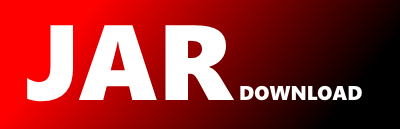
io.alauda.devops.api.model.RoleBindingRestrictionSpecFluentImpl Maven / Gradle / Ivy
package io.alauda.devops.api.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.alauda.kubernetes.api.builder.Nested;
import java.lang.Deprecated;
import io.alauda.kubernetes.api.builder.BaseFluent;
import java.lang.Boolean;
import javax.validation.Valid;
import java.lang.Object;
public class RoleBindingRestrictionSpecFluentImpl> extends io.alauda.kubernetes.api.builder.BaseFluent implements RoleBindingRestrictionSpecFluent{
private GroupRestrictionBuilder grouprestriction;
private ServiceAccountRestrictionBuilder serviceaccountrestriction;
private UserRestrictionBuilder userrestriction;
public RoleBindingRestrictionSpecFluentImpl(){
}
public RoleBindingRestrictionSpecFluentImpl(RoleBindingRestrictionSpec instance){
this.withGrouprestriction(instance.getGrouprestriction());
this.withServiceaccountrestriction(instance.getServiceaccountrestriction());
this.withUserrestriction(instance.getUserrestriction());
}
/**
* This method has been deprecated, please use method buildGrouprestriction instead.
*/
@Deprecated public GroupRestriction getGrouprestriction(){
return this.grouprestriction!=null?this.grouprestriction.build():null;
}
public GroupRestriction buildGrouprestriction(){
return this.grouprestriction!=null?this.grouprestriction.build():null;
}
public A withGrouprestriction(GroupRestriction grouprestriction){
_visitables.remove(this.grouprestriction);
if (grouprestriction!=null){ this.grouprestriction= new GroupRestrictionBuilder(grouprestriction); _visitables.add(this.grouprestriction);} return (A) this;
}
public Boolean hasGrouprestriction(){
return this.grouprestriction!=null;
}
public RoleBindingRestrictionSpecFluent.GrouprestrictionNested withNewGrouprestriction(){
return new GrouprestrictionNestedImpl();
}
public RoleBindingRestrictionSpecFluent.GrouprestrictionNested withNewGrouprestrictionLike(GroupRestriction item){
return new GrouprestrictionNestedImpl(item);
}
public RoleBindingRestrictionSpecFluent.GrouprestrictionNested editGrouprestriction(){
return withNewGrouprestrictionLike(getGrouprestriction());
}
public RoleBindingRestrictionSpecFluent.GrouprestrictionNested editOrNewGrouprestriction(){
return withNewGrouprestrictionLike(getGrouprestriction() != null ? getGrouprestriction(): new GroupRestrictionBuilder().build());
}
public RoleBindingRestrictionSpecFluent.GrouprestrictionNested editOrNewGrouprestrictionLike(GroupRestriction item){
return withNewGrouprestrictionLike(getGrouprestriction() != null ? getGrouprestriction(): item);
}
/**
* This method has been deprecated, please use method buildServiceaccountrestriction instead.
*/
@Deprecated public ServiceAccountRestriction getServiceaccountrestriction(){
return this.serviceaccountrestriction!=null?this.serviceaccountrestriction.build():null;
}
public ServiceAccountRestriction buildServiceaccountrestriction(){
return this.serviceaccountrestriction!=null?this.serviceaccountrestriction.build():null;
}
public A withServiceaccountrestriction(ServiceAccountRestriction serviceaccountrestriction){
_visitables.remove(this.serviceaccountrestriction);
if (serviceaccountrestriction!=null){ this.serviceaccountrestriction= new ServiceAccountRestrictionBuilder(serviceaccountrestriction); _visitables.add(this.serviceaccountrestriction);} return (A) this;
}
public Boolean hasServiceaccountrestriction(){
return this.serviceaccountrestriction!=null;
}
public RoleBindingRestrictionSpecFluent.ServiceaccountrestrictionNested withNewServiceaccountrestriction(){
return new ServiceaccountrestrictionNestedImpl();
}
public RoleBindingRestrictionSpecFluent.ServiceaccountrestrictionNested withNewServiceaccountrestrictionLike(ServiceAccountRestriction item){
return new ServiceaccountrestrictionNestedImpl(item);
}
public RoleBindingRestrictionSpecFluent.ServiceaccountrestrictionNested editServiceaccountrestriction(){
return withNewServiceaccountrestrictionLike(getServiceaccountrestriction());
}
public RoleBindingRestrictionSpecFluent.ServiceaccountrestrictionNested editOrNewServiceaccountrestriction(){
return withNewServiceaccountrestrictionLike(getServiceaccountrestriction() != null ? getServiceaccountrestriction(): new ServiceAccountRestrictionBuilder().build());
}
public RoleBindingRestrictionSpecFluent.ServiceaccountrestrictionNested editOrNewServiceaccountrestrictionLike(ServiceAccountRestriction item){
return withNewServiceaccountrestrictionLike(getServiceaccountrestriction() != null ? getServiceaccountrestriction(): item);
}
/**
* This method has been deprecated, please use method buildUserrestriction instead.
*/
@Deprecated public UserRestriction getUserrestriction(){
return this.userrestriction!=null?this.userrestriction.build():null;
}
public UserRestriction buildUserrestriction(){
return this.userrestriction!=null?this.userrestriction.build():null;
}
public A withUserrestriction(UserRestriction userrestriction){
_visitables.remove(this.userrestriction);
if (userrestriction!=null){ this.userrestriction= new UserRestrictionBuilder(userrestriction); _visitables.add(this.userrestriction);} return (A) this;
}
public Boolean hasUserrestriction(){
return this.userrestriction!=null;
}
public RoleBindingRestrictionSpecFluent.UserrestrictionNested withNewUserrestriction(){
return new UserrestrictionNestedImpl();
}
public RoleBindingRestrictionSpecFluent.UserrestrictionNested withNewUserrestrictionLike(UserRestriction item){
return new UserrestrictionNestedImpl(item);
}
public RoleBindingRestrictionSpecFluent.UserrestrictionNested editUserrestriction(){
return withNewUserrestrictionLike(getUserrestriction());
}
public RoleBindingRestrictionSpecFluent.UserrestrictionNested editOrNewUserrestriction(){
return withNewUserrestrictionLike(getUserrestriction() != null ? getUserrestriction(): new UserRestrictionBuilder().build());
}
public RoleBindingRestrictionSpecFluent.UserrestrictionNested editOrNewUserrestrictionLike(UserRestriction item){
return withNewUserrestrictionLike(getUserrestriction() != null ? getUserrestriction(): item);
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
RoleBindingRestrictionSpecFluentImpl that = (RoleBindingRestrictionSpecFluentImpl) o;
if (grouprestriction != null ? !grouprestriction.equals(that.grouprestriction) :that.grouprestriction != null) return false;
if (serviceaccountrestriction != null ? !serviceaccountrestriction.equals(that.serviceaccountrestriction) :that.serviceaccountrestriction != null) return false;
if (userrestriction != null ? !userrestriction.equals(that.userrestriction) :that.userrestriction != null) return false;
return true;
}
public class GrouprestrictionNestedImpl extends GroupRestrictionFluentImpl> implements RoleBindingRestrictionSpecFluent.GrouprestrictionNested,io.alauda.kubernetes.api.builder.Nested{
private final GroupRestrictionBuilder builder;
GrouprestrictionNestedImpl(GroupRestriction item){
this.builder = new GroupRestrictionBuilder(this, item);
}
GrouprestrictionNestedImpl(){
this.builder = new GroupRestrictionBuilder(this);
}
public N and(){
return (N) RoleBindingRestrictionSpecFluentImpl.this.withGrouprestriction(builder.build());
}
public N endGrouprestriction(){
return and();
}
}
public class ServiceaccountrestrictionNestedImpl extends ServiceAccountRestrictionFluentImpl> implements RoleBindingRestrictionSpecFluent.ServiceaccountrestrictionNested,io.alauda.kubernetes.api.builder.Nested{
private final ServiceAccountRestrictionBuilder builder;
ServiceaccountrestrictionNestedImpl(ServiceAccountRestriction item){
this.builder = new ServiceAccountRestrictionBuilder(this, item);
}
ServiceaccountrestrictionNestedImpl(){
this.builder = new ServiceAccountRestrictionBuilder(this);
}
public N and(){
return (N) RoleBindingRestrictionSpecFluentImpl.this.withServiceaccountrestriction(builder.build());
}
public N endServiceaccountrestriction(){
return and();
}
}
public class UserrestrictionNestedImpl extends UserRestrictionFluentImpl> implements RoleBindingRestrictionSpecFluent.UserrestrictionNested,io.alauda.kubernetes.api.builder.Nested{
private final UserRestrictionBuilder builder;
UserrestrictionNestedImpl(UserRestriction item){
this.builder = new UserRestrictionBuilder(this, item);
}
UserrestrictionNestedImpl(){
this.builder = new UserRestrictionBuilder(this);
}
public N and(){
return (N) RoleBindingRestrictionSpecFluentImpl.this.withUserrestriction(builder.build());
}
public N endUserrestriction(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy