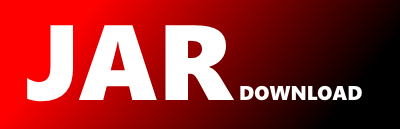
io.alauda.devops.client.dsl.internal.AlaudaOperation Maven / Gradle / Ivy
/**
* Copyright (C) 2018 Alauda
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.alauda.devops.client.dsl.internal;
import io.alauda.kubernetes.api.model.Doneable;
import io.alauda.kubernetes.api.model.HasMetadata;
import io.alauda.kubernetes.api.model.KubernetesResourceList;
import io.alauda.kubernetes.client.Config;
import io.alauda.kubernetes.client.KubernetesClientException;
import io.alauda.kubernetes.client.dsl.Resource;
import io.alauda.kubernetes.client.dsl.base.HasMetadataOperation;
import io.alauda.kubernetes.client.dsl.base.ConfigAndApiGroupsInfo;
import io.alauda.kubernetes.client.utils.URLUtils;
import io.alauda.devops.client.AlaudaAPIGroups;
import io.alauda.devops.client.DefaultAlaudaDevOpsClient;
import io.alauda.devops.client.AlaudaDevOpsClient;
import io.alauda.devops.client.AlaudaDevOpsConfig;
import okhttp3.OkHttpClient;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Map;
public class AlaudaOperation, R extends Resource>
extends HasMetadataOperation {
protected AlaudaOperation(OkHttpClient client, ConfigAndApiGroupsInfo config, String resourceT, String namespace, String name, Boolean cascading, T item, String resourceVersion, Boolean reloadingFromServer, long gracePeriodSeconds, Map labels, Map labelsNot, Map labelsIn, Map labelsNotIn, Map fields) {
super(client, config.getConfig(), config.getApiGroup(), config.getApiGroupVersion(), resourceT, namespace, name, cascading, item, resourceVersion, reloadingFromServer, gracePeriodSeconds, labels, labelsNot, labelsIn, labelsNotIn, fields);
this.apiGroupVersion = config.getApiGroupVersion();
}
/**
* If the current client supports the new API Group REST API at /apis/*.devops.io/v1
* then lets use that URL otherwise lets stick to the legacy /oapi/v1
API
*
* @param alaudaDevOpsClient the AlaudaDevops client to use
* @param apiGroupName the API Group name like devops.alauda.io
or charts.alauda.io
* @param config the current configuration
* @return the current configuration if API groups are not supported otherwise the new configuration
*/
public static ConfigAndApiGroupsInfo withApiGroup(AlaudaDevOpsClient alaudaDevOpsClient, String apiGroupName, String apiVersion, AlaudaDevOpsConfig config) {
String oapiVersion = config.getAlaudaAPIVersion();
if (apiVersion == null) {
apiVersion = oapiVersion;
}
if (config.isAlaudaAPIGroups(alaudaDevOpsClient)) {
String apiGroupUrl = URLUtils.join(config.getMasterUrl(), "apis", apiGroupName, apiVersion);
String apiGroupVersion = URLUtils.join(apiGroupName, apiVersion);
return new ConfigAndApiGroupsInfo(new AlaudaDevOpsConfig(config, apiGroupUrl), apiGroupName, apiGroupVersion);
} else {
if (apiVersion == null) {
apiVersion = oapiVersion;
}
return new ConfigAndApiGroupsInfo(config, apiGroupName, apiVersion);
}
}
/**
* If the current client supports the new API Group REST API at /apis/*.devops.io/v1
* then lets use that URL otherwise lets stick to the legacy /oapi/v1
API
*
* @param httpClient the HTTP client to use
* @param apiGroupName the API Group name like devops.alauda.io
or charts.alauda.io
* @param config the current configuration
* @return the current configuration if API groups are not supported otherwise the new configuration
*/
public static ConfigAndApiGroupsInfo withApiGroup(OkHttpClient httpClient, String apiGroupName, String apiVersion, AlaudaDevOpsConfig config) {
AlaudaDevOpsClient alaudaDevOpsClient = new DefaultAlaudaDevOpsClient(httpClient, config);
String defaultApiVersion = AlaudaAPIGroups.getVersionByAPIGroup(apiGroupName);
if (defaultApiVersion != null && (apiVersion == null || apiVersion.isEmpty()) && defaultApiVersion != apiVersion) {
apiVersion = defaultApiVersion;
}
return withApiGroup(alaudaDevOpsClient, apiGroupName, apiVersion, config);
}
@Override
public URL getRootUrl() {
try {
return new URL(AlaudaDevOpsConfig.wrap(getConfig()).getKubernetesUrl());
} catch (MalformedURLException e) {
throw KubernetesClientException.launderThrowable(e);
}
}
@Override
public AlaudaOperation inNamespace(String namespace) {
try {
return getClass()
.getConstructor(OkHttpClient.class, AlaudaDevOpsConfig.class, String.class, String.class, String.class, Boolean.class, getType(), String.class, Boolean.class, long.class, Map.class, Map.class, Map.class, Map.class, Map.class)
.newInstance(client, getConfig(), getAPIVersion(), namespace, getName(), isCascading(), getItem(), getResourceVersion(), isReloadingFromServer(), getGracePeriodSeconds(), getLabels(), getLabelsNot(), getLabelsIn(), getLabelsNotIn(), getFields());
} catch (Throwable t) {
throw KubernetesClientException.launderThrowable(t);
}
}
@Override
public AlaudaDevOpsConfig getConfig() {
return AlaudaDevOpsConfig.wrap(super.getConfig());
}
protected Class extends Config> getConfigType() {
return AlaudaDevOpsConfig.class;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy