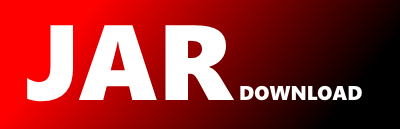
io.alauda.devops.client.dsl.internal.TemplateOperationsImpl Maven / Gradle / Ivy
/**
* Copyright (C) 2018 Alauda
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.alauda.devops.client.dsl.internal;
import com.fasterxml.jackson.core.type.TypeReference;
import com.mifmif.common.regex.Generex;
import io.alauda.kubernetes.api.model.HasMetadata;
import io.alauda.kubernetes.api.model.KubernetesList;
import io.alauda.kubernetes.api.model.KubernetesListBuilder;
import io.alauda.kubernetes.api.model.KubernetesResourceList;
import io.alauda.kubernetes.client.KubernetesClientException;
import io.alauda.kubernetes.client.dsl.MixedOperation;
import io.alauda.kubernetes.client.utils.Serialization;
import io.alauda.kubernetes.client.utils.URLUtils;
import io.alauda.kubernetes.client.utils.Utils;
import io.fabric8.openshift.api.model.DoneableTemplate;
import io.fabric8.openshift.api.model.Parameter;
import io.fabric8.openshift.api.model.Template;
import io.fabric8.openshift.api.model.TemplateBuilder;
import io.fabric8.openshift.api.model.TemplateList;
import io.alauda.devops.client.AlaudaDevOpsConfig;
import io.alauda.devops.client.ParameterValue;
import io.alauda.devops.client.dsl.TemplateOperation;
import io.alauda.devops.client.dsl.TemplateResource;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
import java.util.concurrent.ExecutionException;
import static io.alauda.devops.client.AlaudaAPIGroups.TEMPLATE;
public class TemplateOperationsImpl
extends AlaudaOperation>
implements TemplateOperation {
private static final String EXPRESSION = "expression";
private static final TypeReference> MAPS_REFERENCE = new TypeReference>() {
};
private final Map parameters;
public TemplateOperationsImpl(OkHttpClient client, AlaudaDevOpsConfig config, String namespace) {
this(client, config, null, namespace, null, true, null, null, false, -1, new TreeMap(), new TreeMap(), new TreeMap(), new TreeMap(), new TreeMap());
}
public TemplateOperationsImpl(OkHttpClient client, AlaudaDevOpsConfig config, String apiVersion, String namespace, String name, Boolean cascading, Template item, String resourceVersion, Boolean reloadingFromServer, long gracePeriodSeconds, Map labels, Map labelsNot, Map labelsIn, Map labelsNotIn, Map fields) {
this(client, config, apiVersion, "templates", namespace, name, cascading, item, resourceVersion, reloadingFromServer, gracePeriodSeconds, labels, labelsNot, labelsIn, labelsNotIn, fields, null);
}
public TemplateOperationsImpl(OkHttpClient client, AlaudaDevOpsConfig config, String apiVersion, String namespace, String name, Boolean cascading, Template item, String resourceVersion, Boolean reloadingFromServer, long gracePeriodSeconds, Map labels, Map labelsNot, Map labelsIn, Map labelsNotIn, Map fields, Map parameters) {
this(client, config, apiVersion, "templates", namespace, name, cascading, item, resourceVersion, reloadingFromServer, gracePeriodSeconds, labels, labelsNot, labelsIn, labelsNotIn, fields, parameters);
}
public TemplateOperationsImpl(OkHttpClient client, AlaudaDevOpsConfig config, String apiVersion, String resourceT, String namespace, String name, Boolean cascading, Template item, String resourceVersion, Boolean reloadingFromServer, long gracePeriodSeconds, Map labels, Map labelsNot, Map labelsIn, Map labelsNotIn, Map fields, Map parameters) {
super(client, AlaudaOperation.withApiGroup(client, TEMPLATE, apiVersion, config), resourceT, namespace, name, cascading, item, resourceVersion, reloadingFromServer, gracePeriodSeconds, labels, labelsNot, labelsIn, labelsNotIn, fields);
this.parameters = parameters;
}
@Override
public KubernetesList process(File f) {
try (FileInputStream is = new FileInputStream(f)) {
return process(is);
} catch (IOException e) {
throw KubernetesClientException.launderThrowable(forOperationType("process"), e);
}
}
@Override
public KubernetesList process(InputStream is) {
return process(unmarshal(is, MAPS_REFERENCE));
}
@Override
public KubernetesList process(Map valuesMap) {
Template t = get();
try {
List parameters = t.getParameters();
if (parameters != null) {
for (Parameter p : parameters) {
String v = valuesMap.get(p.getName());
if (v != null) {
p.setGenerate(null);
p.setValue(v);
}
}
}
RequestBody body = RequestBody.create(JSON, JSON_MAPPER.writeValueAsString(t));
Request.Builder requestBuilder = new Request.Builder().post(body).url(getProcessUrl());
t = handleResponse(requestBuilder);
KubernetesList l = new KubernetesList();
l.setItems(t.getObjects());
return l;
} catch (Exception e) {
throw KubernetesClientException.launderThrowable(forOperationType("process"), e);
}
}
@Override
public KubernetesList process(ParameterValue... values) {
Map valuesMap = new HashMap<>(values.length);
for (ParameterValue pv : values) {
valuesMap.put(pv.getName(), pv.getValue());
}
return process(valuesMap);
}
@Override
public KubernetesList processLocally(File f) {
try (FileInputStream is = new FileInputStream(f)) {
return processLocally(is);
} catch (IOException e) {
throw KubernetesClientException.launderThrowable(forOperationType("processLocally"), e);
}
}
@Override
public KubernetesList processLocally(InputStream is) {
return processLocally(unmarshal(is, MAPS_REFERENCE));
}
@Override
public KubernetesList processLocally(ParameterValue... values) {
Map valuesMap = new HashMap<>(values.length);
for (ParameterValue pv : values) {
valuesMap.put(pv.getName(), pv.getValue());
}
return processLocally(valuesMap);
}
@Override
public MixedOperation> withParameters(Map parameters) {
return new TemplateOperationsImpl(client, AlaudaDevOpsConfig.wrap(config), getAPIVersion(), namespace, getName(), isCascading(), getItem(), getResourceVersion(), isReloadingFromServer(), getGracePeriodSeconds(),
getLabels(), getLabelsNot(), getLabelsIn(), getLabelsNotIn(), getFields(), parameters);
}
public KubernetesList processLocally(Map valuesMap) {
String namespace = getItem() != null ? getItem().getMetadata().getNamespace() : getNamespace();
if (namespace == null) {
namespace = getConfig().getNamespace();
}
String name = getItem() != null ? getItem().getMetadata().getName() : getName();
Template t = withParameters(valuesMap)
.inNamespace(namespace)
.withName(name)
.get();
List parameters = t != null ? t.getParameters() : null;
KubernetesList list = new KubernetesListBuilder()
.withItems(t != null && t.getObjects() != null ? t.getObjects() : Collections.emptyList())
.build();
try {
String json = JSON_MAPPER.writeValueAsString(list);
if (parameters != null && !parameters.isEmpty()) {
// lets make a few passes in case there's expressions in values
for (int i = 0; i < 5; i++) {
for (Parameter parameter : parameters) {
String parameterName = parameter.getName();
String regex = "${" + parameterName + "}";
String parameterValue;
if (valuesMap.containsKey(parameterName)) {
parameterValue = valuesMap.get(parameterName);
} else if (Utils.isNotNullOrEmpty(parameter.getValue())) {
parameterValue = parameter.getValue();
} else if (EXPRESSION.equals(parameter.getGenerate())) {
Generex generex = new Generex(parameter.getFrom());
parameterValue = generex.random();
} else if (parameter.getRequired() == null || !parameter.getRequired()) {
parameterValue = "";
} else {
throw new IllegalArgumentException("No value available for parameter name: " + parameterName);
}
json = json.replace(regex, parameterValue);
}
}
}
list = JSON_MAPPER.readValue(json, KubernetesList.class);
} catch (IOException e) {
throw KubernetesClientException.launderThrowable(e);
}
return list;
}
private URL getProcessUrl() throws MalformedURLException {
URL requestUrl = getRootUrl();
if (getNamespace() != null) {
requestUrl = new URL(URLUtils.join(requestUrl.toString(), "namespaces" , getNamespace()));
}
requestUrl = new URL(URLUtils.join(requestUrl.toString(), "processedtemplates"));
return requestUrl;
}
@Override
public TemplateResource load(InputStream is) {
String generatedName = Utils.randomString("template-", 10);
Template template = null;
Object item = Serialization.unmarshal(is, parameters);
if (item instanceof Template) {
template = (Template) item;
} else if (item instanceof HasMetadata) {
HasMetadata h = (HasMetadata) item;
template = new TemplateBuilder()
.withNewMetadata()
.withName(generatedName)
.withNamespace(h != null && h.getMetadata() != null ? h.getMetadata().getNamespace() : null)
.endMetadata()
.withObjects(h).build();
} else if (item instanceof KubernetesResourceList) {
List list = ((KubernetesResourceList) item).getItems();
template = new TemplateBuilder()
.withNewMetadata()
.withName(generatedName)
.endMetadata()
.withObjects(list.toArray(new HasMetadata[list.size()])).build();
} else if (item instanceof HasMetadata[]) {
template = new TemplateBuilder()
.withNewMetadata()
.withName(generatedName)
.endMetadata()
.withObjects((HasMetadata[]) item).build();
} else if (item instanceof Collection) {
List items = new ArrayList<>();
for (Object o : (Collection) item) {
if (o instanceof HasMetadata) {
items.add((HasMetadata) o);
}
}
template = new TemplateBuilder()
.withNewMetadata()
.withName(generatedName)
.endMetadata()
.withObjects(items.toArray(new HasMetadata[items.size()])).build();
}
return new TemplateOperationsImpl(client, AlaudaDevOpsConfig.wrap(config), null, namespace, null, false, template, null, false, 0L,
null, null, null, null, null, null);
}
@Override
public TemplateResource withName(String name) {
if (name == null || name.length() == 0) {
throw new IllegalArgumentException("Name must be provided.");
}
return new TemplateOperationsImpl(client, AlaudaDevOpsConfig.wrap(config), getAPIVersion(), namespace, name, isCascading(), getItem(), getResourceVersion(), isReloadingFromServer(), getGracePeriodSeconds(),
getLabels(), getLabelsNot(), getLabelsIn(), getLabelsNotIn(), getFields(), parameters);
}
@Override
public AlaudaOperation> inNamespace(String namespace) {
return new TemplateOperationsImpl(client, AlaudaDevOpsConfig.wrap(config), getAPIVersion(), namespace, name, isCascading(), getItem(), getResourceVersion(), isReloadingFromServer(), getGracePeriodSeconds(),
getLabels(), getLabelsNot(), getLabelsIn(), getLabelsNotIn(), getFields(), parameters);
}
@Override
protected Template handleGet(URL resourceUrl) throws InterruptedException, ExecutionException, IOException {
return super.handleGet(resourceUrl, getType(), this.parameters);
}
protected T handleResponse(Request.Builder requestBuilder, Class type) throws ExecutionException, InterruptedException, KubernetesClientException, IOException {
return handleResponse(requestBuilder, type, parameters);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy