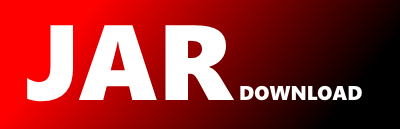
io.alauda.http.AlaudaHttp Maven / Gradle / Ivy
package io.alauda.http;
import okhttp3.*;
import okhttp3.internal.http.HttpMethod;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.function.*;
import java.io.IOException;
public class AlaudaHttp implements IHttpConstants {
private static final Logger log = LoggerFactory.getLogger(AlaudaHttp.class);
private static final MediaType JSON = MediaType.parse(MEDIATYPE_APPLICATION_JSON);
private static final OkHttpClient client = new OkHttpClient();
private String token;
public String getToken() {
return token;
}
public void setToken(String token) {
this.token = token;
}
public String prefixURL(String prefix, String namespace) {
return String.format("v1/%s/%s/", prefix, namespace);
}
public String prefixURLV2(String prefix) {
return String.format("v2/%s/", prefix);
}
public HttpUrl addParamsToUrl(HttpUrl url, String key, String value) {
HttpUrl.Builder builder = url.newBuilder();
builder.addQueryParameter(key, value);
return builder.build();
}
private Response getResponse(String method, HttpUrl url, String data) throws IOException {
log.info(String.format("Request method: %s; Request url: %s;\n Request data: %s", method, url, data));
Request.Builder builder = new Request.Builder()
.header("Authorization", "Token " + token)
.url(url);
if (data == null){
data = "";
}
RequestBody body = RequestBody.create(JSON, data);
switch (method) {
case "get":
break;
case "post":
builder.post(body);
break;
case "put":
builder.put(body);
break;
case "patch":
builder.patch(body);
break;
case "delete":
builder.delete();
break;
}
Request request = builder.build();
return client.newCall(request).execute();
}
private String httpMethodWithResponse(String method, HttpUrl url, String data) throws IOException {
return httpMethodWithResponse(method, url, data, null);
}
private String httpMethodWithResponse(String method, HttpUrl url, String data, Function acceptResponse) throws IOException {
if(acceptResponse == null){
acceptResponse = (response) -> { return response.isSuccessful();};
}
Response response = getResponse(method, url, data);
if (acceptResponse.apply(response)) {
log.info(String.format("Execute %s request successfully. code: %s", method, response.code()));
return response.body().string();
} else {
throw new IOException(String.format("Unexpected code %s \n%s", response, response.body().string()));
}
}
private void httpMethodWithoutResponse(String method, HttpUrl url, String data) throws IOException {
Response response = getResponse(method, url, data);
if (response.isSuccessful()) {
log.info(String.format("Execute %s request successfully. code: %s", method, response.code()));
response.close();
} else {
throw new IOException(String.format("Unexpected code %s \n, content:%s", response, response.body().string()));
}
}
public Response request(String method, HttpUrl url, String data) throws IOException {
return getResponse(method, url, data);
}
public String get(HttpUrl url) throws IOException {
return httpMethodWithResponse("get", url, "");
}
public String get(HttpUrl url, Function acceptResponse) throws IOException {
return httpMethodWithResponse("get", url, "", acceptResponse);
}
public String post(HttpUrl url, String data) throws IOException {
return httpMethodWithResponse("post", url, data);
}
public void put(HttpUrl url, String data) throws IOException {
httpMethodWithoutResponse("put", url, data);
}
public void patch(HttpUrl url, String data) throws IOException {
httpMethodWithoutResponse("patch", url, data);
}
public void delete(HttpUrl url) throws IOException {
httpMethodWithoutResponse("delete", url, "");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy