private String scheme;
private String host;
private int port;
public AlaudaClient(String endPoint, String namespace, String token) {
this.endPoint = validateEndpoint(endPoint);
this.namespace = namespace;
this.token = token;
AlaudaHttp httpClient = new AlaudaHttp();
httpClient.setToken(token);
serviceClient = new ServiceClient(this, httpClient);
buildClient = new BuildClient(this, httpClient);
notifactionClient = new NotifactionClient(this, httpClient);
}
public AlaudaClient(String endPoint, String namespace, String token, String spaceName) {
this(endPoint, namespace, token);
this.spaceName = spaceName;
}
public String getEndPoint() {
return endPoint;
}
public void setEndPoint(String endPoint) {
this.endPoint = validateEndpoint(endPoint);
}
public String getNamespace() {
return namespace;
}
public void setNamespace(String namespace) {
this.namespace = namespace;
}
public String getSpaceName() {
return spaceName;
}
public void setSpaceName(String spaceName) {
this.spaceName = spaceName;
}
public String getToken() {
return token;
}
public void setToken(String token) {
this.token = token;
}
public String getScheme() {
return scheme;
}
public void setScheme(String scheme) {
this.scheme = scheme;
}
public String getHost() {
return host;
}
public void setHost(String host) {
this.host = host;
}
public int getPort() {
return port;
}
public void setPort(int port) {
this.port = port;
}
public IServiceClient getServiceClient() {
return serviceClient;
}
public void setServiceClient(IServiceClient serviceClient) {
this.serviceClient = serviceClient;
}
public IBuildClient getBuildClient() {
return buildClient;
}
public void setBuildClient(IBuildClient buildClient) {
this.buildClient = buildClient;
}
// remove the "/" at end of the endpoint
private String validateEndpoint(String endPoint) {
if ('/' == endPoint.charAt(endPoint.length() - 1)) {
return endPoint.substring(0, (endPoint.length() - 1));
} else {
return endPoint;
}
}
public HttpUrl buildURL(String segment) {
parseEndpoint();
HttpUrl.Builder builder = new HttpUrl.Builder();
builder.scheme(scheme)
.host(host)
.addPathSegments(segment);
if (port != 0) {
builder.port(port);
}
return builder.build();
}
public void parseEndpoint() {
String scheme;
String host;
int port;
String host_port;
String[] scheme_host_port = endPoint.split("//");
if (scheme_host_port.length == 2) {
String tmpScheme = scheme_host_port[0];
scheme = tmpScheme.substring(0, (tmpScheme.length() - 1));
host_port = scheme_host_port[1];
} else {
scheme = "http";
host_port = scheme_host_port[0];
}
String[] host_port_list = host_port.split(":");
if (host_port_list.length == 2) {
host = host_port_list[0];
port = Integer.parseInt(host_port_list[1]);
} else {
host = host_port_list[0];
port = 0;
}
setScheme(scheme);
setHost(host);
setPort(port);
}
public INotifactionClient getNotifactionClient() {
return notifactionClient;
}
public void setNotifactionClient(INotifactionClient notifactionClient) {
this.notifactionClient = notifactionClient;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy