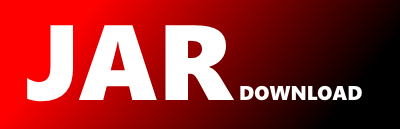
io.annot8.api.data.Item Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of annot8-api Show documentation
Show all versions of annot8-api Show documentation
Core API interfaces for the Annot8 project
/* Annot8 (annot8.io) - Licensed under Apache-2.0. */
package io.annot8.api.data;
import io.annot8.api.exceptions.UnsupportedContentException;
import io.annot8.api.filters.Filter;
import io.annot8.api.helpers.WithFilter;
import io.annot8.api.helpers.WithGroups;
import io.annot8.api.helpers.WithId;
import io.annot8.api.helpers.WithMutableProperties;
import java.util.Optional;
import java.util.stream.Stream;
/** Item interface used by components. */
public interface Item extends WithId, WithMutableProperties, WithGroups, WithFilter> {
/**
* Get id of parent
*
* @return id if exists
*/
Optional getParent();
/**
* Does this item have a parent?
*
* @return true if has a parent.
*/
default boolean hasParent() {
return getParent().isPresent();
}
/**
* The content object for the specified id
*
* @param id the content id
* @return the content if it exists
*/
Optional> getContent(final String id);
/**
* All content objects contained within this item
*
* @return all content
*/
Stream> getContents();
/**
* Filter content to match the test.
*
* @param filter the test to filter with
* @return stream of matching annotations
*/
default Stream> filter(Filter> filter) {
return getContents().filter(filter::test);
}
/**
* All content objects of the specified class contained within this item
*
* @param the content class
* @param clazz the content class to filter against
* @return content
*/
default > Stream getContents(final Class clazz) {
return getContents().filter(clazz::isInstance).map(clazz::cast);
}
/**
* Create a new content builder to generate content.
*
* @param the content class
* @param the data class
* @param clazz the top level content type required
* @return content builder
* @throws UnsupportedContentException if the clazz can't be created
*/
, D> Content.Builder createContent(Class clazz);
/**
* Remove the specified content object from this item
*
* @param id the content id
*/
void removeContent(final String id);
/**
* Remove the specified content object from this item
*
* @param content the content
*/
default void removeContent(final Content> content) {
if (content != null) {
removeContent(content.getId());
}
}
/**
* Stop processing this item any further.
*
* Note that it is up to the underlying implementation as to whether they delete existing
* output from this item or not.
*/
void discard();
/**
* If this item is to be discarded at the end of current processing
*
* @return true if discarded
*/
boolean isDiscarded();
/**
* Create a child item of this item, which will be processed independently
*
* @return new item, with this item as its parent
*/
Item createChild();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy