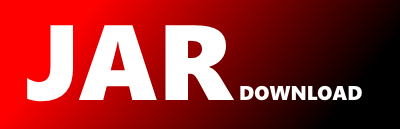
io.alauda.devops.client.dsl.internal.ProjectRequestsOperationImpl Maven / Gradle / Ivy
/**
* Copyright (C) 2018 io.alauda.kuberneteslauda
*
* Licensed under the io.alauda.kubernetespache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "io.alauda.kubernetesS IS" Bio.alauda.kubernetesSIS,
* WITHOUT Wio.alauda.kubernetesRRio.alauda.kubernetesNTIES OR CONDITIONS OF io.alauda.kubernetesNY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.alauda.devops.client.dsl.internal;
import io.alauda.devops.client.AlaudaDevOpsConfig;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import io.alauda.kubernetes.api.builder.Function;
import io.alauda.kubernetes.api.model.Status;
import io.alauda.kubernetes.client.KubernetesClientException;
import io.alauda.kubernetes.client.dsl.base.OperationSupport;
import io.fabric8.openshift.api.model.DoneableProjectRequest;
import io.fabric8.openshift.api.model.ProjectRequest;
import io.alauda.devops.client.dsl.ProjectRequestOperation;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.concurrent.ExecutionException;
import static io.alauda.devops.client.AlaudaAPIGroups.PROJECT;
public class ProjectRequestsOperationImpl extends OperationSupport implements ProjectRequestOperation {
private final ProjectRequest item;
public ProjectRequestsOperationImpl(OkHttpClient client, AlaudaDevOpsConfig config) {
this(client, config, null, null);
}
public ProjectRequestsOperationImpl(OkHttpClient client, AlaudaDevOpsConfig config, String apiVersion, ProjectRequest item) {
super(client, AlaudaOperation.withApiGroup(client, PROJECT, apiVersion, config), "projectrequests", null, null);
this.item = item;
}
@Override
public boolean isResourceNamespaced() {
return false;
}
@Override
public URL getRootUrl() {
try {
return new URL(AlaudaDevOpsConfig.wrap(getConfig()).getKubernetesUrl());
} catch (MalformedURLException e) {
throw KubernetesClientException.launderThrowable(e);
}
}
private ProjectRequest updateApiVersion(ProjectRequest p) {
p.setApiVersion(this.apiVersion);
return p;
}
@Override
public ProjectRequest create(ProjectRequest... resources) {
try {
if (resources.length > 1) {
throw new IllegalArgumentException("Too many items to create.");
} else if (resources.length == 1) {
return handleCreate(updateApiVersion(resources[0]), ProjectRequest.class);
} else if (getItem() == null) {
throw new IllegalArgumentException("Nothing to create.");
} else {
return handleCreate(updateApiVersion(getItem()), ProjectRequest.class);
}
} catch (InterruptedException | ExecutionException | IOException e) {
throw KubernetesClientException.launderThrowable(e);
}
}
@Override
public DoneableProjectRequest createNew() {
return new DoneableProjectRequest(new Function() {
@Override
public ProjectRequest apply(ProjectRequest item) {
try {
return create(item);
} catch (Exception e) {
throw KubernetesClientException.launderThrowable(e);
}
}
});
}
@Override
public Status list() {
try {
URL requestUrl = getNamespacedUrl();
Request.Builder requestBuilder = new Request.Builder().get().url(requestUrl);
return handleResponse(requestBuilder, Status.class);
} catch (InterruptedException | ExecutionException | IOException e) {
throw KubernetesClientException.launderThrowable(e);
}
}
public ProjectRequest getItem() {
return item;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy