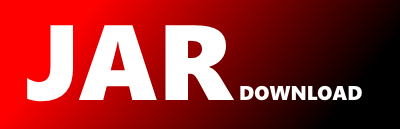
io.alauda.devops.api.model.BuildSource Maven / Gradle / Ivy
package io.alauda.devops.api.model;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.Generated;
import javax.validation.Valid;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import io.alauda.kubernetes.api.model.Doneable;
import io.alauda.kubernetes.api.model.KubernetesResource;
import io.alauda.kubernetes.api.model.LocalObjectReference;
import io.sundr.builder.annotations.Buildable;
import io.sundr.builder.annotations.Inline;
import lombok.EqualsAndHashCode;
import lombok.ToString;
/**
*
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@Generated("org.jsonschema2pojo")
@JsonPropertyOrder({
"apiVersion",
"kind",
"metadata",
"binary",
"contextDir",
"dockerfile",
"git",
"images",
"secrets",
"sourceSecret",
"type"
})
@JsonDeserialize(using = com.fasterxml.jackson.databind.JsonDeserializer.None.class)
@ToString
@EqualsAndHashCode
@Buildable(editableEnabled = false, validationEnabled = true, generateBuilderPackage = true, builderPackage = "io.alauda.kubernetes.api.builder", inline = @Inline(type = Doneable.class, prefix = "Doneable", value = "done"))
public class BuildSource implements KubernetesResource
{
/**
*
*
*/
@JsonProperty("binary")
@Valid
private BinaryBuildSource binary;
/**
*
*
*/
@JsonProperty("contextDir")
private String contextDir;
/**
*
*
*/
@JsonProperty("dockerfile")
private String dockerfile;
/**
*
*
*/
@JsonProperty("git")
@Valid
private GitBuildSource git;
/**
*
*
*/
@JsonProperty("images")
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@Valid
private List images = new ArrayList();
/**
*
*
*/
@JsonProperty("secrets")
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@Valid
private List secrets = new ArrayList();
/**
*
*
*/
@JsonProperty("sourceSecret")
@Valid
private LocalObjectReference sourceSecret;
/**
*
*
*/
@JsonProperty("type")
private String type;
@JsonIgnore
private Map additionalProperties = new HashMap();
/**
* No args constructor for use in serialization
*
*/
public BuildSource() {
}
/**
*
* @param sourceSecret
* @param images
* @param git
* @param binary
* @param dockerfile
* @param type
* @param secrets
* @param contextDir
*/
public BuildSource(BinaryBuildSource binary, String contextDir, String dockerfile, GitBuildSource git, List images, List secrets, LocalObjectReference sourceSecret, String type) {
this.binary = binary;
this.contextDir = contextDir;
this.dockerfile = dockerfile;
this.git = git;
this.images = images;
this.secrets = secrets;
this.sourceSecret = sourceSecret;
this.type = type;
}
/**
*
*
* @return
* The binary
*/
@JsonProperty("binary")
public BinaryBuildSource getBinary() {
return binary;
}
/**
*
*
* @param binary
* The binary
*/
@JsonProperty("binary")
public void setBinary(BinaryBuildSource binary) {
this.binary = binary;
}
/**
*
*
* @return
* The contextDir
*/
@JsonProperty("contextDir")
public String getContextDir() {
return contextDir;
}
/**
*
*
* @param contextDir
* The contextDir
*/
@JsonProperty("contextDir")
public void setContextDir(String contextDir) {
this.contextDir = contextDir;
}
/**
*
*
* @return
* The dockerfile
*/
@JsonProperty("dockerfile")
public String getDockerfile() {
return dockerfile;
}
/**
*
*
* @param dockerfile
* The dockerfile
*/
@JsonProperty("dockerfile")
public void setDockerfile(String dockerfile) {
this.dockerfile = dockerfile;
}
/**
*
*
* @return
* The git
*/
@JsonProperty("git")
public GitBuildSource getGit() {
return git;
}
/**
*
*
* @param git
* The git
*/
@JsonProperty("git")
public void setGit(GitBuildSource git) {
this.git = git;
}
/**
*
*
* @return
* The images
*/
@JsonProperty("images")
public List getImages() {
return images;
}
/**
*
*
* @param images
* The images
*/
@JsonProperty("images")
public void setImages(List images) {
this.images = images;
}
/**
*
*
* @return
* The secrets
*/
@JsonProperty("secrets")
public List getSecrets() {
return secrets;
}
/**
*
*
* @param secrets
* The secrets
*/
@JsonProperty("secrets")
public void setSecrets(List secrets) {
this.secrets = secrets;
}
/**
*
*
* @return
* The sourceSecret
*/
@JsonProperty("sourceSecret")
public LocalObjectReference getSourceSecret() {
return sourceSecret;
}
/**
*
*
* @param sourceSecret
* The sourceSecret
*/
@JsonProperty("sourceSecret")
public void setSourceSecret(LocalObjectReference sourceSecret) {
this.sourceSecret = sourceSecret;
}
/**
*
*
* @return
* The type
*/
@JsonProperty("type")
public String getType() {
return type;
}
/**
*
*
* @param type
* The type
*/
@JsonProperty("type")
public void setType(String type) {
this.type = type;
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy