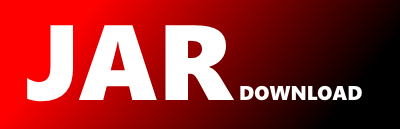
io.alauda.client.ServiceClient Maven / Gradle / Ivy
package io.alauda.client;
import io.alauda.http.AlaudaHttp;
import io.alauda.model.*;
import io.alauda.util.JacksonUtil;
import net.sf.json.JSONObject;
import okhttp3.HttpUrl;
import okhttp3.Response;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.net.HttpURLConnection;
public class ServiceClient implements IServiceClient {
private IAlaudaClient client;
private AlaudaHttp httpClient;
private static Logger log = LoggerFactory.getLogger(ServiceClient.class);
public ServiceClient(IAlaudaClient client, AlaudaHttp httpClient) {
this.client = client;
this.httpClient = httpClient;
}
private HttpUrl buildURL(String segment) {
return this.client.buildURL(segment);
}
private String prefixURL() {
return this.httpClient.prefixURLV2("services");
}
private ServiceList listService(String clusterName, String projectName) throws IOException {
return listService(clusterName, "", "", projectName);
}
private ServiceList listService(String clusterName, String namespace, String projectName) throws IOException {
return listService(clusterName, namespace, "", projectName);
}
private ServiceList listService(String clusterName, String namespace, String serviceName, String projectName) throws IOException {
log.info(String.format("listService clusterName is %s; namespace is %s; serviceName is %s; projectName is %s", clusterName, namespace, serviceName, projectName));
HttpUrl url = this.client.buildURL(prefixURL());
url = httpClient.addParamsToUrl(url, "name", serviceName);
url = httpClient.addParamsToUrl(url, "cluster", clusterName);
url = httpClient.addParamsToUrl(url, "namespace", namespace);
url = httpClient.addParamsToUrl(url, "project_name", projectName);
String response = httpClient.get(url);
return JacksonUtil.json2Bean(response, ServiceList.class);
}
// retrieve service details
public ServiceDetails retrieveService(String serviceID) throws IOException {
log.info(String.format("retrieveService serviceID is: %s", serviceID));
String segment = prefixURL() + serviceID;
HttpUrl url = this.client.buildURL(segment);
Response resp = httpClient.request("get", url, null);
if (resp.code() == HttpURLConnection.HTTP_NOT_FOUND){
log.warn(String.format("service %s is not found", serviceID));
return null;
}
return JacksonUtil.json2Bean(resp.body().string(), ServiceDetails.class);
}
// retrieve service details
public ServiceDetails retrieveService(String serviceName, String clusterName, String namespace, String projectName) throws IOException {
log.info(String.format("retrieveService clusterName is %s; namespace is %s; serviceName is %s; projectName is %s", clusterName, namespace, serviceName, projectName));
ServiceList serviceList = listService(clusterName, namespace, serviceName, projectName);
// api is not support find by name, so we do it .
ServiceDetails serviceDetails= serviceList.findByName(clusterName, namespace, serviceName);
if (serviceDetails == null){
log.warn(String.format("service %s in cluster %s:%s is not exist", serviceName, clusterName, namespace));
return null;
}
String serviceID = serviceDetails.getResource().getUuid();
log.info(String.format("retrieve service: serviceID is %s", serviceID));
String segment = prefixURL() + serviceID;
HttpUrl url = this.client.buildURL(segment);
String response = httpClient.get(url);
return JacksonUtil.json2Bean(response, ServiceDetails.class);
}
public String createService(ServiceCreatePayload payload) throws IOException {
String segment = prefixURL();
HttpUrl url = this.client.buildURL(segment);
String response = httpClient.post(url, JacksonUtil.bean2Json(payload));
JSONObject jsonObject = JSONObject.fromObject(response);
return jsonObject.getJSONObject("resource").getString("uuid");
}
public String updateService(String serviceID, ServiceUpdatePayload payload) throws IOException {
String segment = prefixURL() + serviceID;
HttpUrl url = this.client.buildURL(segment);
String data = JacksonUtil.bean2Json(payload);
httpClient.patch(url, data);
return serviceID;
}
public String updateService(String serviceName, String clusterName, String namespace, String projectName, ServiceUpdatePayload payload) throws IOException {
ServiceDetails details = this.retrieveService(serviceName, clusterName, namespace, projectName);
String serviceID = details.getResource().getUuid();
return this.updateService(serviceID, payload);
}
public void rollbackService(String serviceID) throws IOException {
String segment = prefixURL() + serviceID +"/rollback";
HttpUrl url = this.client.buildURL(segment);
httpClient.put(url, null);
return;
}
public void deleteService(String serviceID) throws IOException {
log.info(String.format("deleteService serviceID is %s; ", serviceID));
String segment = prefixURL() + serviceID;
HttpUrl url = this.client.buildURL(segment);
httpClient.delete(url);
}
public void deleteService(String serviceName, String clusterName, String namespace, String projectName) throws IOException {
log.info(String.format("deleteService clusterName is %s; namespace is %s; serviceName is %s; ", clusterName, namespace, serviceName));
ServiceDetails details = this.retrieveService(serviceName, clusterName, namespace, projectName);
if (details == null) {
throw new IOException(String.format("Service %s does not exist.", serviceName));
}
this.deleteService(details.getResource().getUuid());
}
public void startService(String serviceID) throws IOException {
log.info(String.format("startService serviceID is %s; ", serviceID));
}
public void startService(String serviceName, String clusterName, String namespace, String projectName) throws IOException {
log.info(String.format("startService clusterName is %s; namespace is %s; serviceName is %s; projectName is %s", clusterName, namespace, serviceName, projectName));
}
public void stopService(String serviceID) throws IOException {
log.info(String.format("stopService serviceID is %s; ", serviceID));
}
public void stopService(String serviceName, String clusterName, String namespace, String projectName) throws IOException {
log.info(String.format("stopService clusterName is %s; namespace is %s; serviceName is %s; projectName is %s", clusterName, namespace, serviceName, projectName));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy