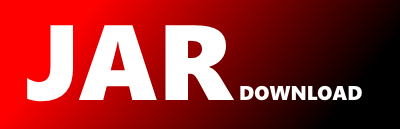
io.alauda.devops.client.AlaudaDevOpsAdapterSupport Maven / Gradle / Ivy
/**
* Copyright (C) 2018 Alauda
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.alauda.devops.client;
import io.alauda.kubernetes.api.model.RootPaths;
import io.alauda.kubernetes.client.Client;
import io.alauda.kubernetes.client.Config;
import io.alauda.kubernetes.client.KubernetesClientException;
import java.net.URI;
import java.net.URL;
import java.util.List;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
public class AlaudaDevOpsAdapterSupport {
static final ConcurrentMap IS_ALAUDA_KUBERNETES = new ConcurrentHashMap<>();
static final ConcurrentMap USES_ALAUDA_APIGROUPS = new ConcurrentHashMap<>();
public Boolean isAdaptable(Client client) {
AlaudaDevOpsConfig config = new AlaudaDevOpsConfig(client.getConfiguration());
if (!hasCustomKubernetesUrl(config) && !isAlaudaKubernetes(client)) {
return false;
}
return true;
}
/**
* Check if Alauda Kubernetes apis are available.
* @param client The client.
* @return True if alauda k8s is found in the root paths.
*/
static boolean isAlaudaKubernetes(Client client) {
URL masterUrl = client.getMasterUrl();
if (IS_ALAUDA_KUBERNETES.containsKey(masterUrl)) {
return IS_ALAUDA_KUBERNETES.get(masterUrl);
} else {
RootPaths rootPaths = client.rootPaths();
if (rootPaths != null) {
List paths = rootPaths.getPaths();
if (paths != null) {
for (String path : paths) {
// lets detect the new API Groups APIs for OpenShift
if (path.endsWith(".alauda.io") || path.contains(".alauda.io/")) {
USES_ALAUDA_APIGROUPS.putIfAbsent(masterUrl, true);
IS_ALAUDA_KUBERNETES.putIfAbsent(masterUrl, true);
return true;
}
}
}
}
}
IS_ALAUDA_KUBERNETES.putIfAbsent(masterUrl, false);
return false;
}
/**
* Check if Alauda API Groups are available
* @param client The client.
* @return True if the new /apis/*.alauda.io/
APIs are found in the root paths.
*/
static boolean isAlaudaAPIGroups(Client client) {
Config configuration = client.getConfiguration();
if (configuration instanceof AlaudaDevOpsConfig) {
AlaudaDevOpsConfig alaudaDevOpsConfig = (AlaudaDevOpsConfig) configuration;
if (alaudaDevOpsConfig.isDisableApiGroupCheck()) {
return false;
}
}
URL masterUrl = client.getMasterUrl();
if (isAlaudaKubernetes(client) && USES_ALAUDA_APIGROUPS.containsKey(masterUrl)) {
return USES_ALAUDA_APIGROUPS.get(masterUrl);
}
return false;
}
/**
* Checks if a custom URL for Kubernetes has been used.
* @param config The AlaudaDevOps configuration.
* @return True if both master and AlaudaDevOps url have the same root.
*/
static boolean hasCustomKubernetesUrl(AlaudaDevOpsConfig config) {
try {
URI masterUri = new URI(config.getMasterUrl()).resolve("/");
URI kubernetesUri = new URI(config.getKubernetesUrl()).resolve("/");
return !masterUri.equals(kubernetesUri);
} catch (Exception e) {
throw KubernetesClientException.launderThrowable(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy