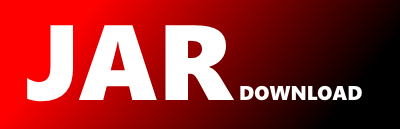
io.alauda.devops.client.dsl.internal.SubjectAccessReviewOperationImpl Maven / Gradle / Ivy
/**
* Copyright (C) 2018 Alauda
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.alauda.devops.client.dsl.internal;
import io.alauda.devops.client.AlaudaAPIGroups;
import io.alauda.devops.client.AlaudaDevOpsConfig;
import io.alauda.devops.client.dsl.CreateableLocalSubjectAccessReview;
import io.alauda.devops.client.dsl.CreateableSubjectAccessReview;
import io.alauda.devops.client.dsl.SubjectAccessReviewOperation;
import okhttp3.OkHttpClient;
import io.alauda.kubernetes.api.model.HasMetadata;
import io.alauda.kubernetes.client.KubernetesClientException;
import io.alauda.kubernetes.client.dsl.base.OperationSupport;
import io.alauda.kubernetes.client.utils.Utils;
import io.fabric8.openshift.api.model.LocalSubjectAccessReview;
import io.fabric8.openshift.api.model.LocalSubjectAccessReviewBuilder;
import io.fabric8.openshift.api.model.SubjectAccessReview;
import io.fabric8.openshift.api.model.SubjectAccessReviewBuilder;
import io.fabric8.openshift.api.model.SubjectAccessReviewResponse;
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.concurrent.ExecutionException;
public class SubjectAccessReviewOperationImpl extends OperationSupport implements SubjectAccessReviewOperation {
public SubjectAccessReviewOperationImpl(OkHttpClient client, AlaudaDevOpsConfig config) {
this(client, config, null, null);
}
public SubjectAccessReviewOperationImpl(OkHttpClient client, AlaudaDevOpsConfig config, String apiVersion, String namespace) {
super(client, AlaudaOperation.withApiGroup(client, AlaudaAPIGroups.AUTHORIZATION, apiVersion, config), "subjectaccessreviews", namespace, null);
}
@Override
public CreateableLocalSubjectAccessReview inNamespace(String namespace) {
return new SubjectAccessReviewOperationImpl(client, AlaudaDevOpsConfig.wrap(getConfig()), null, namespace).local();
}
@Override
public SubjectAccessReviewResponse create(SubjectAccessReview... item) {
return new CreateableSubjectAccessReviewImpl(client).create(item);
}
@Override
public CreateableSubjectAccessReview createNew() {
return new CreateableSubjectAccessReviewImpl(client).createNew();
}
@Override
public URL getRootUrl() {
try {
return new URL(AlaudaDevOpsConfig.wrap(getConfig()).getKubernetesUrl());
} catch (MalformedURLException e) {
throw KubernetesClientException.launderThrowable(e);
}
}
private class CreateableLocalSubjectAccessReviewImpl extends CreateableLocalSubjectAccessReview {
private final OkHttpClient client;
private final LocalSubjectAccessReviewBuilder builder;
private CreateableLocalSubjectAccessReviewImpl(OkHttpClient client) {
this.client = client;
this.builder = new LocalSubjectAccessReviewBuilder(CreateableLocalSubjectAccessReviewImpl.this);
}
private CreateableLocalSubjectAccessReviewImpl(OkHttpClient client, LocalSubjectAccessReviewBuilder builder) {
this.client = client;
this.builder = builder;
}
@Override
public SubjectAccessReviewResponse create(LocalSubjectAccessReview... resources) {
try {
if (resources.length > 1) {
throw new IllegalArgumentException("Too many items to create.");
} else if (resources.length == 1) {
return handleCreate(resources[0], SubjectAccessReviewResponse.class);
} else {
throw new IllegalArgumentException("Nothing to create.");
}
} catch (InterruptedException | ExecutionException | IOException e) {
throw KubernetesClientException.launderThrowable(e);
}
}
@Override
public CreateableLocalSubjectAccessReview createNew() {
return this;
}
@Override
public SubjectAccessReviewResponse done() {
return create(builder.build());
}
}
private CreateableLocalSubjectAccessReview local() {
return new CreateableLocalSubjectAccessReviewImpl(client);
}
//Subject Access Review is a category on its own so we need to override the default behavior.
@Override
protected String checkNamespace(T item) {
String operationNs = getNamespace();
String itemNs = (item instanceof HasMetadata && ((HasMetadata)item).getMetadata() != null) ? ((HasMetadata) item).getMetadata().getNamespace() : null;
if (Utils.isNullOrEmpty(operationNs) && Utils.isNullOrEmpty(itemNs)) {
return null;
} else if (Utils.isNullOrEmpty(itemNs)) {
return operationNs;
} else if (Utils.isNullOrEmpty(operationNs)) {
return itemNs;
} else if (itemNs.equals(operationNs)) {
return itemNs;
}
throw new KubernetesClientException("Namespace mismatch. Item namespace:" + itemNs + ". Operation namespace:" + operationNs + ".");
}
private class CreateableSubjectAccessReviewImpl extends CreateableSubjectAccessReview {
private final OkHttpClient client;
private final SubjectAccessReviewBuilder builder;
private CreateableSubjectAccessReviewImpl(OkHttpClient client) {
this.client = client;
this.builder = new SubjectAccessReviewBuilder(CreateableSubjectAccessReviewImpl.this);
}
private CreateableSubjectAccessReviewImpl(OkHttpClient client, SubjectAccessReviewBuilder builder) {
this.client = client;
this.builder = builder;
}
@Override
public SubjectAccessReviewResponse create(SubjectAccessReview... resources) {
try {
if (resources.length > 1) {
throw new IllegalArgumentException("Too many items to create.");
} else if (resources.length == 1) {
return handleCreate(resources[0], SubjectAccessReviewResponse.class);
} else {
throw new IllegalArgumentException("Nothing to create.");
}
} catch (InterruptedException | ExecutionException | IOException e) {
throw KubernetesClientException.launderThrowable(e);
}
}
@Override
public CreateableSubjectAccessReview createNew() {
return this;
}
@Override
public SubjectAccessReviewResponse done() {
return create(builder.build());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy